Spatial Computing: Integrating Spatial Mapping and Gesture Recognition in Apps
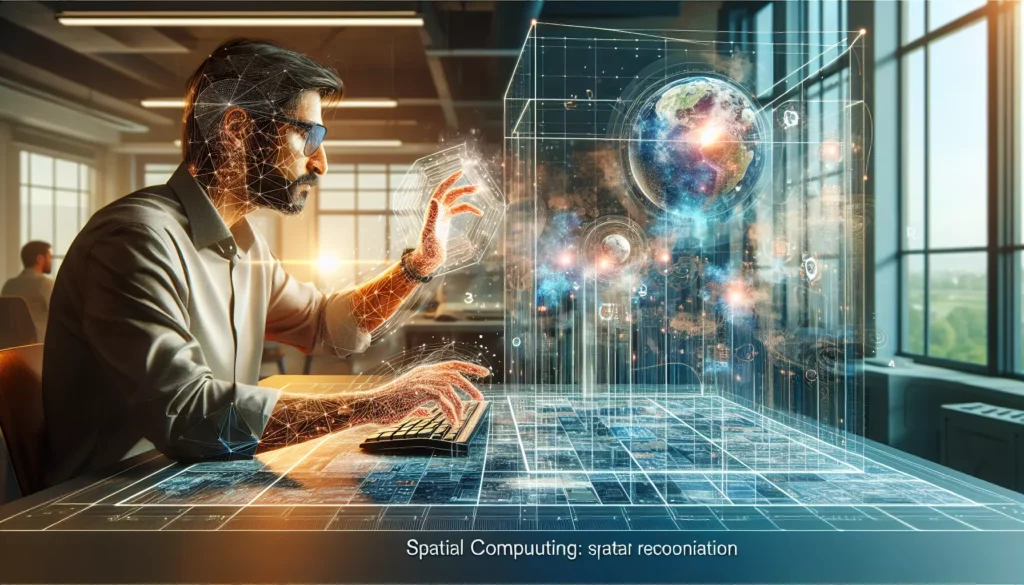
Welcome to AlgoCademy’s comprehensive guide on spatial computing, with a focus on integrating spatial mapping and gesture recognition in apps. As we continue to push the boundaries of technology, spatial computing is emerging as a groundbreaking field that’s reshaping how we interact with digital environments. In this article, we’ll dive deep into the world of spatial computing, exploring its core concepts, applications, and how you can start implementing these exciting technologies in your own projects.
Table of Contents
- What is Spatial Computing?
- Spatial Mapping: Understanding the Environment
- Gesture Recognition: Natural Interaction
- Integrating Spatial Mapping and Gesture Recognition
- Real-world Applications of Spatial Computing
- Getting Started with Spatial Computing
- Challenges and Considerations
- The Future of Spatial Computing
- Conclusion
1. What is Spatial Computing?
Spatial computing is an emerging technology paradigm that bridges the gap between our physical world and the digital realm. It involves creating immersive experiences by understanding and interacting with the three-dimensional space around us. At its core, spatial computing combines various technologies such as augmented reality (AR), virtual reality (VR), computer vision, and artificial intelligence to create a seamless blend of the physical and digital worlds.
The key components of spatial computing include:
- Spatial Mapping: Understanding and digitally representing the physical environment
- Gesture Recognition: Interpreting human movements and gestures as input
- 3D Visualization: Rendering digital content in three-dimensional space
- Spatial Audio: Creating immersive sound experiences based on the user’s position
- Object Recognition: Identifying and tracking real-world objects in the environment
By combining these elements, spatial computing enables us to create applications that can understand and interact with the physical world in ways that were previously impossible. This opens up a whole new realm of possibilities for developers, designers, and businesses across various industries.
2. Spatial Mapping: Understanding the Environment
Spatial mapping is a fundamental aspect of spatial computing that involves creating a digital representation of the physical environment. This process allows applications to understand the layout, dimensions, and objects present in the real world, enabling them to interact with and augment the space in meaningful ways.
