How to Write Pseudocode That Impresses Interviewers
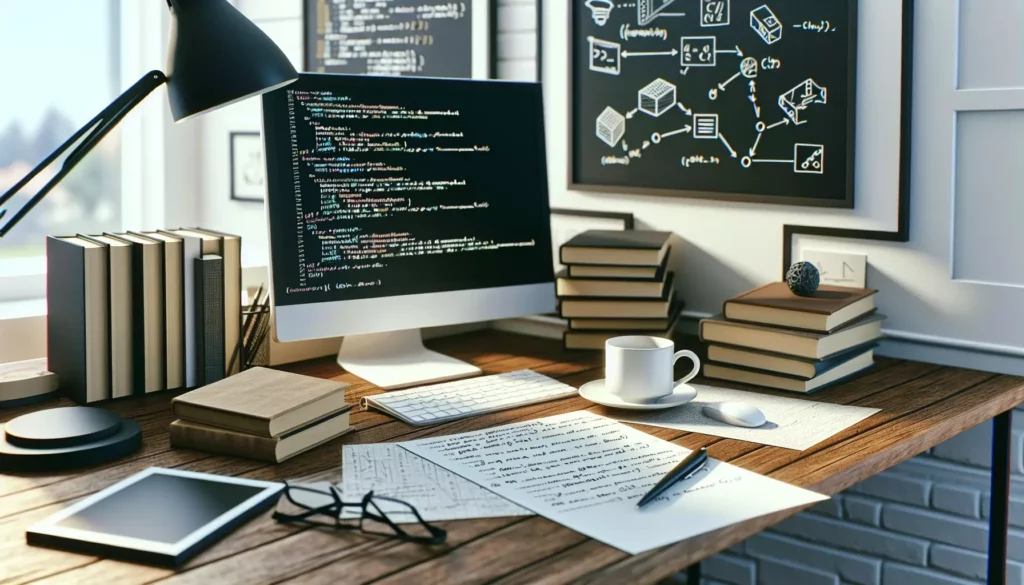
In the competitive world of tech interviews, particularly for coveted positions at FAANG companies (Facebook, Amazon, Apple, Netflix, and Google), your ability to communicate your problem-solving process is just as crucial as your coding skills. One powerful tool in your arsenal is pseudocode. When used effectively, pseudocode can demonstrate your algorithmic thinking, showcase your problem-solving approach, and impress your interviewers. In this comprehensive guide, we’ll explore the art of writing pseudocode that not only solves problems but also leaves a lasting impression on your interviewers.
What is Pseudocode?
Before diving into the techniques of writing impressive pseudocode, let’s establish a clear understanding of what pseudocode is and why it’s valuable in technical interviews.
Pseudocode is a informal, high-level description of a computer program or algorithm. It uses structural conventions of a normal programming language but is intended for human reading rather than machine reading. Essentially, it’s a bridge between human thinking and computer code.
Key characteristics of pseudocode:
- Written in plain English (or your native language)
- Uses simple, concise statements to describe operations
- Employs indentation to show structure and hierarchy
- Is not bound by strict syntax rules of any particular programming language
- Focuses on logic and flow rather than implementation details
Why Pseudocode Matters in Interviews
In a technical interview, especially for roles at major tech companies, your interviewer is interested in more than just your ability to write code. They want to understand your thought process, problem-solving approach, and communication skills. Pseudocode serves several crucial purposes in this context:
- Demonstrates logical thinking: It shows how you break down complex problems into manageable steps.
- Facilitates communication: It allows you to explain your approach clearly before diving into actual code.
- Reveals problem-solving skills: Your pseudocode can showcase how you handle edge cases, optimize for efficiency, and consider different scenarios.
- Saves time: Writing pseudocode first can help you avoid syntax errors and logical mistakes when you move to actual coding.
- Shows adaptability: Good pseudocode is language-agnostic, demonstrating your ability to think in algorithmic terms regardless of the specific programming language used.
Techniques for Writing Impressive Pseudocode
Now that we understand the importance of pseudocode in interviews, let’s explore techniques to elevate your pseudocode game and impress your interviewers.
