The Right Way to Handle Unknown API Specifications in System Design
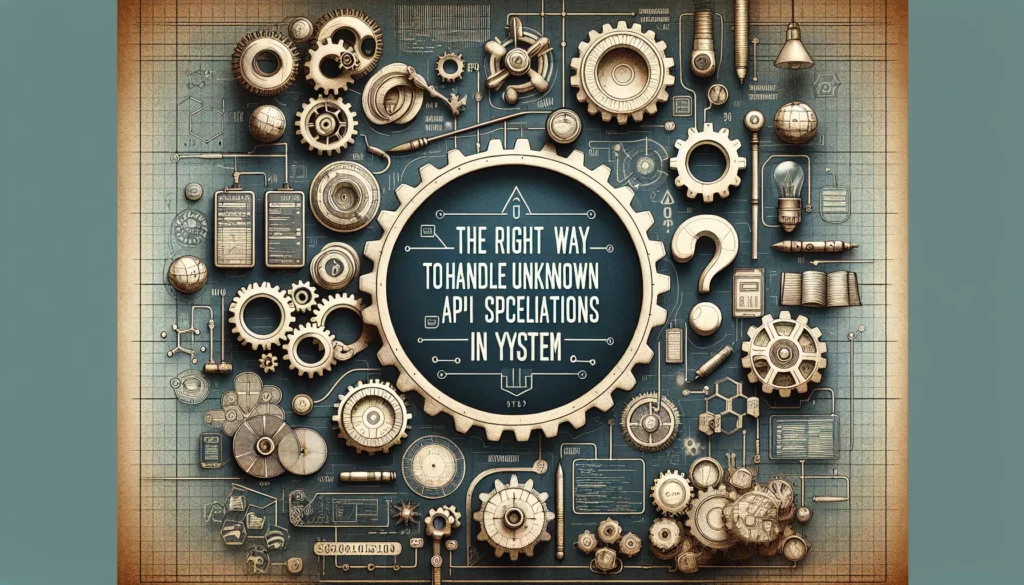
In the ever-evolving landscape of software development, system designers and architects often find themselves in situations where they need to integrate with external APIs whose specifications are not fully known or documented. This scenario is particularly common when dealing with third-party services, legacy systems, or APIs that are still under development. As aspiring software engineers or those preparing for technical interviews at top tech companies, it’s crucial to understand how to approach this challenge effectively. In this comprehensive guide, we’ll explore the best practices for handling unknown API specifications in system design, providing you with the knowledge and strategies to tackle this common scenario.
Understanding the Challenge of Unknown API Specifications
Before diving into solutions, it’s essential to grasp the complexity of the problem at hand. When faced with unknown API specifications, developers encounter several challenges:
- Uncertainty about data formats and structures
- Lack of information on available endpoints and their functionalities
- Unclear authentication and authorization mechanisms
- Potential inconsistencies in error handling and status codes
- Unknown rate limits and performance characteristics
These unknowns can significantly impact the design and implementation of your system, potentially leading to integration issues, performance bottlenecks, and maintenance headaches down the line. However, with the right approach, you can mitigate these risks and create a robust, flexible system that can adapt to evolving API specifications.
Best Practices for Handling Unknown API Specifications
1. Adopt an Iterative Approach
When dealing with unknown API specifications, it’s crucial to embrace an iterative development process. Instead of trying to design the entire system upfront, start with a minimal viable integration and gradually expand your understanding and implementation. This approach allows you to:
- Gain insights into the API’s behavior through practical experimentation
- Identify and address integration challenges early in the development process
- Adapt your design as you uncover more details about the API
- Minimize the risk of large-scale rework due to incorrect assumptions
To implement this iterative approach effectively, consider using agile methodologies and breaking down the integration process into small, manageable sprints. Each sprint should focus on a specific aspect of the API integration, allowing you to incrementally build your understanding and implementation.
