Techniques for Breaking Infinite Loops During Live Coding
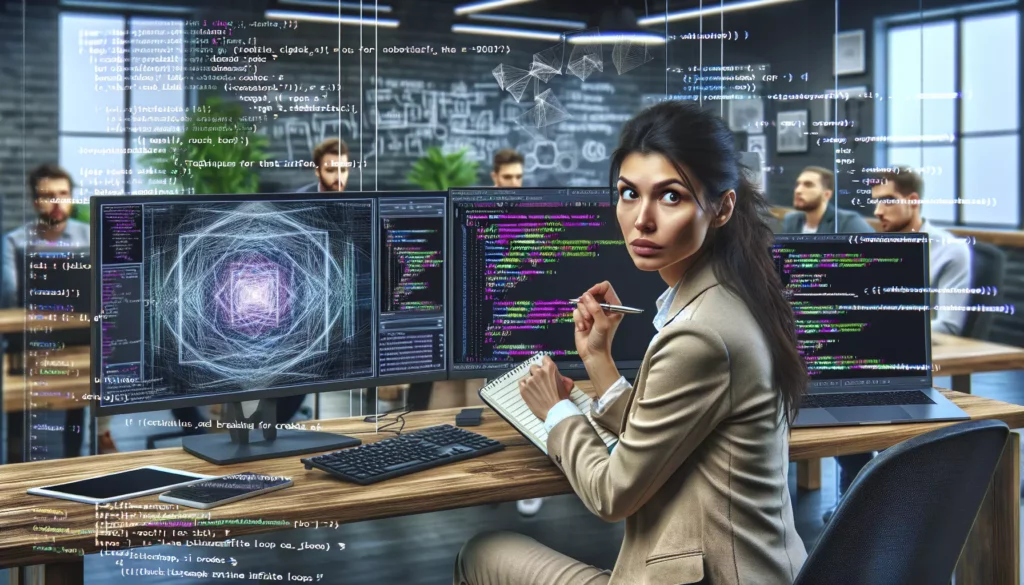
As a programmer, you’ve likely encountered the dreaded infinite loop at some point in your coding journey. These pesky bugs can be particularly frustrating during live coding sessions, technical interviews, or when you’re trying to demonstrate your skills in real-time. In this comprehensive guide, we’ll explore various techniques for identifying, preventing, and breaking infinite loops, ensuring you’re well-equipped to handle these situations with confidence.
Understanding Infinite Loops
Before we dive into the techniques for breaking infinite loops, let’s briefly review what an infinite loop is and why it occurs.
An infinite loop is a sequence of instructions in a program that repeats indefinitely. This happens when the loop’s termination condition is never met, causing the program to continue executing the same set of instructions over and over again. Infinite loops can cause your program to hang, consume excessive system resources, and potentially crash your development environment.
Common Causes of Infinite Loops
- Incorrect loop conditions
- Failure to update loop counters
- Logical errors in the loop body
- Unintended recursion without a proper base case
- Improper use of break statements
Techniques for Breaking Infinite Loops
Now that we understand what infinite loops are and why they occur, let’s explore various techniques to break out of them during live coding sessions.
1. Use Keyboard Shortcuts
One of the quickest ways to halt an infinite loop is by using keyboard shortcuts to stop the program’s execution. Familiarize yourself with the following shortcuts based on your development environment:
- Ctrl + C (Command + C on macOS): This universal shortcut terminates the current process in most command-line interfaces and integrated development environments (IDEs).
- Ctrl + Break (Windows): In some Windows environments, this combination can interrupt a running program.
- IDE-specific shortcuts: Many IDEs have their own shortcuts for stopping program execution. For example, in Visual Studio Code, you can use Shift + F5 to stop debugging.
2. Implement a Manual Break Condition
When writing loops, especially during live coding or debugging sessions, it’s helpful to include a manual break condition. This allows you to exit the loop after a certain number of iterations, preventing it from running indefinitely.
let counter = 0;
const MAX_ITERATIONS = 1000000;
while (true) {
// Your loop logic here
counter++;
if (counter >= MAX_ITERATIONS) {
console.log("Maximum iterations reached. Breaking loop.");
break;
}
}
This technique is particularly useful when you’re unsure about the loop’s termination condition or when you’re working with complex logic that might lead to unexpected infinite loops.
