Understanding the Principles of Modularity in Software Development
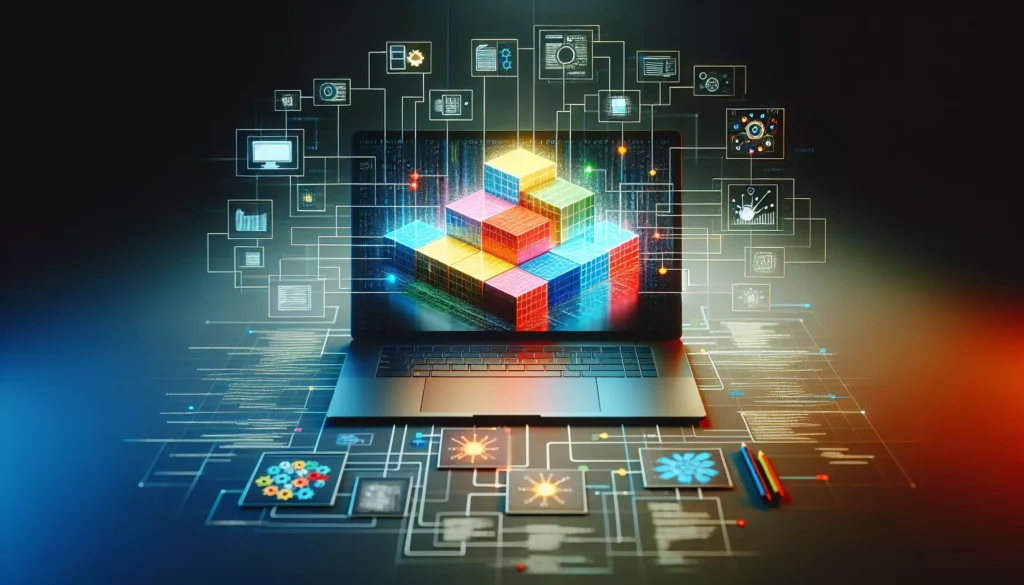
In the ever-evolving landscape of software development, one principle stands out as a cornerstone of efficient and maintainable code: modularity. As we delve into the world of coding education and programming skills development, it’s crucial to understand how modularity shapes the way we approach software design and implementation. This article will explore the principles of modularity, its importance in modern software development, and how it aligns with the goals of platforms like AlgoCademy in preparing aspiring developers for success in the tech industry.
What is Modularity in Software Development?
Modularity is a software design technique that emphasizes separating a program’s functionality into independent, interchangeable modules. Each module contains everything necessary to execute only one aspect of the desired functionality. In essence, modularity is about breaking down complex systems into smaller, manageable parts that can work together seamlessly.
The concept of modularity isn’t new; it has its roots in hardware design and has been a fundamental principle in software engineering for decades. However, its importance has only grown with the increasing complexity of modern software systems.
Key Principles of Modularity
To truly understand and implement modularity in software development, it’s essential to grasp its core principles:
1. Separation of Concerns
This principle suggests that software should be separated into distinct sections, each addressing a specific feature or functionality. By doing so, developers can focus on one area of functionality at a time without affecting others.
2. Encapsulation
Encapsulation involves bundling the data and the methods that operate on that data within a single unit or object. This principle helps in hiding the internal state of an object and requiring all interaction to be performed through an object’s methods.
3. Information Hiding
Closely related to encapsulation, information hiding involves concealing the implementation details of a module from other parts of the program. This principle promotes a “need-to-know” basis, where modules only expose what is necessary for others to use them effectively.
4. Interface-Based Design
Modules should communicate with each other through well-defined interfaces. This principle ensures that changes to one module don’t ripple through the entire system, as long as the interface remains consistent.
5. Loose Coupling
Modules should have minimal dependencies on each other. Loose coupling allows for easier maintenance, testing, and reusability of individual modules.
6. High Cohesion
Within a module, the elements should be closely related and focused on a single task or responsibility. High cohesion contributes to more robust and maintainable code.
The Importance of Modularity in Modern Software Development
Understanding and implementing modularity is crucial for several reasons:
Maintainability
Modular code is easier to maintain and update. When changes are required, developers can focus on specific modules without worrying about unintended consequences in other parts of the system.
Reusability
Well-designed modules can be reused in different projects or parts of the same project, saving development time and reducing code duplication.
Scalability
As software systems grow, modularity allows for easier scaling. New features can be added by introducing new modules or extending existing ones without overhauling the entire system.
Testability
Modular code is inherently more testable. Individual modules can be tested in isolation, making it easier to identify and fix bugs.
Collaboration
In team environments, modularity allows different developers or teams to work on separate modules simultaneously, improving productivity and reducing conflicts.
Implementing Modularity in Practice
Now that we understand the principles and importance of modularity, let’s look at how to implement it in practice:
1. Design with Modularity in Mind
Before writing any code, plan your software architecture with modularity as a guiding principle. Break down the system into logical components and define clear interfaces between them.
2. Use Object-Oriented Programming (OOP) Concepts
OOP languages like Java, C++, or Python provide built-in support for creating modular code through classes and objects. Utilize concepts like inheritance, polymorphism, and abstraction to create flexible and modular designs.
3. Implement Design Patterns
Many design patterns, such as the Factory Method, Observer, or Strategy patterns, inherently promote modularity. Familiarize yourself with these patterns and use them where appropriate.
4. Leverage Dependency Injection
Dependency Injection is a technique that helps achieve loose coupling between modules. Instead of hard-coding dependencies, modules receive their dependencies through constructors, methods, or setters.
