Breaking Down Complex Requirements into Smaller Sub-problems: A Comprehensive Guide
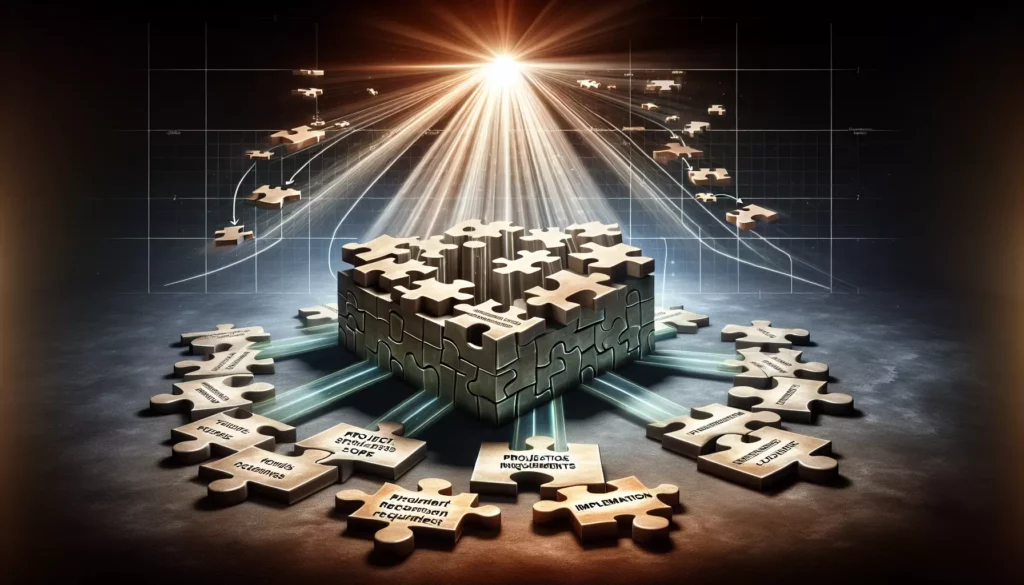
In the world of software development and programming, tackling complex problems is a daily occurrence. Whether you’re a beginner learning to code or an experienced developer preparing for technical interviews at major tech companies, the ability to break down complex requirements into smaller, manageable sub-problems is an essential skill. This approach, often referred to as “divide and conquer,” is a fundamental problem-solving technique that can significantly improve your coding efficiency and effectiveness.
In this comprehensive guide, we’ll explore the art of breaking down complex requirements, discuss its importance in coding education and skills development, and provide practical strategies to help you master this crucial skill. By the end of this article, you’ll be better equipped to tackle even the most daunting programming challenges with confidence.
Why Breaking Down Complex Requirements Matters
Before we dive into the specifics of how to break down complex requirements, let’s first understand why this skill is so important in the context of coding education and professional development:
- Improved Problem-Solving: By breaking down complex problems into smaller, more manageable pieces, you can approach each sub-problem with greater focus and clarity. This often leads to more efficient and effective solutions.
- Enhanced Code Organization: Decomposing complex requirements naturally leads to better-organized code, as each sub-problem can be addressed in its own function or module.
- Easier Debugging: When your code is broken down into smaller components, it becomes much easier to identify and fix bugs, as you can isolate and test each part independently.
- Increased Collaboration: In team environments, breaking down complex requirements allows for better task distribution and parallel development, as different team members can work on different sub-problems simultaneously.
- Gradual Skill Development: For learners, tackling smaller sub-problems helps build confidence and skills incrementally, making the overall learning process less overwhelming.
- Interview Preparation: Many technical interviews, especially at major tech companies, involve solving complex algorithmic problems. The ability to break these problems down is often key to success in these high-pressure situations.
The Process of Breaking Down Complex Requirements
Now that we understand the importance of this skill, let’s explore a step-by-step process for breaking down complex requirements into smaller sub-problems:
1. Understand the Problem
Before you can break down a complex requirement, you need to fully understand what’s being asked. This involves:
- Reading the problem statement carefully, multiple times if necessary
- Identifying the inputs and expected outputs
- Clarifying any ambiguities or assumptions
- Considering edge cases and potential constraints
For example, let’s say you’re given the following problem:
“Create a function that takes a string of parentheses and determines if the order of the parentheses is valid. The function should return true if the string is valid, and false if it’s invalid.”
