Study Performance Optimization Techniques: Boosting Your Code Efficiency
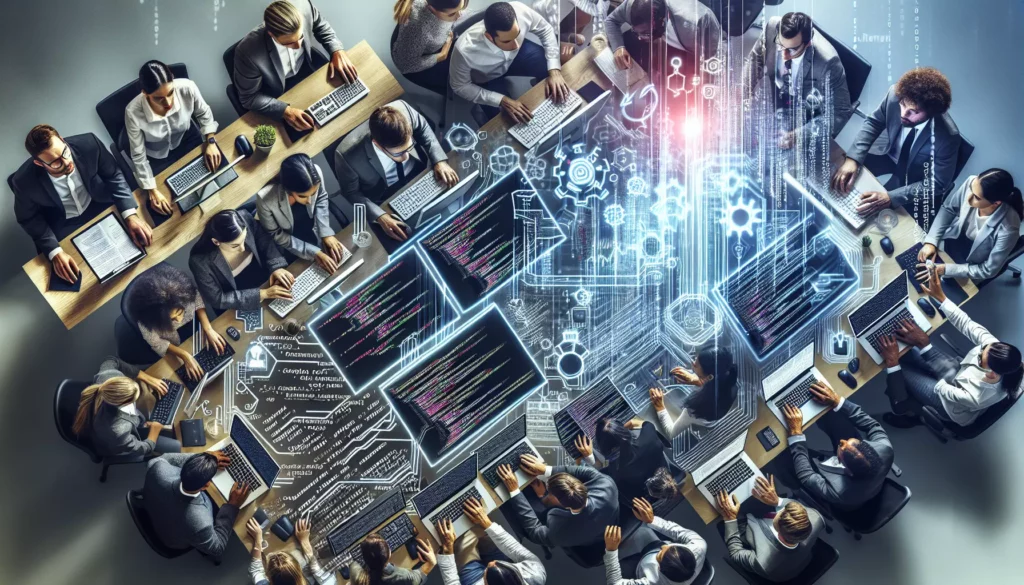
In the world of software development, writing functional code is just the beginning. As applications grow in complexity and scale, the need for efficient and optimized code becomes increasingly critical. This is where performance optimization techniques come into play. In this comprehensive guide, we’ll explore various strategies to improve the efficiency of your code and applications, helping you become a more proficient developer and enhancing your ability to tackle technical interviews, especially for major tech companies.
Why Performance Optimization Matters
Before diving into specific techniques, it’s essential to understand why performance optimization is crucial:
- User Experience: Faster, more responsive applications lead to better user satisfaction.
- Resource Utilization: Optimized code uses fewer system resources, allowing for better scalability.
- Cost Efficiency: Efficient applications can reduce infrastructure costs, especially in cloud environments.
- Competitive Advantage: High-performing applications can set your product apart in the market.
- Interview Success: Understanding optimization techniques is often crucial for technical interviews, particularly at FAANG companies.
1. Algorithmic Optimization
The foundation of performance optimization lies in choosing the right algorithms and data structures for your specific problem. This is where your algorithmic thinking skills come into play.
Time Complexity Analysis
Understanding the time complexity of your algorithms is crucial. Always aim for the most efficient algorithm possible for your use case. Here’s a quick refresher on common time complexities:
- O(1) – Constant time
- O(log n) – Logarithmic time
- O(n) – Linear time
- O(n log n) – Linearithmic time
- O(n^2) – Quadratic time
- O(2^n) – Exponential time
For example, if you’re frequently searching through a large dataset, consider using a hash table (O(1) average case) instead of a linear search (O(n)).
Space-Time Trade-offs
Sometimes, you can trade memory for speed. Techniques like memoization or caching can significantly improve performance by storing results of expensive function calls and returning the cached result when the same inputs occur again.
Here’s a simple example of memoization in Python:
def memoize(f):
cache = {}
def memoized_func(*args):
if args not in cache:
cache[args] = f(*args)
return cache[args]
return memoized_func
@memoize
def fibonacci(n):
if n < 2:
return n
return fibonacci(n-1) + fibonacci(n-2)
2. Code-Level Optimizations
While algorithmic optimizations often yield the most significant improvements, code-level optimizations can also make a substantial difference.
Use Appropriate Data Structures
Choosing the right data structure can have a significant impact on performance. For example:
- Use sets instead of lists when you need to check for membership frequently.
- Use dictionaries when you need key-value pairs with fast lookup times.
- Consider using specialized data structures like heaps for priority queues.
Avoid Unnecessary Work
Look for opportunities to reduce unnecessary computations:
- Use lazy evaluation when possible.
- Avoid recalculating values that don’t change.
- Break out of loops early when the desired condition is met.
Optimize Loops
Loops are often hotspots for performance issues. Here are some tips:
- Move invariant computations outside the loop.
- Use list comprehensions in Python for simple loops.
- Consider using itertools in Python for efficient iteration.
Here’s an example of loop optimization in Python:
# Unoptimized
result = []
for i in range(1000000):
if i % 2 == 0:
result.append(i ** 2)
# Optimized
result = [i ** 2 for i in range(1000000) if i % 2 == 0]
3. Memory Management
Efficient memory management is crucial for performance, especially in languages without automatic garbage collection.
Minimize Object Creation
Creating and destroying objects can be expensive. Consider object pooling for frequently used objects or use immutable objects when possible.
Use Generators for Large Datasets
In Python, generators can help you work with large datasets without loading everything into memory at once. Here’s an example:
def large_dataset_generator(n):
for i in range(n):
yield i ** 2
# Using the generator
for value in large_dataset_generator(1000000):
# Process value
pass
Be Mindful of Closures and Global Variables
In languages like JavaScript, closures can inadvertently keep large objects in memory. Be aware of what your closures are capturing. Similarly, overuse of global variables can lead to memory bloat.
