When to Consider Using Two Pointers in Array Problems
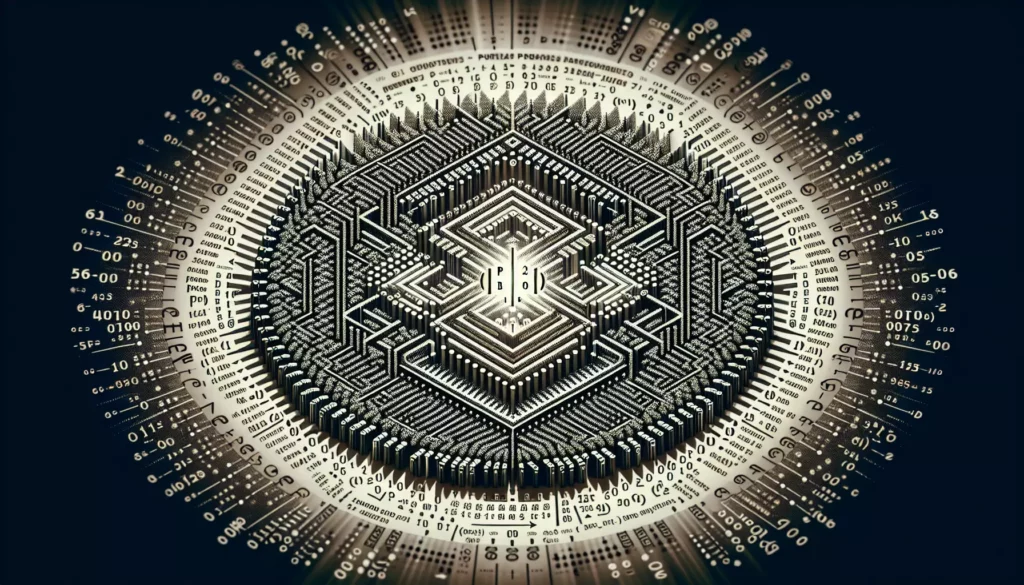
In the world of coding interviews and algorithmic problem-solving, efficiency is key. One technique that often proves invaluable when working with arrays is the “Two Pointers” approach. This method can significantly optimize your solutions, especially when dealing with sorted arrays or when you need to find pairs of elements that satisfy certain conditions. In this comprehensive guide, we’ll explore when and how to use the Two Pointers technique, providing you with the knowledge to ace your next coding interview or solve complex array problems with ease.
Understanding the Two Pointers Technique
Before we dive into specific scenarios, let’s establish a clear understanding of what the Two Pointers technique entails.
The Two Pointers technique, as the name suggests, involves using two pointers to traverse an array or sequence. These pointers can move towards each other, in the same direction, or even at different speeds. The key idea is to use these pointers to efficiently compare or manipulate elements within the array without the need for nested loops, thus often reducing the time complexity from O(n²) to O(n).
When to Consider Using Two Pointers
Now, let’s explore the specific scenarios where the Two Pointers technique shines:
1. Searching Pairs in a Sorted Array
When you have a sorted array and need to find a pair of elements that sum up to a target value, the Two Pointers technique is incredibly efficient.
Example Problem: Given a sorted array of integers, find two numbers such that they add up to a specific target number.
function findTwoSum(nums, target) {
let left = 0;
let right = nums.length - 1;
while (left < right) {
const sum = nums[left] + nums[right];
if (sum === target) {
return [nums[left], nums[right]];
} else if (sum < target) {
left++;
} else {
right--;
}
}
return null; // No solution found
}
const nums = [2, 7, 11, 15];
const target = 9;
console.log(findTwoSum(nums, target)); // Output: [2, 7]
In this example, we start with pointers at both ends of the array. We move the pointers based on whether the sum is greater or less than the target, efficiently narrowing down the search space.
2. Reversing Arrays or Strings
When you need to reverse an array or string in-place, Two Pointers is an excellent choice.
Example Problem: Reverse a string in-place.
function reverseString(s) {
let left = 0;
let right = s.length - 1;
while (left < right) {
// Swap characters
const temp = s[left];
s[left] = s[right];
s[right] = temp;
left++;
right--;
}
return s;
}
const str = ['h', 'e', 'l', 'l', 'o'];
console.log(reverseString(str)); // Output: ['o', 'l', 'l', 'e', 'h']
Here, we use two pointers starting from opposite ends of the string, swapping characters as we move towards the center.
3. Removing Duplicates from Sorted Arrays
The Two Pointers technique is particularly useful when you need to remove duplicates from a sorted array in-place.
Example Problem: Remove duplicates from a sorted array in-place and return the new length.
function removeDuplicates(nums) {
if (nums.length === 0) return 0;
let i = 0;
for (let j = 1; j < nums.length; j++) {
if (nums[j] !== nums[i]) {
i++;
nums[i] = nums[j];
}
}
return i + 1;
}
const nums = [1, 1, 2, 2, 3, 4, 4, 5];
console.log(removeDuplicates(nums)); // Output: 5
console.log(nums.slice(0, 5)); // Output: [1, 2, 3, 4, 5]
In this case, we use two pointers: one to keep track of the last unique element, and another to scan through the array.
