Identifying When Dynamic Programming Can Optimize Your Solution
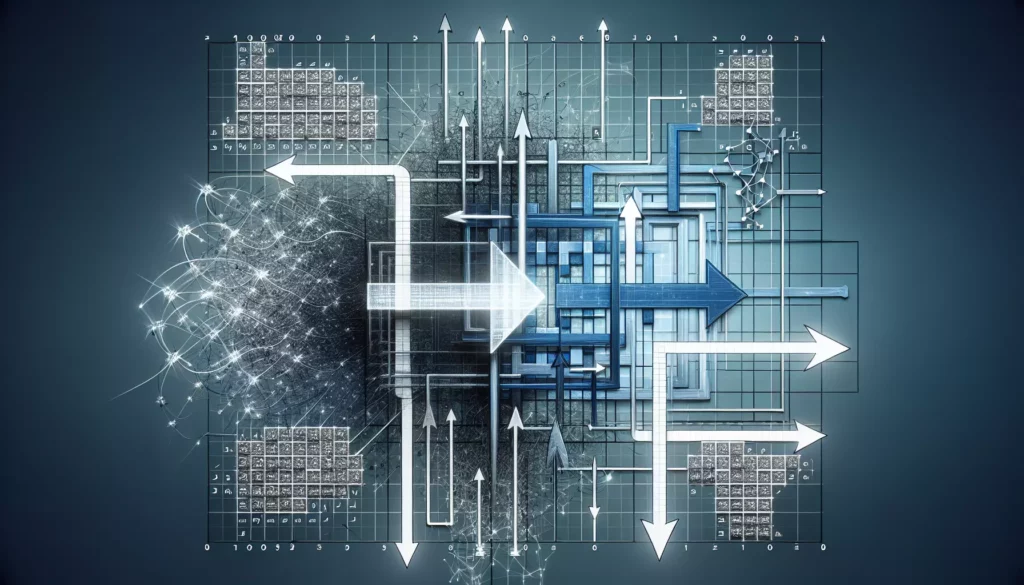
Dynamic programming (DP) is a powerful algorithmic technique that can significantly optimize solutions to complex problems. However, recognizing when to apply DP isn’t always straightforward. In this comprehensive guide, we’ll explore how to identify scenarios where dynamic programming can enhance your solution, providing you with the skills to tackle challenging coding problems more efficiently.
Understanding Dynamic Programming
Before diving into identification strategies, let’s briefly review what dynamic programming is and how it works.
What is Dynamic Programming?
Dynamic programming is a method for solving complex problems by breaking them down into simpler subproblems. It is applicable when the problem has the following characteristics:
- Overlapping subproblems: The same subproblems are solved multiple times.
- Optimal substructure: An optimal solution to the problem can be constructed from optimal solutions of its subproblems.
DP solutions typically involve storing the results of subproblems to avoid redundant computations, a technique known as memoization.
Key Indicators for Dynamic Programming
Now, let’s explore the telltale signs that a problem might benefit from a dynamic programming approach.
1. Optimization Problems
DP is often used for optimization problems where you need to find the best solution among many possible ones. Look for keywords like:
- Maximize or minimize
- Longest or shortest
- Least or most
- Maximum or minimum
Example: “Find the longest increasing subsequence in an array.”
2. Counting Problems
Problems that ask to count the number of ways to do something are often good candidates for DP. Watch for phrases like:
- How many ways…?
- Count the number of…
- In how many different ways…?
Example: “Count the number of ways to reach the top of n stairs if you can climb 1 or 2 stairs at a time.”
3. Recursive Problems with Repetitive Calculations
If you notice that a recursive solution to a problem involves many repeated calculations, it’s a strong indicator that DP can be applied to optimize it.
Example: The naive recursive solution for calculating Fibonacci numbers.
4. Problems with Choices at Each Step
When a problem involves making a series of interconnected decisions, each affecting future choices, DP might be applicable.
Example: “Given a set of items with weights and values, maximize the value that can be put in a knapsack of capacity W.”
5. Grid Traversal Problems
Problems involving movement through a grid, especially when finding optimal paths, are often solvable with DP.
Example: “Find the minimum path sum from the top-left to the bottom-right of a grid.”
6. String Manipulation Problems
Many string-related problems, especially those involving subsequences or substrings, can be efficiently solved using DP.
Example: “Find the length of the longest common subsequence between two strings.”
Practical Examples
Let’s examine some concrete examples to illustrate how to identify and apply dynamic programming.
Example 1: Fibonacci Sequence
The Fibonacci sequence is a classic example where DP can significantly optimize the solution.
Problem: Calculate the nth Fibonacci number.
Naive recursive approach:
def fibonacci(n):
if n <= 1:
return n
return fibonacci(n-1) + fibonacci(n-2)
This solution has a time complexity of O(2^n), which is extremely inefficient for large n.
