Mastering Data Mining Techniques: Unlocking the Power of Large Datasets
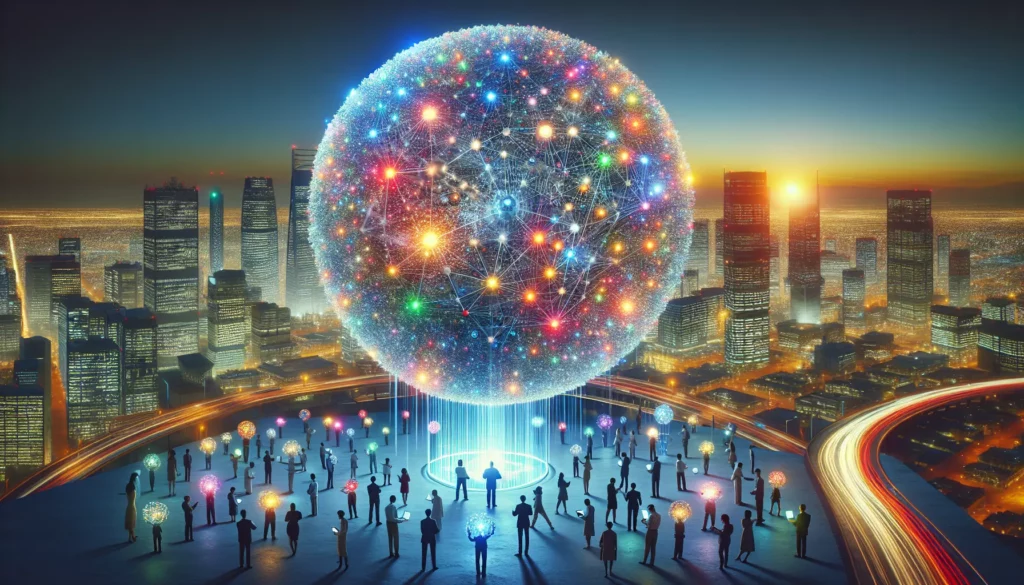
In today’s digital age, data has become one of the most valuable assets for businesses and organizations. With the exponential growth of information, the ability to extract meaningful insights from large datasets has become crucial. This is where data mining techniques come into play. In this comprehensive guide, we’ll explore the world of data mining, its importance in the field of coding education and programming skills development, and how it relates to platforms like AlgoCademy.
What is Data Mining?
Data mining is the process of discovering patterns, correlations, and insights from large datasets. It involves using various statistical and machine learning techniques to extract valuable information that can be used for decision-making, prediction, and problem-solving. Data mining is an interdisciplinary field that combines elements of computer science, statistics, and domain expertise.
The Importance of Data Mining in Coding Education
As platforms like AlgoCademy focus on providing interactive coding tutorials and resources for learners, data mining plays a crucial role in enhancing the learning experience and improving educational outcomes. Here are some ways data mining techniques can be applied in coding education:
- Personalized Learning Paths: By analyzing user data, educational platforms can create tailored learning experiences for individual students, recommending appropriate courses and exercises based on their skill level and learning style.
- Performance Prediction: Data mining can help identify patterns in student performance, allowing educators to predict which students may struggle with certain concepts and provide targeted support.
- Content Optimization: By analyzing user engagement data, platforms can optimize their content to make it more effective and engaging for learners.
- Skill Gap Analysis: Data mining techniques can be used to identify skill gaps in the job market, helping educational platforms align their curriculum with industry demands.
