Exploring Speech Synthesis and Text-to-Speech (TTS): Creating Applications That Read Aloud
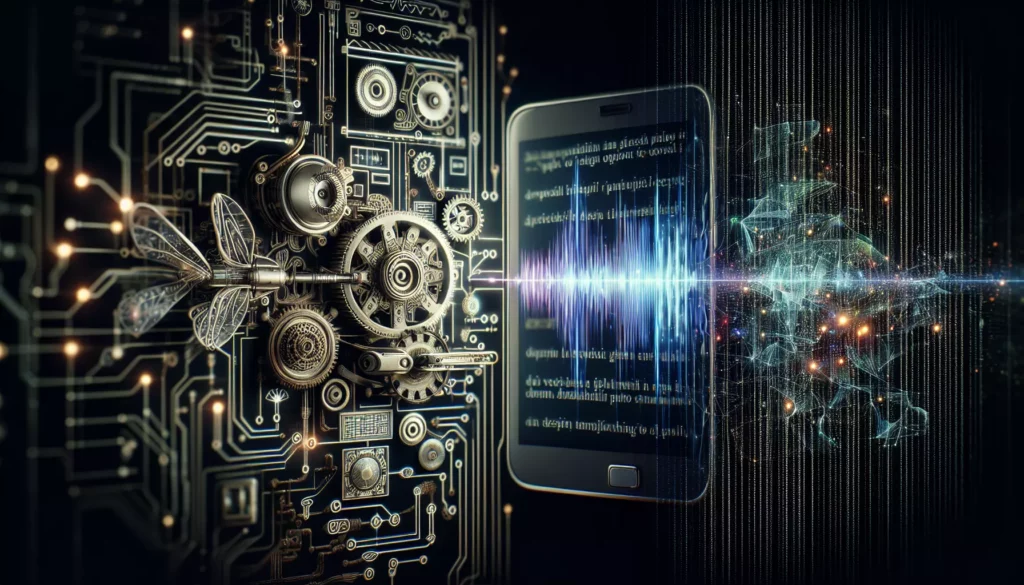
In the ever-evolving landscape of technology, speech synthesis and text-to-speech (TTS) applications have become increasingly prevalent. These innovative tools have revolutionized the way we interact with digital content, making information more accessible and enhancing user experiences across various platforms. In this comprehensive guide, we’ll delve into the world of speech synthesis and TTS, exploring their applications, technologies, and how you can create your own applications that can read text aloud.
Understanding Speech Synthesis and Text-to-Speech
Before we dive into creating applications, it’s essential to understand what speech synthesis and text-to-speech entail:
- Speech Synthesis: This is the artificial production of human speech. It involves converting written text into spoken words using computer algorithms and voice models.
- Text-to-Speech (TTS): TTS is a specific application of speech synthesis that focuses on converting digital text into spoken voice output.
Both technologies work together to create natural-sounding speech from written text, opening up a world of possibilities for developers and users alike.
Applications of Speech Synthesis and TTS
The applications of speech synthesis and TTS are vast and diverse. Here are some common use cases:
- Accessibility: Assisting visually impaired individuals in accessing written content.
- Education: Supporting language learning and reading comprehension.
- Navigation Systems: Providing voice guidance in GPS and mapping applications.
- Virtual Assistants: Enabling voice interactions with AI assistants like Siri, Alexa, and Google Assistant.
- Audiobook Production: Automating the creation of audiobooks from digital texts.
- Customer Service: Powering interactive voice response (IVR) systems in call centers.
- Content Consumption: Allowing users to listen to articles, emails, or documents while multitasking.
Technologies Behind Speech Synthesis and TTS
Several technologies and techniques are employed in modern speech synthesis and TTS systems:
1. Concatenative Synthesis
This method involves stitching together pre-recorded speech segments to create new utterances. It can produce natural-sounding speech but requires a large database of recorded speech samples.
2. Formant Synthesis
Formant synthesis creates artificial speech by modeling the frequencies of sound produced by the human vocal tract. While it can generate speech with a small footprint, it often sounds more robotic than other methods.
3. Articulatory Synthesis
This approach models the human speech production system, including the movement of the tongue, lips, and vocal cords. It can produce very natural-sounding speech but is computationally intensive.
4. Statistical Parametric Synthesis
This method uses statistical models to generate speech parameters, which are then converted into speech waveforms. It offers flexibility and can adapt to different speaking styles.
5. Neural Network-based Synthesis
Leveraging deep learning techniques, neural network-based synthesis has made significant strides in producing highly natural and expressive speech. Technologies like WaveNet and Tacotron have pushed the boundaries of speech quality.
Creating Your Own TTS Application
Now that we’ve covered the basics, let’s explore how you can create your own TTS application. We’ll use Python, as it offers a rich ecosystem of libraries and tools for speech synthesis.
Step 1: Choose a TTS Library
Several Python libraries are available for TTS. We’ll use pyttsx3, which is easy to use and works offline.
Install pyttsx3 using pip:
pip install pyttsx3
Step 2: Basic TTS Implementation
Here’s a simple Python script that converts text to speech:
import pyttsx3
def text_to_speech(text):
engine = pyttsx3.init()
engine.say(text)
engine.runAndWait()
# Example usage
text_to_speech("Hello, welcome to AlgoCademy! Let's learn about text-to-speech.")