2. Identify the Main Components
Once you understand the problem, try to identify the main components or steps involved in solving it. For our parentheses problem, we might identify the following components:
- Iterating through the string of parentheses
- Keeping track of open parentheses
- Checking if each closing parenthesis matches the most recent open parenthesis
- Ensuring all parentheses are closed by the end
3. Break Down Each Component
For each main component, consider if it can be further broken down into smaller, more manageable tasks. Let’s break down the “Keeping track of open parentheses” component:
- Initialize a data structure (e.g., a stack) to store open parentheses
- When encountering an open parenthesis, add it to the stack
- When encountering a closing parenthesis, check if the stack is empty (if so, return false)
- If the stack is not empty, compare the closing parenthesis with the top of the stack
4. Identify Common Patterns or Algorithms
As you break down the problem, look for common patterns or well-known algorithms that might apply. In our parentheses example, we can recognize that a stack data structure is well-suited for this problem, as it follows a Last-In-First-Out (LIFO) order, which matches the nested nature of parentheses.
5. Prioritize and Order Sub-problems
Determine the logical order in which to tackle the sub-problems. Some sub-problems may depend on others, so it’s important to establish a sequence. For our parentheses problem, a logical order might be:
- Initialize the stack
- Iterate through the string
- Handle open parentheses
- Handle closing parentheses
- Check if all parentheses are closed
6. Solve Each Sub-problem
Now that you have broken down the complex requirement into smaller, manageable pieces, you can focus on solving each sub-problem individually. This is where your coding skills come into play. Let’s implement a solution for our parentheses problem:
def is_valid_parentheses(s):
stack = []
for char in s:
if char == "(":
stack.append(char)
elif char == ")":
if not stack:
return False
if stack.pop() != "(":
return False
return len(stack) == 0
7. Integrate and Test
After solving each sub-problem, integrate them into a complete solution. Test your solution with various inputs, including edge cases, to ensure it works correctly. For our parentheses problem, we might test with inputs like:
print(is_valid_parentheses("()")) # True
print(is_valid_parentheses("(())")) # True
print(is_valid_parentheses("(()")) # False
print(is_valid_parentheses(")(")) # False
print(is_valid_parentheses("")) # True
Strategies for Effective Problem Breakdown
Now that we’ve walked through the process of breaking down a complex requirement, let’s explore some additional strategies that can help you become more proficient at this skill:
1. Use Visual Aids
Sometimes, visualizing a problem can help you identify its components more easily. Try using diagrams, flowcharts, or even simple sketches to represent the problem and its parts. For example, in our parentheses problem, we might draw a simple diagram showing how parentheses are pushed onto and popped off the stack.
2. Start with a Simpler Version
If a problem seems too complex at first, try solving a simpler version of it. Then gradually add complexity until you reach the original problem. For instance, before tackling matching parentheses, you might start with a problem that just counts the number of open and closed parentheses.
3. Use the “Five Whys” Technique
Borrowed from root cause analysis, the “Five Whys” technique involves asking “why” repeatedly to dig deeper into a problem. This can help you identify underlying sub-problems or requirements that might not be immediately obvious.
4. Leverage Existing Libraries or Functions
Don’t reinvent the wheel. If a sub-problem can be solved using an existing library or built-in function, use it. This allows you to focus on the unique aspects of your problem. In our parentheses example, we leveraged Python’s built-in list as a stack data structure.
5. Consider Different Perspectives
Try to approach the problem from different angles. Sometimes, changing your perspective can reveal new ways to break down the problem or solve it more efficiently.
6. Use Recursive Thinking
For problems that have a naturally recursive structure, consider how you might break them down into smaller, similar sub-problems. Many complex problems in computer science, such as tree traversals or certain dynamic programming problems, lend themselves well to recursive solutions.
7. Apply the Single Responsibility Principle
Borrowed from object-oriented design, the Single Responsibility Principle suggests that each component (or in our case, sub-problem) should have a single, well-defined responsibility. This can help you identify clear boundaries between sub-problems.
Common Pitfalls to Avoid
While breaking down complex requirements is a powerful problem-solving technique, there are some common pitfalls to be aware of:
1. Over-complicating
Sometimes, in an attempt to break down a problem, you might end up making it more complex than necessary. Always strive for the simplest breakdown that effectively solves the problem.
2. Losing Sight of the Big Picture
While focusing on individual sub-problems is important, don’t forget to periodically step back and ensure that your solution still aligns with the overall requirement.
3. Ignoring Edge Cases
When breaking down a problem, it’s easy to focus on the “happy path” and forget about edge cases. Make sure to consider and address these as part of your breakdown.
4. Premature Optimization
While efficiency is important, don’t let it paralyze you in the early stages of problem-solving. Focus first on breaking down the problem and getting a working solution. You can optimize later if necessary.
5. Not Validating Assumptions
When breaking down a problem, you might make certain assumptions. Always validate these assumptions to ensure your breakdown is sound.
