Mastering Remote Procedure Calls (RPCs) and gRPC: Powering Distributed Systems Communication
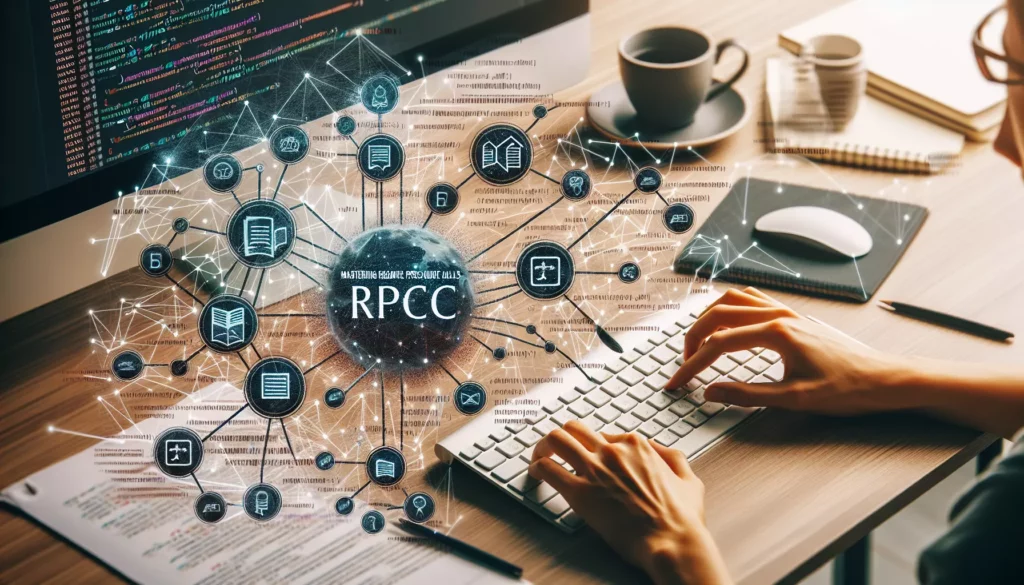
In the ever-evolving landscape of software development, distributed systems have become increasingly prevalent. As applications grow in complexity and scale, the need for efficient communication between different components of a system becomes paramount. This is where Remote Procedure Calls (RPCs) and gRPC come into play, offering powerful solutions for enabling seamless communication between distributed systems. In this comprehensive guide, we’ll dive deep into the world of RPCs and gRPC, exploring their concepts, benefits, and practical applications.
Understanding Remote Procedure Calls (RPCs)
Remote Procedure Calls (RPCs) are a protocol that allows a program to execute a procedure or function on another computer as if it were a local procedure call. This abstraction simplifies the development of distributed systems by hiding the complexities of network communication from the developer.
Key Concepts of RPCs
- Client-Server Model: RPCs follow a client-server model where the client initiates a request to execute a procedure on a remote server.
- Stub Generation: RPCs typically involve generating client and server stubs, which handle the serialization and deserialization of data.
- Transparency: RPCs aim to make remote procedure calls appear as local calls to the developer, abstracting away network details.
- Synchronous Communication: Traditional RPCs are synchronous, meaning the client waits for the server’s response before continuing execution.
Benefits of Using RPCs
- Simplicity: RPCs simplify the development of distributed systems by providing a familiar procedure call interface.
- Language Agnostic: Many RPC implementations support multiple programming languages, enabling cross-language communication.
- Performance: RPCs can be more efficient than other communication protocols, especially for frequent, small data exchanges.
- Standardization: RPC protocols often provide standardized ways of defining interfaces and data structures.
Introduction to gRPC
gRPC is a modern, open-source framework developed by Google that builds upon the concepts of traditional RPCs. It uses Protocol Buffers as its interface definition language and supports features like streaming, flow control, and authentication.
Key Features of gRPC
- Protocol Buffers: gRPC uses Protocol Buffers (protobuf) for efficient, binary serialization of data.
- HTTP/2: Built on top of HTTP/2, gRPC supports features like multiplexing and header compression.
- Bi-directional Streaming: gRPC allows for both client-side and server-side streaming.
- Language Support: gRPC has official support for multiple programming languages, including Java, C++, Python, Go, and more.
- Interceptors: gRPC provides a mechanism for intercepting and modifying RPC calls, useful for implementing cross-cutting concerns.
Advantages of gRPC
- Performance: gRPC’s use of Protocol Buffers and HTTP/2 results in efficient, low-latency communication.
- Strong Typing: The use of Protocol Buffers ensures strong typing and contract-first API development.
- Streaming: gRPC’s support for bi-directional streaming enables real-time, event-driven architectures.
- Cross-platform: With support for multiple languages and platforms, gRPC facilitates the development of polyglot systems.
- Tooling: gRPC comes with a rich ecosystem of tools for tasks like code generation, testing, and monitoring.
Implementing RPCs and gRPC
Now that we’ve covered the basics, let’s dive into the practical aspects of implementing RPCs and gRPC in your projects.
Implementing Traditional RPCs
While there are various RPC implementations, we’ll focus on a simple example using Python’s xmlrpc library.
