Mastering Problem-Solving Speed for Coding Challenges: A Comprehensive Guide
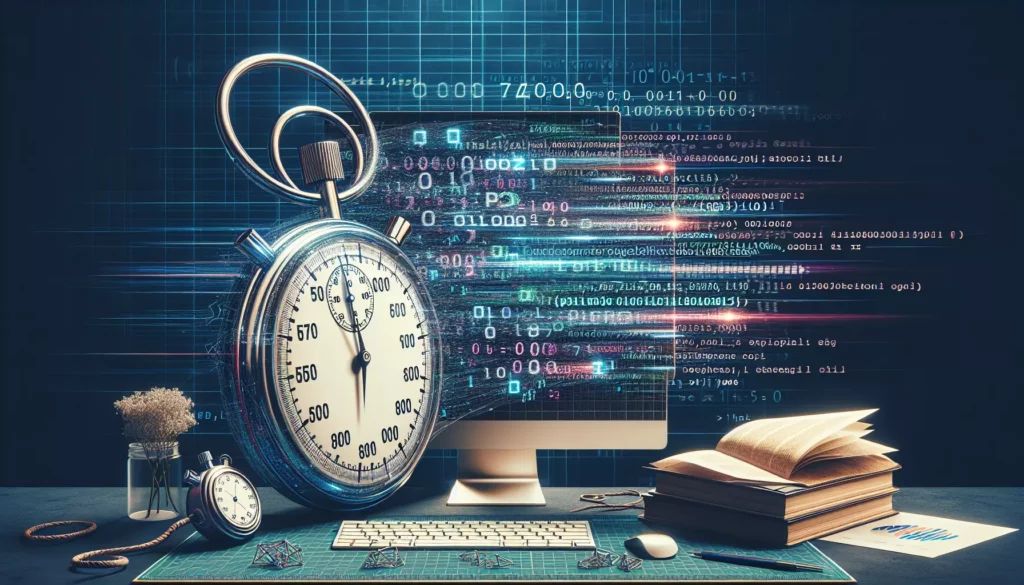
In the fast-paced world of software development and competitive programming, the ability to solve coding challenges quickly and efficiently is a highly sought-after skill. Whether you’re preparing for technical interviews at top tech companies like FAANG (Facebook, Amazon, Apple, Netflix, Google) or aiming to excel in coding competitions, improving your problem-solving speed is crucial. This comprehensive guide will explore various strategies and techniques to help you enhance your problem-solving abilities and tackle coding challenges with greater speed and accuracy.
Understanding the Importance of Problem-Solving Speed
Before diving into specific strategies, it’s essential to understand why problem-solving speed matters in the context of coding challenges:
- Technical Interviews: Many tech companies use timed coding challenges as part of their interview process. Being able to solve problems quickly demonstrates your ability to think on your feet and work efficiently under pressure.
- Competitive Programming: In coding competitions, speed is often a deciding factor. The faster you can solve problems, the more challenges you can tackle within the given time frame.
- Real-world Applications: In professional settings, the ability to quickly identify and solve problems can significantly impact your productivity and value to your team.
- Confidence Building: As you become faster at solving problems, your confidence in your coding abilities will grow, leading to improved performance in various coding-related tasks.
Strategies to Improve Problem-Solving Speed
1. Master the Fundamentals
A solid foundation in programming basics is crucial for quick problem-solving. Ensure you have a strong grasp of the following:
- Data structures (arrays, linked lists, trees, graphs, etc.)
- Algorithms (sorting, searching, dynamic programming, etc.)
- Time and space complexity analysis
- Basic mathematical concepts used in programming
Resources like AlgoCademy offer comprehensive tutorials and interactive coding exercises to help you strengthen your fundamental knowledge.
2. Practice Regularly
Consistent practice is key to improving your problem-solving speed. Here are some ways to incorporate regular practice into your routine:
- Daily Coding Challenges: Solve at least one coding problem every day. Platforms like LeetCode, HackerRank, and CodeForces offer a wide variety of challenges.
- Timed Practice Sessions: Set a timer and try to solve problems within a specific time frame to simulate interview or competition conditions.
- Mock Interviews: Participate in mock technical interviews to get used to solving problems under pressure.
- Coding Competitions: Join online coding contests to challenge yourself and learn from others.
3. Develop a Systematic Approach
Having a structured approach to problem-solving can significantly improve your speed. Follow these steps:
- Read and understand the problem thoroughly: Don’t rush into coding without fully grasping the requirements.
- Identify the input and expected output: Clarify what data you’re working with and what result you need to produce.
- Consider edge cases and constraints: Think about potential special cases or limitations that might affect your solution.
- Devise a high-level strategy: Outline your approach before diving into the implementation details.
- Implement your solution: Write clean, efficient code based on your strategy.
- Test and debug: Verify your solution with sample inputs and edge cases.
- Optimize if necessary: Look for ways to improve the time or space complexity of your solution.
4. Learn to Recognize Problem Patterns
Many coding challenges follow common patterns. By learning to recognize these patterns, you can quickly identify the appropriate solution strategy. Some common patterns include:
- Two-pointer technique
- Sliding window
- Depth-first search (DFS) and Breadth-first search (BFS)
- Dynamic programming
- Divide and conquer
- Greedy algorithms
Platforms like AlgoCademy often categorize problems by their underlying patterns, helping you develop pattern recognition skills.
5. Improve Your Coding Speed
While problem-solving is crucial, the ability to translate your thoughts into code quickly is equally important. Here are some tips to improve your coding speed:
- Master your programming language: Become proficient in the syntax and built-in functions of your chosen language.
