How to Avoid Common Syntax Errors During Coding Interviews: A Comprehensive Guide
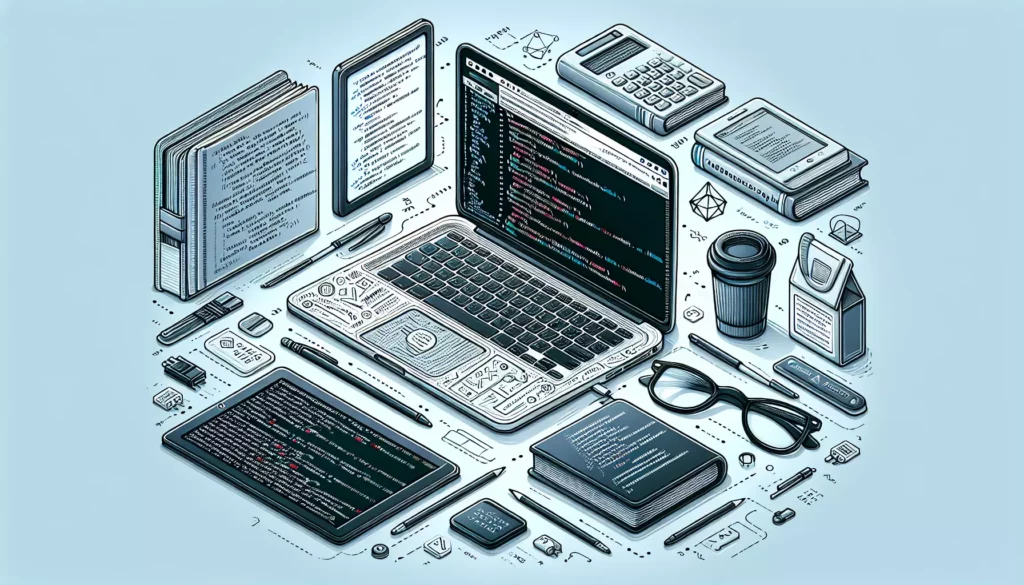
Coding interviews can be nerve-wracking experiences, especially when you’re trying to showcase your skills under pressure. One of the most frustrating things that can happen during these interviews is making simple syntax errors that could easily be avoided. These mistakes not only waste precious time but can also shake your confidence and potentially impact the interviewer’s perception of your abilities. In this comprehensive guide, we’ll explore various strategies to help you avoid common syntax errors during coding interviews, allowing you to focus on demonstrating your problem-solving skills and algorithmic thinking.
Understanding the Importance of Syntax in Coding Interviews
Before we dive into specific strategies, it’s crucial to understand why syntax matters in coding interviews. While interviewers are primarily interested in your problem-solving approach and ability to think algorithmically, they also expect a certain level of proficiency in writing clean, error-free code. Syntax errors can:
- Distract from your overall solution
- Waste valuable interview time
- Indicate a lack of attention to detail
- Potentially mask the correctness of your algorithm
By minimizing syntax errors, you can present your solutions more clearly and confidently, allowing the interviewer to focus on your problem-solving skills rather than getting caught up in minor coding mistakes.
Common Syntax Errors to Watch Out For
Let’s start by identifying some of the most common syntax errors that candidates make during coding interviews. Being aware of these pitfalls is the first step in avoiding them:
1. Missing or Misplaced Semicolons
In languages like Java, C++, or JavaScript, forgetting to end statements with semicolons is a frequent error. For example:
// Incorrect
var x = 5
console.log(x)
// Correct
var x = 5;
console.log(x);
2. Incorrect Indentation
Proper indentation is crucial for readability and, in some languages like Python, it’s syntactically important. For instance:
# Incorrect (Python)
def calculate_sum(a, b):
return a + b
# Correct
def calculate_sum(a, b):
return a + b
3. Mismatched Parentheses, Brackets, or Braces
Forgetting to close parentheses, brackets, or braces is a common mistake that can lead to syntax errors:
// Incorrect
if (x > 0 {
console.log("Positive";
}
// Correct
if (x > 0) {
console.log("Positive");
}
4. Using Assignment (=) Instead of Comparison (==) in Conditionals
This is a subtle but common error, especially in languages that use ‘==’ for equality comparison:
// Incorrect
if (x = 5) {
// Do something
}
// Correct
if (x == 5) {
// Do something
}
5. Forgetting to Declare Variables
In some languages, using a variable without declaring it first can lead to errors:
// Incorrect (JavaScript in strict mode)
x = 10;
// Correct
let x = 10;
6. Off-by-One Errors in Loops
These errors often occur when dealing with array indices or loop boundaries:
// Incorrect (accessing out of bounds)
for (int i = 0; i <= arr.length; i++) {
// Do something with arr[i]
}
// Correct
for (int i = 0; i < arr.length; i++) {
// Do something with arr[i]
}
Strategies to Avoid Syntax Errors
Now that we’ve identified common syntax errors, let’s explore strategies to help you avoid them during coding interviews:
1. Practice, Practice, Practice
The most effective way to avoid syntax errors is to write code regularly. The more you code, the more natural the syntax becomes. Here are some ways to incorporate practice into your routine:
- Solve coding problems on platforms like AlgoCademy, LeetCode, or HackerRank daily
- Participate in coding competitions or hackathons
- Work on personal coding projects
- Contribute to open-source projects
2. Use a Consistent Coding Style
Adopting a consistent coding style helps reduce errors and improves readability. Consider the following tips:
- Use meaningful variable and function names
- Maintain consistent indentation (use spaces or tabs, but not both)
- Follow language-specific style guides (e.g., PEP 8 for Python, Google’s Java Style Guide)
- Use proper spacing around operators and after commas
3. Leverage IDE Features
While you may not have access to a full-featured IDE during the interview, practicing with one can help you develop good habits. Modern IDEs offer features like:
- Syntax highlighting
- Auto-completion
- Real-time error detection
- Code formatting tools
Familiarize yourself with these features and try to internalize the correct syntax they suggest.
