When to Use a Stack vs Queue: Decision-Making in Interviews
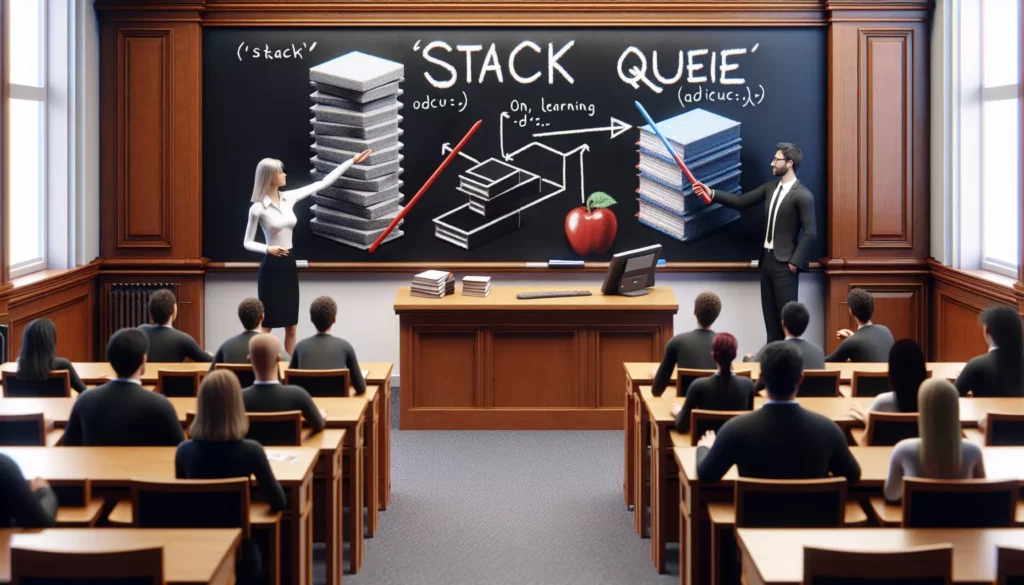
In the world of data structures and algorithms, stacks and queues are fundamental concepts that every programmer should master. These structures are not only crucial for solving various coding problems but are also frequent topics in technical interviews, especially for positions at major tech companies. Understanding when to use a stack versus a queue can significantly impact your problem-solving approach and the efficiency of your solutions. In this comprehensive guide, we’ll explore the characteristics of stacks and queues, their implementations, and most importantly, when to choose one over the other in different scenarios.
Understanding Stacks and Queues
Before we dive into the decision-making process, let’s briefly review what stacks and queues are and their basic operations.
What is a Stack?
A stack is a linear data structure that follows the Last-In-First-Out (LIFO) principle. This means that the last element added to the stack will be the first one to be removed. Think of a stack of plates – you add plates to the top and remove them from the top.
The main operations of a stack are:
- Push: Add an element to the top of the stack
- Pop: Remove the top element from the stack
- Peek or Top: View the top element without removing it
- IsEmpty: Check if the stack is empty
What is a Queue?
A queue is a linear data structure that follows the First-In-First-Out (FIFO) principle. This means that the first element added to the queue will be the first one to be removed. Think of a queue of people waiting in line – the first person to join the line is the first to be served.
The main operations of a queue are:
- Enqueue: Add an element to the rear of the queue
- Dequeue: Remove the front element from the queue
- Front: View the front element without removing it
- IsEmpty: Check if the queue is empty
Implementing Stacks and Queues
Before we discuss when to use each structure, let’s look at basic implementations in Python to better understand their behavior.
Implementing a Stack
Here’s a simple implementation of a stack using a Python list:
class Stack:
def __init__(self):
self.items = []
def push(self, item):
self.items.append(item)
def pop(self):
if not self.is_empty():
return self.items.pop()
def peek(self):
if not self.is_empty():
return self.items[-1]
def is_empty(self):
return len(self.items) == 0
def size(self):
return len(self.items)
# Usage example
stack = Stack()
stack.push(1)
stack.push(2)
stack.push(3)
print(stack.pop()) # Output: 3
print(stack.peek()) # Output: 2
print(stack.size()) # Output: 2
Implementing a Queue
Here’s a simple implementation of a queue using a Python list:
class Queue:
def __init__(self):
self.items = []
def enqueue(self, item):
self.items.append(item)
def dequeue(self):
if not self.is_empty():
return self.items.pop(0)
def front(self):
if not self.is_empty():
return self.items[0]
def is_empty(self):
return len(self.items) == 0
def size(self):
return len(self.items)
# Usage example
queue = Queue()
queue.enqueue(1)
queue.enqueue(2)
queue.enqueue(3)
print(queue.dequeue()) # Output: 1
print(queue.front()) # Output: 2
print(queue.size()) # Output: 2
When to Use a Stack
Now that we understand the basics of stacks and queues, let’s explore scenarios where using a stack is the optimal choice. During interviews, recognizing these patterns can help you quickly identify when to reach for a stack-based solution.