Key Data Mining Techniques
Let’s explore some of the most important data mining techniques that are widely used in various applications, including coding education platforms:
1. Classification
Classification is a supervised learning technique used to categorize data into predefined classes or categories. In the context of coding education, classification can be used to:
- Categorize learners based on their skill level (e.g., beginner, intermediate, advanced)
- Predict whether a student will successfully complete a course
- Classify coding problems by difficulty level
Example of a simple classification algorithm in Python using scikit-learn:
from sklearn.tree import DecisionTreeClassifier
from sklearn.model_selection import train_test_split
from sklearn.metrics import accuracy_score
# Assume X is our feature set and y is our target variable
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
clf = DecisionTreeClassifier()
clf.fit(X_train, y_train)
y_pred = clf.predict(X_test)
accuracy = accuracy_score(y_test, y_pred)
print(f"Accuracy: {accuracy}")
2. Clustering
Clustering is an unsupervised learning technique used to group similar data points together. In coding education, clustering can be applied to:
- Group learners with similar learning patterns or preferences
- Identify common mistakes or misconceptions among students
- Organize coding problems into related topics or concepts
Example of K-means clustering in Python:
from sklearn.cluster import KMeans
import numpy as np
# Assume X is our dataset
kmeans = KMeans(n_clusters=3, random_state=42)
kmeans.fit(X)
# Get cluster labels and centroids
labels = kmeans.labels_
centroids = kmeans.cluster_centers_
print("Cluster labels:", labels)
print("Centroids:", centroids)
3. Association Rule Mining
Association rule mining is used to discover interesting relationships or patterns in large datasets. In the context of coding education, it can be used to:
- Identify which coding concepts are often learned together
- Suggest related courses or topics based on a learner’s interests
- Discover common coding patterns or best practices
Example of association rule mining using the apyori library in Python:
from apyori import apriori
# Assume transactions is a list of lists containing items
rules = list(apriori(transactions, min_support=0.003, min_confidence=0.2, min_lift=3, min_length=2))
# Print the rules
for rule in rules:
print(rule)
4. Regression Analysis
Regression analysis is used to predict continuous numerical values based on input features. In coding education, regression can be applied to:
- Predict the time a student might take to complete a coding challenge
- Estimate a learner’s progress over time
- Forecast the number of users who might enroll in a specific course
Example of linear regression using scikit-learn:
from sklearn.linear_model import LinearRegression
from sklearn.model_selection import train_test_split
from sklearn.metrics import mean_squared_error, r2_score
# Assume X is our feature set and y is our target variable
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
reg = LinearRegression()
reg.fit(X_train, y_train)
y_pred = reg.predict(X_test)
mse = mean_squared_error(y_test, y_pred)
r2 = r2_score(y_test, y_pred)
print(f"Mean squared error: {mse}")
print(f"R-squared score: {r2}")
5. Anomaly Detection
Anomaly detection is used to identify unusual patterns or outliers in data. In coding education platforms, it can be used to:
- Detect potential cheating or plagiarism in coding assignments
- Identify students who may be struggling or excelling in their learning journey
- Spot unusual patterns in user behavior that might indicate technical issues
Example of anomaly detection using the Isolation Forest algorithm:
from sklearn.ensemble import IsolationForest
# Assume X is our dataset
clf = IsolationForest(contamination=0.1, random_state=42)
clf.fit(X)
# Predict anomalies (-1 for anomalies, 1 for normal points)
predictions = clf.predict(X)
# Get anomaly scores
anomaly_scores = clf.decision_function(X)
print("Predictions:", predictions)
print("Anomaly scores:", anomaly_scores)
Data Mining Process
The data mining process typically involves the following steps:
- Data Collection: Gather relevant data from various sources, such as user interactions, quiz results, and course completion rates.
- Data Cleaning and Preprocessing: Remove inconsistencies, handle missing values, and transform data into a suitable format for analysis.
- Exploratory Data Analysis: Perform initial data exploration to understand the characteristics and distributions of the dataset.
- Feature Selection and Engineering: Choose relevant features and create new ones to improve the performance of data mining algorithms.
- Model Selection and Training: Choose appropriate data mining techniques and train models on the prepared data.
- Model Evaluation: Assess the performance of the models using various metrics and validation techniques.
- Interpretation and Visualization: Analyze the results and create meaningful visualizations to communicate insights.
- Deployment and Monitoring: Implement the data mining solution in a production environment and continuously monitor its performance.
Challenges in Data Mining for Coding Education
While data mining offers numerous benefits for coding education platforms like AlgoCademy, there are several challenges to consider:
- Data Privacy and Security: Ensuring the protection of user data and compliance with privacy regulations is crucial.
- Data Quality: Maintaining high-quality, consistent data across various sources can be challenging.
- Scalability: As the user base grows, data mining techniques need to scale efficiently to handle large volumes of data.
- Interpretability: Ensuring that the insights derived from data mining are interpretable and actionable for educators and platform developers.
- Bias and Fairness: Addressing potential biases in data and algorithms to ensure fair and equitable learning experiences for all users.