Server-side Implementation
from xmlrpc.server import SimpleXMLRPCServer
def add(x, y):
return x + y
server = SimpleXMLRPCServer(("localhost", 8000))
print("Listening on port 8000...")
server.register_function(add, "add")
server.serve_forever()
Client-side Implementation
import xmlrpc.client
proxy = xmlrpc.client.ServerProxy("http://localhost:8000/")
result = proxy.add(5, 3)
print(f"5 + 3 = {result}")
In this example, we’ve created a simple RPC server that exposes an “add” function. The client can call this function as if it were local, despite it being executed on the server.
Implementing gRPC
Now, let’s look at how to implement the same functionality using gRPC with Python.
Defining the Protocol Buffer
First, we need to define our service and message types in a .proto file:
syntax = "proto3";
package calculator;
service Calculator {
rpc Add (AddRequest) returns (AddResponse) {}
}
message AddRequest {
int32 x = 1;
int32 y = 2;
}
message AddResponse {
int32 result = 1;
}
Server-side Implementation
import grpc
from concurrent import futures
import calculator_pb2
import calculator_pb2_grpc
class CalculatorServicer(calculator_pb2_grpc.CalculatorServicer):
def Add(self, request, context):
result = request.x + request.y
return calculator_pb2.AddResponse(result=result)
def serve():
server = grpc.server(futures.ThreadPoolExecutor(max_workers=10))
calculator_pb2_grpc.add_CalculatorServicer_to_server(CalculatorServicer(), server)
server.add_insecure_port('[::]:50051')
server.start()
server.wait_for_termination()
if __name__ == '__main__':
serve()
Client-side Implementation
import grpc
import calculator_pb2
import calculator_pb2_grpc
def run():
with grpc.insecure_channel('localhost:50051') as channel:
stub = calculator_pb2_grpc.CalculatorStub(channel)
response = stub.Add(calculator_pb2.AddRequest(x=5, y=3))
print(f"5 + 3 = {response.result}")
if __name__ == '__main__':
run()
This gRPC implementation provides the same functionality as our RPC example but with the added benefits of strong typing, efficient serialization, and the potential for streaming and other advanced features.
Best Practices for Using RPCs and gRPC
When working with RPCs and gRPC, consider the following best practices to ensure optimal performance and maintainability:
- Define Clear Interfaces: Use Protocol Buffers (for gRPC) or other interface definition languages to clearly define your service interfaces.
- Version Your APIs: Implement versioning in your RPC interfaces to allow for backwards compatibility as your system evolves.
- Handle Errors Gracefully: Implement proper error handling and propagate meaningful error messages back to clients.
- Use Streaming Judiciously: In gRPC, use streaming for scenarios that truly benefit from it, such as real-time updates or processing large datasets.
- Implement Security: Use TLS/SSL for secure communication and implement authentication and authorization mechanisms.
- Monitor and Profile: Implement monitoring and profiling to track the performance and health of your RPC services.
- Consider Load Balancing: For high-traffic services, implement load balancing to distribute requests across multiple servers.
- Optimize for Performance: Use appropriate data types, minimize payload sizes, and consider caching frequently accessed data.
Challenges and Considerations
While RPCs and gRPC offer numerous benefits, it’s important to be aware of potential challenges:
- Network Reliability: RPCs are susceptible to network issues. Implement proper error handling and retry mechanisms.
- Latency: Remote calls inherently involve network latency. Design your system to minimize unnecessary calls.
- Versioning: As your system evolves, managing versioning of RPC interfaces can become complex.
- Language Limitations: Some languages may have limited support for certain RPC features or may require additional setup.
- Learning Curve: Especially with gRPC, there can be a significant learning curve for teams new to the technology.
RPCs and gRPC in the Context of Coding Education
Understanding RPCs and gRPC is crucial for aspiring software engineers, especially those preparing for technical interviews at major tech companies. Here’s why:
- System Design Questions: Technical interviews often include system design questions where knowledge of efficient communication protocols like gRPC can be a significant advantage.
- Distributed Systems: As companies build increasingly complex distributed systems, familiarity with RPCs and gRPC becomes more valuable.
- Performance Optimization: Understanding how to optimize communication between services is a key skill for building scalable systems.
- Modern Architecture Patterns: Microservices and other modern architecture patterns often rely on efficient inter-service communication, making RPC knowledge essential.
- Language Agnostic Skills: RPC concepts, especially with gRPC, apply across multiple programming languages, demonstrating versatility to potential employers.
Conclusion
Remote Procedure Calls (RPCs) and gRPC are powerful tools in the modern software developer’s toolkit. They enable efficient communication between distributed systems, simplifying the development of complex, scalable applications. By mastering these technologies, you’ll be better equipped to design and implement robust distributed systems, a skill highly valued in today’s software industry.
As you continue your journey in coding education and prepare for technical interviews, remember that understanding RPCs and gRPC is not just about memorizing syntax or protocols. It’s about grasping the underlying concepts of distributed communication, efficiency, and scalability. Practice implementing RPC systems, experiment with gRPC’s advanced features, and consider how these technologies can be applied to solve real-world problems.
With the knowledge and skills you’ve gained from this guide, you’re now better prepared to tackle complex system design questions, contribute to modern software architectures, and showcase your understanding of critical concepts in distributed systems. Keep practicing, stay curious, and you’ll be well on your way to excelling in your coding journey and future technical interviews.