Software-defined Networking (SDN): Revolutionizing Network Management and Control
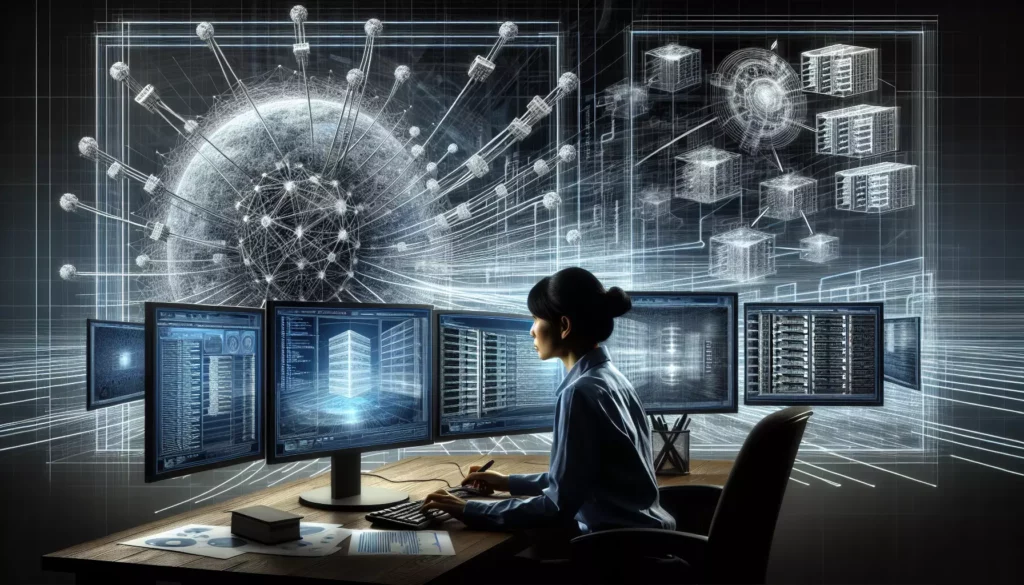
In today’s rapidly evolving technological landscape, the way we manage and control networks is undergoing a significant transformation. At the forefront of this revolution is Software-defined Networking (SDN), a paradigm shift that promises to bring unprecedented flexibility, efficiency, and programmability to network infrastructure. In this comprehensive guide, we’ll delve deep into the world of SDN, exploring its concepts, benefits, and real-world applications. Whether you’re a seasoned network engineer or a coding enthusiast looking to expand your horizons, this article will provide valuable insights into the future of networking.
What is Software-defined Networking (SDN)?
Software-defined Networking is an approach to network management that enables dynamic, programmatically efficient network configuration to improve network performance and monitoring. SDN decouples the network control and forwarding functions, allowing for more direct programmability of network control and abstracting the underlying infrastructure for applications and network services.
At its core, SDN separates the control plane (which decides how to handle network traffic) from the data plane (which forwards traffic based on decisions made by the control plane). This separation allows for centralized control of the network, making it more flexible and easier to manage.
Key Components of SDN
- SDN Controller: The brain of the network, responsible for making decisions about traffic flow.
- Southbound APIs: Interfaces that allow the controller to communicate with network devices.
- Northbound APIs: Interfaces that allow applications to communicate with the controller.
- Network Devices: Switches, routers, and other hardware that forward traffic based on instructions from the controller.
The Benefits of Software-defined Networking
SDN offers numerous advantages over traditional networking approaches:
- Centralized Network Provisioning: Simplifies network management by providing a centralized view of the entire network.
- Increased Network Flexibility: Allows for rapid changes to network configuration without manual intervention.
- Improved Network Performance: Enables more efficient traffic management and load balancing.
- Enhanced Security: Facilitates the implementation of network-wide security policies and rapid response to threats.
- Cost Reduction: Reduces hardware costs by leveraging commodity hardware and software-based solutions.
- Innovation Acceleration: Enables faster deployment of new applications and services.
SDN Architecture: A Closer Look
To fully appreciate the power of SDN, it’s essential to understand its architecture. The SDN architecture consists of three main layers:
1. Application Layer
This layer contains the network applications and services that define network behavior. These applications communicate their network requirements to the SDN Controller through Northbound APIs.