4. Palindrome Checking
Two Pointers is an efficient way to check if a string is a palindrome.
Example Problem: Determine if a string is a palindrome, considering only alphanumeric characters and ignoring cases.
function isPalindrome(s) {
s = s.toLowerCase().replace(/[^a-z0-9]/g, '');
let left = 0;
let right = s.length - 1;
while (left < right) {
if (s[left] !== s[right]) {
return false;
}
left++;
right--;
}
return true;
}
console.log(isPalindrome("A man, a plan, a canal: Panama")); // Output: true
console.log(isPalindrome("race a car")); // Output: false
We use two pointers moving from both ends towards the center, comparing characters along the way.
5. Partitioning Arrays
The Two Pointers technique is valuable when you need to partition an array based on certain conditions.
Example Problem: Given an array of integers, partition it so that all even numbers come before odd numbers.
function partitionArray(nums) {
let left = 0;
let right = nums.length - 1;
while (left < right) {
while (left < right && nums[left] % 2 === 0) {
left++;
}
while (left < right && nums[right] % 2 !== 0) {
right--;
}
if (left < right) {
// Swap elements
[nums[left], nums[right]] = [nums[right], nums[left]];
left++;
right--;
}
}
return nums;
}
const nums = [1, 4, 3, 2, 5, 8, 7, 6];
console.log(partitionArray(nums)); // Output: [6, 4, 2, 8, 5, 3, 7, 1]
In this example, we use two pointers to swap elements, ensuring all even numbers are moved to the left side of the array.
6. Sliding Window Problems
While not strictly a Two Pointers technique, the Sliding Window approach often uses two pointers and is worth mentioning in this context.
Example Problem: Find the longest substring with at most k distinct characters.
function longestSubstringKDistinct(s, k) {
const charCount = new Map();
let left = 0;
let maxLength = 0;
for (let right = 0; right < s.length; right++) {
charCount.set(s[right], (charCount.get(s[right]) || 0) + 1);
while (charCount.size > k) {
charCount.set(s[left], charCount.get(s[left]) - 1);
if (charCount.get(s[left]) === 0) {
charCount.delete(s[left]);
}
left++;
}
maxLength = Math.max(maxLength, right - left + 1);
}
return maxLength;
}
console.log(longestSubstringKDistinct("aabacbebebe", 3)); // Output: 7
Here, we use two pointers to define a window that satisfies our condition (at most k distinct characters), adjusting the window as we traverse the string.
Benefits of Using Two Pointers
Now that we’ve seen various scenarios where Two Pointers can be applied, let’s discuss the benefits of this technique:
- Improved Time Complexity: Two Pointers often reduces the time complexity from O(n²) to O(n), making solutions much more efficient.
- Space Efficiency: Many Two Pointers solutions work in-place, requiring O(1) extra space.
- Simplicity: Once you understand the concept, Two Pointers solutions are often more straightforward and easier to implement than their nested-loop counterparts.
- Versatility: As we’ve seen, the technique can be applied to a wide range of array and string problems.
Common Pitfalls and How to Avoid Them
While the Two Pointers technique is powerful, there are some common mistakes to watch out for:
- Not Considering Edge Cases: Always test your solution with empty arrays, arrays with a single element, or other edge cases.
- Incorrect Pointer Movement: Ensure your pointers move correctly and don’t overlap when they shouldn’t.
- Forgetting to Check Bounds: Always make sure your pointers stay within the array bounds to avoid index out of range errors.
- Overusing the Technique: While powerful, Two Pointers isn’t always the best solution. Make sure to consider other approaches as well.
Practice Problems
To solidify your understanding of the Two Pointers technique, here are some practice problems you can try:
- Three Sum: Given an array of integers, find all unique triplets in the array which give the sum of zero.
- Container With Most Water: Given n non-negative integers representing an elevation map where the width of each bar is 1, compute how much water it can trap after raining.
- Sort Colors: Given an array with n objects colored red, white or blue, sort them in-place so that objects of the same color are adjacent, with the colors in the order red, white and blue.
- Minimum Window Substring: Given a string S and a string T, find the minimum window in S which will contain all the characters in T in complexity O(n).
- Longest Substring Without Repeating Characters: Given a string, find the length of the longest substring without repeating characters.
Conclusion
The Two Pointers technique is a powerful tool in any programmer’s arsenal, especially when tackling array and string problems. By understanding when and how to apply this technique, you can significantly improve the efficiency of your solutions and tackle a wide range of coding challenges with confidence.
Remember, like any programming concept, mastery comes with practice. As you work through more problems, you’ll develop an intuition for when Two Pointers might be the optimal approach. Keep coding, keep practicing, and soon you’ll find yourself naturally reaching for this technique when faced with suitable problems in coding interviews or real-world programming scenarios.
Happy coding, and may your pointers always point you in the right direction!