Mastering Algorithms and Data Structures: A Comprehensive Guide for Aspiring Programmers
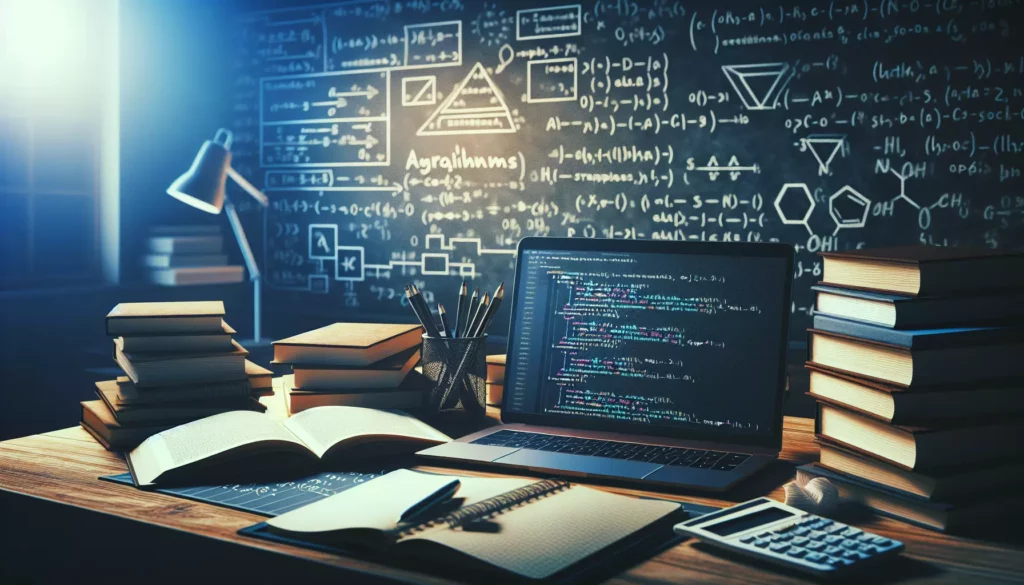
In the ever-evolving world of technology, mastering algorithms and data structures has become an essential skill for programmers and software engineers. Whether you’re a beginner looking to build a strong foundation or an experienced developer aiming to ace technical interviews at top tech companies, understanding these fundamental concepts is crucial. This comprehensive guide will walk you through the best approaches to mastering algorithms and data structures, providing you with practical tips, resources, and strategies to enhance your coding skills.
Why Are Algorithms and Data Structures Important?
Before diving into the best approaches for mastering these concepts, it’s important to understand why algorithms and data structures are so critical in the field of computer science and software development:
- Efficiency: Proper use of algorithms and data structures can significantly improve the performance and efficiency of your code.
- Problem-solving: They provide a structured approach to solving complex computational problems.
- Scalability: Understanding these concepts allows you to write code that can handle large amounts of data and scale effectively.
- Interview preparation: Many technical interviews, especially at FAANG (Facebook, Amazon, Apple, Netflix, Google) companies, focus heavily on algorithms and data structures.
- Foundation for advanced topics: A strong grasp of these fundamentals is essential for understanding more advanced computer science concepts.
The Best Approach to Mastering Algorithms and Data Structures
1. Start with the Basics
Before diving into complex algorithms, it’s crucial to have a solid understanding of the basics. Begin with fundamental data structures such as arrays, linked lists, stacks, and queues. Familiarize yourself with basic algorithmic concepts like time and space complexity, Big O notation, and basic sorting and searching algorithms.
Key steps:
- Learn about basic data types and variables in your preferred programming language.
- Understand how memory works and how data is stored and accessed.
- Study the implementation of basic data structures from scratch.
- Practice writing simple algorithms to manipulate these data structures.
2. Choose the Right Programming Language
While algorithms and data structures are language-agnostic concepts, choosing the right programming language can make your learning journey smoother. Popular choices include:
- Python: Known for its simplicity and readability, Python is an excellent choice for beginners.
- Java: Widely used in industry and coding interviews, Java provides a good balance of performance and ease of use.
- C++: Offers low-level control and is often used in competitive programming.
- JavaScript: Useful for web developers and those interested in full-stack development.
Choose a language you’re comfortable with or one that aligns with your career goals. Consistency is key, so stick with your chosen language throughout your learning process.
3. Follow a Structured Learning Path
Having a structured approach to learning algorithms and data structures can help you progress more efficiently. Here’s a suggested learning path:
- Basic data structures (arrays, linked lists, stacks, queues)
- Basic algorithms (searching, sorting)
- Recursion and dynamic programming
- Trees and graphs
- Advanced data structures (heaps, hash tables, tries)
- Advanced algorithms (graph algorithms, string algorithms)
- Algorithm design techniques (divide and conquer, greedy algorithms)
4. Utilize Online Resources and Courses
There are numerous online resources available to help you master algorithms and data structures. Some popular options include:
- MOOCs (Massive Open Online Courses): Platforms like Coursera, edX, and Udacity offer comprehensive courses on algorithms and data structures from top universities.
- Interactive coding platforms: Websites like LeetCode, HackerRank, and CodeSignal provide a vast array of coding problems to practice your skills.
- Video tutorials: YouTube channels like MIT OpenCourseWare and mycodeschool offer in-depth video explanations of various algorithms and data structures.
- Books: Classic texts like “Introduction to Algorithms” by Cormen et al. and “Cracking the Coding Interview” by Gayle Laakmann McDowell are invaluable resources.