1. Function Call Management and Recursion
One of the most common uses of stacks is in managing function calls and recursion. When a function is called, the computer uses a stack to keep track of where to return after the function completes. This is known as the call stack.
Example scenario: Implementing a depth-first search (DFS) algorithm for traversing or searching tree or graph data structures.
def dfs(graph, start, visited=None):
if visited is None:
visited = set()
visited.add(start)
print(start) # Process the current node
for neighbor in graph[start]:
if neighbor not in visited:
dfs(graph, neighbor, visited)
# Example usage
graph = {
'A': ['B', 'C'],
'B': ['A', 'D', 'E'],
'C': ['A', 'F'],
'D': ['B'],
'E': ['B', 'F'],
'F': ['C', 'E']
}
dfs(graph, 'A')
In this example, the recursive calls to dfs
utilize the system’s call stack to keep track of the nodes to visit.
2. Expression Evaluation and Syntax Parsing
Stacks are excellent for evaluating expressions, especially those involving nested structures like parentheses. They’re also used in syntax parsing for programming languages.
Example scenario: Evaluating a postfix expression.
def evaluate_postfix(expression):
stack = []
for char in expression:
if char.isdigit():
stack.append(int(char))
else:
b = stack.pop()
a = stack.pop()
if char == '+':
stack.append(a + b)
elif char == '-':
stack.append(a - b)
elif char == '*':
stack.append(a * b)
elif char == '/':
stack.append(a / b)
return stack.pop()
# Example usage
expression = "23*5+"
result = evaluate_postfix(expression)
print(result) # Output: 11
3. Undo Mechanisms
Stacks are perfect for implementing undo functionality in applications. Each action is pushed onto a stack, and undoing simply means popping the last action off the stack.
Example scenario: Implementing a simple text editor with undo capability.
class TextEditor:
def __init__(self):
self.text = ""
self.undo_stack = []
def insert(self, string):
self.undo_stack.append(self.text)
self.text += string
def delete(self, num_chars):
if num_chars <= len(self.text):
self.undo_stack.append(self.text)
self.text = self.text[:-num_chars]
def undo(self):
if self.undo_stack:
self.text = self.undo_stack.pop()
# Example usage
editor = TextEditor()
editor.insert("Hello")
editor.insert(" World")
editor.delete(3)
print(editor.text) # Output: Hello Wo
editor.undo()
print(editor.text) # Output: Hello World
4. Balancing Symbols
Stacks are ideal for checking balanced symbols, such as parentheses, brackets, and braces in code or mathematical expressions.
Example scenario: Checking if parentheses are balanced in an expression.
def are_parentheses_balanced(expression):
stack = []
for char in expression:
if char == '(':
stack.append(char)
elif char == ')':
if not stack:
return False
stack.pop()
return len(stack) == 0
# Example usage
print(are_parentheses_balanced("((()))")) # Output: True
print(are_parentheses_balanced("(()")) # Output: False
When to Use a Queue
Queues have their own set of scenarios where they shine. Let’s explore when you should opt for a queue-based solution during your coding interviews.
1. Breadth-First Search (BFS)
Queues are fundamental in implementing breadth-first search algorithms for traversing or searching tree or graph data structures.
Example scenario: Implementing BFS for a graph.
from collections import deque
def bfs(graph, start):
visited = set()
queue = deque([start])
visited.add(start)
while queue:
vertex = queue.popleft()
print(vertex) # Process the current node
for neighbor in graph[vertex]:
if neighbor not in visited:
visited.add(neighbor)
queue.append(neighbor)
# Example usage
graph = {
'A': ['B', 'C'],
'B': ['A', 'D', 'E'],
'C': ['A', 'F'],
'D': ['B'],
'E': ['B', 'F'],
'F': ['C', 'E']
}
bfs(graph, 'A')
2. Task Scheduling
Queues are perfect for managing tasks or processes that need to be executed in a first-come, first-served order.
