Vehicle-to-Everything (V2X) Communication: Revolutionizing Connected Vehicles
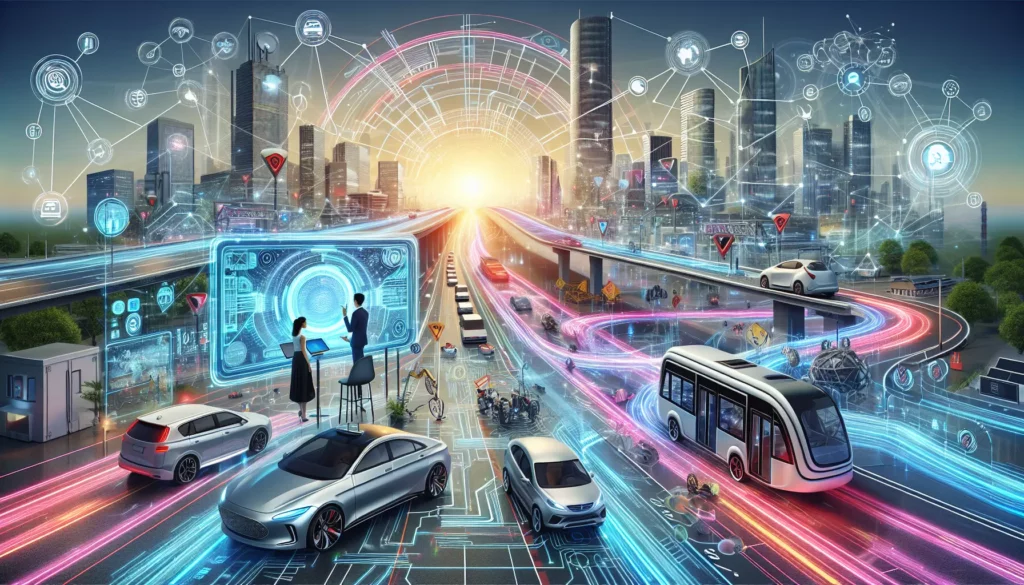
In the rapidly evolving landscape of automotive technology, Vehicle-to-Everything (V2X) communication stands out as a groundbreaking innovation that promises to revolutionize the way vehicles interact with their surroundings. This comprehensive guide will delve into the intricacies of V2X communication, exploring its potential impact on road safety, traffic efficiency, and the future of transportation. As we navigate through this topic, we’ll draw parallels to the world of coding education and programming skills development, demonstrating how the principles of algorithmic thinking and problem-solving are crucial in both domains.
Understanding Vehicle-to-Everything (V2X) Communication
V2X communication refers to the passing of information from a vehicle to any entity that may affect the vehicle, and vice versa. This technology enables vehicles to communicate with other vehicles (V2V), infrastructure (V2I), pedestrians (V2P), networks (V2N), and even the grid (V2G). The primary goal of V2X is to improve road safety, enhance traffic efficiency, and reduce environmental impact.
Much like how coding education platforms such as AlgoCademy focus on building a strong foundation in programming concepts, V2X communication systems are built on a robust framework of protocols and standards. These systems require a deep understanding of networking principles, data processing, and real-time communication – all of which have parallels in the world of software development.
Key Components of V2X Communication
- On-Board Units (OBUs): These are the devices installed in vehicles that enable V2X communication.
- Roadside Units (RSUs): Infrastructure components that facilitate communication between vehicles and the surrounding environment.
- Communication Protocols: Standards such as DSRC (Dedicated Short-Range Communications) and C-V2X (Cellular V2X) that define how data is transmitted.
- Sensors and Actuators: Devices that collect and respond to data from the vehicle and its environment.
- Data Processing Units: On-board computers that analyze incoming data and make decisions.
These components work together seamlessly, much like how different modules and libraries collaborate in a well-structured software application. The integration of these elements requires a systems-thinking approach, similar to how programmers must consider the overall architecture of their code.
The Importance of V2X in Modern Transportation
As we progress towards a future of autonomous and connected vehicles, V2X communication becomes increasingly crucial. Here are some key reasons why V2X is gaining prominence:
1. Enhanced Road Safety
V2X technology has the potential to significantly reduce road accidents by providing real-time information about potential hazards. For instance, a vehicle can receive warnings about an approaching emergency vehicle, a sudden brake application by a vehicle ahead, or a pedestrian crossing the road outside the driver’s line of sight.
This concept of preemptive warning systems in V2X is analogous to error handling and exception management in programming. Just as developers write code to anticipate and handle potential errors, V2X systems are designed to foresee and mitigate potential dangers on the road.
2. Improved Traffic Management
By enabling communication between vehicles and traffic infrastructure, V2X can help optimize traffic flow. Smart traffic lights can adjust their timing based on real-time traffic conditions, and vehicles can be rerouted to avoid congestion.
