Understanding Real-Time Data Streaming Technologies: A Deep Dive into Apache Kafka
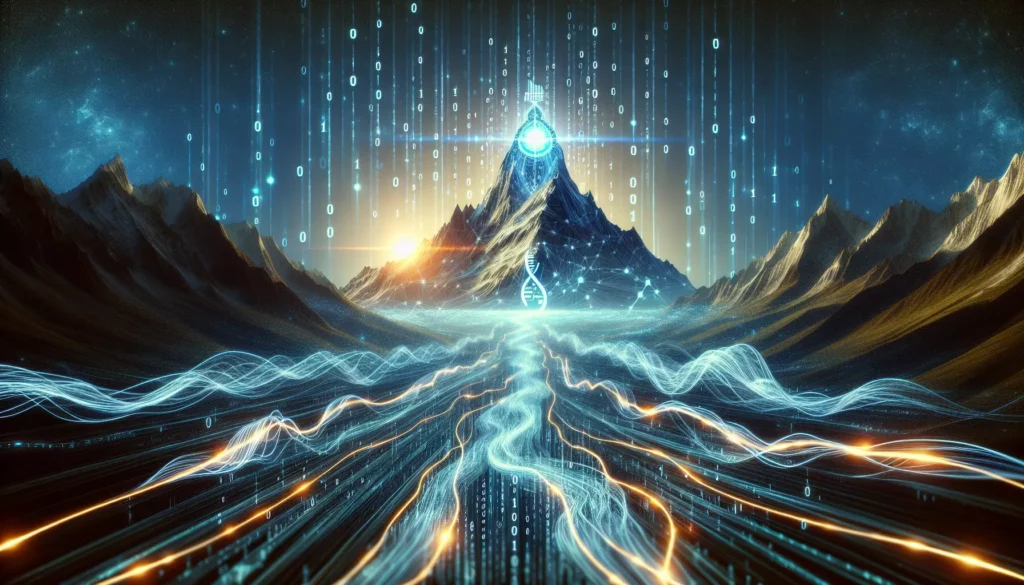
In today’s fast-paced digital world, the ability to process and analyze data in real-time has become crucial for businesses across various industries. Real-time data streaming technologies have emerged as powerful tools to handle the massive influx of data generated by modern applications, IoT devices, and user interactions. Among these technologies, Apache Kafka stands out as a robust and widely adopted solution for building real-time data pipelines and streaming applications.
In this comprehensive guide, we’ll explore the world of real-time data streaming, with a particular focus on Apache Kafka. We’ll discuss its architecture, key concepts, and how it can be leveraged to build scalable and efficient data streaming solutions. Whether you’re a beginner looking to understand the basics or an experienced developer seeking to enhance your skills, this article will provide valuable insights into the world of real-time data streaming.
Table of Contents
- Introduction to Real-Time Data Streaming
- Apache Kafka: An Overview
- Key Concepts in Apache Kafka
- Apache Kafka Architecture
- Setting Up Apache Kafka
- Producing and Consuming Messages in Kafka
- Kafka Streams API
- Use Cases and Applications
- Best Practices and Considerations
- Comparison with Other Streaming Technologies
- Conclusion
1. Introduction to Real-Time Data Streaming
Real-time data streaming refers to the continuous flow of data from various sources to target systems for immediate processing and analysis. Unlike traditional batch processing, where data is collected over time and processed in large chunks, real-time streaming allows for instantaneous data handling, enabling businesses to make quick decisions based on up-to-the-minute information.
Some key characteristics of real-time data streaming include:
- Low latency: Data is processed as soon as it’s generated or received.
- High throughput: Large volumes of data can be handled efficiently.
- Scalability: The system can easily adapt to increasing data loads.
- Fault-tolerance: The ability to handle failures and ensure data integrity.
- Real-time analytics: Immediate insights can be derived from streaming data.
Real-time data streaming has numerous applications across industries, including:
- Financial services: Real-time fraud detection and stock market analysis
- E-commerce: Personalized recommendations and inventory management
- IoT: Monitoring and analyzing sensor data from connected devices
- Social media: Trend analysis and content moderation
- Transportation: Real-time traffic monitoring and route optimization
2. Apache Kafka: An Overview
Apache Kafka is an open-source distributed event streaming platform initially developed by LinkedIn and later donated to the Apache Software Foundation. It is designed to handle high-throughput, fault-tolerant, and scalable real-time data streaming.
Key features of Apache Kafka include:
- Publish-subscribe messaging system
- Distributed architecture for high scalability and fault-tolerance
- Persistent storage of stream data
- High throughput for both publishing and subscribing
- Stream processing capabilities
- Connector ecosystem for easy integration with various data sources and sinks
Kafka has gained widespread adoption in the industry, with companies like LinkedIn, Netflix, Uber, and Airbnb using it as a core component of their data infrastructure.