DP approach:
def fibonacci_dp(n):
if n <= 1:
return n
dp = [0] * (n + 1)
dp[1] = 1
for i in range(2, n + 1):
dp[i] = dp[i-1] + dp[i-2]
return dp[n]
The DP solution has a time complexity of O(n), a significant improvement.
Example 2: Longest Increasing Subsequence
Problem: Find the length of the longest increasing subsequence in an array.
This problem exhibits optimal substructure and overlapping subproblems, making it ideal for DP.
def longest_increasing_subsequence(arr):
n = len(arr)
dp = [1] * n
for i in range(1, n):
for j in range(i):
if arr[i] > arr[j]:
dp[i] = max(dp[i], dp[j] + 1)
return max(dp)
This solution has a time complexity of O(n^2), which is much better than the exponential time complexity of a naive recursive approach.
Example 3: Knapsack Problem
Problem: Given weights and values of n items, put these items in a knapsack of capacity W to get the maximum total value in the knapsack.
This is a classic optimization problem that can be solved efficiently using DP.
def knapsack(W, wt, val, n):
K = [[0 for x in range(W + 1)] for x in range(n + 1)]
for i in range(n + 1):
for w in range(W + 1):
if i == 0 or w == 0:
K[i][w] = 0
elif wt[i-1] <= w:
K[i][w] = max(val[i-1] + K[i-1][w-wt[i-1]], K[i-1][w])
else:
K[i][w] = K[i-1][w]
return K[n][W]
This DP solution has a time complexity of O(nW), which is pseudo-polynomial and much more efficient than the exponential time complexity of a naive recursive approach.
Common Patterns in Dynamic Programming
As you encounter more DP problems, you’ll start to recognize common patterns. Here are some frequently occurring patterns:
1. Linear DP
In linear DP, the solution is built in a one-dimensional array. The Fibonacci sequence example above is a case of linear DP.
2. Matrix DP
Matrix DP uses a two-dimensional array to store solutions to subproblems. The knapsack problem solution above is an example of matrix DP.
3. State Reduction
Sometimes, you can optimize space complexity by reducing the number of states you need to store. For example, in the Fibonacci sequence, you only need the last two numbers to calculate the next one.
def fibonacci_optimized(n):
if n <= 1:
return n
a, b = 0, 1
for _ in range(2, n + 1):
a, b = b, a + b
return b
4. Interval DP
Interval DP is used when the problem involves intervals or subarrays. The longest palindromic subsequence problem is a good example of interval DP.
5. Tree DP
Tree DP is applied to problems involving tree structures. For example, finding the maximum path sum in a binary tree.
Steps to Approach a DP Problem
When you suspect a problem might be solvable with DP, follow these steps:
- Identify the subproblems and define the state clearly.
- Establish the recurrence relation between subproblems.
- Identify the base cases.
- Decide on a bottom-up or top-down approach.
- Implement the solution, ensuring you’re storing and reusing subproblem results.
- Optimize for space if necessary.
Common Pitfalls and How to Avoid Them
Even when you’ve identified that a problem can be solved with DP, there are some common mistakes to watch out for:
1. Incorrect State Definition
Ensure your state captures all necessary information to solve subproblems. Missing crucial information in your state definition can lead to incorrect solutions.
2. Overlooking Base Cases
Always carefully consider and handle base cases. They’re crucial for the correctness of your DP solution.
3. Inefficient State Transitions
Try to minimize the number of state transitions. Inefficient transitions can lead to suboptimal time complexity.
4. Not Memoizing Results
In top-down (recursive) DP, always remember to memoize results to avoid redundant calculations.
5. Unnecessary Computation
In bottom-up DP, make sure you’re not computing unnecessary states. Only compute what’s needed for the final solution.
Advanced DP Techniques
As you become more comfortable with basic DP, you can explore more advanced techniques:
1. Bitmasking DP
Bitmasking can be combined with DP to solve problems involving subsets efficiently.
2. DP on Trees
DP can be applied to tree structures, often involving post-order traversal.
3. Digit DP
Digit DP is used to solve problems involving digit properties of numbers in a given range.
4. DP with Probability
Some problems involve calculating probabilities, where DP can be applied to compute expected values.
Conclusion
Identifying when to use dynamic programming is a skill that develops with practice. By recognizing the key indicators and common patterns we’ve discussed, you’ll be better equipped to tackle complex problems efficiently. Remember, the essence of DP lies in breaking down problems into manageable subproblems and avoiding redundant computations.
As you continue your journey in algorithmic problem-solving, keep an eye out for problems that exhibit overlapping subproblems and optimal substructure. With time and practice, applying DP will become second nature, allowing you to optimize your solutions and tackle even more challenging coding problems.
Happy coding, and may your solutions always be optimally dynamic!