The Ultimate Guide to Acing Your MAANG Coding Interview
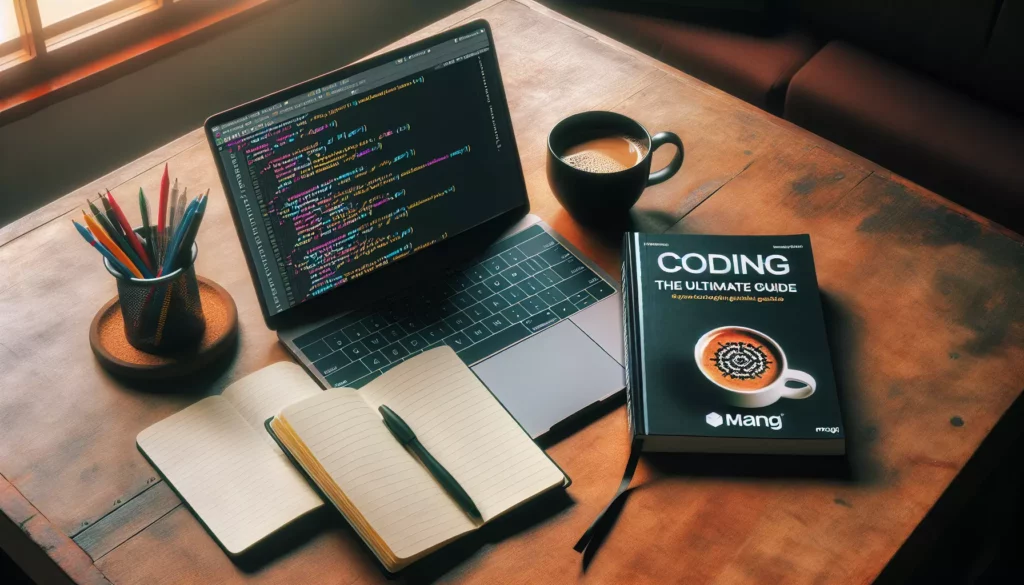
If you’re aiming for a career at one of the tech giants like Meta (formerly Facebook), Amazon, Apple, Netflix, or Google – collectively known as MAANG – you’re probably aware that their coding interviews are notoriously challenging. These companies are known for their rigorous selection process, particularly when it comes to technical skills. But don’t worry! With the right preparation strategy, you can significantly increase your chances of success. In this comprehensive guide, we’ll walk you through the best ways to prepare for a MAANG coding interview, covering everything from fundamental concepts to advanced techniques and essential soft skills.
Understanding the MAANG Interview Process
Before diving into preparation strategies, it’s crucial to understand what you’re up against. MAANG companies typically follow a multi-stage interview process:
- Initial Screening: Usually a phone or video call with a recruiter to discuss your background and interest in the role.
- Technical Phone Screen: A coding interview conducted remotely, often using a shared coding platform.
- On-site Interviews: Multiple rounds of face-to-face (or virtual) interviews, including coding challenges, system design discussions, and behavioral questions.
- Final Decision: The hiring committee reviews all feedback to make a decision.
The coding interviews, which are the focus of this guide, typically involve solving algorithmic problems in real-time while explaining your thought process.
Essential Areas to Master
To excel in a MAANG coding interview, you need to be well-versed in several key areas:
1. Data Structures
A solid understanding of data structures is fundamental. Focus on:
- Arrays and Strings
- Linked Lists
- Stacks and Queues
- Trees (Binary Trees, Binary Search Trees, Balanced Trees)
- Graphs
- Hash Tables
- Heaps
2. Algorithms
Familiarize yourself with common algorithmic techniques:
- Sorting (QuickSort, MergeSort, HeapSort)
- Searching (Binary Search, Depth-First Search, Breadth-First Search)
- Dynamic Programming
- Greedy Algorithms
- Recursion and Backtracking
- Divide and Conquer
3. Big O Notation
Understanding time and space complexity is crucial. Be prepared to analyze the efficiency of your solutions and discuss trade-offs between different approaches.
