How to Spot Pattern Similarities with Previously Solved Problems
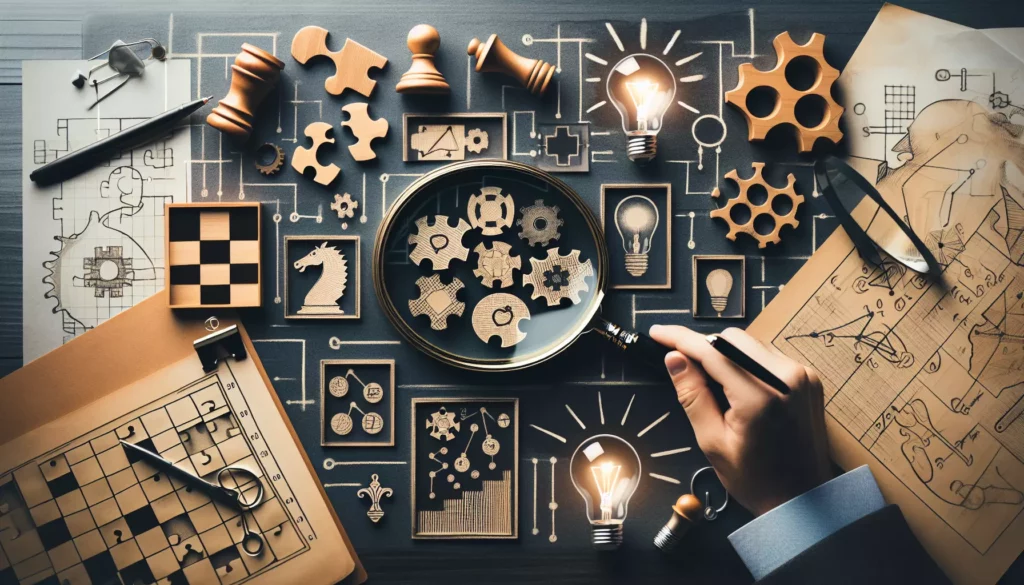
In the world of coding and algorithmic problem-solving, one of the most valuable skills you can develop is the ability to recognize patterns and similarities between new problems and those you’ve encountered before. This skill not only speeds up your problem-solving process but also helps you approach complex challenges with greater confidence and efficiency. In this comprehensive guide, we’ll explore various techniques and strategies to help you spot pattern similarities with previously solved problems, ultimately enhancing your coding prowess and interview preparation.
1. Understanding the Importance of Pattern Recognition
Before diving into specific techniques, it’s crucial to understand why pattern recognition is so important in coding and problem-solving:
- Efficiency: Recognizing patterns allows you to apply proven solutions more quickly, saving time and mental energy.
- Problem-solving framework: Patterns provide a structured approach to tackling new challenges.
- Algorithmic thinking: Identifying similarities helps develop a more intuitive understanding of algorithmic concepts.
- Interview preparation: Many technical interviews, especially for FAANG companies, test your ability to recognize and apply common patterns.
2. Building a Strong Foundation of Problem-Solving Patterns
To effectively spot similarities, you need a solid foundation of common problem-solving patterns. Here are some essential patterns to familiarize yourself with:
2.1. Two Pointers
The two-pointer technique involves using two pointers to traverse an array or string, often moving in opposite directions or at different speeds.
Example problem: Find a pair of numbers in a sorted array that sum up to a target value.
def two_sum(arr, target):
left, right = 0, len(arr) - 1
while left < right:
current_sum = arr[left] + arr[right]
if current_sum == target:
return [arr[left], arr[right]]
elif current_sum < target:
left += 1
else:
right -= 1
return None
2.2. Sliding Window
The sliding window technique involves maintaining a subset of elements as a “window” and sliding it over the data structure to solve the problem efficiently.
Example problem: Find the maximum sum of a subarray of size k in an array.
def max_subarray_sum(arr, k):
window_sum = sum(arr[:k])
max_sum = window_sum
for i in range(k, len(arr)):
window_sum = window_sum - arr[i-k] + arr[i]
max_sum = max(max_sum, window_sum)
return max_sum
2.3. Fast and Slow Pointers
This pattern uses two pointers moving at different speeds, often used for cycle detection or finding the middle of a linked list.