- Use keyboard shortcuts: Learn and utilize shortcuts in your preferred code editor or IDE to minimize time spent on non-coding tasks.
- Practice typing: Improve your typing speed and accuracy to reduce the time spent on code input.
- Develop code templates: Create reusable code snippets for common operations to save time during implementation.
6. Analyze and Learn from Your Mistakes
After solving a problem, take time to reflect on your approach and identify areas for improvement:
- Review your solution and compare it with other efficient solutions.
- Analyze where you spent the most time during the problem-solving process.
- Identify concepts or algorithms you struggled with and focus on strengthening those areas.
- Keep a log of problems you’ve solved and revisit them periodically to reinforce your learning.
7. Utilize Visualization Tools
Visual aids can help you understand complex algorithms and data structures more quickly. Use tools like:
- Whiteboards or paper: Sketch out your ideas and algorithm flow before coding.
- Online visualization tools: Websites like VisuAlgo offer interactive visualizations of various algorithms and data structures.
- Debugger visualizations: Many IDEs provide visual representations of data structures during debugging, which can help you understand your code’s behavior more quickly.
8. Collaborate and Learn from Others
Engaging with other programmers can significantly accelerate your learning and problem-solving skills:
- Join coding communities: Participate in forums, Discord servers, or local meetups focused on competitive programming or interview preparation.
- Pair programming: Practice solving problems with a partner to gain new perspectives and learn different approaches.
- Code reviews: Share your solutions with others and ask for feedback to identify areas for improvement.
- Study high-quality solutions: After solving a problem, review top-rated solutions to learn alternative approaches and optimizations.
9. Focus on Time Management
Effective time management is crucial when solving coding challenges under time constraints:
- Allocate time wisely: Divide your available time between understanding the problem, devising a strategy, implementing the solution, and testing.
- Learn when to move on: If you’re stuck on a problem, know when to skip it and come back later rather than wasting too much time.
- Practice with a timer: Regularly solve problems under timed conditions to improve your time management skills.
10. Develop a Growth Mindset
Approach problem-solving with a positive and growth-oriented mindset:
- Embrace challenges as opportunities to learn and improve.
- Don’t get discouraged by difficult problems; view them as chances to expand your skills.
- Celebrate your progress and small victories along the way.
- Stay motivated by setting achievable goals and tracking your improvement over time.
Implementing These Strategies with AlgoCademy
AlgoCademy provides a comprehensive platform to help you implement many of these strategies effectively:
- Structured Learning Path: Follow a curated curriculum that covers fundamental concepts and gradually increases in complexity.
- Interactive Coding Exercises: Practice coding challenges with immediate feedback and step-by-step guidance.
- AI-Powered Assistance: Receive personalized hints and explanations to help you overcome obstacles quickly.
- Problem Pattern Recognition: Learn to identify common problem patterns through categorized challenges and explanations.
- Performance Tracking: Monitor your progress and identify areas for improvement with detailed analytics.
- Community Features: Collaborate with other learners, share solutions, and participate in discussions to enhance your learning experience.
Sample Problem-Solving Approach
Let’s walk through a sample problem to demonstrate how to apply these strategies in practice:
Problem: Two Sum
Given an array of integers nums
and an integer target
, return indices of the two numbers such that they add up to target
. You may assume that each input would have exactly one solution, and you may not use the same element twice.
Step 1: Understand the Problem
- Input: An array of integers and a target sum
- Output: Indices of two numbers that add up to the target
- Constraints: Each input has exactly one solution, can’t use the same element twice
Step 2: Consider Approaches
- Brute Force: Check all pairs of numbers (O(n^2) time complexity)
- Hash Table: Use a hash map to store complements (O(n) time complexity)
Step 3: Implement the Solution
Let’s implement the hash table approach for better time complexity:
class Solution:
def twoSum(self, nums: List[int], target: int) -> List[int]:
num_map = {}
for i, num in enumerate(nums):
complement = target - num
if complement in num_map:
return [num_map[complement], i]
num_map[num] = i
return [] # No solution found
Step 4: Test the Solution
Test with sample inputs:
nums = [2, 7, 11, 15]
target = 9
result = Solution().twoSum(nums, target)
print(result) # Expected output: [0, 1]
nums = [3, 2, 4]
target = 6
result = Solution().twoSum(nums, target)
print(result) # Expected output: [1, 2]
Step 5: Analyze and Optimize
The current solution has:
- Time Complexity: O(n) – we iterate through the array once
- Space Complexity: O(n) – in the worst case, we store n-1 elements in the hash map
This solution is already optimized for time complexity. However, if space is a concern, we could consider the two-pointer approach for sorted arrays, which would have O(1) space complexity but require sorting the array first.
Conclusion
Improving your problem-solving speed for coding challenges is a journey that requires dedication, practice, and a systematic approach. By mastering the fundamentals, practicing regularly, developing a structured problem-solving method, and utilizing resources like AlgoCademy, you can significantly enhance your ability to tackle coding challenges quickly and effectively.
Remember that improvement takes time, and it’s essential to stay patient and persistent. Celebrate your progress along the way, and don’t be discouraged by difficult problems – they are opportunities to learn and grow. With consistent effort and the right strategies, you’ll be well-equipped to excel in technical interviews, coding competitions, and real-world programming challenges.
Keep pushing yourself, stay curious, and enjoy the process of becoming a more efficient and skilled problem solver. Your efforts will pay off not only in coding challenges but also in your overall growth as a software developer.