This optimization process is reminiscent of algorithm efficiency in computer science. Just as programmers strive to write efficient code that minimizes resource usage, V2X systems aim to maximize the efficiency of road networks and reduce travel times.
3. Environmental Benefits
V2X can contribute to reduced emissions by enabling more efficient driving patterns. For example, vehicles can adjust their speed to hit green lights consistently, reducing unnecessary acceleration and deceleration.
This eco-friendly approach in V2X mirrors the concept of code optimization in software development. Both aim to achieve the desired outcome with minimal waste of resources, whether it’s fuel consumption or CPU cycles.
4. Enabler for Autonomous Vehicles
V2X communication is a crucial component in the development of fully autonomous vehicles. It provides an additional layer of information beyond what on-board sensors can capture, enhancing the vehicle’s awareness of its surroundings.
The role of V2X in autonomous driving is comparable to the importance of external libraries and APIs in software development. Just as developers leverage existing tools and resources to enhance their applications, autonomous vehicles rely on V2X to augment their decision-making capabilities.
Technical Deep Dive: V2X Communication Protocols
At the heart of V2X communication are the protocols that govern how data is transmitted between entities. The two primary competing standards in this space are DSRC and C-V2X. Let’s explore these in detail:
Dedicated Short-Range Communications (DSRC)
DSRC is a Wi-Fi-based technology specifically designed for automotive use. It operates in the 5.9 GHz band and is based on the IEEE 802.11p standard.
Key Features of DSRC:
- Low latency (less than 50ms)
- High reliability in high-mobility scenarios
- Direct communication without network infrastructure
- Limited range (about 300 meters)
The implementation of DSRC in V2X systems can be likened to working with low-level programming languages. It offers fine-grained control and efficiency but requires careful handling and optimization.
Cellular V2X (C-V2X)
C-V2X is a more recent technology that leverages cellular networks for vehicle communication. It’s based on 4G LTE and 5G standards and is seen as a more future-proof solution.
Key Features of C-V2X:
- Longer range compared to DSRC
- Ability to leverage existing cellular infrastructure
- Support for both direct (PC5) and network-based (Uu) communication
- Better non-line-of-sight performance
C-V2X can be compared to high-level programming languages that offer more abstraction and built-in features. It provides a wider range of capabilities out-of-the-box but may have more overhead in certain scenarios.
DSRC vs C-V2X: A Coding Analogy
The debate between DSRC and C-V2X in the V2X community is reminiscent of discussions in the programming world about choosing the right language or framework for a project. Just as some developers prefer the control and efficiency of C++ for certain applications, while others opt for the versatility and ease of use of Python, the choice between DSRC and C-V2X often depends on specific use cases and future scalability requirements.
Implementing V2X Communication Systems
Developing V2X communication systems is a complex task that requires expertise in various domains, including wireless communication, embedded systems, and software engineering. Let’s break down the process and draw parallels to software development practices:
1. System Architecture Design
The first step in implementing a V2X system is designing its overall architecture. This involves defining the components, their interactions, and the data flow between them.
In software development, this is akin to the initial planning phase where developers define the structure of their application, decide on the design patterns to use, and plan the interactions between different modules.
2. Protocol Implementation
Once the architecture is defined, the next step is to implement the chosen communication protocol (DSRC or C-V2X). This involves writing low-level code to handle data transmission and reception according to the protocol specifications.
This stage is similar to implementing network protocols in software development. It requires a deep understanding of the protocol specifications and careful attention to detail to ensure reliable communication.
3. Data Processing and Decision Making
V2X systems need to process incoming data in real-time and make decisions based on this information. This involves developing algorithms for tasks such as threat assessment, path planning, and traffic optimization.
This aspect of V2X development strongly aligns with the core focus of coding education platforms like AlgoCademy. It requires strong algorithmic thinking and problem-solving skills, much like those needed for tackling complex coding challenges or preparing for technical interviews.
4. Security Implementation
Security is paramount in V2X systems to prevent malicious attacks that could compromise road safety. This involves implementing encryption, authentication mechanisms, and secure key management.
In the world of software development, this is analogous to implementing cybersecurity measures in web applications or securing communication channels in distributed systems.
5. Testing and Validation
Rigorous testing is crucial in V2X systems to ensure reliability and safety. This includes unit testing of individual components, integration testing of the entire system, and field testing in real-world scenarios.
This mirrors the testing practices in software development, where unit tests, integration tests, and user acceptance tests are standard parts of the development process.