5. Practice, Practice, Practice
The key to mastering algorithms and data structures is consistent practice. Set aside regular time for solving coding problems and implementing algorithms from scratch. Here are some strategies to make your practice more effective:
- Start with easy problems: Build confidence by solving simpler problems before moving on to more complex ones.
- Implement from scratch: Try implementing data structures and algorithms without relying on built-in libraries.
- Time yourself: Practice solving problems within a time limit to improve your speed and efficiency.
- Participate in coding contests: Platforms like Codeforces and TopCoder host regular coding competitions that can help you hone your skills.
6. Understand the Underlying Principles
While memorizing solutions can be tempting, it’s crucial to understand the underlying principles and thought processes behind different algorithms and data structures. Focus on:
- The problem-solving approach
- Time and space complexity analysis
- Trade-offs between different solutions
- Real-world applications of various algorithms and data structures
7. Visualize Algorithms and Data Structures
Visual representations can greatly enhance your understanding of complex concepts. Utilize tools and resources that provide visual explanations of algorithms and data structures:
- Visualization websites: Platforms like VisuAlgo and Algorithm Visualizer offer interactive visualizations of various algorithms and data structures.
- Draw diagrams: Practice drawing out data structures and algorithm steps on paper or a whiteboard.
- Use debugging tools: Step through your code using debuggers to visualize how algorithms work with specific inputs.
8. Join Coding Communities
Engaging with other learners and experienced programmers can accelerate your learning process. Consider:
- Joining online forums like Stack Overflow or Reddit’s r/learnprogramming
- Participating in local coding meetups or hackathons
- Collaborating on open-source projects
- Forming study groups with peers
9. Apply Your Knowledge to Real Projects
To solidify your understanding, apply the algorithms and data structures you’ve learned to real-world projects. This could involve:
- Building a simple search engine
- Implementing a basic database system
- Creating a pathfinding algorithm for a game
- Developing a compression algorithm for file storage
10. Prepare for Technical Interviews
If your goal is to ace technical interviews at top tech companies, focus on:
- Solving problems on platforms like LeetCode and HackerRank
- Practicing whiteboard coding and explaining your thought process
- Studying common interview questions and patterns
- Conducting mock interviews with peers or mentors
Common Pitfalls to Avoid
As you embark on your journey to master algorithms and data structures, be aware of these common pitfalls:
- Memorizing without understanding: Focus on grasping the underlying concepts rather than memorizing specific solutions.
- Neglecting time and space complexity: Always analyze the efficiency of your solutions.
- Avoiding difficult topics: Don’t shy away from challenging concepts; they often provide the most valuable learning experiences.
- Inconsistent practice: Regular, consistent practice is key to long-term retention and improvement.
- Ignoring the importance of clean code: While solving problems, also focus on writing clean, readable, and maintainable code.
Advanced Topics to Explore
Once you’ve mastered the fundamentals, consider diving into more advanced topics to further enhance your skills:
- Advanced graph algorithms (e.g., network flow, strongly connected components)
- Computational geometry algorithms
- Randomized algorithms
- Approximation algorithms for NP-hard problems
- Parallel and distributed algorithms
- Machine learning algorithms
Tools and Resources for Learning
To support your learning journey, consider using the following tools and resources:
Online Judges and Practice Platforms
- LeetCode
- HackerRank
- CodeSignal
- Codeforces
- TopCoder
Books
- “Introduction to Algorithms” by Cormen, Leiserson, Rivest, and Stein
- “Algorithms” by Robert Sedgewick and Kevin Wayne
- “Cracking the Coding Interview” by Gayle Laakmann McDowell
- “The Algorithm Design Manual” by Steven S. Skiena
Online Courses
- Coursera’s “Algorithms Specialization” by Stanford University
- edX’s “Algorithms and Data Structures” by Microsoft
- Udacity’s “Data Structures and Algorithms Nanodegree”
Visualization Tools
- VisuAlgo
- Algorithm Visualizer
- Data Structure Visualizations by the University of San Francisco
Implementing a Sample Algorithm
To give you a practical example of how to approach learning an algorithm, let’s implement a basic sorting algorithm: Bubble Sort. We’ll use Python for this example:
def bubble_sort(arr):
n = len(arr)
for i in range(n):
for j in range(0, n - i - 1):
if arr[j] > arr[j + 1]:
arr[j], arr[j + 1] = arr[j + 1], arr[j]
return arr
# Test the algorithm
test_array = [64, 34, 25, 12, 22, 11, 90]
print("Original array:", test_array)
sorted_array = bubble_sort(test_array)
print("Sorted array:", sorted_array)
When learning this algorithm, focus on understanding:
- How the algorithm works step-by-step
- The time complexity (O(n^2) in this case)
- Potential optimizations (e.g., early termination if no swaps occur in a pass)
- Comparison with other sorting algorithms
Conclusion
Mastering algorithms and data structures is a journey that requires dedication, consistent practice, and a structured approach. By following the steps outlined in this guide, you’ll be well on your way to becoming proficient in these essential computer science concepts. Remember that the key to success is persistence and continuous learning. As you progress, you’ll find that your problem-solving skills improve dramatically, opening up new opportunities in your programming career.
Whether you’re preparing for technical interviews at top tech companies or simply aiming to become a better programmer, the skills you develop while mastering algorithms and data structures will serve you well throughout your career. Embrace the challenges, stay curious, and enjoy the process of becoming a more skilled and confident programmer.
Happy coding!