1. Start with a Clear Problem Statement
Begin your pseudocode by clearly stating the problem you’re solving. This shows the interviewer that you’ve understood the task at hand and sets the context for your solution.
// Problem: Find the maximum sum of a contiguous subarray within a one-dimensional array of numbers (Kadane's Algorithm)
// Input: An array of integers
// Output: The maximum sum of any contiguous subarray
2. Use Descriptive Variable Names
Choose variable names that clearly convey their purpose. This makes your pseudocode more readable and demonstrates your ability to write clean, self-documenting code.
max_sum = negative infinity
current_sum = 0
for each number in array:
// Your logic here
3. Employ Consistent Indentation
Use indentation to show the structure of your algorithm, particularly for loops, conditionals, and function definitions. This visual hierarchy makes your pseudocode easier to follow.
function find_max_subarray_sum(array):
max_sum = negative infinity
current_sum = 0
for each number in array:
if current_sum + number > number:
current_sum = current_sum + number
else:
current_sum = number
if current_sum > max_sum:
max_sum = current_sum
return max_sum
4. Break Down Complex Operations
For complex algorithms, break down the operations into smaller, manageable steps. This demonstrates your ability to tackle complex problems methodically.
// Step 1: Initialize variables
initialize max_sum to negative infinity
initialize current_sum to 0
// Step 2: Iterate through the array
for each number in array:
// Step 3: Update current sum
current_sum = max(number, current_sum + number)
// Step 4: Update max sum if necessary
max_sum = max(max_sum, current_sum)
// Step 5: Return the result
return max_sum
5. Include Comments for Clarity
Use comments to explain the purpose of specific sections or to clarify complex logic. This shows your commitment to writing understandable code and your ability to communicate your thought process.
function find_max_subarray_sum(array):
max_sum = negative infinity
current_sum = 0
for each number in array:
// Choose between starting a new subarray or extending the existing one
current_sum = max(number, current_sum + number)
// Update the maximum sum if the current sum is greater
max_sum = max(max_sum, current_sum)
// The maximum sum represents the largest sum of any contiguous subarray
return max_sum
6. Address Edge Cases
Demonstrate your thoroughness by addressing edge cases in your pseudocode. This shows that you consider various scenarios and don’t just focus on the happy path.
function find_max_subarray_sum(array):
// Edge case: Empty array
if array is empty:
return 0 // or throw an exception, depending on requirements
max_sum = array[0] // Initialize with the first element
current_sum = array[0]
for i = 1 to length of array - 1:
// Your main logic here
7. Optimize for Readability and Efficiency
While pseudocode doesn’t need to be as efficient as actual code, showing consideration for performance can impress interviewers. Balance readability with hints at efficiency.
function find_max_subarray_sum(array):
max_sum = current_sum = array[0] // Initialize both in one line
for number in array[1:]: // Start from the second element
current_sum = max(number, current_sum + number)
max_sum = max(max_sum, current_sum)
return max_sum
8. Use Appropriate Level of Abstraction
Adjust the level of detail in your pseudocode based on the complexity of the problem and the interviewer’s expectations. For simpler problems, you might use more abstract descriptions, while for complex algorithms, you might need more detailed steps.
Abstract version:
function find_max_subarray_sum(array):
initialize variables
iterate through array:
update current sum
update max sum if necessary
return max sum
More detailed version:
function find_max_subarray_sum(array):
max_sum = negative infinity
current_sum = 0
for each number in array:
current_sum = max(number, current_sum + number)
max_sum = max(max_sum, current_sum)
return max_sum
9. Incorporate Error Handling
Show your attention to robustness by including basic error handling in your pseudocode. This demonstrates that you consider potential issues and edge cases.
function find_max_subarray_sum(array):
if array is null or empty:
throw InvalidInputException("Array must contain at least one element")
max_sum = current_sum = array[0]
for number in array[1:]:
current_sum = max(number, current_sum + number)
max_sum = max(max_sum, current_sum)
return max_sum
10. Use Consistent Formatting
Maintain consistent formatting throughout your pseudocode. This includes consistent use of indentation, capitalization, and notation for operations. Consistency demonstrates attention to detail and makes your pseudocode more professional.
