Digital Signal Processing (DSP): Unlocking the Power of Signal Manipulation
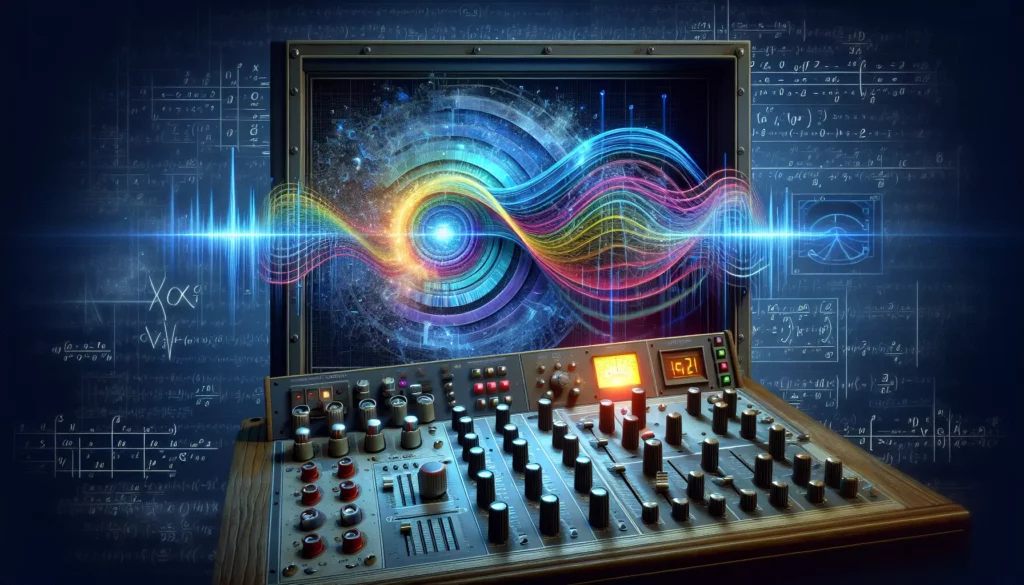
In today’s digital age, where information is constantly flowing through various channels, the ability to process and manipulate signals has become increasingly important. This is where Digital Signal Processing (DSP) comes into play. DSP is a powerful field that combines mathematics, computer science, and electrical engineering to analyze, modify, and synthesize signals in digital form. In this comprehensive guide, we’ll dive deep into the world of DSP, exploring its applications, fundamental concepts, and how it relates to coding education and programming skills development.
What is Digital Signal Processing?
Digital Signal Processing is the manipulation of signals, such as audio, video, or communication signals, using digital techniques. It involves converting analog signals into digital form, processing them using algorithms and mathematical operations, and then converting them back to analog form if necessary. DSP has revolutionized various industries, including telecommunications, audio and video processing, medical imaging, and many more.
Key Concepts in DSP
- Sampling and Quantization
- Fourier Transform
- Filtering
- Convolution
- Modulation and Demodulation
- Compression and Decompression
Applications of Digital Signal Processing
DSP finds applications in numerous fields, making it an essential skill for modern engineers and programmers. Let’s explore some of the most common applications:
1. Audio Processing
DSP plays a crucial role in audio processing, enabling various operations such as:
- Noise reduction
- Equalization
- Audio compression (e.g., MP3)
- Voice recognition
- Audio effects (e.g., reverb, delay)
For example, noise reduction algorithms use DSP techniques to analyze the frequency content of an audio signal and selectively attenuate unwanted noise while preserving the desired audio information.
2. Image and Video Processing
DSP techniques are extensively used in image and video processing applications, including:
- Image enhancement and restoration
- Edge detection and object recognition
- Video compression (e.g., MPEG, H.264)
- Medical imaging (e.g., CT scans, MRI)
- Computer vision
For instance, edge detection algorithms use DSP to identify boundaries between different objects in an image, which is crucial for tasks like object recognition and image segmentation.
3. Telecommunications
The telecommunications industry heavily relies on DSP for various operations:
- Signal modulation and demodulation
- Error correction coding
- Echo cancellation
- Frequency hopping in spread spectrum systems
- Digital filtering for signal separation
DSP enables efficient and reliable communication by allowing signals to be processed, compressed, and transmitted over various channels with minimal loss of information.