Example problem: Detect a cycle in a linked list.
def has_cycle(head):
if not head or not head.next:
return False
slow = head
fast = head.next
while slow != fast:
if not fast or not fast.next:
return False
slow = slow.next
fast = fast.next.next
return True
2.4. Merge Intervals
This pattern deals with problems involving overlapping intervals or ranges.
Example problem: Merge overlapping intervals in a list of intervals.
def merge_intervals(intervals):
intervals.sort(key=lambda x: x[0])
merged = []
for interval in intervals:
if not merged or merged[-1][1] < interval[0]:
merged.append(interval)
else:
merged[-1][1] = max(merged[-1][1], interval[1])
return merged
2.5. Dynamic Programming
Dynamic programming involves breaking down a problem into smaller subproblems and storing their solutions to avoid redundant computations.
Example problem: Calculate the nth Fibonacci number.
def fibonacci(n):
if n <= 1:
return n
dp = [0] * (n + 1)
dp[1] = 1
for i in range(2, n + 1):
dp[i] = dp[i-1] + dp[i-2]
return dp[n]
3. Techniques for Spotting Pattern Similarities
Now that we’ve covered some common patterns, let’s explore techniques to help you recognize similarities between new problems and those you’ve encountered before:
3.1. Identify the Problem Type
When faced with a new problem, try to categorize it based on its core characteristics:
- Is it a search problem?
- Does it involve optimization?
- Is it a graph-related problem?
- Does it require string manipulation?
- Is it a mathematical or numerical problem?
By identifying the problem type, you can narrow down the potential patterns that might apply.
3.2. Analyze the Input and Output
Carefully examine the input and output requirements of the problem:
- What are the input data structures (e.g., arrays, linked lists, trees)?
- What is the expected output format?
- Are there any constraints on the input or output?
Often, the input and output characteristics can provide clues about which patterns might be applicable.
3.3. Look for Key Words and Phrases
Problem descriptions often contain key words or phrases that hint at specific patterns:
- “Subarray” or “substring” might suggest a sliding window approach.
- “Overlapping” or “merge” could indicate an interval-related problem.
- “Cycle” or “loop” might point towards using fast and slow pointers.
- “Optimal” or “maximum/minimum” could suggest dynamic programming.
3.4. Consider Time and Space Complexity Requirements
The expected time and space complexity can often guide you towards the appropriate pattern:
- O(n) time complexity might suggest a single-pass solution, possibly using two pointers or a sliding window.
- O(log n) time complexity often involves binary search or divide-and-conquer approaches.
- O(1) space complexity might rule out certain approaches and push you towards in-place algorithms.
3.5. Draw Parallels with Known Problems
Try to relate the new problem to problems you’ve solved before:
- Is the core concept similar to a known problem, even if the context is different?
- Can you modify a solution from a similar problem to fit the current one?
- Are there any subproblems within the main problem that resemble familiar patterns?
3.6. Visualize the Problem
Sometimes, visualizing the problem can help reveal patterns:
- Draw diagrams or flowcharts to represent the problem.
- Use physical objects or props to model the problem scenario.
- Try to animate the problem-solving process in your mind.
3.7. Break Down Complex Problems
For more complex problems, try breaking them down into smaller, more manageable subproblems:
- Identify the main components or steps required to solve the problem.
- Look for familiar patterns within each subproblem.
- Consider how the subproblems might be combined to form a complete solution.
4. Practicing Pattern Recognition
Improving your ability to spot pattern similarities requires consistent practice and exposure to a wide range of problems. Here are some strategies to enhance your pattern recognition skills:
4.1. Solve Problems Systematically
When solving problems, follow a structured approach:
- Read the problem statement carefully.
- Identify the problem type and potential patterns.
- Brainstorm possible solutions.
- Implement the chosen solution.
- Test and refine your approach.
- Analyze the time and space complexity.
- Look for alternative solutions or optimizations.
4.2. Review and Categorize Solved Problems
After solving a problem, take time to review and categorize it:
- Which pattern(s) did you use to solve the problem?
- What were the key characteristics that led you to choose that pattern?
- How does this problem relate to others you’ve solved before?
- Create a personal database or notes system to organize problems by pattern and difficulty.
