Decoding the Complexity: Why Object-Oriented Programming Concepts Can Be Confusing
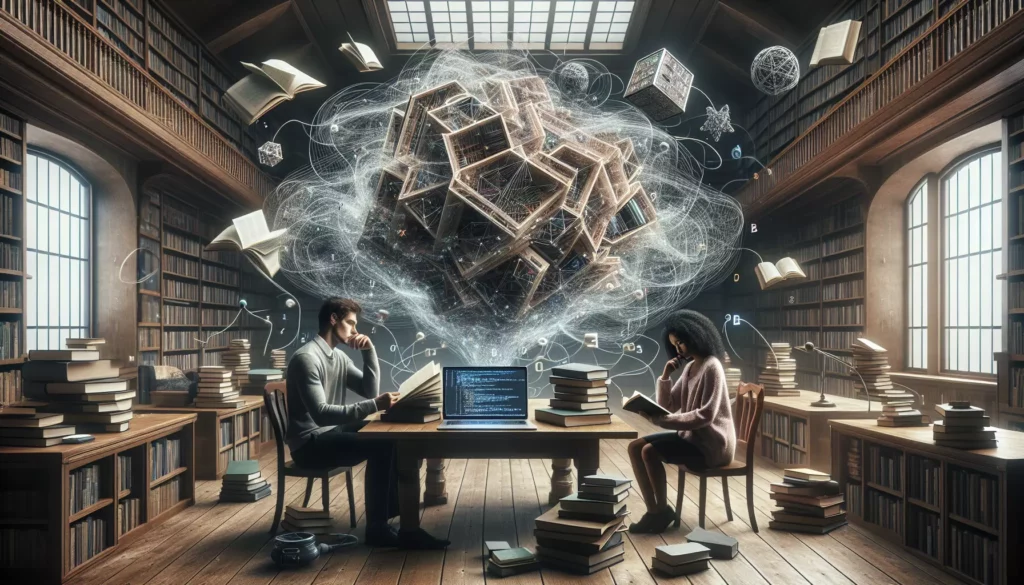
Object-Oriented Programming (OOP) is a fundamental paradigm in modern software development, powering countless applications and systems we use daily. Yet, for many aspiring programmers and even some experienced developers, OOP concepts can be a source of confusion and frustration. In this comprehensive guide, we’ll explore why OOP can be challenging to grasp and provide strategies to overcome these hurdles.
The Essence of Object-Oriented Programming
Before diving into the complexities, let’s briefly recap what OOP is all about. Object-Oriented Programming is a programming paradigm based on the concept of “objects,” which can contain data in the form of fields (attributes or properties) and code in the form of procedures (methods). The main principles of OOP include:
- Encapsulation
- Inheritance
- Polymorphism
- Abstraction
These principles aim to organize code into reusable, modular structures that model real-world entities or abstract concepts. While this approach offers numerous benefits, it also introduces layers of complexity that can be challenging for learners to navigate.
Why OOP Concepts Can Be Confusing
1. Abstract Thinking Required
One of the primary reasons OOP concepts can be confusing is that they require a high level of abstract thinking. Unlike procedural programming, where you simply list out steps for the computer to follow, OOP asks you to think in terms of objects, their properties, and their interactions.
For instance, consider modeling a car in OOP:
class Car {
private String make;
private String model;
private int year;
public void startEngine() {
// Method implementation
}
public void accelerate() {
// Method implementation
}
}
This abstraction requires you to think about what a car “is” (its attributes) and what it can “do” (its methods). For beginners, this shift from concrete, step-by-step thinking to abstract, object-based thinking can be a significant mental leap.
2. Multiple Layers of Complexity
OOP introduces several layers of complexity that interact with each other. Understanding each concept individually might be manageable, but grasping how they all work together can be overwhelming. For example:
- Encapsulation involves hiding internal details and providing a public interface.
- Inheritance allows creating new classes based on existing ones.
- Polymorphism enables objects of different types to be treated uniformly.
- Abstraction focuses on essential features while hiding unnecessary details.
Each of these concepts is powerful on its own, but when combined, they create a complex system that can be difficult to visualize and understand fully.
3. Paradigm Shift from Procedural Programming
Many learners come to OOP with a background in procedural programming. The shift from thinking in terms of procedures and functions to objects and methods can be jarring. In procedural programming, the focus is on writing functions that operate on data. In OOP, the data and the functions that operate on it are bundled together into objects.
Consider this procedural approach:
int[] numbers = {1, 2, 3, 4, 5};
int sum = calculateSum(numbers);
function calculateSum(int[] arr) {
int total = 0;
for (int num : arr) {
total += num;
}
return total;
}
Now, compare it to an OOP approach:
class NumberArray {
private int[] numbers;
public NumberArray(int[] nums) {
this.numbers = nums;
}
public int calculateSum() {
int total = 0;
for (int num : numbers) {
total += num;
}
return total;
}
}
NumberArray arr = new NumberArray(new int[]{1, 2, 3, 4, 5});
int sum = arr.calculateSum();
This paradigm shift requires a fundamental change in how one approaches problem-solving and code organization.
4. Conceptual Overhead
OOP introduces a lot of new terminology and concepts that can be overwhelming for beginners. Terms like classes, objects, instances, constructors, and methods all need to be understood before one can start writing effective OOP code. This conceptual overhead can be a significant barrier to entry.