Key Concepts in Spatial Mapping
- Point Clouds: A collection of 3D points that represent the surfaces of objects in the environment
- Mesh Generation: Creating a 3D mesh from the point cloud data to represent surfaces
- Occlusion: Handling the visibility of virtual objects based on real-world obstacles
- Persistence: Saving and loading spatial maps for future use
Implementing Spatial Mapping
To implement spatial mapping in your applications, you’ll need to use specialized hardware (such as depth sensors or stereo cameras) and software libraries. Here’s a basic example of how you might start implementing spatial mapping using Unity and the ARFoundation framework:
using UnityEngine;
using UnityEngine.XR.ARFoundation;
public class SpatialMappingManager : MonoBehaviour
{
public ARMeshManager meshManager;
void Start()
{
meshManager = GetComponent<ARMeshManager>();
meshManager.meshPrefab = Resources.Load<GameObject>("MeshPrefab");
}
void Update()
{
// Update mesh generation settings
meshManager.density = 1f; // Adjust density as needed
meshManager.normals = true; // Generate normals for better lighting
}
}
This simple script sets up an ARMeshManager component to handle spatial mapping in an AR application. It loads a mesh prefab and configures some basic settings for mesh generation.
3. Gesture Recognition: Natural Interaction
Gesture recognition is another crucial component of spatial computing that allows users to interact with digital content using natural hand movements and gestures. This technology enables more intuitive and immersive user experiences by eliminating the need for traditional input devices like keyboards or controllers.
Types of Gestures
- Static Gestures: Specific hand poses or configurations (e.g., peace sign, thumbs up)
- Dynamic Gestures: Movements over time (e.g., swiping, pinching to zoom)
- Multi-touch Gestures: Interactions using multiple fingers or hands
Implementing Gesture Recognition
Implementing gesture recognition typically involves using computer vision techniques and machine learning models. Here’s a simplified example of how you might implement a basic gesture recognition system using OpenCV and Python:
import cv2
import numpy as np
import mediapipe as mp
mp_hands = mp.solutions.hands
hands = mp_hands.Hands()
mp_draw = mp.solutions.drawing_utils
cap = cv2.VideoCapture(0)
while True:
success, image = cap.read()
image_rgb = cv2.cvtColor(image, cv2.COLOR_BGR2RGB)
results = hands.process(image_rgb)
if results.multi_hand_landmarks:
for hand_landmarks in results.multi_hand_landmarks:
mp_draw.draw_landmarks(image, hand_landmarks, mp_hands.HAND_CONNECTIONS)
# Get landmark positions
landmarks = [(lm.x, lm.y) for lm in hand_landmarks.landmark]
# Implement gesture recognition logic here
# For example, check the distance between thumb tip and index finger tip
thumb_tip = landmarks[4]
index_tip = landmarks[8]
distance = np.sqrt((thumb_tip[0] - index_tip[0])**2 + (thumb_tip[1] - index_tip[1])**2)
if distance < 0.1:
cv2.putText(image, "Pinch detected", (10, 30), cv2.FONT_HERSHEY_SIMPLEX, 1, (0, 255, 0), 2)
cv2.imshow("Hand Tracking", image)
if cv2.waitKey(1) & 0xFF == ord('q'):
break
cap.release()
cv2.destroyAllWindows()
This example uses the MediaPipe library to detect hand landmarks and implements a simple pinch gesture detection based on the distance between the thumb and index finger tips.
4. Integrating Spatial Mapping and Gesture Recognition
The true power of spatial computing comes from combining spatial mapping and gesture recognition to create truly immersive and interactive experiences. By integrating these technologies, you can create applications that allow users to manipulate virtual objects in the context of their physical environment.