4.3. Study Problem-Solving Techniques
Continuously expand your knowledge of problem-solving techniques:
- Read books and articles on algorithms and data structures.
- Watch video tutorials and coding interviews.
- Participate in coding competitions and hackathons.
- Join online coding communities and forums to discuss problems and solutions.
4.4. Implement a Spaced Repetition System
Use spaced repetition to reinforce your understanding of patterns:
- Revisit problems you’ve solved after increasing intervals (e.g., 1 day, 1 week, 1 month).
- Try to solve the problems again without looking at your previous solutions.
- Analyze how your approach may have changed or improved over time.
4.5. Teach Others
Teaching is an excellent way to solidify your understanding of patterns:
- Explain problem-solving patterns to peers or junior developers.
- Write blog posts or create video tutorials about specific patterns.
- Participate in code reviews and provide feedback on pattern usage.
5. Common Pitfalls to Avoid
While developing your pattern recognition skills, be aware of these common pitfalls:
5.1. Over-reliance on Memorization
Don’t simply memorize solutions to specific problems. Instead, focus on understanding the underlying principles and patterns that make those solutions effective.
5.2. Forcing Patterns
Avoid trying to force a familiar pattern onto a problem where it doesn’t fit. Be open to the possibility that a problem might require a unique approach or a combination of patterns.
5.3. Neglecting Edge Cases
When applying a pattern, don’t forget to consider edge cases and boundary conditions. These often require special handling and can reveal limitations in your chosen approach.
5.4. Ignoring Problem Constraints
Always pay attention to the specific constraints of each problem. A pattern that works well for small inputs might not be suitable for large-scale scenarios.
5.5. Overlooking Simpler Solutions
Don’t automatically jump to complex patterns if a simpler solution exists. Sometimes, a straightforward approach can be more efficient and easier to implement.
6. Advanced Pattern Recognition Techniques
As you become more proficient in recognizing basic patterns, you can explore more advanced techniques:
6.1. Hybrid Patterns
Learn to combine multiple patterns to solve more complex problems. For example, you might use a sliding window technique within a dynamic programming solution.
6.2. Pattern Variations
Recognize variations of common patterns. For instance, the two-pointer technique can be applied to arrays, linked lists, or even trees in different ways.
6.3. Meta-patterns
Identify higher-level patterns that encompass multiple problem-solving strategies. For example, the “divide and conquer” approach can be applied to various algorithm designs.
6.4. Cross-domain Pattern Recognition
Look for patterns that apply across different domains of computer science, such as similarities between graph algorithms and dynamic programming approaches.
7. Applying Pattern Recognition in Technical Interviews
Pattern recognition skills are particularly valuable in technical interviews, especially for FAANG companies. Here’s how to leverage these skills effectively:
7.1. Verbalize Your Thought Process
As you analyze the problem, explain your thought process to the interviewer:
- Describe the patterns you’re considering and why.
- Discuss the pros and cons of different approaches.
- Explain how you’re drawing parallels with previously solved problems.
7.2. Start with a Brute Force Approach
Begin with a simple, brute force solution to demonstrate your problem-solving ability. Then, optimize it by applying appropriate patterns.
7.3. Discuss Multiple Solutions
If time allows, discuss alternative solutions using different patterns. This showcases your breadth of knowledge and analytical skills.
7.4. Practice Mock Interviews
Conduct mock interviews with peers or use online platforms to simulate the interview experience. This helps you practice articulating your pattern recognition process under pressure.
Conclusion
Developing the ability to spot pattern similarities with previously solved problems is a crucial skill for any programmer, particularly those preparing for technical interviews at top tech companies. By building a strong foundation of common patterns, practicing systematic problem-solving, and continuously expanding your knowledge, you can significantly enhance your coding abilities and problem-solving efficiency.
Remember that pattern recognition is not about memorizing solutions, but rather about developing an intuitive understanding of problem structures and algorithmic approaches. As you continue to practice and refine your skills, you’ll find yourself approaching new challenges with greater confidence and creativity.
Keep in mind that the journey to mastering pattern recognition is ongoing. Even experienced developers continue to discover new patterns and refine their problem-solving techniques. Stay curious, embrace challenges, and never stop learning. With persistence and dedication, you’ll be well-equipped to tackle even the most complex coding problems and excel in your programming career.