Exploring Satellite and Remote Sensing Data Programming: A Comprehensive Guide
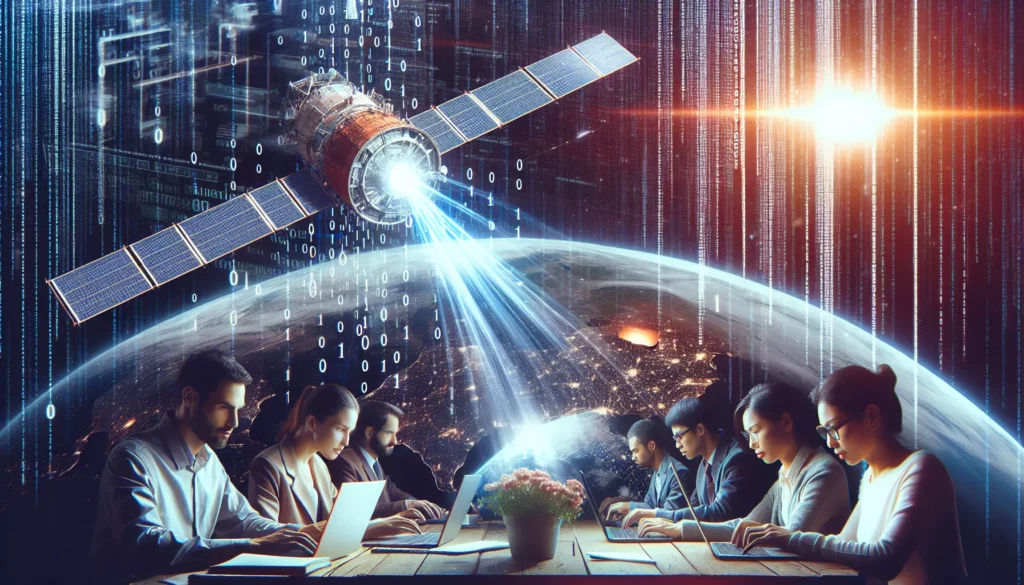
In today’s data-driven world, satellite and remote sensing technologies play a crucial role in environmental monitoring, urban planning, disaster management, and countless other applications. As a programmer, diving into this field opens up exciting opportunities to work with vast amounts of data and contribute to solving real-world problems. In this comprehensive guide, we’ll explore the world of satellite and remote sensing data programming, covering everything from the basics to advanced techniques and practical applications.
Table of Contents
- Introduction to Satellite and Remote Sensing Data
- Types of Satellite and Remote Sensing Data
- Data Sources and Acquisition
- Programming Tools and Libraries
- Data Processing Techniques
- Image Analysis and Interpretation
- Machine Learning in Remote Sensing
- Real-world Applications
- Challenges and Considerations
- Future Trends and Opportunities
- Conclusion
1. Introduction to Satellite and Remote Sensing Data
Satellite and remote sensing data refer to information collected about the Earth’s surface and atmosphere from a distance, typically using satellites or aircraft. This data provides valuable insights into various phenomena, including land use changes, climate patterns, and natural disasters.
As a programmer working with this data, you’ll be dealing with large datasets that often require specialized processing techniques and analysis methods. The field combines elements of computer science, geography, environmental science, and data analysis, making it an interdisciplinary and exciting area to explore.
2. Types of Satellite and Remote Sensing Data
There are several types of satellite and remote sensing data, each with its own characteristics and applications:
- Optical imagery: Captures visible and near-infrared light reflected from the Earth’s surface.
- Radar imagery: Uses microwave signals to penetrate clouds and darkness, providing data in all weather conditions.
- Thermal imagery: Detects heat signatures, useful for monitoring wildfires and urban heat islands.
- Hyperspectral imagery: Collects data across hundreds of spectral bands, enabling detailed analysis of surface materials.
- LiDAR (Light Detection and Ranging): Uses laser pulses to create high-resolution 3D maps of the Earth’s surface.
Understanding these data types is crucial for choosing the right dataset for your specific application and interpreting the results accurately.
3. Data Sources and Acquisition
There are numerous sources for satellite and remote sensing data, many of which provide free access for research and educational purposes:
- NASA Earth Observing System Data and Information System (EOSDIS): Offers a wide range of Earth science data products.
- European Space Agency (ESA) Copernicus Open Access Hub: Provides free, full and open access to Sentinel satellite data.
- USGS Earth Explorer: Offers access to satellite imagery, aerial photography, and other remote sensing datasets.