2. Implement a Robust Error Handling Mechanism
When working with unknown API specifications, it’s crucial to expect the unexpected. Implementing a comprehensive error handling mechanism will help your system gracefully manage unforeseen scenarios and provide valuable insights for debugging and improvement. Consider the following strategies:
- Implement try-catch blocks to capture and log unexpected exceptions
- Create a centralized error logging system to track and analyze API-related issues
- Design fallback mechanisms for critical functionalities in case of API failures
- Implement retry logic with exponential backoff for transient errors
Here’s an example of how you might implement a robust error handling mechanism in Python:
import requests
import logging
from tenacity import retry, stop_after_attempt, wait_exponential
logging.basicConfig(level=logging.INFO)
logger = logging.getLogger(__name__)
@retry(stop=stop_after_attempt(3), wait=wait_exponential(multiplier=1, min=4, max=10))
def call_unknown_api(endpoint, params):
try:
response = requests.get(endpoint, params=params)
response.raise_for_status()
return response.json()
except requests.exceptions.RequestException as e:
logger.error(f"API call failed: {e}")
raise
def main():
try:
data = call_unknown_api("https://api.example.com/data", {"key": "value"})
process_data(data)
except Exception as e:
logger.critical(f"Unhandled exception: {e}")
# Implement fallback mechanism here
if __name__ == "__main__":
main()
This example demonstrates error logging, retry logic, and a centralized error handling approach, which are essential when working with unknown APIs.
3. Design a Flexible Data Model
When the structure of the data returned by the API is uncertain, it’s crucial to design a flexible data model that can accommodate various scenarios. Consider the following strategies:
- Use dynamic typing or flexible data structures (e.g., dictionaries in Python) to handle varying data formats
- Implement data validation and sanitization to ensure consistency in your system
- Create abstraction layers to decouple your internal data model from the API’s structure
- Use design patterns like Adapter or Facade to normalize data from different sources
Here’s an example of how you might implement a flexible data model in Python:
from typing import Any, Dict
from pydantic import BaseModel, validator
class FlexibleDataModel(BaseModel):
raw_data: Dict[str, Any]
@validator("raw_data")
def validate_raw_data(cls, v):
# Implement custom validation logic here
return v
def get_value(self, key: str, default: Any = None) -> Any:
return self.raw_data.get(key, default)
def to_internal_format(self) -> Dict[str, Any]:
# Convert the raw data to your internal format
return {
"id": self.get_value("id") or self.get_value("_id"),
"name": self.get_value("name") or self.get_value("title"),
"description": self.get_value("description") or self.get_value("summary"),
# Add more fields as needed
}
# Usage
api_response = {"_id": "123", "title": "Example", "summary": "This is a test"}
flexible_data = FlexibleDataModel(raw_data=api_response)
internal_data = flexible_data.to_internal_format()
print(internal_data)
This example demonstrates a flexible data model that can handle varying API responses while providing a consistent internal representation.
4. Implement Comprehensive Logging and Monitoring
When working with unknown API specifications, visibility into the system’s behavior becomes crucial. Implementing comprehensive logging and monitoring allows you to:
- Track API calls and their responses
- Identify patterns and inconsistencies in the API’s behavior
- Detect and diagnose issues quickly
- Gather data to inform future optimizations and design decisions
Consider implementing the following logging and monitoring strategies:
- Use structured logging to capture detailed information about API interactions
- Implement distributed tracing to understand the flow of requests across your system
- Set up alerts for unusual patterns or errors in API communication
- Use visualization tools to analyze API performance and behavior over time
Here’s an example of how you might implement structured logging for API calls:
import logging
import json
from datetime import datetime
class APILogger:
def __init__(self):
self.logger = logging.getLogger(__name__)
self.logger.setLevel(logging.INFO)
handler = logging.FileHandler("api_logs.json")
self.logger.addHandler(handler)
def log_api_call(self, endpoint, method, params, response):
log_entry = {
"timestamp": datetime.now().isoformat(),
"endpoint": endpoint,
"method": method,
"params": params,
"status_code": response.status_code,
"response_time": response.elapsed.total_seconds(),
"response_body": response.text[:1000] # Truncate long responses
}
self.logger.info(json.dumps(log_entry))
# Usage
api_logger = APILogger()
response = requests.get("https://api.example.com/data", params={"key": "value"})
api_logger.log_api_call("/data", "GET", {"key": "value"}, response)
This example demonstrates how to implement structured logging for API calls, which can be invaluable when working with unknown API specifications.