Advanced Data Mining Techniques for Coding Education
As coding education platforms evolve, more advanced data mining techniques are being employed to enhance the learning experience:
1. Natural Language Processing (NLP)
NLP techniques can be used to analyze code submissions, comments, and forum discussions to gain insights into learners’ understanding and common misconceptions. For example:
from sklearn.feature_extraction.text import TfidfVectorizer
from sklearn.cluster import KMeans
# Assume code_submissions is a list of code strings
vectorizer = TfidfVectorizer()
X = vectorizer.fit_transform(code_submissions)
kmeans = KMeans(n_clusters=5, random_state=42)
kmeans.fit(X)
# Analyze cluster centers to identify common patterns or mistakes
for i, center in enumerate(kmeans.cluster_centers_):
top_words = [word for word, _ in sorted(zip(vectorizer.get_feature_names(), center), key=lambda x: x[1], reverse=True)[:10]]
print(f"Cluster {i}: {top_words}")
2. Deep Learning for Code Analysis
Deep learning models, such as recurrent neural networks (RNNs) or transformers, can be used to analyze code structure and predict potential bugs or suggest improvements. Here’s a simple example using a basic RNN for code classification:
import tensorflow as tf
from tensorflow.keras.preprocessing.text import Tokenizer
from tensorflow.keras.preprocessing.sequence import pad_sequences
# Assume code_samples is a list of code strings and labels is a list of corresponding labels
tokenizer = Tokenizer()
tokenizer.fit_on_texts(code_samples)
X = tokenizer.texts_to_sequences(code_samples)
X = pad_sequences(X, maxlen=100)
model = tf.keras.Sequential([
tf.keras.layers.Embedding(input_dim=len(tokenizer.word_index) + 1, output_dim=64, input_length=100),
tf.keras.layers.LSTM(64),
tf.keras.layers.Dense(1, activation='sigmoid')
])
model.compile(optimizer='adam', loss='binary_crossentropy', metrics=['accuracy'])
model.fit(X, labels, epochs=10, validation_split=0.2)
3. Reinforcement Learning for Adaptive Learning Paths
Reinforcement learning algorithms can be used to create adaptive learning paths that optimize for each student’s individual needs and goals. Here’s a conceptual example using Q-learning:
import numpy as np
# Assume we have a set of states (topics) and actions (next topics to learn)
n_states = 10
n_actions = 5
# Initialize Q-table
Q = np.zeros((n_states, n_actions))
# Q-learning parameters
alpha = 0.1
gamma = 0.9
epsilon = 0.1
def choose_action(state):
if np.random.uniform(0, 1) < epsilon:
return np.random.choice(n_actions)
else:
return np.argmax(Q[state, :])
def update_q_table(state, action, reward, next_state):
Q[state, action] += alpha * (reward + gamma * np.max(Q[next_state, :]) - Q[state, action])
# Training loop (simplified)
for episode in range(1000):
state = 0 # Start state
while state != n_states - 1: # Until reaching the final state
action = choose_action(state)
next_state = min(state + action + 1, n_states - 1) # Simplified transition
reward = 1 if next_state == n_states - 1 else 0 # Reward for reaching the goal
update_q_table(state, action, reward, next_state)
state = next_state
print("Optimal learning path:")
state = 0
while state != n_states - 1:
action = np.argmax(Q[state, :])
print(f"State {state} -> Action {action}")
state = min(state + action + 1, n_states - 1)
Integrating Data Mining into AlgoCademy-like Platforms
To leverage the power of data mining in coding education platforms like AlgoCademy, consider the following strategies:
- Real-time Analytics: Implement streaming data processing to analyze user interactions in real-time, allowing for immediate personalization and intervention.
- A/B Testing: Use data mining techniques to design and analyze A/B tests for new features or content, ensuring continuous improvement of the platform.
- Collaborative Filtering: Implement recommendation systems based on user behavior and preferences to suggest relevant coding challenges, courses, or resources.
- Predictive Maintenance: Use anomaly detection and predictive modeling to anticipate and prevent technical issues or performance bottlenecks in the platform.
- Sentiment Analysis: Apply NLP techniques to analyze user feedback, comments, and reviews to gauge user satisfaction and identify areas for improvement.
Ethical Considerations in Data Mining for Coding Education
As we harness the power of data mining in coding education, it’s crucial to consider the ethical implications:
- Data Privacy: Implement robust data protection measures and obtain informed consent from users for data collection and analysis.
- Algorithmic Fairness: Regularly audit data mining algorithms for potential biases and ensure equitable treatment of all users.
- Transparency: Provide clear explanations of how data is used and how algorithmic decisions are made that affect users’ learning experiences.
- User Empowerment: Give users control over their data and the ability to opt-out of certain data collection or analysis practices.
- Responsible Use: Ensure that data mining insights are used to enhance the learning experience and not for manipulative or exploitative purposes.
Conclusion
Data mining techniques offer immense potential for enhancing coding education platforms like AlgoCademy. By leveraging these powerful tools, we can create more personalized, effective, and engaging learning experiences for aspiring programmers. From predicting student performance to optimizing content delivery, data mining enables us to unlock valuable insights from the vast amounts of data generated in online learning environments.
As we continue to advance in this field, it’s crucial to balance the benefits of data mining with ethical considerations and user privacy. By doing so, we can harness the full potential of data-driven education while maintaining trust and transparency with our learners.
The future of coding education lies in the intelligent application of data mining techniques, creating adaptive, responsive, and highly effective learning platforms that cater to the diverse needs of students worldwide. As technology evolves, so too will our ability to extract meaningful insights from data, continually improving the way we teach and learn programming skills.