- Google Earth Engine: A cloud-based platform for planetary-scale geospatial analysis.
- Planet Labs: Provides high-resolution, frequently updated satellite imagery (some datasets are commercial).
To acquire data from these sources, you’ll typically need to create an account and use their respective APIs or download tools. Many of these platforms provide Python libraries or RESTful APIs for programmatic access to their data catalogs.
4. Programming Tools and Libraries
Several programming languages and libraries are commonly used in satellite and remote sensing data analysis:
Python Libraries:
- GDAL (Geospatial Data Abstraction Library): For reading and writing raster and vector geospatial data formats.
- Rasterio: A high-level interface to GDAL for working with geospatial raster data.
- GeoPandas: Extends pandas to allow spatial operations on geometric types.
- Xarray: For working with labeled multi-dimensional arrays, particularly useful for satellite data.
- Satpy: A python library for reading and manipulating meteorological remote sensing data.
- EarthPy: Makes it easier to plot and work with spatial raster and vector data.
Other Tools:
- QGIS: An open-source Geographic Information System (GIS) that can be extended with Python plugins.
- ArcGIS: A commercial GIS software suite with Python scripting capabilities.
- R: Offers packages like ‘raster’ and ‘sf’ for spatial data analysis.
Here’s a simple example of reading a GeoTIFF file using Rasterio in Python:
import rasterio
import matplotlib.pyplot as plt
# Open the GeoTIFF file
with rasterio.open('path/to/your/geotiff.tif') as src:
# Read the first band
band1 = src.read(1)
# Plot the image
plt.imshow(band1)
plt.colorbar()
plt.title('Satellite Image')
plt.show()
5. Data Processing Techniques
Processing satellite and remote sensing data often involves several steps:
5.1 Preprocessing
- Radiometric correction: Adjusting for atmospheric effects and sensor calibration.
- Geometric correction: Aligning images to a map projection.
- Orthorectification: Correcting for terrain-induced distortions.
5.2 Data Fusion
Combining data from multiple sensors or time periods to create more informative datasets.
5.3 Change Detection
Analyzing differences between images of the same area taken at different times.
5.4 Spectral Analysis
Extracting information from different spectral bands to identify materials or land cover types.
Here’s a simple example of calculating the Normalized Difference Vegetation Index (NDVI) using Rasterio:
import rasterio
import numpy as np
# Open the red and near-infrared bands
with rasterio.open('path/to/red_band.tif') as red:
red_band = red.read(1)
with rasterio.open('path/to/nir_band.tif') as nir:
nir_band = nir.read(1)
# Calculate NDVI
ndvi = (nir_band - red_band) / (nir_band + red_band)
# Save the result
profile = red.profile
profile.update(dtype=rasterio.float32, count=1)
with rasterio.open('path/to/ndvi_output.tif', 'w', **profile) as dst:
dst.write(ndvi.astype(rasterio.float32), 1)
6. Image Analysis and Interpretation
Image analysis is a crucial step in extracting meaningful information from satellite and remote sensing data. Some common techniques include:
6.1 Classification
Categorizing pixels into different land cover types (e.g., forest, urban, water) using supervised or unsupervised methods.
6.2 Object-Based Image Analysis (OBIA)
Segmenting images into meaningful objects and analyzing their characteristics.
6.3 Time Series Analysis
Analyzing changes in an area over time, useful for monitoring deforestation, urban growth, or crop cycles.
6.4 Texture Analysis
Examining spatial patterns in image data to extract additional information about surface characteristics.
Here’s a basic example of performing unsupervised classification using scikit-learn:
from sklearn.cluster import KMeans
import rasterio
import numpy as np
# Read the image
with rasterio.open('path/to/image.tif') as src:
image = src.read()
profile = src.profile
# Reshape the image for clustering
n_bands, height, width = image.shape
image_2d = image.reshape((n_bands, height * width)).T
# Perform K-means clustering
n_clusters = 5
kmeans = KMeans(n_clusters=n_clusters, random_state=42)
labels = kmeans.fit_predict(image_2d)
# Reshape the result back to 2D
classified_image = labels.reshape((height, width))
# Save the classified image
profile.update(dtype=rasterio.uint8, count=1)
with rasterio.open('path/to/classified_image.tif', 'w', **profile) as dst:
dst.write(classified_image.astype(rasterio.uint8), 1)
7. Machine Learning in Remote Sensing
Machine learning techniques are increasingly being applied to satellite and remote sensing data analysis, offering powerful tools for extracting insights from large and complex datasets.