5. Use Contract Testing and Mocking
Even with unknown API specifications, it’s crucial to establish a testing strategy that ensures your system’s reliability and adaptability. Contract testing and mocking can be particularly useful in this scenario:
- Contract testing allows you to define and verify the expected behavior of the API, even as it evolves
- Mocking enables you to simulate various API responses, including edge cases and error scenarios
- These techniques help you build a more robust and resilient system
Here’s an example of how you might implement contract testing and mocking using Python and the `pytest` framework:
import pytest
import requests
from unittest.mock import patch
# The function we want to test
def get_user_data(user_id):
response = requests.get(f"https://api.example.com/users/{user_id}")
response.raise_for_status()
return response.json()
# Contract test
def test_get_user_data_contract():
user_id = 1
response = get_user_data(user_id)
assert "id" in response
assert "name" in response
assert "email" in response
assert isinstance(response["id"], int)
assert isinstance(response["name"], str)
assert isinstance(response["email"], str)
# Mock test
@patch("requests.get")
def test_get_user_data_mock(mock_get):
mock_response = requests.Response()
mock_response.status_code = 200
mock_response._content = b'{"id": 1, "name": "John Doe", "email": "john@example.com"}'
mock_get.return_value = mock_response
response = get_user_data(1)
assert response == {"id": 1, "name": "John Doe", "email": "john@example.com"}
# Error scenario mock test
@patch("requests.get")
def test_get_user_data_error(mock_get):
mock_response = requests.Response()
mock_response.status_code = 404
mock_get.return_value = mock_response
with pytest.raises(requests.exceptions.HTTPError):
get_user_data(999) # Non-existent user ID
These tests demonstrate how you can use contract testing to verify the structure of the API response and mocking to simulate various scenarios, including error cases.
6. Implement Caching and Rate Limiting
When working with unknown API specifications, it’s crucial to implement caching and rate limiting mechanisms to protect your system and the external API:
- Caching reduces the number of API calls and improves performance
- Rate limiting ensures that your system doesn’t overwhelm the API with requests
- These mechanisms help you stay within unknown API usage limits and improve overall system reliability
Here’s an example of how you might implement caching and rate limiting in Python:
import time
from functools import lru_cache
from ratelimit import limits, sleep_and_retry
# Caching
@lru_cache(maxsize=100)
def get_cached_data(key):
# Simulate API call
time.sleep(1)
return f"Data for {key}"
# Rate limiting
@sleep_and_retry
@limits(calls=5, period=10)
def rate_limited_api_call(endpoint):
# Simulate API call
time.sleep(0.1)
return f"Response from {endpoint}"
# Usage
def main():
# Caching example
for _ in range(5):
print(get_cached_data("example_key")) # Only the first call will take 1 second
# Rate limiting example
for _ in range(10):
try:
print(rate_limited_api_call("/data"))
except Exception as e:
print(f"Rate limit exceeded: {e}")
if __name__ == "__main__":
main()
This example demonstrates how to implement basic caching using the `lru_cache` decorator and rate limiting using the `ratelimit` library. These techniques can help you manage unknown API limitations effectively.
Advanced Strategies for Handling Unknown API Specifications
7. Implement a Circuit Breaker Pattern
The Circuit Breaker pattern is particularly useful when dealing with unknown APIs, as it helps prevent cascading failures and allows your system to degrade gracefully when the API becomes unresponsive or unreliable. Here’s how it works:
- The circuit starts in a closed state, allowing requests to pass through
- If the number of failures exceeds a threshold, the circuit opens, blocking requests
- After a timeout period, the circuit enters a half-open state, allowing a test request
- If the test request succeeds, the circuit closes; otherwise, it remains open
Here’s an example implementation of the Circuit Breaker pattern in Python:
import time
from functools import wraps
class CircuitBreaker:
def __init__(self, max_failures=3, reset_timeout=30):
self.max_failures = max_failures
self.reset_timeout = reset_timeout
self.failures = 0
self.last_failure_time = None
self.state = "closed"
def __call__(self, func):
@wraps(func)
def wrapper(*args, **kwargs):
if self.state == "open":
if time.time() - self.last_failure_time > self.reset_timeout:
self.state = "half-open"
else:
raise Exception("Circuit is open")
try:
result = func(*args, **kwargs)
if self.state == "half-open":
self.reset()
return result
except Exception as e:
self.record_failure()
raise e
return wrapper
def record_failure(self):
self.failures += 1
self.last_failure_time = time.time()
if self.failures >= self.max_failures:
self.state = "open"
def reset(self):
self.failures = 0
self.state = "closed"
# Usage
circuit_breaker = CircuitBreaker(max_failures=3, reset_timeout=10)
@circuit_breaker
def call_unknown_api():
# Simulate API call that sometimes fails
if time.time() % 2 == 0:
raise Exception("API Error")
return "API Response"
# Test the circuit breaker
for _ in range(10):
try:
result = call_unknown_api()
print(f"Success: {result}")
except Exception as e:
print(f"Error: {e}")
time.sleep(2)
This implementation demonstrates how the Circuit Breaker pattern can help manage interactions with an unstable or unknown API, preventing cascading failures in your system.