Applying Problem Breakdown in Different Contexts
The skill of breaking down complex requirements is valuable in various contexts within software development and beyond. Let’s explore how this skill applies in different scenarios:
1. Algorithm Design
When designing complex algorithms, breaking them down into smaller steps or sub-algorithms is crucial. For example, if you’re implementing a sorting algorithm like Merge Sort, you might break it down into:
- Dividing the array into two halves
- Recursively sorting each half
- Merging the sorted halves
Here’s a simple implementation of Merge Sort in Python:
def merge_sort(arr):
if len(arr) <= 1:
return arr
mid = len(arr) // 2
left = merge_sort(arr[:mid])
right = merge_sort(arr[mid:])
return merge(left, right)
def merge(left, right):
result = []
i, j = 0, 0
while i < len(left) and j < len(right):
if left[i] < right[j]:
result.append(left[i])
i += 1
else:
result.append(right[j])
j += 1
result.extend(left[i:])
result.extend(right[j:])
return result
# Test the merge sort function
arr = [64, 34, 25, 12, 22, 11, 90]
sorted_arr = merge_sort(arr)
print(sorted_arr) # [11, 12, 22, 25, 34, 64, 90]
2. System Design
In system design, breaking down complex requirements is essential for creating scalable and maintainable architectures. For instance, when designing a social media platform, you might break it down into components like:
- User Authentication Service
- Post Creation and Storage Service
- News Feed Generation Service
- Notification Service
- Analytics Service
Each of these components can then be further broken down into smaller services or modules.
3. Project Management
In project management, breaking down complex projects into smaller, manageable tasks is a fundamental practice. This is often done using techniques like Work Breakdown Structure (WBS). For a software development project, this might look like:
- Requirements Gathering
- Stakeholder Interviews
- User Story Creation
- Requirements Documentation
- Design
- System Architecture
- Database Schema
- UI/UX Design
- Implementation
- Backend Development
- Frontend Development
- API Integration
- Testing
- Unit Testing
- Integration Testing
- User Acceptance Testing
- Deployment
- Server Setup
- Application Deployment
- Post-Deployment Monitoring
4. Learning and Skill Development
When learning new programming concepts or preparing for technical interviews, breaking down complex topics into smaller, learnable chunks can be very effective. For example, if you’re learning about data structures, you might break it down like this:
- Arrays and Strings
- Linked Lists
- Stacks and Queues
- Trees and Graphs
- Hash Tables
- Heaps
For each data structure, you can further break down your learning into:
- Basic concepts and terminology
- Implementation details
- Common operations and their time complexities
- Practical use cases
- Practice problems
Tools and Techniques for Problem Breakdown
There are various tools and techniques that can aid in the process of breaking down complex requirements. Here are some popular ones:
1. Mind Mapping
Mind mapping is a visual technique that can help you organize and structure complex ideas. Start with the main problem in the center and branch out into sub-problems and related concepts. Tools like MindMeister or XMind can be useful for creating digital mind maps.
2. Pseudocode
Writing pseudocode can help you break down a problem into logical steps without getting bogged down in syntax details. Here’s an example of pseudocode for our parentheses matching problem:
function is_valid_parentheses(string):
initialize empty stack
for each character in string:
if character is an opening parenthesis:
push it onto the stack
else if character is a closing parenthesis:
if stack is empty:
return false
if top of stack doesn't match current character:
return false
pop from stack
return true if stack is empty, false otherwise
3. Flowcharts
Flowcharts are excellent for visualizing the flow of a process or algorithm. Tools like Lucidchart or draw.io can be used to create flowcharts easily.
4. User Stories
In Agile methodologies, user stories are often used to break down complex requirements from a user’s perspective. A user story typically follows the format: “As a [type of user], I want [an action] so that [a benefit/a value].”
5. SDLC Models
Software Development Life Cycle (SDLC) models like Waterfall or Agile inherently involve breaking down the development process into phases or sprints, which can help in managing complex projects.
6. Design Patterns
Familiarity with common design patterns can help you recognize standard ways of breaking down and solving certain types of problems in software design.
Conclusion
Breaking down complex requirements into smaller sub-problems is a crucial skill in software development, problem-solving, and beyond. It allows us to tackle seemingly insurmountable challenges by addressing them piece by piece. This approach not only makes the problem-solving process more manageable but also often leads to more elegant, efficient, and maintainable solutions.
As you continue your journey in coding education and skills development, remember that mastering this skill takes practice. Each time you encounter a complex problem, challenge yourself to break it down systematically. Over time, you’ll find that this approach becomes second nature, enabling you to tackle even the most daunting coding challenges with confidence.
Whether you’re preparing for technical interviews at major tech companies, working on a complex project, or simply trying to learn a new programming concept, the ability to break down complex requirements will serve you well. It’s a skill that distinguishes great problem-solvers and is highly valued in the tech industry.
So, the next time you face a complex coding challenge, take a deep breath, and start breaking it down. You might be surprised at how manageable even the most complex problems become when approached one piece at a time.