Example scenario: Implementing a simple task scheduler.
from collections import deque
class TaskScheduler:
def __init__(self):
self.task_queue = deque()
def add_task(self, task):
self.task_queue.append(task)
def execute_next_task(self):
if not self.task_queue:
print("No tasks to execute")
else:
task = self.task_queue.popleft()
print(f"Executing task: {task}")
# Example usage
scheduler = TaskScheduler()
scheduler.add_task("Send email")
scheduler.add_task("Generate report")
scheduler.add_task("Update database")
scheduler.execute_next_task() # Output: Executing task: Send email
scheduler.execute_next_task() # Output: Executing task: Generate report
3. Buffer for Data Streams
Queues are used to create buffers for data streams, especially in scenarios where data is produced and consumed at different rates.
Example scenario: Implementing a simple buffer for processing data streams.
from collections import deque
class DataBuffer:
def __init__(self, capacity):
self.buffer = deque(maxlen=capacity)
def add_data(self, data):
self.buffer.append(data)
def process_data(self):
if not self.buffer:
print("No data to process")
else:
data = self.buffer.popleft()
print(f"Processing data: {data}")
# Example usage
buffer = DataBuffer(3)
buffer.add_data("Packet 1")
buffer.add_data("Packet 2")
buffer.add_data("Packet 3")
buffer.add_data("Packet 4") # This will push out "Packet 1"
buffer.process_data() # Output: Processing data: Packet 2
buffer.process_data() # Output: Processing data: Packet 3
4. Print Queue / Printer Spooler
Queues are ideal for managing print jobs in a printer spooler, where documents are printed in the order they are received.
Example scenario: Implementing a simple printer queue.
from collections import deque
class PrinterQueue:
def __init__(self):
self.queue = deque()
def add_job(self, document):
self.queue.append(document)
print(f"Added {document} to the print queue")
def print_next(self):
if not self.queue:
print("No documents in the queue")
else:
document = self.queue.popleft()
print(f"Printing: {document}")
# Example usage
printer = PrinterQueue()
printer.add_job("Report.pdf")
printer.add_job("Letter.docx")
printer.add_job("Image.jpg")
printer.print_next() # Output: Printing: Report.pdf
printer.print_next() # Output: Printing: Letter.docx
Decision-Making: Stack vs Queue
Now that we’ve explored various scenarios for both stacks and queues, let’s summarize the key factors to consider when deciding between the two in an interview situation:
Choose a Stack when:
- You need to implement a Last-In-First-Out (LIFO) behavior.
- You’re dealing with nested or hierarchical structures (e.g., parsing expressions, managing function calls).
- You need to reverse the order of elements or actions (e.g., undo functionality).
- You’re implementing depth-first algorithms or backtracking.
- You need to check for balanced symbols or matching pairs.
Choose a Queue when:
- You need to implement a First-In-First-Out (FIFO) behavior.
- You’re dealing with sequential processing or scheduling tasks.
- You’re implementing breadth-first algorithms.
- You need to buffer or cache data in the order it’s received.
- You’re managing resources that should be shared in a fair, first-come-first-served manner.
Interview Tips for Stack and Queue Problems
When faced with a problem in an interview that might involve stacks or queues, consider these tips:
- Identify the order of operations: If the problem requires LIFO ordering, lean towards a stack. If it requires FIFO ordering, consider a queue.
- Look for nested structures: Problems involving nested elements (like parentheses matching) often benefit from stack-based solutions.
- Consider the traversal method: If you need to explore neighbors or related elements before moving to the next level, a queue-based BFS might be appropriate. If you need to explore as far as possible along each branch before backtracking, a stack-based DFS could be the way to go.
- Think about reversing or undoing: If the problem involves reversing operations or implementing undo functionality, a stack is likely the best choice.
- Analyze the data flow: If data needs to be processed in the exact order it’s received, a queue is often the right choice.
- Consider memory constraints: Both stacks and queues can be implemented with arrays or linked lists. Consider the space complexity and any specific memory constraints mentioned in the problem.
Conclusion
Mastering the use of stacks and queues is crucial for success in coding interviews, especially when applying for positions at major tech companies. By understanding the characteristics and appropriate use cases for each data structure, you’ll be better equipped to tackle a wide range of algorithmic problems.
Remember, the key to excelling in interviews is not just knowing these data structures, but also recognizing when and how to apply them effectively. Practice implementing stacks and queues from scratch, and solve various problems that utilize these structures. This hands-on experience will help you develop the intuition needed to make quick decisions during high-pressure interview situations.
As you continue your journey in mastering data structures and algorithms, keep in mind that stacks and queues are just the beginning. They often serve as building blocks for more complex data structures and algorithms. By solidifying your understanding of these fundamental concepts, you’re laying a strong foundation for tackling more advanced topics in computer science.
Happy coding, and best of luck in your future interviews!