4. Concurrency and Parallelism
Leveraging multiple cores or processors can significantly boost performance for certain types of tasks.
Use Multi-threading or Multi-processing
For CPU-bound tasks, consider using multiple processes. For I/O-bound tasks, multi-threading might be more appropriate. Here’s a simple example using Python’s multiprocessing module:
from multiprocessing import Pool
def process_item(item):
# Some CPU-intensive task
return item * item
if __name__ == '__main__':
with Pool(4) as p:
result = p.map(process_item, range(1000000))
Asynchronous Programming
For I/O-bound applications, asynchronous programming can significantly improve performance by allowing other tasks to run while waiting for I/O operations. Here’s a simple example using Python’s asyncio:
import asyncio
async def fetch_data(url):
# Simulating an API call
await asyncio.sleep(1)
return f"Data from {url}"
async def main():
urls = ["url1", "url2", "url3"]
tasks = [fetch_data(url) for url in urls]
results = await asyncio.gather(*tasks)
print(results)
asyncio.run(main())
5. Database Optimization
For applications that interact with databases, optimizing database queries can lead to significant performance improvements.
Index Your Tables
Proper indexing can dramatically speed up query execution times. However, be cautious not to over-index, as it can slow down write operations.
Use Efficient Queries
Write SQL queries that minimize the amount of data processed. Use JOINs efficiently, avoid SELECT *, and use LIMIT when you don’t need all results.
Caching
Implement caching mechanisms to store frequently accessed data in memory. This can significantly reduce database load and improve response times.
6. Front-end Optimization
For web applications, front-end optimization is crucial for a smooth user experience.
Minimize HTTP Requests
Reduce the number of HTTP requests by combining files, using CSS sprites, and inlining small resources.
Optimize Images
Use appropriate image formats, compress images, and implement lazy loading for images not immediately visible.
Use Content Delivery Networks (CDNs)
CDNs can significantly reduce latency by serving static assets from servers geographically closer to the user.
7. Profiling and Monitoring
To effectively optimize your code, you need to identify where the bottlenecks are. This is where profiling comes in.
Use Profiling Tools
Most programming languages have built-in or third-party profiling tools. For example, Python has cProfile:
import cProfile
def function_to_profile():
# Your code here
pass
cProfile.run('function_to_profile()')
Implement Logging
Strategic logging can help you understand the flow of your application and identify potential bottlenecks.
Use Monitoring Tools
For production applications, use monitoring tools to track performance metrics over time and identify trends or sudden changes.
8. Language-Specific Optimizations
Each programming language has its own set of best practices and optimization techniques. Here are a few examples:
Python
- Use built-in functions and libraries when possible (they’re often implemented in C and are very fast).
- Use list comprehensions instead of map() and filter() for better readability and sometimes better performance.
- Use the collections module for specialized container datatypes.
JavaScript
- Use const and let instead of var for better scoping.
- Avoid using eval() as it’s slow and can be a security risk.
- Use Web Workers for CPU-intensive tasks to avoid blocking the main thread.
Java
- Use StringBuilder for string concatenation in loops.
- Prefer primitive types over wrapper classes when possible.
- Use the appropriate collection type (ArrayList, LinkedList, HashSet, etc.) based on your use case.
9. Caching Strategies
Caching is a powerful technique for improving performance by storing frequently accessed data or computed results for quick retrieval.
In-Memory Caching
Use in-memory caching for frequently accessed data that doesn’t change often. Many languages have built-in or third-party libraries for this. For example, in Python, you can use functools.lru_cache:
from functools import lru_cache
@lru_cache(maxsize=None)
def fibonacci(n):
if n < 2:
return n
return fibonacci(n-1) + fibonacci(n-2)
Distributed Caching
For distributed systems, consider using distributed caching solutions like Redis or Memcached to share cached data across multiple servers.
HTTP Caching
For web applications, implement proper HTTP caching headers to allow browsers and CDNs to cache responses effectively.
10. Code Compilation and Interpretation Optimization
Understanding how your code is compiled or interpreted can lead to performance improvements.
Just-In-Time (JIT) Compilation
Languages like Java and JavaScript use JIT compilation. Understanding how it works can help you write code that’s more likely to be optimized by the JIT compiler.
Ahead-of-Time (AOT) Compilation
For languages that support AOT compilation, like C++ or Rust, understanding compiler optimizations can help you write more efficient code.
Use Appropriate Compiler Flags
When compiling code, use appropriate optimization flags. For example, in C++:
g++ -O3 myprogram.cpp -o myprogram
Conclusion
Performance optimization is a vast and complex field, and mastering it is a journey that never truly ends. As you continue to develop your skills on platforms like AlgoCademy, remember that optimization is not just about making your code faster—it’s about making it more efficient, scalable, and maintainable.
When preparing for technical interviews, especially for major tech companies, having a solid understanding of these optimization techniques can set you apart. Many interview questions, particularly those focusing on algorithmic thinking and problem-solving, often have an implicit expectation of efficient solutions.
Remember, premature optimization is the root of all evil (or so said Donald Knuth). Always start by writing clear, correct code, and then optimize where necessary. Use profiling tools to identify real bottlenecks, and focus your optimization efforts there.
As you practice and learn, try to develop an intuition for performance. Ask yourself: “How will this code behave with larger inputs?” or “What’s the worst-case scenario here?” This kind of thinking will not only make you a better developer but will also prepare you well for the rigorous technical interviews at top tech companies.
Keep coding, keep optimizing, and never stop learning!