8. Implement API Versioning Strategy
Even when dealing with unknown API specifications, it’s crucial to prepare for potential changes and updates. Implementing an API versioning strategy in your system design can help you manage these changes effectively:
- Use API version headers or URL parameters to specify the version you’re targeting
- Create abstraction layers that can handle multiple API versions
- Implement feature flags to gradually roll out support for new API versions
Here’s an example of how you might implement API versioning in your system:
class APIClient:
def __init__(self, base_url, version="v1"):
self.base_url = base_url
self.version = version
def make_request(self, endpoint, method="GET", params=None):
url = f"{self.base_url}/{self.version}/{endpoint}"
headers = {"Accept-Version": self.version}
response = requests.request(method, url, params=params, headers=headers)
response.raise_for_status()
return response.json()
def get_user(self, user_id):
if self.version == "v1":
return self.make_request(f"users/{user_id}")
elif self.version == "v2":
# Handle differences in v2 API
data = self.make_request(f"users/{user_id}")
return {"id": data["userId"], "name": data["userName"]}
else:
raise ValueError(f"Unsupported API version: {self.version}")
# Usage
client_v1 = APIClient("https://api.example.com", version="v1")
client_v2 = APIClient("https://api.example.com", version="v2")
user_v1 = client_v1.get_user(1)
user_v2 = client_v2.get_user(1)
print(f"V1 User: {user_v1}")
print(f"V2 User: {user_v2}")
This example demonstrates how to create an API client that can handle multiple API versions, allowing your system to adapt to changes in the API specification over time.
9. Implement Fallback Mechanisms
When working with unknown or unreliable APIs, it’s crucial to implement fallback mechanisms to ensure your system remains functional even when the API fails or behaves unexpectedly. Consider the following strategies:
- Implement local caching to serve stale data when the API is unavailable
- Use alternative data sources or APIs as backups
- Degrade functionality gracefully when certain API features are unavailable
Here’s an example of how you might implement a fallback mechanism:
import requests
from cachetools import TTLCache
class APIWithFallback:
def __init__(self, primary_url, fallback_url, cache_ttl=3600):
self.primary_url = primary_url
self.fallback_url = fallback_url
self.cache = TTLCache(maxsize=100, ttl=cache_ttl)
def get_data(self, endpoint):
try:
# Try primary API
data = self._fetch_from_api(self.primary_url, endpoint)
self.cache[endpoint] = data # Update cache
return data
except requests.RequestException:
# Try fallback API
try:
return self._fetch_from_api(self.fallback_url, endpoint)
except requests.RequestException:
# Use cached data if available
if endpoint in self.cache:
return self.cache[endpoint]
else:
raise Exception("All data sources failed")
def _fetch_from_api(self, base_url, endpoint):
response = requests.get(f"{base_url}/{endpoint}")
response.raise_for_status()
return response.json()
# Usage
api = APIWithFallback(
primary_url="https://api.example.com",
fallback_url="https://backup-api.example.com"
)
try:
data = api.get_data("users/1")
print(f"User data: {data}")
except Exception as e:
print(f"Failed to fetch data: {e}")
This example demonstrates a fallback mechanism that tries a primary API, then a fallback API, and finally uses cached data if both APIs fail. This approach ensures that your system can continue to function even when facing API unavailability or unexpected behavior.
Conclusion
Handling unknown API specifications in system design is a complex challenge that requires a combination of robust architecture, flexible implementation, and proactive error management. By adopting the strategies outlined in this guide, you can create systems that are resilient, adaptable, and capable of integrating with APIs even when their specifications are not fully known or documented.
Remember that dealing with unknown APIs is an iterative process. As you gain more information about the API’s behavior and characteristics, continuously refine your system design and implementation. This approach will help you build a system that not only handles the current unknowns but is also well-prepared for future changes and challenges.
By mastering these techniques, you’ll be well-equipped to tackle complex system design problems involving unknown APIs, giving you a significant advantage in technical interviews and real-world software development scenarios. Keep practicing these concepts, and you’ll be well on your way to becoming a proficient system designer capable of handling even the most challenging integration scenarios.