4. Control Systems
DSP is used in control systems for:
- Feedback control loops
- System identification
- Adaptive filtering
- Predictive maintenance
These applications help in creating more efficient and responsive control systems in various industries, from robotics to industrial automation.
5. Biomedical Engineering
In the field of biomedical engineering, DSP is used for:
- ECG and EEG signal analysis
- Medical imaging reconstruction
- Prosthetic device control
- Hearing aid design
DSP techniques allow for more accurate diagnosis and treatment in healthcare by processing and analyzing complex biological signals.
Fundamental Concepts in Digital Signal Processing
To understand and implement DSP algorithms, it’s essential to grasp some fundamental concepts. Let’s explore these in more detail:
1. Sampling and Quantization
Sampling is the process of converting a continuous-time signal into a discrete-time signal by taking measurements at regular intervals. The sampling rate, or sampling frequency, determines how often samples are taken. The Nyquist-Shannon sampling theorem states that to accurately reconstruct a signal, the sampling rate must be at least twice the highest frequency component in the signal.
Quantization is the process of mapping a large set of input values to a smaller set of output values. In digital systems, this involves converting continuous amplitude values to discrete levels, typically represented by binary numbers.
2. Fourier Transform
The Fourier Transform is a fundamental tool in DSP that allows us to analyze signals in the frequency domain. It decomposes a signal into its constituent frequencies, providing valuable insights into the signal’s spectral content. The most commonly used forms in digital signal processing are:
- Discrete Fourier Transform (DFT)
- Fast Fourier Transform (FFT) – an efficient algorithm for computing the DFT
Here’s a simple example of how to implement an FFT using Python and the NumPy library:
import numpy as np
import matplotlib.pyplot as plt
# Generate a simple signal
t = np.linspace(0, 1, 1000)
signal = np.sin(2 * np.pi * 10 * t) + 0.5 * np.sin(2 * np.pi * 20 * t)
# Compute the FFT
fft_result = np.fft.fft(signal)
frequencies = np.fft.fftfreq(len(t), t[1] - t[0])
# Plot the original signal and its frequency spectrum
plt.subplot(2, 1, 1)
plt.plot(t, signal)
plt.title("Original Signal")
plt.subplot(2, 1, 2)
plt.plot(frequencies, np.abs(fft_result))
plt.title("Frequency Spectrum")
plt.xlim(0, 30)
plt.tight_layout()
plt.show()
This code generates a signal composed of two sine waves, computes its FFT, and plots both the original signal and its frequency spectrum.
3. Filtering
Filtering is a fundamental operation in DSP used to remove unwanted components or features from a signal. Common types of filters include:
- Low-pass filters: Allow low-frequency components to pass while attenuating high-frequency components
- High-pass filters: Allow high-frequency components to pass while attenuating low-frequency components
- Band-pass filters: Allow a specific range of frequencies to pass while attenuating others
- Notch filters: Attenuate a specific frequency while allowing others to pass
Filters can be implemented in both the time domain (using convolution) and the frequency domain (using the Fourier Transform).
4. Convolution
Convolution is a mathematical operation that combines two signals to produce a third signal. It’s a fundamental concept in DSP, used in filtering, system analysis, and many other applications. The convolution of two discrete-time signals x[n] and h[n] is defined as:
y[n] = x[n] * h[n] = Σ x[k] * h[n-k]
Where ‘*’ denotes convolution, and the sum is taken over all possible values of k.
5. Modulation and Demodulation
Modulation is the process of encoding information onto a carrier signal, while demodulation is the process of extracting the original information from the modulated signal. These techniques are crucial in telecommunications for transmitting information over long distances or through different media.
Common modulation techniques include:
- Amplitude Modulation (AM)
- Frequency Modulation (FM)
- Phase Modulation (PM)
- Quadrature Amplitude Modulation (QAM)
6. Compression and Decompression
Signal compression is the process of reducing the amount of data required to represent a signal, while decompression is the reverse process of reconstructing the original signal from the compressed data. Compression techniques are essential for efficient storage and transmission of digital signals.