Code Example: Basic V2X Message Processing
To illustrate how V2X message processing might look in code, here’s a simplified Python example:
import time
class V2XSystem:
def __init__(self):
self.vehicle_id = "CAR001"
self.position = (0, 0) # (x, y) coordinates
self.speed = 0
def send_basic_safety_message(self):
message = {
"type": "BSM",
"vehicle_id": self.vehicle_id,
"position": self.position,
"speed": self.speed,
"timestamp": time.time()
}
return message
def receive_message(self, message):
if message["type"] == "BSM":
self.process_basic_safety_message(message)
# Add more message types as needed
def process_basic_safety_message(self, message):
sender_id = message["vehicle_id"]
sender_position = message["position"]
sender_speed = message["speed"]
# Calculate distance to sender
distance = self.calculate_distance(self.position, sender_position)
# Check for potential collision
if distance < 50 and sender_speed > self.speed:
self.trigger_collision_warning()
def calculate_distance(self, pos1, pos2):
# Simple Euclidean distance calculation
return ((pos1[0] - pos2[0])**2 + (pos1[1] - pos2[1])**2)**0.5
def trigger_collision_warning(self):
print("WARNING: Potential collision detected!")
# Usage
v2x_system = V2XSystem()
message = v2x_system.send_basic_safety_message()
v2x_system.receive_message(message)
This example demonstrates basic concepts of V2X communication, including sending and receiving messages, processing incoming data, and making decisions based on that data. It showcases how algorithmic thinking and data processing, key skills in software development, are applied in V2X systems.
Challenges in V2X Communication
Despite its potential, V2X communication faces several challenges that need to be addressed for widespread adoption:
1. Standardization
The lack of a single, globally accepted standard for V2X communication poses challenges for interoperability. This is similar to the fragmentation seen in programming languages and frameworks, where different standards can lead to compatibility issues.
2. Security and Privacy
Ensuring the security of V2X systems and protecting user privacy are critical challenges. This parallels the ongoing concerns in software development about data protection and secure coding practices.
3. Scalability
As the number of connected vehicles increases, V2X systems need to be able to handle massive amounts of data in real-time. This challenge is akin to scaling large software systems to handle increasing user loads.
4. Integration with Legacy Systems
Integrating V2X technology with existing vehicle systems and infrastructure presents significant challenges. This is reminiscent of the difficulties in maintaining and updating legacy code in software development.
The Future of V2X Communication
As we look to the future, V2X communication is poised to play a crucial role in shaping the landscape of transportation. Here are some exciting prospects:
1. Smart Cities
V2X will be a key enabler for smart cities, allowing for more efficient traffic management, reduced congestion, and improved urban planning. This holistic approach to city management mirrors the trend in software development towards creating comprehensive, interconnected systems.
2. Platooning
V2X could enable truck platooning, where a group of trucks closely follows each other, reducing air resistance and fuel consumption. This concept of optimization through cooperation has parallels in distributed computing and parallel processing in software development.
3. Enhanced Autonomous Driving
As V2X technology matures, it will provide critical support for fully autonomous vehicles, enhancing their ability to navigate complex traffic scenarios. This progression towards more advanced autonomous systems is similar to the evolution of AI and machine learning in software development.
4. Predictive Maintenance
V2X could enable vehicles to communicate their maintenance needs to service centers proactively. This predictive approach is analogous to the use of logging and monitoring in software systems to preempt and address issues before they become critical.
Conclusion: The Intersection of V2X and Coding Education
As we’ve explored the world of Vehicle-to-Everything (V2X) communication, it’s clear that this technology shares many parallels with the field of software development and coding education. Both domains require a strong foundation in problem-solving, algorithmic thinking, and systems design – skills that are at the core of platforms like AlgoCademy.
The development of V2X systems demands expertise in various areas of computer science, from low-level protocol implementation to high-level data processing and decision-making algorithms. As such, the skills cultivated through coding education and practice are directly applicable to this cutting-edge field.
Moreover, the challenges faced in V2X development – such as ensuring security, achieving scalability, and integrating with existing systems – mirror many of the challenges that software developers grapple with daily. By honing their coding skills and tackling complex algorithmic problems, aspiring developers are not only preparing themselves for traditional software roles but also positioning themselves to contribute to innovative fields like V2X communication.
As we move towards a future of smart, connected transportation, the demand for skilled professionals who can bridge the gap between software development and automotive technology will only grow. By focusing on building strong coding fundamentals and problem-solving skills, learners can prepare themselves for exciting opportunities in this evolving landscape.
The journey from writing your first “Hello, World!” program to developing sophisticated V2X communication systems may seem long, but with dedication, continuous learning, and platforms like AlgoCademy to guide the way, it’s a path full of exciting possibilities. As V2X technology continues to evolve, it will undoubtedly create new challenges and opportunities for innovative solutions – a perfect playground for those with a passion for coding and problem-solving.