Key Considerations for Integration
- Coordinate System Alignment: Ensure that the gesture recognition system’s coordinate space aligns with the spatial mapping’s coordinate space
- Performance Optimization: Balance the computational requirements of both systems to maintain smooth performance
- User Experience Design: Create intuitive interactions that leverage both the understanding of the environment and natural gesture input
Example Integration Scenario
Imagine creating an AR interior design application that allows users to place virtual furniture in their real-world space. Here’s a high-level overview of how you might integrate spatial mapping and gesture recognition:
- Use spatial mapping to create a 3D representation of the user’s room
- Implement gesture recognition to detect hand movements and poses
- Allow users to select virtual furniture items using hand gestures
- Use the spatial map to place furniture on detected surfaces (e.g., floors, tables)
- Enable users to move, rotate, and scale furniture using gestures, while respecting the constraints of the physical environment
Here’s a pseudo-code example of how this integration might look:
class ARInteriorDesignApp:
def __init__(self):
self.spatial_map = SpatialMap()
self.gesture_recognizer = GestureRecognizer()
self.virtual_furniture = []
def update(self):
# Update spatial mapping
self.spatial_map.update()
# Process gesture input
gestures = self.gesture_recognizer.detect_gestures()
for gesture in gestures:
if gesture.type == "select":
self.select_furniture(gesture.position)
elif gesture.type == "move":
self.move_furniture(gesture.start_position, gesture.end_position)
elif gesture.type == "scale":
self.scale_furniture(gesture.scale_factor)
def select_furniture(self, position):
# Raycast from the gesture position to find intersecting furniture
selected_furniture = self.raycast(position)
if selected_furniture:
self.highlight_furniture(selected_furniture)
def move_furniture(self, start_position, end_position):
# Calculate movement delta
delta = end_position - start_position
# Move selected furniture
for furniture in self.selected_furniture:
new_position = furniture.position + delta
# Check for collisions with the spatial map
if not self.spatial_map.check_collision(furniture, new_position):
furniture.position = new_position
def scale_furniture(self, scale_factor):
# Scale selected furniture
for furniture in self.selected_furniture:
new_scale = furniture.scale * scale_factor
# Check if new scale fits within the spatial map constraints
if self.spatial_map.check_scale_valid(furniture, new_scale):
furniture.scale = new_scale
def raycast(self, position):
# Implement raycasting logic to find furniture at the given position
pass
def highlight_furniture(self, furniture):
# Implement furniture highlighting logic
pass
This pseudo-code demonstrates how spatial mapping and gesture recognition can work together to create an interactive AR experience. The spatial map is used to understand the environment and enforce constraints, while gesture recognition provides the user input for selecting and manipulating virtual objects.
5. Real-world Applications of Spatial Computing
Spatial computing has a wide range of applications across various industries. Here are some examples of how spatial computing is being used in different fields:
1. Architecture and Interior Design
- Visualizing building designs in real-world contexts
- Virtual staging of properties for real estate
- Interactive furniture placement and room layout planning
2. Education and Training
- Immersive historical reconstructions
- Interactive 3D models for science and engineering education
- Virtual training simulations for complex procedures
3. Healthcare
- Surgical planning and visualization
- Rehabilitation exercises with real-time feedback
- Medical imaging overlays for diagnosis and treatment
4. Manufacturing and Industry
- Assembly line optimization and worker guidance
- Remote expert assistance for maintenance and repairs
- Virtual prototyping and product design
5. Entertainment and Gaming
- Location-based AR games
- Immersive VR experiences
- Interactive art installations
6. Retail and E-commerce
- Virtual try-on for clothing and accessories
- AR product visualization in the home
- Interactive in-store displays and navigation
6. Getting Started with Spatial Computing
If you’re interested in diving into spatial computing development, here are some steps to get you started:
1. Choose a Development Platform
Select a platform that supports spatial computing features. Some popular options include:
- Unity with ARFoundation
- Unreal Engine with ARCore or ARKit plugins
- Web-based AR using frameworks like A-Frame or AR.js
2. Learn the Fundamentals
Familiarize yourself with key concepts in computer vision, 3D graphics, and AR/VR development. Some areas to focus on include:
- 3D mathematics (vectors, matrices, quaternions)
- Computer vision algorithms
- Shader programming
- AR/VR design principles
3. Experiment with SDKs and APIs
Try out various spatial computing SDKs and APIs to get hands-on experience:
- ARCore (Android)
- ARKit (iOS)