3. Key Concepts in Apache Kafka
To understand Apache Kafka, it’s essential to familiarize yourself with its core concepts:
3.1. Topics
A topic is a category or feed name to which records are published. Topics in Kafka are always multi-subscriber; that is, a topic can have zero, one, or many consumers that subscribe to the data written to it.
3.2. Partitions
Each topic is divided into one or more partitions, which are the unit of parallelism in Kafka. Partitions allow Kafka to distribute data across multiple servers, enabling horizontal scalability and parallel processing.
3.3. Producers
Producers are client applications that publish (write) events to Kafka topics. They are responsible for choosing which partition to send a record to within a topic.
3.4. Consumers
Consumers are client applications that subscribe to (read and process) events from Kafka topics. Consumers can be part of a consumer group, which allows for load balancing and parallel processing of data.
3.5. Brokers
Brokers are the servers that form the Kafka cluster. They are responsible for storing data and serving client requests. Each broker can handle hundreds of thousands of reads and writes per second without impacting performance.
3.6. ZooKeeper
ZooKeeper is a centralized service for maintaining configuration information, naming, providing distributed synchronization, and providing group services. Kafka uses ZooKeeper to manage the broker cluster and to perform leader election for partitions.
4. Apache Kafka Architecture
The Apache Kafka architecture consists of several key components working together to provide a scalable and fault-tolerant streaming platform:
4.1. Kafka Cluster
A Kafka cluster is composed of one or more servers (brokers), each of which is responsible for storing and serving data. The cluster is the central component of the Kafka ecosystem, providing storage and retrieval of published data streams.
4.2. Topics and Partitions
Topics are logical channels to which data is published. Each topic is divided into one or more partitions, which are the unit of parallelism in Kafka. Partitions are distributed across the brokers in the cluster, allowing for horizontal scalability.
4.3. Replication
Kafka provides built-in replication of partitions across multiple brokers. This ensures fault tolerance and high availability. Each partition has one leader broker and zero or more follower brokers.
4.4. Producers and Consumers
Producers publish data to topics, while consumers subscribe to topics and process the published data. Producers and consumers are completely decoupled, allowing for great flexibility in system design.
4.5. Consumer Groups
Consumers can be organized into consumer groups for load balancing and parallel processing. Each partition is consumed by only one consumer within a group, ensuring that data is processed efficiently and in order.
4.6. ZooKeeper Ensemble
ZooKeeper is used for managing and coordinating Kafka brokers. It stores metadata about the Kafka cluster, topics, and partitions, and helps in leader election for partitions.
5. Setting Up Apache Kafka
To get started with Apache Kafka, you’ll need to set up a Kafka environment. Here’s a step-by-step guide to setting up a basic Kafka installation:
5.1. Prerequisites
- Java 8 or higher
- ZooKeeper (Kafka comes bundled with ZooKeeper, but it’s recommended to use a separate installation for production environments)
5.2. Download and Extract Kafka
Download the latest version of Kafka from the official Apache Kafka website and extract it to a directory of your choice.
5.3. Configure Kafka
Edit the config/server.properties
file to set up basic configurations such as the broker ID, listeners, and log directories.
5.4. Start ZooKeeper
Start the ZooKeeper server using the following command:
bin/zookeeper-server-start.sh config/zookeeper.properties
5.5. Start Kafka Server
Start the Kafka server using the following command:
bin/kafka-server-start.sh config/server.properties
5.6. Create a Topic
Create a test topic using the kafka-topics script:
bin/kafka-topics.sh --create --topic test-topic --bootstrap-server localhost:9092 --partitions 1 --replication-factor 1
With these steps, you’ll have a basic Kafka setup running on your local machine, ready for producing and consuming messages.
6. Producing and Consuming Messages in Kafka
Once you have Kafka set up, you can start producing and consuming messages. Here’s a basic example of how to produce and consume messages using Kafka’s command-line tools:
6.1. Producing Messages
To produce messages to a Kafka topic, you can use the kafka-console-producer script:
bin/kafka-console-producer.sh --topic test-topic --bootstrap-server localhost:9092
After running this command, you can type messages and press Enter to send them to the topic.
6.2. Consuming Messages
To consume messages from a Kafka topic, you can use the kafka-console-consumer script:
bin/kafka-console-consumer.sh --topic test-topic --from-beginning --bootstrap-server localhost:9092
This command will display all messages in the topic, including those that were sent before the consumer was started.