For example, understanding the difference between a class and an object can be confusing:
// Class definition
class Dog {
String name;
String breed;
void bark() {
System.out.println("Woof!");
}
}
// Creating objects (instances) of the class
Dog dog1 = new Dog();
dog1.name = "Buddy";
dog1.breed = "Labrador";
Dog dog2 = new Dog();
dog2.name = "Max";
dog2.breed = "German Shepherd";
Here, Dog
is a class (a blueprint), while dog1
and dog2
are objects (instances of that blueprint). This distinction is crucial but can be confusing for newcomers.
5. Design Patterns and Best Practices
OOP isn’t just about syntax; it’s also about design patterns and best practices. Concepts like the SOLID principles, design patterns (e.g., Singleton, Factory, Observer), and architectural patterns add another layer of complexity. These patterns are essential for writing maintainable and scalable code, but they require experience and deep understanding to apply effectively.
For instance, consider the Singleton pattern:
public class Singleton {
private static Singleton instance;
private Singleton() {}
public static Singleton getInstance() {
if (instance == null) {
instance = new Singleton();
}
return instance;
}
}
Understanding why and when to use such patterns can be challenging for those new to OOP.
6. Language-Specific Implementations
While OOP concepts are generally universal, their implementation can vary significantly between programming languages. This variation can lead to confusion, especially when learning multiple languages or transitioning between them.
For example, Python’s approach to OOP looks quite different from Java’s:
Python:
class Dog:
def __init__(self, name, breed):
self.name = name
self.breed = breed
def bark(self):
print("Woof!")
dog = Dog("Buddy", "Labrador")
dog.bark()
Java:
public class Dog {
private String name;
private String breed;
public Dog(String name, String breed) {
this.name = name;
this.breed = breed;
}
public void bark() {
System.out.println("Woof!");
}
}
Dog dog = new Dog("Buddy", "Labrador");
dog.bark();
These differences in syntax and conventions can be a source of confusion for learners trying to grasp OOP concepts across different languages.
Strategies to Overcome OOP Confusion
While OOP concepts can be challenging, there are several strategies that can help in understanding and mastering them:
1. Start with the Basics
Begin with the fundamental concepts of OOP before diving into more complex topics. Understand what objects and classes are, how methods work, and the basic principles of encapsulation before moving on to inheritance and polymorphism.
2. Use Real-World Analogies
OOP is designed to model real-world entities and concepts. Use analogies to help understand OOP principles. For example, think of a class as a blueprint for a house, and objects as the actual houses built from that blueprint.
3. Practice, Practice, Practice
OOP concepts become clearer with practice. Start with simple projects and gradually increase complexity. Implement small programs that use classes and objects, then move on to more complex systems that utilize inheritance and polymorphism.
4. Visualize the Concepts
Use diagrams and visual representations to understand relationships between classes and objects. UML (Unified Modeling Language) diagrams can be particularly helpful in visualizing class hierarchies and object interactions.
5. Learn Design Patterns Gradually
Don’t try to learn all design patterns at once. Start with a few common patterns and understand their use cases. As you gain experience, you’ll naturally encounter situations where different patterns are applicable.
6. Embrace the Learning Curve
Recognize that OOP is a complex paradigm and it’s normal to feel confused initially. Embrace the learning curve and be patient with yourself as you develop your understanding.
7. Collaborate and Discuss
Join coding communities, participate in forums, and discuss OOP concepts with peers. Explaining concepts to others and hearing different perspectives can significantly enhance your understanding.
8. Review and Refactor Code
Regularly review and refactor your code. This practice helps in understanding how OOP principles can improve code quality and maintainability.
The Role of OOP in Modern Software Development
Despite its complexities, OOP remains a crucial paradigm in modern software development. Its benefits include:
- Improved code organization and modularity
- Enhanced code reusability
- Better data security through encapsulation
- Easier maintenance and scalability of large projects
- More intuitive modeling of real-world problems
These advantages make OOP an essential skill for developers, particularly those aiming to work on large-scale applications or in team environments.
OOP in the Context of Technical Interviews
For those preparing for technical interviews, especially with major tech companies, a solid understanding of OOP is crucial. Interviewers often assess candidates’ ability to design systems using OOP principles. Common interview questions might include:
- Designing a parking lot system
- Implementing a deck of cards
- Creating a basic file system structure
These questions test not just coding skills, but also the ability to apply OOP concepts to real-world scenarios.
Conclusion
Object-Oriented Programming, while powerful and widely used, can indeed be confusing due to its abstract nature, multiple layers of complexity, and the paradigm shift it represents from procedural programming. However, with the right approach, patience, and consistent practice, these concepts can be mastered.
Remember, the journey to understanding OOP is as important as the destination. Each challenge you overcome in grasping these concepts contributes to your growth as a programmer. Whether you’re a beginner just starting out or an experienced developer looking to deepen your understanding, embracing the complexities of OOP will ultimately make you a more versatile and effective programmer.
As you continue your coding journey, platforms like AlgoCademy can be invaluable resources. With interactive tutorials, AI-powered assistance, and a focus on practical coding skills, AlgoCademy can help you navigate the complexities of OOP and prepare you for the challenges of technical interviews and real-world programming tasks.
Keep coding, keep learning, and remember that every great programmer once struggled with these same concepts. Your persistence and dedication will pay off as you unlock the full potential of Object-Oriented Programming in your development career.