3. Use Timeouts
In scenarios where you’re dealing with asynchronous operations or when you want to limit the execution time of a potentially problematic loop, you can use timeouts. This technique involves setting a maximum time limit for the loop to execute.
const MAX_EXECUTION_TIME = 5000; // 5 seconds
const startTime = Date.now();
while (true) {
// Your loop logic here
if (Date.now() - startTime > MAX_EXECUTION_TIME) {
console.log("Maximum execution time reached. Breaking loop.");
break;
}
}
This approach ensures that your loop doesn’t run for longer than the specified time, preventing it from becoming an infinite loop.
4. Implement Watchdog Timers
A watchdog timer is a hardware or software mechanism that triggers a system reset or other corrective action if the main program fails to respond or refresh the watchdog within a specified time period. While typically used in embedded systems, you can implement a similar concept in your code to break potential infinite loops.
class Watchdog {
constructor(timeout) {
this.timeout = timeout;
this.timer = null;
}
start() {
this.timer = setTimeout(() => {
console.error("Watchdog timer expired. Breaking potential infinite loop.");
process.exit(1);
}, this.timeout);
}
reset() {
clearTimeout(this.timer);
this.start();
}
stop() {
clearTimeout(this.timer);
}
}
const watchdog = new Watchdog(5000); // 5 seconds timeout
watchdog.start();
while (true) {
// Your loop logic here
// Reset the watchdog timer if the loop is still running correctly
watchdog.reset();
}
// Don't forget to stop the watchdog when you're done
watchdog.stop();
This watchdog implementation allows you to set a timeout for your loop. If the loop doesn’t reset the watchdog timer within the specified time, it will trigger an error and exit the program.
5. Use Debugger Breakpoints
When coding in an IDE, you can leverage the power of debuggers to help identify and break infinite loops. Set strategic breakpoints in your code, especially at the beginning and end of loop bodies. This allows you to step through the code execution and observe variable values, helping you pinpoint the cause of the infinite loop.
Here’s how you can use breakpoints effectively:
- Set a breakpoint at the start of the loop.
- Run the code in debug mode.
- When the debugger hits the breakpoint, step through the code line by line.
- Observe the values of loop variables and conditions.
- If you notice the loop continuing unexpectedly, you can manually break the execution or modify variables to exit the loop.
6. Implement Logging and Output
Adding strategic logging statements within your loop can help you identify when a loop is running longer than expected. By outputting relevant information at each iteration, you can track the loop’s progress and potentially identify the cause of the infinite behavior.
let i = 0;
while (i < 10) {
console.log(`Iteration ${i}: Current value of i is ${i}`);
// Your loop logic here
i++;
}
If you see the same values being logged repeatedly or if the output continues indefinitely, it’s a clear sign that you’ve encountered an infinite loop.
7. Use Exception Handling
In some cases, you can use exception handling to break out of potential infinite loops. This technique involves wrapping your loop in a try-catch block and throwing an exception when certain conditions are met.
try {
let i = 0;
while (true) {
// Your loop logic here
i++;
if (i > 1000000) {
throw new Error("Potential infinite loop detected");
}
}
} catch (error) {
console.error("Loop terminated:", error.message);
}
This approach allows you to gracefully exit the loop and handle the error condition appropriately.
Preventing Infinite Loops
While knowing how to break infinite loops is crucial, it’s even better to prevent them from occurring in the first place. Here are some best practices to help you avoid infinite loops:
1. Double-Check Loop Conditions
Always verify that your loop conditions are correctly formulated. Ensure that the condition will eventually evaluate to false, allowing the loop to terminate.
// Incorrect (potential infinite loop)
while (x != 10) {
x += 2;
}
// Correct
while (x < 10) {
x += 2;
}
2. Use For Loops When Possible
For loops provide a clear structure for iteration, making it less likely to introduce infinite loops accidentally. They explicitly define the initialization, condition, and increment/decrement steps.
for (let i = 0; i < 10; i++) {
// Loop body
}
3. Be Cautious with While True Loops
While while (true)
loops have their uses, they require careful handling to ensure proper termination. Always include a clear exit condition within the loop body.
while (true) {
// Loop logic
if (exitCondition) {
break;
}
}
4. Review Recursive Functions
When working with recursive functions, ensure that you have a proper base case that will eventually be reached. Without a valid base case, recursion can lead to stack overflow errors or infinite loops.
function factorial(n) {
// Base case
if (n === 0 || n === 1) {
return 1;
}
// Recursive case
return n * factorial(n - 1);
}
5. Use Code Review and Pair Programming
Collaborating with other developers through code reviews or pair programming can help catch potential infinite loops before they make it into production. A fresh pair of eyes can often spot issues that you might have overlooked.