- Microsoft Mixed Reality Toolkit
- Vuforia
- OpenCV for computer vision tasks
4. Start with Simple Projects
Begin with small, focused projects to build your skills:
- Create an AR business card viewer
- Develop a simple gesture-controlled game
- Build an AR measuring tool using spatial mapping
5. Join the Community
Engage with the spatial computing community to learn from others and stay up-to-date:
- Attend AR/VR meetups and conferences
- Participate in online forums and discussion groups
- Follow spatial computing experts and companies on social media
6. Explore Advanced Topics
As you progress, delve into more advanced areas of spatial computing:
- SLAM (Simultaneous Localization and Mapping) algorithms
- Machine learning for gesture and object recognition
- Spatial audio implementation
- Haptic feedback integration
7. Challenges and Considerations
While spatial computing offers exciting possibilities, there are several challenges and considerations to keep in mind:
1. Hardware Limitations
- Processing power requirements for real-time spatial mapping and gesture recognition
- Battery life constraints on mobile devices
- Limitations of current AR/VR display technologies
2. Environmental Factors
- Varying lighting conditions affecting computer vision algorithms
- Complex or dynamic environments challenging spatial mapping accuracy
- Interference from reflective or transparent surfaces
3. User Experience Design
- Creating intuitive and ergonomic gesture-based interfaces
- Balancing immersion with user comfort and safety
- Addressing motion sickness and eye strain in VR applications
4. Privacy and Security Concerns
- Handling sensitive spatial data about users’ environments
- Ensuring user privacy in shared AR experiences
- Protecting against potential AR/VR-based security threats
5. Standardization and Interoperability
- Lack of universal standards for spatial computing technologies
- Challenges in creating cross-platform experiences
- Integration difficulties with existing systems and workflows
6. Ethical Considerations
- Potential for AR/VR addiction and escapism
- Impact on social interactions and public spaces
- Ensuring accessibility and inclusivity in spatial computing experiences
8. The Future of Spatial Computing
As technology continues to advance, the future of spatial computing looks incredibly promising. Here are some trends and predictions for the field:
1. Improved Hardware
- More powerful and energy-efficient mobile processors
- Advanced depth sensors and cameras for better environmental understanding
- Lightweight and high-resolution AR/VR displays
2. Enhanced AI Integration
- More sophisticated object recognition and scene understanding
- Personalized spatial experiences driven by AI
- Improved natural language processing for voice-controlled spatial interfaces
3. Ubiquitous AR
- AR-enabled smart glasses becoming mainstream
- Persistent AR layers overlaying the physical world
- Integration of AR into everyday objects and environments
4. Advanced Haptics and Sensory Feedback
- More realistic tactile feedback in virtual environments
- Integration of other sensory inputs (e.g., temperature, smell)
- Non-invasive brain-computer interfaces for direct neural feedback
5. Collaborative Spatial Experiences
- Seamless multi-user AR/VR interactions
- Global-scale persistent AR worlds
- Integration of spatial computing in remote work and collaboration tools
6. Spatial Computing in Smart Cities
- AR-enhanced navigation and information systems
- Spatial computing for urban planning and management
- Interactive public art and entertainment experiences
9. Conclusion
Spatial computing, with its integration of spatial mapping and gesture recognition, is poised to revolutionize how we interact with digital information and our physical environment. As we’ve explored in this article, the possibilities are vast, ranging from enhancing everyday tasks to creating entirely new forms of entertainment and productivity tools.
For developers and entrepreneurs, spatial computing presents an exciting frontier full of opportunities for innovation. By mastering the fundamentals of spatial mapping and gesture recognition, and staying abreast of the latest developments in the field, you’ll be well-positioned to create groundbreaking applications that bridge the gap between the physical and digital worlds.
As with any emerging technology, there are challenges to overcome and ethical considerations to address. However, the potential benefits of spatial computing in areas such as education, healthcare, and industry are immense. As the technology continues to mature and become more accessible, we can expect to see spatial computing play an increasingly important role in shaping our digital future.
We encourage you to dive into spatial computing, experiment with the technologies discussed in this article, and contribute to the growing community of spatial computing developers. The future of computing is spatial, and the possibilities are limited only by our imagination and creativity.
Happy coding, and welcome to the exciting world of spatial computing!