How to Discuss Space-Time Tradeoffs Without Getting Technical
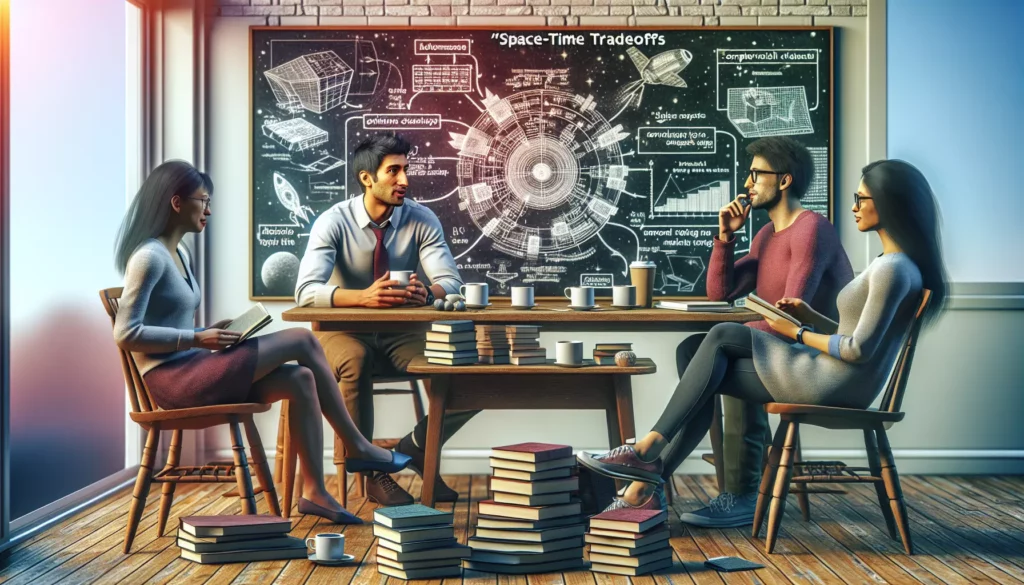
When it comes to coding and algorithm design, one of the most fundamental concepts that developers need to understand is the idea of space-time tradeoffs. This concept is crucial for creating efficient and effective programs, especially when preparing for technical interviews at major tech companies. However, explaining these tradeoffs to non-technical individuals or beginners can be challenging. In this article, we’ll explore how to discuss space-time tradeoffs in a way that’s accessible and easy to understand, without getting bogged down in technical jargon.
Understanding the Basics: What Are Space-Time Tradeoffs?
Before we dive into how to explain space-time tradeoffs, let’s briefly define what they are:
- Space refers to the amount of memory a program uses.
- Time refers to how long it takes for a program to run or complete a task.
- Tradeoff implies that there’s often a balance between these two resources – improving one might come at the cost of the other.
In essence, space-time tradeoffs are about finding the right balance between how much memory a program uses and how quickly it runs.
Using Everyday Analogies to Explain Space-Time Tradeoffs
One of the best ways to discuss space-time tradeoffs without getting technical is to use relatable analogies from everyday life. Here are a few examples:
1. The Closet Organizer Analogy
Imagine you’re organizing your closet. You have two options:
- Folding everything and stacking it (optimizing for space): This takes up less space in your closet but requires more time to find and retrieve specific items.
- Hanging everything (optimizing for time): This makes it quicker to find and access your clothes but takes up more space in the closet.
This analogy illustrates how you might trade space (closet size) for time (speed of finding items) or vice versa.
2. The Grocery Shopping Analogy
Consider two approaches to grocery shopping:
- Buying in bulk (optimizing for time): You spend less time shopping overall but need more storage space at home.
- Buying only what you need for the week (optimizing for space): You have less to store at home but spend more time making frequent trips to the store.
This example shows how you might trade time (shopping frequency) for space (storage at home) depending on your priorities.
3. The Road Trip Analogy
When planning a road trip, you have two options for navigation:
- Using a physical map (optimizing for space): It takes up less space in the car but requires more time to plan routes and navigate.
- Using a GPS device (optimizing for time): It’s quicker and easier to navigate but requires more resources (battery power, data connection).
This analogy demonstrates how you might trade physical space and simplicity for speed and convenience.
Practical Examples in Programming
While we’re aiming to avoid getting too technical, it can be helpful to provide some simple, real-world programming examples to illustrate space-time tradeoffs. Here are a few scenarios that can be explained in non-technical terms:
1. Sorting a List of Names
Imagine you have a list of names that you need to sort alphabetically. You have two approaches:
- Sort as you go (optimizing for space): You look at each name and place it in the correct position relative to the names you’ve already sorted. This uses less memory but takes longer, especially for large lists.
- Create a new sorted list (optimizing for time): You create a separate list where you put each name in its correct alphabetical position. This is faster, especially for large lists, but requires more memory to store the new list.
2. Searching for a Phone Number
Consider two ways to store and search for phone numbers:
- Using a simple list (optimizing for space): You store phone numbers in a basic list. This uses less memory, but searching for a specific number might require checking every entry, which takes longer.
- Using a more complex structure (optimizing for time): You organize numbers in a way that allows for quicker searches (like alphabetically or by area code). This uses more memory to maintain the structure but makes searches much faster.
3. Calculating Fibonacci Numbers
The Fibonacci sequence is a series of numbers where each number is the sum of the two preceding ones. There are two common ways to calculate these numbers:
- Recursive method (optimizing for space): You calculate each number by adding the two previous numbers, which you calculate in the same way. This uses less memory but can be very slow for large numbers.