2. Control Layer
At the heart of SDN architecture is the Control Layer, dominated by the SDN Controller. This layer is responsible for:
- Translating application requirements into network instructions
- Providing an abstract view of the network to applications
- Making decisions on how traffic should flow through the network
3. Infrastructure Layer
This layer consists of the physical network devices (switches, routers, etc.) that forward network traffic based on instructions received from the Control Layer through Southbound APIs.
SDN Protocols and Standards
Several protocols and standards have emerged to support SDN implementation:
OpenFlow
OpenFlow is one of the most widely used SDN protocols. It defines the communication interface between the control and forwarding layers of an SDN architecture. OpenFlow allows direct access to and manipulation of the forwarding plane of network devices such as switches and routers, both physical and virtual (hypervisor-based).
NETCONF and YANG
NETCONF (Network Configuration Protocol) is a protocol defined by the IETF to install, manipulate, and delete the configuration of network devices. YANG (Yet Another Next Generation) is a data modeling language used to model configuration and state data manipulated by NETCONF.
P4 (Programming Protocol-independent Packet Processors)
P4 is a domain-specific language for network devices, allowing programmers to specify how a switch should process packets without being tied to specific hardware.
Implementing SDN: A Practical Approach
Implementing SDN in your network involves several steps:
- Assessment: Evaluate your current network infrastructure and identify areas where SDN can bring improvements.
- Planning: Design your SDN architecture, choosing appropriate controllers, protocols, and network devices.
- Controller Setup: Install and configure your SDN controller. Popular open-source options include OpenDaylight and ONOS.
- Network Device Configuration: Configure your network devices to support SDN protocols like OpenFlow.
- Application Development: Develop or deploy applications that leverage the SDN infrastructure to meet your network requirements.
- Testing and Optimization: Thoroughly test your SDN implementation and optimize its performance.
SDN in Action: Real-world Use Cases
SDN has found applications across various industries and use cases:
1. Cloud Computing
SDN enables cloud providers to offer more flexible and efficient network services to their customers. It allows for rapid provisioning of network resources and improved multi-tenancy support.
2. Network Function Virtualization (NFV)
SDN complements NFV by providing the flexible network infrastructure needed to support virtualized network functions.
3. Data Centers
SDN simplifies data center network management, improves resource utilization, and enables more efficient traffic management.
4. Wide Area Networks (WANs)
SDN-enabled WANs (SD-WANs) offer improved performance, security, and cost-efficiency for enterprise networks spanning multiple locations.
5. Internet of Things (IoT)
SDN can help manage the complex network requirements of IoT deployments, providing the scalability and flexibility needed to support a large number of connected devices.
Coding for SDN: Getting Started
For programmers looking to dive into SDN, there are several areas to focus on:
1. Python for Network Programming
Python is widely used in SDN due to its simplicity and powerful libraries. Here’s a simple example of using Python to interact with an OpenFlow switch:
from ryu.base import app_manager
from ryu.controller import ofp_event
from ryu.controller.handler import CONFIG_DISPATCHER, MAIN_DISPATCHER
from ryu.controller.handler import set_ev_cls
from ryu.ofproto import ofproto_v1_3
class SimpleSwitch13(app_manager.RyuApp):
OFP_VERSIONS = [ofproto_v1_3.OFP_VERSION]
def __init__(self, *args, **kwargs):
super(SimpleSwitch13, self).__init__(*args, **kwargs)
self.mac_to_port = {}
@set_ev_cls(ofp_event.EventOFPSwitchFeatures, CONFIG_DISPATCHER)
def switch_features_handler(self, ev):
datapath = ev.msg.datapath
ofproto = datapath.ofproto
parser = datapath.ofproto_parser
# Install table-miss flow entry
match = parser.OFPMatch()
actions = [parser.OFPActionOutput(ofproto.OFPP_CONTROLLER,
ofproto.OFPCML_NO_BUFFER)]
self.add_flow(datapath, 0, match, actions)
def add_flow(self, datapath, priority, match, actions, buffer_id=None):
ofproto = datapath.ofproto
parser = datapath.ofproto_parser
inst = [parser.OFPInstructionActions(ofproto.OFPIT_APPLY_ACTIONS,
actions)]
if buffer_id:
mod = parser.OFPFlowMod(datapath=datapath, buffer_id=buffer_id,