Step 3: Customizing Voice Properties
You can customize various properties of the synthesized voice:
import pyttsx3
def customized_tts(text, rate=150, volume=1.0, voice_id=None):
engine = pyttsx3.init()
# Set properties
engine.setProperty('rate', rate)
engine.setProperty('volume', volume)
if voice_id:
engine.setProperty('voice', voice_id)
engine.say(text)
engine.runAndWait()
# Get available voices
engine = pyttsx3.init()
voices = engine.getProperty('voices')
# Example usage
customized_tts("This is a customized voice.", rate=120, volume=0.8, voice_id=voices[1].id)
Step 4: Creating a Simple TTS Application
Let’s create a simple command-line application that reads text from a file:
import pyttsx3
import sys
def read_file_aloud(file_path):
try:
with open(file_path, 'r') as file:
text = file.read()
engine = pyttsx3.init()
engine.say(text)
engine.runAndWait()
except FileNotFoundError:
print(f"Error: File '{file_path}' not found.")
except Exception as e:
print(f"An error occurred: {str(e)}")
if __name__ == "__main__":
if len(sys.argv) != 2:
print("Usage: python tts_app.py <file_path>")
else:
read_file_aloud(sys.argv[1])
To use this application, save it as tts_app.py
and run it from the command line:
python tts_app.py path/to/your/text/file.txt
Advanced TTS Techniques
As you become more comfortable with basic TTS implementation, you can explore more advanced techniques:
1. Using Cloud-based TTS Services
Cloud services like Google Cloud Text-to-Speech, Amazon Polly, or Microsoft Azure Speech Service offer high-quality, natural-sounding voices and support multiple languages.
Here’s an example using Google Cloud Text-to-Speech:
from google.cloud import texttospeech
def google_cloud_tts(text, output_file):
client = texttospeech.TextToSpeechClient()
synthesis_input = texttospeech.SynthesisInput(text=text)
voice = texttospeech.VoiceSelectionParams(
language_code="en-US",
name="en-US-Wavenet-D"
)
audio_config = texttospeech.AudioConfig(
audio_encoding=texttospeech.AudioEncoding.MP3
)
response = client.synthesize_speech(
input=synthesis_input, voice=voice, audio_config=audio_config
)
with open(output_file, "wb") as out:
out.write(response.audio_content)
# Example usage
google_cloud_tts("Welcome to advanced text-to-speech with Google Cloud.", "output.mp3")
2. Implementing SSML (Speech Synthesis Markup Language)
SSML allows for fine-grained control over speech synthesis, including pronunciation, pitch, rate, and more.
from google.cloud import texttospeech
def tts_with_ssml(ssml_text, output_file):
client = texttospeech.TextToSpeechClient()
synthesis_input = texttospeech.SynthesisInput(ssml=ssml_text)
voice = texttospeech.VoiceSelectionParams(
language_code="en-US",
name="en-US-Wavenet-D"
)
audio_config = texttospeech.AudioConfig(
audio_encoding=texttospeech.AudioEncoding.MP3
)
response = client.synthesize_speech(
input=synthesis_input, voice=voice, audio_config=audio_config
)
with open(output_file, "wb") as out:
out.write(response.audio_content)
# Example usage
ssml_text = '''
<speak>
Here's a number spoken as a cardinal number:
<say-as interpret-as="cardinal">12345</say-as>
Now here's the same number spoken as digits:
<say-as interpret-as="digits">12345</say-as>
</speak>
'''
tts_with_ssml(ssml_text, "ssml_output.mp3")
3. Implementing Neural TTS
For those interested in cutting-edge TTS technology, you can explore neural network-based TTS models like Tacotron or FastSpeech. These models can produce highly natural speech but require more computational resources and expertise to implement.
Challenges and Considerations in TTS Development
While developing TTS applications, you may encounter several challenges:
- Language and Accent Support: Ensuring your TTS system supports multiple languages and regional accents can be complex.
- Pronunciation of Proper Nouns: Names, places, and technical terms may require custom pronunciation dictionaries.
- Emotional and Prosodic Expression: Conveying emotions and appropriate intonation in synthesized speech remains a challenge.
- Real-time Performance: Balancing speech quality with processing speed for real-time applications can be tricky.
- Voice Customization: Creating custom voices or cloning specific voices requires extensive data and sophisticated models.
Future Trends in Speech Synthesis and TTS
The field of speech synthesis and TTS is rapidly evolving. Here are some exciting trends to watch:
- Emotional TTS: Development of systems that can convey a wide range of emotions in synthesized speech.
- Multilingual and Code-switching TTS: Systems that can seamlessly switch between languages or dialects within the same utterance.
- Voice Cloning: Advancements in creating synthetic voices that mimic specific individuals with minimal training data.
- Real-time Voice Conversion: Technology that can transform a speaker’s voice into another voice in real-time.
- Integration with AR/VR: TTS systems optimized for augmented and virtual reality experiences.
Conclusion
Speech synthesis and text-to-speech technologies have come a long way, offering developers powerful tools to create applications that can read text aloud. From basic implementations using libraries like pyttsx3 to advanced cloud-based services and neural TTS models, the possibilities are vast.
As you explore this fascinating field, remember that the key to success lies in understanding your specific use case, choosing the right technology, and continuously refining your implementation based on user feedback. Whether you’re building accessibility tools, enhancing educational applications, or creating the next generation of voice assistants, mastering TTS can open up a world of opportunities in software development.
So, dive in, experiment with different TTS techniques, and let your applications find their voice. The future of human-computer interaction is speaking to us, and it’s time for your code to join the conversation!