7.1 Supervised Learning
Used for tasks like land cover classification, crop yield prediction, and object detection. Common algorithms include Random Forests, Support Vector Machines (SVM), and Neural Networks.
7.2 Unsupervised Learning
Useful for discovering patterns in data without predefined categories. Techniques like K-means clustering and Principal Component Analysis (PCA) are often used.
7.3 Deep Learning
Convolutional Neural Networks (CNNs) and other deep learning architectures have shown great promise in tasks like image classification, object detection, and semantic segmentation of satellite imagery.
Here’s an example of using a simple CNN for classifying satellite image patches:
from tensorflow.keras.models import Sequential
from tensorflow.keras.layers import Conv2D, MaxPooling2D, Flatten, Dense
# Define the model
model = Sequential([
Conv2D(32, (3, 3), activation='relu', input_shape=(64, 64, 3)),
MaxPooling2D((2, 2)),
Conv2D(64, (3, 3), activation='relu'),
MaxPooling2D((2, 2)),
Conv2D(64, (3, 3), activation='relu'),
Flatten(),
Dense(64, activation='relu'),
Dense(10, activation='softmax')
])
# Compile the model
model.compile(optimizer='adam',
loss='sparse_categorical_crossentropy',
metrics=['accuracy'])
# Train the model (assuming you have X_train and y_train)
model.fit(X_train, y_train, epochs=10, validation_split=0.2)
8. Real-world Applications
Satellite and remote sensing data have a wide range of applications across various fields:
8.1 Environmental Monitoring
- Tracking deforestation and reforestation
- Monitoring air and water quality
- Studying climate change impacts
8.2 Agriculture
- Crop yield prediction
- Precision agriculture and irrigation management
- Monitoring soil health
8.3 Urban Planning
- Mapping urban growth and land use changes
- Traffic and transportation analysis
- Green space monitoring
8.4 Disaster Management
- Natural disaster impact assessment
- Early warning systems for floods, wildfires, etc.
- Post-disaster recovery monitoring
8.5 Ocean and Coastal Monitoring
- Tracking oil spills
- Monitoring coral reef health
- Studying ocean currents and temperatures
9. Challenges and Considerations
Working with satellite and remote sensing data comes with several challenges:
9.1 Data Volume
Satellite data often comes in large volumes, requiring efficient data management and processing strategies.
9.2 Data Quality
Issues like cloud cover, atmospheric interference, and sensor errors can affect data quality and need to be addressed.
9.3 Temporal and Spatial Resolution
Different applications require different temporal and spatial resolutions, which may not always be available.
9.4 Data Integration
Combining data from multiple sources and sensors can be challenging due to differences in formats, resolutions, and coordinate systems.
9.5 Computational Resources
Processing large satellite datasets often requires significant computational resources, potentially necessitating the use of cloud computing or high-performance computing facilities.
10. Future Trends and Opportunities
The field of satellite and remote sensing data programming is rapidly evolving. Some exciting trends and opportunities include:
10.1 Increased Data Availability
More satellites are being launched, providing higher resolution and more frequent data coverage.
10.2 Advanced AI and Machine Learning
Continued advancements in AI and machine learning algorithms will enable more sophisticated analysis of satellite data.
10.3 Cloud Computing and Big Data
Cloud platforms like Google Earth Engine are making it easier to process and analyze large satellite datasets without the need for extensive local infrastructure.
10.4 Integration with IoT and Ground Sensors
Combining satellite data with ground-based sensors and Internet of Things (IoT) devices will provide more comprehensive environmental monitoring capabilities.
10.5 Democratization of Space
The increasing accessibility of small satellites and CubeSats is opening up new opportunities for targeted Earth observation missions.
11. Conclusion
Satellite and remote sensing data programming offers a fascinating intersection of technology, environmental science, and data analysis. As a programmer in this field, you have the opportunity to work with cutting-edge technologies and contribute to solving some of the world’s most pressing environmental and social challenges.
Whether you’re interested in climate change research, urban planning, disaster management, or any of the numerous applications of remote sensing, developing skills in this area can open up exciting career opportunities. As the field continues to evolve with advancements in satellite technology, data processing techniques, and machine learning algorithms, there will be an ongoing need for skilled programmers who can extract meaningful insights from these vast and complex datasets.
By mastering the tools and techniques discussed in this guide, you’ll be well-equipped to dive into the world of satellite and remote sensing data programming. Remember to stay curious, keep learning, and don’t hesitate to explore the wealth of open-source tools and datasets available in this field. Happy coding and Earth observing!