Understanding Embedded AI: Implementing AI Algorithms in Embedded Systems
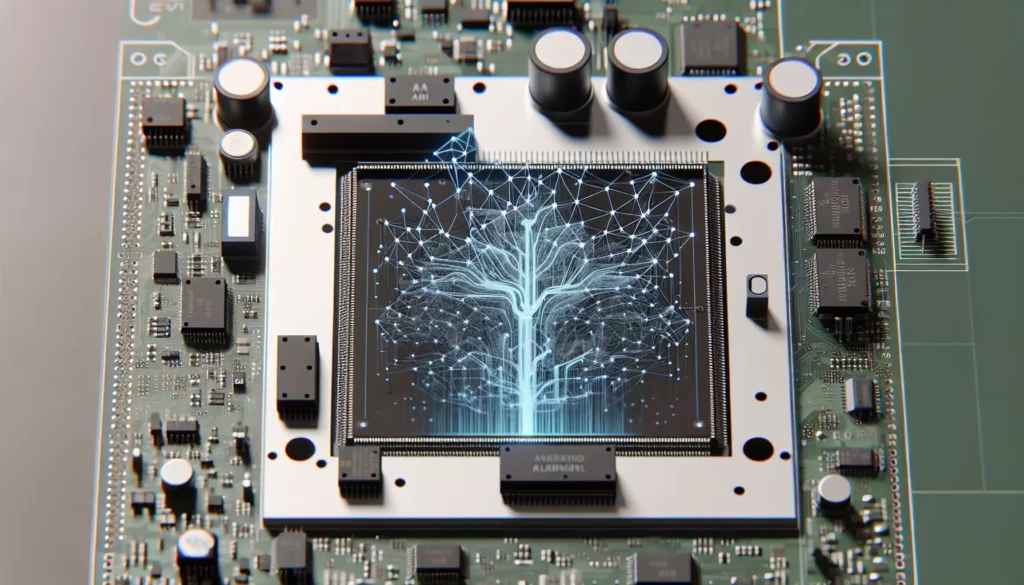
In today’s rapidly evolving technological landscape, the integration of Artificial Intelligence (AI) into embedded systems has become a game-changing trend. This fusion, known as Embedded AI, is revolutionizing various industries by bringing intelligent decision-making capabilities to resource-constrained devices. In this comprehensive guide, we’ll explore the intricacies of implementing AI algorithms in embedded systems, discussing the challenges, benefits, and best practices along the way.
What is Embedded AI?
Embedded AI refers to the implementation of artificial intelligence algorithms and techniques directly on embedded systems. These systems are typically small, specialized computing devices designed to perform specific tasks within larger mechanical or electrical systems. By incorporating AI capabilities, embedded systems can process data, make decisions, and adapt to changing conditions autonomously, without relying on cloud-based or centralized computing resources.
The Importance of Embedded AI
The rise of Embedded AI is driven by several factors:
- Real-time processing: Many applications require immediate decision-making, which cloud-based AI solutions may not provide due to latency issues.
- Privacy and security: Processing data locally reduces the risk of sensitive information being intercepted during transmission to remote servers.
- Reduced bandwidth requirements: By processing data on-device, embedded AI minimizes the need for constant communication with external networks.
- Energy efficiency: Optimized AI algorithms can be more power-efficient when run on specialized hardware, extending battery life for portable devices.
- Offline functionality: Embedded AI allows devices to maintain intelligent capabilities even when network connectivity is unavailable.
Challenges in Implementing AI on Embedded Systems
While the benefits of Embedded AI are substantial, developers face several challenges when implementing AI algorithms on resource-constrained devices:
1. Limited Computational Resources
Embedded systems typically have less processing power, memory, and storage compared to desktop computers or servers. This constraint requires careful optimization of AI algorithms to fit within these limitations.
2. Power Consumption
Many embedded devices operate on battery power, making energy efficiency a critical concern. AI algorithms must be designed to minimize power consumption without sacrificing performance.
3. Real-time Performance
Embedded systems often require real-time responsiveness. AI algorithms must be optimized to provide quick inference times while maintaining accuracy.
4. Memory Constraints
With limited RAM and storage, embedded AI implementations must be memory-efficient, both in terms of model size and runtime memory usage.
5. Diverse Hardware Architectures
Embedded systems come in various hardware configurations, making it challenging to create one-size-fits-all AI solutions. Developers must often tailor their implementations to specific architectures.
Strategies for Implementing AI in Embedded Systems
To overcome these challenges and successfully implement AI algorithms in embedded systems, consider the following strategies:
1. Model Compression and Quantization
Reducing the size and complexity of AI models is crucial for embedded deployment. Techniques like pruning, quantization, and knowledge distillation can significantly decrease model size without substantial loss in accuracy.
Example of quantization in TensorFlow Lite:
import tensorflow as tf
# Convert the model to TensorFlow Lite format
converter = tf.lite.TFLiteConverter.from_keras_model(model)
# Enable quantization
converter.optimizations = [tf.lite.Optimize.DEFAULT]
# Convert the model
tflite_model = converter.convert()
# Save the quantized model
with open('quantized_model.tflite', 'wb') as f:
f.write(tflite_model)
2. Hardware Acceleration
Leveraging specialized hardware accelerators like GPUs, FPGAs, or dedicated AI chips can significantly improve performance and energy efficiency. Many embedded platforms now offer built-in AI acceleration capabilities.