There are two main types of compression:
- Lossless compression: Preserves all original information
- Lossy compression: Achieves higher compression ratios by discarding some less important information
DSP in Coding Education and Programming Skills Development
Understanding DSP concepts and implementing DSP algorithms can significantly enhance a programmer’s skill set. Here’s how DSP relates to coding education and programming skills development:
1. Algorithm Design and Implementation
DSP provides an excellent opportunity to practice algorithm design and implementation. Many DSP algorithms, such as the Fast Fourier Transform (FFT) or various filtering techniques, require efficient implementation to handle large datasets in real-time. This challenges programmers to optimize their code and think critically about algorithmic complexity.
2. Mathematical Foundations
DSP relies heavily on mathematical concepts such as complex numbers, linear algebra, and calculus. Implementing DSP algorithms helps reinforce these mathematical foundations and their practical applications in programming.
3. Signal Processing Libraries
Learning to use signal processing libraries like NumPy, SciPy, or TensorFlow for DSP applications expands a programmer’s toolkit and familiarizes them with industry-standard tools.
4. Real-world Problem Solving
DSP problems often involve real-world scenarios, such as noise reduction in audio signals or image enhancement. Solving these problems helps developers bridge the gap between theoretical knowledge and practical applications.
5. Interdisciplinary Skills
DSP sits at the intersection of various fields, including electrical engineering, computer science, and mathematics. Exposure to DSP concepts helps programmers develop interdisciplinary skills, which are highly valued in the tech industry.
6. Preparation for Technical Interviews
Many tech companies, especially those working on audio, video, or communication systems, may include DSP-related questions in their technical interviews. Understanding DSP concepts can give candidates an edge in these situations.
Implementing DSP Algorithms: A Practical Example
Let’s walk through a practical example of implementing a simple DSP algorithm: a moving average filter. This filter is often used for noise reduction in time-series data.
import numpy as np
import matplotlib.pyplot as plt
def moving_average_filter(signal, window_size):
"""
Apply a moving average filter to the input signal.
Args:
signal (array): Input signal
window_size (int): Size of the moving average window
Returns:
array: Filtered signal
"""
return np.convolve(signal, np.ones(window_size)/window_size, mode='valid')
# Generate a noisy signal
t = np.linspace(0, 10, 1000)
clean_signal = np.sin(2 * np.pi * 1 * t)
noise = np.random.normal(0, 0.5, len(t))
noisy_signal = clean_signal + noise
# Apply moving average filter
filtered_signal = moving_average_filter(noisy_signal, window_size=20)
# Plot results
plt.figure(figsize=(12, 8))
plt.subplot(3, 1, 1)
plt.plot(t, clean_signal)
plt.title("Clean Signal")
plt.subplot(3, 1, 2)
plt.plot(t, noisy_signal)
plt.title("Noisy Signal")
plt.subplot(3, 1, 3)
plt.plot(t[10:-9], filtered_signal)
plt.title("Filtered Signal")
plt.tight_layout()
plt.show()
This example demonstrates how to implement a simple moving average filter to reduce noise in a signal. It showcases several important DSP concepts:
- Signal generation: Creating a clean sinusoidal signal and adding random noise
- Filtering: Implementing a moving average filter using convolution
- Visualization: Plotting the original, noisy, and filtered signals for comparison
Conclusion
Digital Signal Processing is a vast and powerful field with applications across numerous industries. As we’ve explored in this article, DSP concepts and techniques are essential for manipulating and analyzing signals in our increasingly digital world. From audio and image processing to telecommunications and biomedical engineering, DSP plays a crucial role in shaping the technologies we use every day.
For programmers and aspiring developers, understanding DSP principles and implementing DSP algorithms can significantly enhance their skill set. It provides opportunities to work on challenging problems, reinforces mathematical foundations, and bridges the gap between theoretical knowledge and practical applications. As the demand for signal processing in various industries continues to grow, proficiency in DSP will remain a valuable asset for any programmer.
Whether you’re interested in audio processing, computer vision, or communication systems, diving into the world of DSP will open up new possibilities and deepen your understanding of how digital information is processed and manipulated. As you continue your journey in coding education and programming skills development, consider exploring DSP concepts and implementing DSP algorithms to broaden your expertise and tackle exciting challenges in the field of signal processing.