4. Problem-Solving Techniques
Develop a systematic approach to problem-solving:
- Clarify the problem and requirements
- Work through examples
- Break down complex problems into smaller, manageable parts
- Identify patterns and edge cases
- Formulate a solution before coding
Effective Preparation Strategies
Now that we know what to focus on, let’s discuss how to prepare effectively:
1. Practice, Practice, Practice
Consistent practice is key to success. Here are some ways to incorporate regular practice into your routine:
- Online Coding Platforms: Websites like LeetCode, HackerRank, and CodeSignal offer a vast array of coding problems, many of which are similar to those asked in MAANG interviews.
- Daily Coding Challenge: Set a goal to solve at least one problem every day. This helps build consistency and keeps your skills sharp.
- Mock Interviews: Platforms like Pramp or interviewing.io allow you to participate in mock interviews with peers, simulating real interview conditions.
2. Study Core Computer Science Concepts
While practicing problems is essential, don’t neglect the underlying theory. Review fundamental computer science concepts:
- Operating Systems
- Database Management Systems
- Computer Networks
- Object-Oriented Programming
- Design Patterns
These topics may come up in system design discussions or as part of more complex coding problems.
3. Master Your Programming Language
Choose a programming language you’re comfortable with and become proficient in it. While most MAANG companies allow you to code in your preferred language, it’s crucial to have a deep understanding of its syntax, standard libraries, and best practices. Popular choices include:
- Python
- Java
- C++
- JavaScript
Be prepared to write clean, efficient code without relying heavily on IDE features or documentation.
4. Implement Data Structures from Scratch
To truly understand data structures, try implementing them from scratch. This exercise will deepen your knowledge and prepare you for questions that require modifying or optimizing standard data structures. For example, try implementing:
- A hash table with collision resolution
- A balanced binary search tree (like a Red-Black tree or AVL tree)
- A priority queue using a heap
5. Study and Implement Classic Algorithms
Similarly, implement classic algorithms to understand their inner workings. Some examples include:
- Dijkstra’s algorithm for finding the shortest path in a graph
- The Knuth-Morris-Pratt (KMP) algorithm for string matching
- The Floyd-Warshall algorithm for finding all shortest paths in a graph
6. Focus on Problem-Solving Techniques
Develop a systematic approach to problem-solving. When practicing, follow these steps:
- Read the problem carefully and ask clarifying questions
- Work through examples, including edge cases
- Brainstorm possible approaches
- Choose an approach and outline the algorithm
- Implement the solution
- Test your code with various inputs
- Analyze the time and space complexity
- Discuss potential optimizations or alternative approaches
7. Practice Explaining Your Thought Process
In MAANG interviews, how you approach a problem is often as important as solving it. Practice thinking out loud while coding. Explain your thought process, discuss trade-offs, and articulate your reasoning for choosing a particular approach.
8. Familiarize Yourself with Coding Environments
Many technical interviews are conducted using online coding platforms or whiteboards. Practice coding without the assistance of an IDE to simulate interview conditions. Get comfortable with:
- Writing code on a whiteboard (or a digital equivalent)
- Coding in a simple text editor without auto-completion or syntax highlighting
- Using online coding platforms like CoderPad or HackerRank’s CodePair
Advanced Preparation Techniques
Once you’ve mastered the basics, consider these advanced preparation techniques:
1. Tackle Company-Specific Questions
Each MAANG company has its own focus and preferred question types. Research and practice questions that are frequently asked by your target company. Websites like LeetCode often have company-specific problem sets.
2. Study System Design
For more senior positions, system design questions are common. Study topics like:
- Scalability and Load Balancing
- Database Sharding
- Caching Strategies
- Microservices Architecture
- Distributed Systems
3. Explore Machine Learning and AI (if relevant)
If you’re applying for roles that involve machine learning or AI, make sure to brush up on relevant concepts:
- Basic ML algorithms (Linear Regression, Decision Trees, Neural Networks)
- Feature Engineering
- Model Evaluation Techniques
- Deep Learning Frameworks (TensorFlow, PyTorch)
4. Contribute to Open Source Projects
Contributing to open source projects can help you gain real-world coding experience and demonstrate your ability to work on large codebases. It’s also a great talking point during interviews.