- Storing previous results (optimizing for time): You save each calculated number so you don’t have to recalculate it later. This is much faster but requires more memory to store all the previous results.
The Importance of Balance
When discussing space-time tradeoffs, it’s crucial to emphasize that there’s no one-size-fits-all solution. The best approach depends on the specific situation and requirements. Here are some factors to consider:
1. Available Resources
Just as you might choose different grocery shopping strategies based on the size of your pantry, programmers need to consider the available resources. If memory is limited but processing power is abundant, they might opt for time-efficient solutions that use more space, and vice versa.
2. Scale of the Problem
The size of the task matters. For small datasets or simple operations, the difference between space-efficient and time-efficient solutions might be negligible. However, as the scale increases, these differences become more pronounced and important.
3. Frequency of Operations
How often a task needs to be performed can influence the choice between space and time optimization. If an operation is performed frequently, it might be worth using more memory to speed it up.
4. User Experience
In many applications, especially those with user interfaces, the speed of operations can significantly impact user experience. In such cases, using more memory to improve speed might be preferable.
Real-World Applications of Space-Time Tradeoffs
To further illustrate the importance of understanding space-time tradeoffs, let’s look at some real-world applications:
1. Web Browsers
Web browsers often cache (store) frequently visited web pages. This is a classic space-time tradeoff:
- It uses more space on your device to store the cached pages.
- But it saves time by loading these pages faster on subsequent visits.
2. Video Streaming Services
Services like Netflix use complex algorithms to balance video quality (which requires more data and thus more space) with streaming speed:
- Higher quality video uses more bandwidth (space) but provides a better viewing experience.
- Lower quality video uses less bandwidth but can be delivered more quickly and reliably.
3. Mobile Apps
Mobile app developers often face tough decisions about what data to store on the device versus retrieving from a server:
- Storing more data on the device uses more space but allows for faster operation and offline use.
- Retrieving data from a server as needed uses less space on the device but can be slower and requires an internet connection.
Teaching Space-Time Tradeoffs to Beginners
If you’re in a position to teach or explain space-time tradeoffs to beginners, here are some strategies that can help:
1. Start with Concrete Examples
Begin with tangible, real-world examples like the analogies we discussed earlier. This helps establish a foundation of understanding before diving into more abstract concepts.
2. Use Visual Aids
Diagrams, flowcharts, or even simple animations can help illustrate the differences between space-efficient and time-efficient approaches.
3. Encourage Hands-On Experience
If possible, provide simple coding exercises that demonstrate space-time tradeoffs. For example, have students implement both a space-efficient and a time-efficient solution to a problem and compare the results.
4. Relate to Everyday Technology
Discuss how space-time tradeoffs affect the technology students use every day, like smartphones, laptops, or gaming consoles. This helps make the concept more relevant and interesting.
5. Gradually Introduce Technical Terms
As students become more comfortable with the concept, you can start introducing more technical terms and specific algorithmic examples.
Common Misconceptions About Space-Time Tradeoffs
When discussing space-time tradeoffs, it’s important to address some common misconceptions:
1. “Faster is Always Better”
While speed is often crucial, it’s not always the most important factor. Sometimes, conserving memory or ensuring reliability is more critical.
2. “More Memory is Always Available”
With today’s powerful computers, it’s easy to assume that memory is unlimited. However, in many situations (like embedded systems or mobile devices), memory constraints are still very real.
3. “Tradeoffs Are Always Significant”
For small-scale problems or with modern hardware, the difference between space-efficient and time-efficient solutions might be negligible. The importance of these tradeoffs often becomes apparent only at larger scales.
4. “One Solution Fits All”
The best balance between space and time efficiency can vary greatly depending on the specific problem, available resources, and user requirements.
The Role of Space-Time Tradeoffs in Technical Interviews
Understanding space-time tradeoffs is particularly important for those preparing for technical interviews, especially at major tech companies. Here’s why:
1. Problem-Solving Skills
Interviewers often present problems where candidates need to consider both space and time efficiency. Being able to discuss and implement different approaches demonstrates strong problem-solving skills.
2. Scalability Considerations
Tech companies often deal with large-scale systems. Understanding how different solutions scale in terms of both time and space is crucial.
3. Optimization Techniques
Knowing how to optimize for either space or time (or find a balance between the two) is a valuable skill in software development.
4. System Design
In system design interviews, candidates often need to make high-level decisions that involve space-time tradeoffs, such as choosing between caching strategies or data storage solutions.
Conclusion
Understanding and being able to explain space-time tradeoffs is a valuable skill, not just for programmers and computer science students, but for anyone involved in technology-related fields. By using relatable analogies, practical examples, and emphasizing the importance of balance, it’s possible to discuss this complex topic without getting overly technical.
Remember, the goal is not to always choose the fastest or the most memory-efficient solution, but to find the right balance for each specific situation. As technology continues to evolve, the ability to make informed decisions about space-time tradeoffs will remain a crucial skill for problem-solving and efficient system design.
Whether you’re preparing for a technical interview, developing software, or simply trying to understand how your favorite apps and devices work, having a solid grasp of space-time tradeoffs will serve you well. So the next time you’re organizing your closet, planning a road trip, or even just grocery shopping, take a moment to consider the space-time tradeoffs you’re making – you might be surprised at how often this concept applies in everyday life!