priority=priority, match=match,
instructions=inst)
else:
mod = parser.OFPFlowMod(datapath=datapath, priority=priority,
match=match, instructions=inst)
datapath.send_msg(mod)
2. RESTful APIs
Many SDN controllers expose RESTful APIs for configuration and management. Understanding how to interact with these APIs is crucial. Here’s an example of using Python requests library to interact with an SDN controller’s REST API:
import requests
import json
# Define the base URL for the SDN controller's API
base_url = 'http://controller-ip:port/api'
# Function to get all flows from a switch
def get_flows(switch_id):
url = f'{base_url}/switches/{switch_id}/flows'
response = requests.get(url)
if response.status_code == 200:
return json.loads(response.text)
else:
return None
# Function to add a new flow to a switch
def add_flow(switch_id, flow_data):
url = f'{base_url}/switches/{switch_id}/flows'
headers = {'Content-Type': 'application/json'}
response = requests.post(url, headers=headers, data=json.dumps(flow_data))
return response.status_code == 201
# Example usage
switch_id = '00:00:00:00:00:00:00:01'
flows = get_flows(switch_id)
print(f'Current flows on switch {switch_id}:')
print(json.dumps(flows, indent=2))
new_flow = {
'priority': 100,
'match': {'in_port': 1},
'actions': [{'type': 'OUTPUT', 'port': 2}]
}
if add_flow(switch_id, new_flow):
print('New flow added successfully')
else:
print('Failed to add new flow')
3. Network Simulation
Tools like Mininet allow you to create virtual networks for SDN experimentation. Here’s a simple Mininet script to create a basic SDN topology:
from mininet.net import Mininet
from mininet.node import Controller, OVSKernelSwitch
from mininet.cli import CLI
from mininet.log import setLogLevel, info
def createNetwork():
net = Mininet(controller=Controller, switch=OVSKernelSwitch)
info('*** Adding controller\n')
net.addController('c0')
info('*** Adding switches\n')
s1 = net.addSwitch('s1')
s2 = net.addSwitch('s2')
info('*** Adding hosts\n')
h1 = net.addHost('h1', ip='10.0.0.1')
h2 = net.addHost('h2', ip='10.0.0.2')
h3 = net.addHost('h3', ip='10.0.0.3')
h4 = net.addHost('h4', ip='10.0.0.4')
info('*** Creating links\n')
net.addLink(h1, s1)
net.addLink(h2, s1)
net.addLink(h3, s2)
net.addLink(h4, s2)
net.addLink(s1, s2)
info('*** Starting network\n')
net.start()
info('*** Running CLI\n')
CLI(net)
info('*** Stopping network\n')
net.stop()
if __name__ == '__main__':
setLogLevel('info')
createNetwork()
Challenges and Future of SDN
While SDN offers numerous benefits, it also faces several challenges:
- Security Concerns: Centralization of network control can create a single point of failure if not properly secured.
- Scalability: As networks grow, ensuring the scalability of SDN controllers becomes crucial.
- Interoperability: Ensuring compatibility between different vendors’ SDN solutions can be challenging.
- Skill Gap: The shift to SDN requires network professionals to develop new skills, particularly in programming and automation.
Despite these challenges, the future of SDN looks promising. As 5G networks roll out and edge computing becomes more prevalent, SDN is expected to play a crucial role in managing these complex, distributed networks. Emerging technologies like Intent-Based Networking (IBN) and AI-driven network management are building upon SDN principles to create even more intelligent and autonomous networks.
Conclusion
Software-defined Networking represents a paradigm shift in how we approach network management and control. By separating the control plane from the data plane and introducing programmability into network infrastructure, SDN offers unprecedented flexibility, efficiency, and innovation potential. As we move towards more complex and distributed network architectures, the principles of SDN will become increasingly important.
For coding enthusiasts and aspiring network professionals, understanding SDN opens up a world of opportunities. The convergence of networking and programming skills is creating new roles and responsibilities in the IT industry. By mastering SDN concepts and related programming techniques, you’ll be well-positioned to tackle the networking challenges of the future.
As you continue your journey in coding education and skills development, consider exploring SDN as a way to broaden your expertise and stay at the forefront of networking technology. Whether you’re preparing for technical interviews at major tech companies or looking to innovate in the field of network management, SDN knowledge will be a valuable asset in your toolkit.