5. Use Microservices Architecture
For large-scale applications, consider a microservices architecture. This approach takes modularity to the system level, where each service is a separate, deployable module.
6. Practice Clean Code Principles
Follow clean code practices such as writing small, focused functions and classes. This naturally leads to more modular code.
Modularity in Different Programming Paradigms
While we often associate modularity with object-oriented programming, it’s a principle that can be applied across different programming paradigms:
Procedural Programming
In procedural languages like C, modularity can be achieved through the use of functions and structs. Each function should perform a single, well-defined task, and related functions can be grouped into modules or libraries.
Functional Programming
Functional programming languages like Haskell or Scala emphasize modularity through pure functions and immutable data. Higher-order functions and function composition are powerful tools for creating modular designs in functional programming.
Object-Oriented Programming
As mentioned earlier, OOP provides robust support for modularity through classes, interfaces, and inheritance. Here’s a simple example in Python:
class Animal:
def __init__(self, name):
self.name = name
def speak(self):
pass
class Dog(Animal):
def speak(self):
return f"{self.name} says Woof!"
class Cat(Animal):
def speak(self):
return f"{self.name} says Meow!"
# Usage
dog = Dog("Buddy")
cat = Cat("Whiskers")
print(dog.speak()) # Output: Buddy says Woof!
print(cat.speak()) # Output: Whiskers says Meow!
In this example, we have a modular design where the Animal
class defines a common interface, and specific animal types (Dog
and Cat
) implement this interface in their own way.
Challenges in Achieving Modularity
While the benefits of modularity are clear, implementing it effectively can be challenging:
1. Over-modularization
Breaking down a system into too many small modules can lead to increased complexity and reduced readability. It’s important to find the right balance.
2. Performance Overhead
In some cases, highly modular systems may introduce performance overhead due to increased function calls or network communication in distributed systems.
3. Design Complexity
Designing a truly modular system requires careful planning and a deep understanding of the problem domain. It can be more time-consuming upfront compared to a monolithic approach.
4. Maintaining Consistency
As a system evolves, maintaining consistency across modules and their interfaces can become challenging, especially in large teams.
Tools and Frameworks Supporting Modularity
Modern development ecosystems provide various tools and frameworks that support and encourage modular development:
1. Package Managers
Tools like npm for JavaScript, pip for Python, or Maven for Java help manage dependencies and promote the use of modular, reusable packages.
2. Module Bundlers
For web development, tools like Webpack or Rollup allow developers to write modular JavaScript code and bundle it efficiently for production.
3. Dependency Injection Frameworks
Frameworks like Spring for Java or Angular’s dependency injection system help manage dependencies between modules in a loosely coupled manner.
4. Microservices Frameworks
Frameworks like Spring Boot, Express.js, or Django REST framework provide support for building modular, microservices-based architectures.
Modularity and AlgoCademy: Preparing for Tech Interviews
Understanding and applying modularity principles is crucial for aspiring developers preparing for technical interviews, especially for positions at major tech companies. Here’s how modularity ties into the goals of platforms like AlgoCademy:
1. Problem Decomposition
The ability to break down complex problems into smaller, manageable modules is a key skill in algorithmic thinking and problem-solving – core focus areas for technical interviews.
2. Code Organization
Interviewers often assess a candidate’s ability to write clean, organized code. Modular code demonstrates good software engineering practices and can set you apart in coding assessments.
3. System Design Questions
Many technical interviews, especially for senior positions, include system design questions. Understanding modularity is crucial for designing scalable and maintainable software architectures.
4. Reusable Components
Creating modular, reusable components is a valuable skill in real-world software development. Demonstrating this ability can impress interviewers and showcase your practical coding skills.
5. Adaptability
Modular thinking allows developers to adapt to different programming paradigms and languages more easily – a valuable trait in the ever-changing tech landscape.
Practical Exercises to Improve Modular Thinking
To help aspiring developers improve their modular thinking skills, here are some exercises that could be incorporated into a learning platform like AlgoCademy:
1. Refactoring Challenge
Provide students with a monolithic piece of code and challenge them to refactor it into a more modular structure. This exercise helps in identifying separate concerns and creating appropriate modules.
2. Module Interface Design
Given a problem description, ask students to design the interfaces for different modules without implementing the full functionality. This focuses on the important skill of defining clear boundaries between components.
3. Microservices Architecture
Present a complex system and ask students to break it down into microservices. This exercise scales up modular thinking to a system architecture level.
4. Dependency Injection Implementation
Provide a set of tightly coupled classes and challenge students to redesign them using dependency injection principles. This reinforces the concept of loose coupling.
5. Design Pattern Application
Give students scenarios where they need to apply appropriate design patterns to create modular solutions. This helps in understanding how established patterns can contribute to modular design.
Conclusion
Modularity is not just a programming technique; it’s a fundamental approach to managing complexity in software development. By embracing modularity, developers can create more maintainable, scalable, and robust software systems. For aspiring developers using platforms like AlgoCademy to prepare for technical interviews and build their coding skills, understanding and applying modular principles is invaluable.
As you continue your journey in software development, always strive to think in terms of modules – whether you’re solving algorithmic problems, designing systems, or writing day-to-day code. Remember that modularity is not about following a strict set of rules, but about adopting a mindset that promotes clarity, reusability, and maintainability in your code.
By mastering the principles of modularity, you’ll not only become a more effective developer but also be better prepared to tackle the challenges of modern software development in your future career. Keep practicing, stay curious, and always look for ways to apply modular thinking in your coding projects. The skills you develop in creating modular, well-organized code will serve you well in technical interviews and throughout your professional journey in the world of software development.