Handling Infinite Loops in Different Programming Languages
While the general principles for breaking infinite loops are similar across programming languages, there are some language-specific considerations and techniques you should be aware of:
Python
In Python, you can use the KeyboardInterrupt
exception to break out of infinite loops:
try:
while True:
# Your loop logic here
pass
except KeyboardInterrupt:
print("Loop interrupted by user")
JavaScript
For browser-based JavaScript, you can use the window.stop()
method to stop the execution of an infinite loop:
setTimeout(() => {
window.stop();
console.log("Execution stopped after timeout");
}, 5000);
while (true) {
// Your loop logic here
}
Java
In Java, you can use thread interrupts to break infinite loops in multi-threaded applications:
class InfiniteLoopExample implements Runnable {
public void run() {
try {
while (true) {
// Your loop logic here
if (Thread.interrupted()) {
throw new InterruptedException();
}
}
} catch (InterruptedException e) {
System.out.println("Loop interrupted");
}
}
}
public class Main {
public static void main(String[] args) throws InterruptedException {
Thread t = new Thread(new InfiniteLoopExample());
t.start();
Thread.sleep(5000); // Wait for 5 seconds
t.interrupt(); // Interrupt the thread
}
}
Advanced Techniques for Complex Scenarios
In more complex programming scenarios, you might encounter situations where simple loop breaking techniques are not sufficient. Here are some advanced approaches to consider:
1. Implement a State Machine
For complex algorithms or game loops, implementing a state machine can help you manage different stages of execution and provide clear exit points:
enum State {
INITIALIZING,
RUNNING,
PAUSED,
COMPLETED
}
let currentState = State.INITIALIZING;
function runStateMachine() {
while (currentState !== State.COMPLETED) {
switch (currentState) {
case State.INITIALIZING:
// Initialization logic
currentState = State.RUNNING;
break;
case State.RUNNING:
// Main loop logic
if (/* completion condition */) {
currentState = State.COMPLETED;
}
break;
case State.PAUSED:
// Paused state logic
break;
}
}
}
2. Use Worker Threads or Child Processes
For potentially long-running or computationally intensive tasks, consider using worker threads or child processes. This allows you to run the loop in a separate thread or process, making it easier to monitor and terminate if necessary:
const { Worker, isMainThread, parentPort } = require('worker_threads');
if (isMainThread) {
const worker = new Worker(__filename);
worker.on('message', (msg) => {
console.log('Message from worker:', msg);
});
setTimeout(() => {
worker.terminate();
console.log('Worker terminated');
}, 5000);
} else {
// This code runs in the worker thread
while (true) {
// Your loop logic here
parentPort.postMessage('Still running...');
}
}
3. Implement Checkpointing
For long-running processes or algorithms that may take a significant amount of time, implement checkpointing. This allows you to save the current state of the computation periodically, so you can resume from the last checkpoint if the process is interrupted:
function longRunningAlgorithm() {
let state = loadCheckpoint() || { iteration: 0, result: initialValue };
while (state.iteration < MAX_ITERATIONS) {
// Perform computation
state.result = computeNextStep(state.result);
state.iteration++;
if (state.iteration % CHECKPOINT_INTERVAL === 0) {
saveCheckpoint(state);
}
if (shouldTerminate()) {
break;
}
}
return state.result;
}
function saveCheckpoint(state) {
// Save state to disk or database
}
function loadCheckpoint() {
// Load previously saved state
}
function shouldTerminate() {
// Check for termination conditions
}
Conclusion
Mastering the techniques for breaking infinite loops is an essential skill for any programmer, especially when faced with live coding scenarios or technical interviews. By understanding the causes of infinite loops and implementing preventive measures, you can write more robust and efficient code.
Remember to:
- Always have a clear exit condition for your loops
- Use debugging tools and logging to identify issues early
- Implement safeguards like manual break conditions and timeouts
- Practice these techniques regularly to build muscle memory
- Stay calm and methodical when encountering infinite loops during live coding sessions
By incorporating these strategies into your coding practice, you’ll be better prepared to handle infinite loops and other challenging programming scenarios. Keep practicing, stay curious, and happy coding!