FUNCTION FindMaxSubarraySum(array)
IF array IS NULL OR array.Length = 0 THEN
THROW InvalidInputException("Array must contain at least one element")
END IF
maxSum <- array[0]
currentSum <- array[0]
FOR i <- 1 TO array.Length - 1
currentSum <- MAX(array[i], currentSum + array[i])
maxSum <- MAX(maxSum, currentSum)
END FOR
RETURN maxSum
END FUNCTION
Common Pitfalls to Avoid
While striving to write impressive pseudocode, be aware of these common pitfalls that can detract from your presentation:
- Over-complication: Don’t make your pseudocode unnecessarily complex. The goal is clarity and effectiveness, not to showcase every programming concept you know.
- Ignoring time and space complexity: While pseudocode doesn’t need to be as optimized as actual code, completely disregarding efficiency can be a red flag for interviewers.
- Being too language-specific: Avoid using syntax or constructs that are specific to a particular programming language. Keep it general and adaptable.
- Lack of structure: Poorly structured pseudocode can be as confusing as poorly written code. Maintain a clear, logical flow.
- Inconsistency: Switching styles or conventions midway through your pseudocode can make it harder to follow. Stick to one approach throughout.
Practicing Pseudocode for Interviews
To excel at writing pseudocode during interviews, regular practice is key. Here are some strategies to improve your pseudocode skills:
- Solve algorithm problems: Use platforms like AlgoCademy, LeetCode, or HackerRank to practice solving algorithm problems. Before coding, write out your solution in pseudocode.
- Review and refine: After solving a problem, review your pseudocode. Look for ways to make it clearer, more concise, or more efficient.
- Study good examples: Look at pseudocode examples in algorithm textbooks or online resources. Analyze what makes them effective and incorporate those elements into your own style.
- Practice explaining: Get used to explaining your pseudocode out loud, as you would in an interview. This helps you identify areas where your logic might be unclear.
- Time yourself: In interviews, you’ll be under time pressure. Practice writing pseudocode quickly while maintaining quality.
- Peer review: If possible, have peers or mentors review your pseudocode. Fresh eyes can often spot areas for improvement that you might miss.
Adapting Your Pseudocode Style
While there are general best practices for writing pseudocode, it’s important to be adaptable. Different companies, interviewers, or even specific problems might call for slight variations in your approach. Here are some tips for adapting your pseudocode style:
- Read the room: Pay attention to how your interviewer responds to your initial pseudocode. If they seem to prefer more or less detail, adjust accordingly.
- Ask for preferences: It’s perfectly acceptable to ask your interviewer if they have any preferences for how you present your pseudocode.
- Match the problem complexity: For simpler problems, your pseudocode can be more high-level. For complex algorithms, you might need to be more detailed.
- Consider the company culture: If you’re interviewing for a company known for its focus on efficiency, you might want to emphasize optimization in your pseudocode.
- Be ready to translate: Sometimes, an interviewer might ask you to convert your pseudocode into actual code. Practice making this transition smoothly.
Conclusion
Mastering the art of writing impressive pseudocode is a valuable skill that can set you apart in technical interviews, especially for positions at top tech companies. By following the techniques outlined in this guide and avoiding common pitfalls, you can demonstrate your problem-solving skills, logical thinking, and ability to communicate complex ideas effectively.
Remember, the goal of pseudocode in an interview is not just to solve the problem, but to showcase your thought process and approach. It’s a powerful tool that, when used correctly, can give interviewers insight into your potential as a developer beyond just your coding skills.
As you prepare for your interviews, make writing clear, concise, and effective pseudocode a key part of your practice routine. With time and effort, you’ll develop a style that not only solves problems efficiently but also impresses interviewers and increases your chances of landing your dream job in the competitive world of tech.
Keep practicing, stay adaptable, and approach each problem with the mindset of not just solving it, but communicating your solution effectively. Your pseudocode can be the key that unlocks doors to exciting opportunities in your programming career.