3. Efficient Algorithm Design
Developing or selecting algorithms that are inherently efficient for embedded deployment is crucial. This may involve using lightweight neural network architectures or alternative AI techniques that require fewer resources.
4. Optimized Software Libraries
Utilizing optimized libraries specifically designed for embedded AI, such as TensorFlow Lite, CMSIS-NN, or ARM Compute Library, can greatly improve performance and ease of implementation.
5. Edge-Cloud Hybrid Approaches
In some cases, a hybrid approach that combines on-device processing with cloud-based resources can provide a balance between local responsiveness and more complex AI capabilities.
Popular AI Algorithms for Embedded Systems
When implementing AI in embedded systems, certain algorithms and models are more suitable due to their efficiency and lower resource requirements. Here are some popular choices:
1. Convolutional Neural Networks (CNNs)
CNNs are widely used for image processing tasks and can be optimized for embedded deployment through techniques like depthwise separable convolutions.
Example of a simple CNN in TensorFlow:
import tensorflow as tf
model = tf.keras.Sequential([
tf.keras.layers.Conv2D(32, (3, 3), activation='relu', input_shape=(28, 28, 1)),
tf.keras.layers.MaxPooling2D((2, 2)),
tf.keras.layers.Flatten(),
tf.keras.layers.Dense(10, activation='softmax')
])
model.compile(optimizer='adam', loss='sparse_categorical_crossentropy', metrics=['accuracy'])
2. Recurrent Neural Networks (RNNs) and Long Short-Term Memory (LSTM)
For sequential data processing, such as speech recognition or time series analysis, RNNs and LSTMs can be effective when properly optimized for embedded systems.
3. Decision Trees and Random Forests
These algorithms are computationally efficient and can be easily implemented on embedded systems for tasks like classification and regression.
4. K-Nearest Neighbors (KNN)
KNN is a simple yet effective algorithm for classification tasks that can be implemented with minimal resources.
5. Support Vector Machines (SVM)
SVMs can be efficient for binary classification tasks and can be optimized for embedded deployment.
Best Practices for Embedded AI Implementation
To ensure successful implementation of AI algorithms in embedded systems, consider the following best practices:
1. Profile and Benchmark
Thoroughly profile your AI algorithms on the target hardware to identify performance bottlenecks and optimize accordingly.
2. Optimize Data Flow
Minimize data movement between memory and processing units to reduce power consumption and improve performance.
3. Leverage Fixed-Point Arithmetic
Where possible, use fixed-point arithmetic instead of floating-point to improve performance on embedded processors without dedicated FPUs.
4. Implement Efficient Memory Management
Use memory-efficient data structures and algorithms, and consider techniques like memory pooling to reduce fragmentation.
5. Parallelize Computations
Utilize multi-core processors or hardware accelerators to parallelize AI computations when available.
6. Continuous Monitoring and Updating
Implement mechanisms for monitoring the performance and accuracy of embedded AI systems in the field, and provide paths for updates and improvements.
Real-World Applications of Embedded AI
Embedded AI is finding applications across various industries and domains:
1. Automotive
Advanced driver assistance systems (ADAS) and autonomous vehicles rely heavily on embedded AI for tasks like object detection, lane keeping, and decision making.
2. Consumer Electronics
Smart home devices, wearables, and smartphones use embedded AI for voice recognition, image processing, and personalized user experiences.
3. Industrial IoT
Predictive maintenance, quality control, and process optimization in manufacturing benefit from on-device AI processing.
4. Healthcare
Medical devices and wearables use embedded AI for real-time health monitoring, diagnostics, and personalized treatment recommendations.
5. Robotics
Embedded AI enables robots to perceive their environment, make decisions, and interact more naturally with humans.
Future Trends in Embedded AI
As technology continues to evolve, several trends are shaping the future of Embedded AI:
1. Neuromorphic Computing
Bio-inspired computing architectures that mimic the human brain’s neural structure promise to deliver more efficient AI processing for embedded systems.
2. Federated Learning
This approach allows embedded devices to collaboratively learn a shared model while keeping data locally, addressing privacy concerns and reducing bandwidth requirements.
3. Tiny Machine Learning (TinyML)
The development of ultra-low power AI algorithms and hardware is pushing the boundaries of what’s possible on extremely resource-constrained devices.
4. AI-Specific Hardware
The proliferation of specialized AI accelerators and neural processing units (NPUs) will enable more powerful and efficient embedded AI applications.
Conclusion
Implementing AI algorithms in embedded systems presents unique challenges, but the benefits of Embedded AI are transformative across industries. By understanding the constraints, applying appropriate optimization techniques, and following best practices, developers can create powerful, efficient, and intelligent embedded systems.
As we continue to push the boundaries of what’s possible with Embedded AI, we can expect to see even more innovative applications that bring the power of artificial intelligence to the edge, revolutionizing how we interact with and benefit from technology in our daily lives.
The journey of mastering Embedded AI is an exciting one, filled with opportunities for innovation and problem-solving. As you embark on this path, remember that the key to success lies in balancing the capabilities of AI with the constraints of embedded systems, always striving for optimal performance, efficiency, and reliability.