4. Write Code in Small, Testable Chunks
Instead of writing a large block of code at once, break your solution into smaller, manageable pieces. This approach allows you to:
- Test each part individually
- Identify and fix errors more easily
- Maintain focus on one concept at a time
5. Use Comments and Pseudocode
Before diving into the actual code, outline your approach using comments or pseudocode. This can help you:
- Organize your thoughts
- Spot potential issues before they become syntax errors
- Demonstrate your problem-solving process to the interviewer
6. Read Your Code Aloud
After writing a section of code, read it aloud to yourself. This technique can help you:
- Catch missing semicolons or parentheses
- Identify logical errors
- Ensure your code makes sense
7. Learn Keyboard Shortcuts
Familiarize yourself with keyboard shortcuts for common coding actions. This can help you:
- Write code more efficiently
- Reduce the likelihood of typos
- Quickly navigate and edit your code
8. Use Online Code Editors for Practice
Many coding interviews are conducted using online code editors. Practice using platforms similar to what you might encounter in an interview, such as:
- CoderPad
- HackerRank’s code editor
- LeetCode’s coding environment
This will help you get comfortable with the limitations and features of these platforms.
Language-Specific Tips
Different programming languages have their own quirks and common pitfalls. Here are some language-specific tips to help you avoid syntax errors:
Python
- Pay close attention to indentation
- Use colons (:) to denote the start of indented blocks
- Remember that comparison is done with ‘==’ not ‘=’
- Be mindful of the differences between lists and tuples
Java
- Always end statements with semicolons
- Declare variable types explicitly
- Remember to use ‘new’ keyword when instantiating objects
- Pay attention to method signatures, including return types
JavaScript
- Be aware of implicit type coercion
- Use ‘===’ for strict equality comparisons
- Remember to declare variables with ‘let’, ‘const’, or ‘var’
- Be cautious with asynchronous code and callbacks
C++
- Include necessary header files
- Remember to use semicolons to end statements
- Be mindful of pointer syntax and memory management
- Pay attention to the difference between ‘=’ and ‘==’
Handling Syntax Errors During the Interview
Despite your best efforts, you might still encounter syntax errors during the interview. Here’s how to handle them gracefully:
1. Stay Calm
Remember that making mistakes is normal, even for experienced programmers. Take a deep breath and approach the error methodically.
2. Read the Error Message
Most coding environments will provide error messages. Read them carefully as they often point directly to the issue.
3. Check Recent Changes
If you just added or modified code and an error appeared, focus on those recent changes first.
4. Use Print Statements
If allowed, use print statements to debug your code and understand what’s happening at different stages.
5. Explain Your Thought Process
As you debug, explain your thinking to the interviewer. This demonstrates your problem-solving skills and ability to communicate effectively.
6. Ask for Clarification
If you’re stuck or unsure about a particular syntax, it’s okay to ask the interviewer for clarification. They may provide hints or confirm the correct syntax.
Conclusion
Avoiding syntax errors during coding interviews is a skill that comes with practice and attention to detail. By following the strategies outlined in this guide and consistently working on your coding skills, you can significantly reduce the occurrence of these errors. Remember, the goal is not just to write error-free code, but to demonstrate your problem-solving abilities and thought process.
Platforms like AlgoCademy offer excellent resources for honing your coding skills and preparing for technical interviews. With interactive tutorials, AI-powered assistance, and a focus on algorithmic thinking, AlgoCademy can help you build the confidence and proficiency needed to tackle coding interviews with ease.
As you continue your journey in software development, keep in mind that even experienced programmers make syntax errors. The key is to learn from these mistakes, develop good coding habits, and continuously improve your skills. With dedication and practice, you’ll be well-prepared to showcase your abilities in your next coding interview, free from the distractions of common syntax errors.