5. Build Personal Projects
Develop personal projects that showcase your skills and passion for coding. This can set you apart from other candidates and provide concrete examples of your abilities during interviews.
Soft Skills and Interview Etiquette
While technical skills are crucial, don’t underestimate the importance of soft skills and proper interview etiquette:
1. Communication
Practice articulating your thoughts clearly and concisely. Be prepared to explain complex technical concepts in simple terms.
2. Teamwork
MAANG companies value collaboration. Be ready to discuss your experiences working in a team and how you handle conflicts or challenges.
3. Adaptability
Show that you’re open to feedback and can adapt your approach when faced with new information or constraints.
4. Curiosity
Demonstrate a genuine interest in technology and a desire to learn. Ask thoughtful questions about the company and the role.
5. Interview Etiquette
- Be punctual and dress appropriately (even for virtual interviews)
- Listen carefully to the interviewer and ask for clarification when needed
- Stay calm under pressure and maintain a positive attitude
- Follow up with a thank-you email after the interview
Sample Coding Problem and Solution
To illustrate the problem-solving process, let’s walk through a sample coding problem that might come up in a MAANG interview:
Problem: Longest Palindromic Substring
Given a string s, find the longest palindromic substring in s. You may assume that the maximum length of s is 1000.
Example:
Input: "babad"
Output: "bab"
Note: "aba" is also a valid answer.
Input: "cbbd"
Output: "bb"
Solution Approach
We’ll use the dynamic programming approach to solve this problem efficiently:
- Create a 2D boolean array dp[n][n] where n is the length of the string.
- Initialize all substrings of length 1 as palindromes.
- Iterate through different lengths (2 to n) and fill the dp table.
- Keep track of the longest palindrome found.
Python Implementation
def longestPalindrome(s: str) -> str:
n = len(s)
# Create a table to store results of subproblems
dp = [[False for _ in range(n)] for _ in range(n)]
# All substrings of length 1 are palindromes
for i in range(n):
dp[i][i] = True
start = 0
max_length = 1
# Check for substrings of length 2
for i in range(n-1):
if s[i] == s[i+1]:
dp[i][i+1] = True
start = i
max_length = 2
# Check for lengths greater than 2
for length in range(3, n+1):
for i in range(n-length+1):
j = i + length - 1
if s[i] == s[j] and dp[i+1][j-1]:
dp[i][j] = True
if length > max_length:
start = i
max_length = length
return s[start:start+max_length]
# Test the function
print(longestPalindrome("babad")) # Output: "bab" or "aba"
print(longestPalindrome("cbbd")) # Output: "bb"
Explanation
This solution uses dynamic programming to build up palindromes from smaller substrings. The time complexity is O(n^2) and space complexity is also O(n^2), where n is the length of the input string.
In an interview, you would explain your thought process, discuss the time and space complexity, and possibly explore alternative solutions (like the Manacher’s algorithm for O(n) time complexity).
Final Tips for Interview Day
As your interview day approaches, keep these final tips in mind:
- Get a good night’s sleep: Being well-rested will help you think clearly during the interview.
- Review your past solutions: Go over some of the problems you’ve solved during your preparation.
- Prepare questions for the interviewer: This shows your interest in the role and the company.
- Stay calm and confident: Remember, you’ve prepared well. Trust in your abilities.
- Be yourself: MAANG companies are not just looking for technical skills, but also for individuals who fit their culture.
Conclusion
Preparing for a MAANG coding interview is a challenging but rewarding process. It requires dedication, consistent practice, and a strategic approach to learning. By focusing on core computer science concepts, honing your problem-solving skills, and practicing regularly, you can significantly increase your chances of success.
Remember, the journey doesn’t end with the interview. Even if you don’t succeed on your first attempt, the skills you develop during this preparation process will serve you well throughout your career. Keep learning, stay curious, and don’t be afraid to tackle challenging problems. With persistence and the right mindset, you’ll be well on your way to landing your dream job at a MAANG company.
Good luck with your preparation, and may your coding interviews be successful!