6.3. Using Kafka with Programming Languages
While the command-line tools are useful for testing and simple scenarios, in real-world applications, you’ll typically use Kafka client libraries in your preferred programming language. Here’s a simple example of producing and consuming messages using Java:
Producing Messages in Java
import org.apache.kafka.clients.producer.*;
import java.util.Properties;
public class SimpleProducer {
public static void main(String[] args) {
Properties props = new Properties();
props.put("bootstrap.servers", "localhost:9092");
props.put("key.serializer", "org.apache.kafka.common.serialization.StringSerializer");
props.put("value.serializer", "org.apache.kafka.common.serialization.StringSerializer");
Producer<String, String> producer = new KafkaProducer<>(props);
for (int i = 0; i < 10; i++) {
producer.send(new ProducerRecord<String, String>("test-topic",
Integer.toString(i), "Hello World " + i));
}
producer.close();
}
}
Consuming Messages in Java
import org.apache.kafka.clients.consumer.*;
import java.time.Duration;
import java.util.Arrays;
import java.util.Properties;
public class SimpleConsumer {
public static void main(String[] args) {
Properties props = new Properties();
props.put("bootstrap.servers", "localhost:9092");
props.put("group.id", "test-group");
props.put("key.deserializer", "org.apache.kafka.common.serialization.StringDeserializer");
props.put("value.deserializer", "org.apache.kafka.common.serialization.StringDeserializer");
KafkaConsumer<String, String> consumer = new KafkaConsumer<>(props);
consumer.subscribe(Arrays.asList("test-topic"));
while (true) {
ConsumerRecords<String, String> records = consumer.poll(Duration.ofMillis(100));
for (ConsumerRecord<String, String> record : records) {
System.out.printf("offset = %d, key = %s, value = %s%n",
record.offset(), record.key(), record.value());
}
}
}
}
These examples demonstrate the basic principles of producing and consuming messages in Kafka using Java. Similar client libraries are available for other programming languages such as Python, Go, and JavaScript.
7. Kafka Streams API
The Kafka Streams API is a client library for building applications and microservices that process and analyze data stored in Kafka. It enables you to build stream processing applications with just a few lines of code, without the need for a separate processing cluster.
Key features of the Kafka Streams API include:
- Exactly-once processing semantics
- One-record-at-a-time processing (no micro-batching)
- Windowing support
- Local state management with fault-tolerance
- Scalability and elasticity without downtime
Here’s a simple example of a Kafka Streams application that counts the occurrences of words in a stream of text:
import org.apache.kafka.common.serialization.Serdes;
import org.apache.kafka.streams.KafkaStreams;
import org.apache.kafka.streams.StreamsBuilder;
import org.apache.kafka.streams.StreamsConfig;
import org.apache.kafka.streams.kstream.KStream;
import org.apache.kafka.streams.kstream.KTable;
import org.apache.kafka.streams.kstream.Produced;
import java.util.Arrays;
import java.util.Properties;
public class WordCountApplication {
public static void main(String[] args) {
Properties config = new Properties();
config.put(StreamsConfig.APPLICATION_ID_CONFIG, "wordcount-application");
config.put(StreamsConfig.BOOTSTRAP_SERVERS_CONFIG, "localhost:9092");
config.put(StreamsConfig.DEFAULT_KEY_SERDE_CLASS_CONFIG, Serdes.String().getClass());
config.put(StreamsConfig.DEFAULT_VALUE_SERDE_CLASS_CONFIG, Serdes.String().getClass());
StreamsBuilder builder = new StreamsBuilder();
KStream<String, String> textLines = builder.stream("text-lines");
KTable<String, Long> wordCounts = textLines
.flatMapValues(textLine -> Arrays.asList(textLine.toLowerCase().split("\\W+")))
.groupBy((key, word) -> word)
.count();
wordCounts.toStream().to("word-count-output", Produced.with(Serdes.String(), Serdes.Long()));
KafkaStreams streams = new KafkaStreams(builder.build(), config);
streams.start();
Runtime.getRuntime().addShutdownHook(new Thread(streams::close));
}
}
This example demonstrates how to use the Kafka Streams API to process a stream of text lines, split them into words, and count the occurrences of each word. The results are then sent to an output topic.
8. Use Cases and Applications
Apache Kafka’s versatility and scalability make it suitable for a wide range of use cases across various industries. Some common applications of Kafka include:
8.1. Log Aggregation
Kafka can be used to collect logs from multiple services and applications, centralizing them for easier processing and analysis. This is particularly useful in microservices architectures where logs need to be aggregated from numerous distributed services.
8.2. Event-Driven Architectures
Kafka’s publish-subscribe model makes it an excellent choice for building event-driven systems. It can act as a central event bus, allowing different components of a system to communicate asynchronously through events.
8.3. Real-Time Analytics
With its ability to handle high-throughput data streams, Kafka is often used as the backbone for real-time analytics pipelines. It can ingest data from various sources and feed it into analytics engines or stream processing applications for immediate insights.
8.4. Data Integration
Kafka’s Connect API allows for easy integration with various data sources and sinks, making it a powerful tool for building data integration pipelines. It can be used to synchronize data between different systems or to build ETL (Extract, Transform, Load) processes.
8.5. Metrics and Monitoring
Kafka can be used to collect and process metrics and monitoring data from distributed systems. Its ability to handle high volumes of data makes it suitable for scenarios where large amounts of telemetry data need to be processed in real-time.
8.6. Stream Processing
The Kafka Streams API enables the development of stream processing applications directly on top of Kafka. This can be used for various purposes such as data enrichment, anomaly detection, or complex event processing.
9. Best Practices and Considerations
When working with Apache Kafka, it’s important to follow best practices to ensure optimal performance, scalability, and reliability. Here are some key considerations:
9.1. Topic Design
- Choose an appropriate number of partitions based on your throughput requirements and the number of consumers.
- Use meaningful and consistent naming conventions for topics.
- Consider data retention policies and configure them appropriately.
9.2. Producer Configuration
- Set appropriate batch size and linger time to balance between latency and throughput.
- Configure proper acknowledgment settings (acks) based on your reliability requirements.
- Use compression to reduce network bandwidth and storage usage.
9.3. Consumer Configuration
- Choose an appropriate consumer group design to ensure efficient parallel processing.
- Configure proper offset management to handle failures and restarts.
- Set appropriate fetch size and max poll records to optimize throughput.
9.4. Monitoring and Metrics
- Monitor key metrics such as broker and topic-level throughput, latency, and consumer lag.
- Set up alerts for critical issues like broker failures or consumer group rebalances.
- Use tools like Kafka Manager or Confluent Control Center for cluster management and monitoring.
9.5. Security
- Enable SSL/TLS encryption for client-broker communication.
- Implement authentication mechanisms such as SASL.
- Use ACLs (Access Control Lists) to manage permissions on topics and consumer groups.
9.6. Scalability and Performance
- Properly size your Kafka cluster based on your data retention and throughput requirements.
- Use multiple brokers and ensure proper replication for fault tolerance.
- Optimize your hardware configuration, particularly disk I/O and network capacity.
10. Comparison with Other Streaming Technologies
While Apache Kafka is a popular choice for real-time data streaming, it’s not the only option available. Here’s a brief comparison with some other streaming technologies:
10.1. Apache Kafka vs. Apache Pulsar
Apache Pulsar is another distributed messaging and streaming platform that offers some advantages over Kafka, such as:
- Multi-tenancy support
- Built-in support for multiple storage tiers
- Unified messaging and streaming model
However, Kafka still has a larger ecosystem and more widespread adoption.
10.2. Apache Kafka vs. RabbitMQ
RabbitMQ is a message broker that excels at traditional messaging scenarios but lacks some of Kafka’s streaming capabilities. Kafka is generally better suited for high-throughput data streaming, while RabbitMQ might be preferred for complex routing scenarios or when low latency is critical.
10.3. Apache Kafka vs. Apache Flink
Apache Flink is a stream processing framework that can use Kafka as a source or sink. While Kafka provides the messaging and storage layer, Flink offers more advanced stream processing capabilities. They are often used together in streaming architectures.
10.4. Apache Kafka vs. Amazon Kinesis
Amazon Kinesis is a fully managed streaming data service that’s part of the AWS ecosystem. While it offers similar capabilities to Kafka, it’s tightly integrated with other AWS services. Kafka provides more flexibility and can be deployed on-premises or in any cloud environment.
11. Conclusion
Apache Kafka has emerged as a powerful and versatile tool for building real-time data streaming applications. Its distributed architecture, high throughput, and scalability make it suitable for a wide range of use cases, from log aggregation to complex event processing.
By understanding the key concepts, architecture, and best practices of Kafka, developers can leverage its capabilities to build robust and scalable streaming solutions. Whether you’re dealing with real-time analytics, event-driven architectures, or data integration pipelines, Kafka provides a solid foundation for handling the challenges of modern data-intensive applications.
As the field of real-time data processing continues to evolve, staying updated with the latest developments in Kafka and other streaming technologies will be crucial for developers and data engineers. By mastering these tools, you’ll be well-equipped to tackle the growing demands of real-time data processing in today’s fast-paced digital landscape.