Understanding Software Configuration Management: Tracking and Controlling Changes with SVN and Git
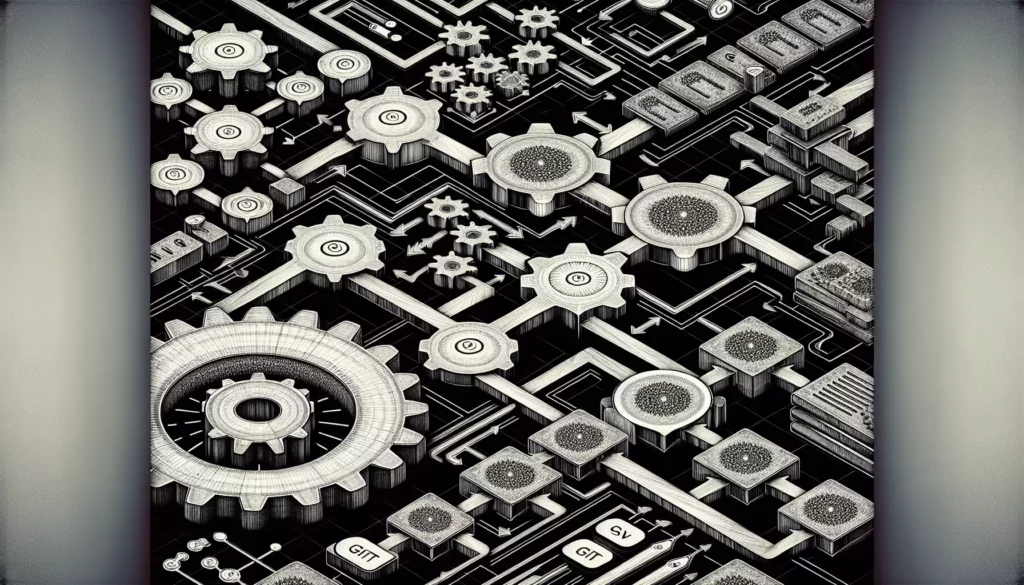
In the ever-evolving world of software development, managing changes and maintaining control over different versions of your codebase is crucial. This is where Software Configuration Management (SCM) comes into play. As aspiring developers or seasoned professionals looking to enhance their skills, understanding SCM and mastering tools like Subversion (SVN) and Git is essential. In this comprehensive guide, we’ll delve deep into the world of Software Configuration Management, exploring its importance, key concepts, and how to effectively use SVN and Git to track and control changes in your software projects.
What is Software Configuration Management?
Software Configuration Management (SCM) is a set of processes, tools, and techniques used to manage, organize, and control changes in software development projects. It encompasses version control, build management, environment configuration, change control, release management, and deployment. The primary goal of SCM is to maintain the integrity and traceability of software throughout its lifecycle, from development to deployment and maintenance.
Key aspects of SCM include:
- Version Control: Tracking and managing different versions of source code and related files
- Change Management: Controlling and documenting changes to the software
- Build Management: Automating the process of compiling source code into executable programs
- Release Management: Planning, scheduling, and controlling the release of software versions
- Environment Configuration: Managing the setup and configuration of development, testing, and production environments
- Deployment: Automating and managing the process of deploying software to various environments
The Importance of Software Configuration Management
Implementing effective SCM practices offers numerous benefits to software development teams and organizations:
- Improved Collaboration: SCM tools facilitate team collaboration by allowing multiple developers to work on the same project simultaneously without conflicts.
- Version Control: Maintaining a history of changes makes it easy to revert to previous versions if needed and track the evolution of the software.
- Traceability: SCM provides a clear audit trail of who made what changes and when, enhancing accountability and helping in debugging.
- Consistency: Ensures that all team members are working with the latest version of the code and reduces integration issues.
- Time and Cost Savings: Automating build and deployment processes saves time and reduces the likelihood of human errors.
- Quality Assurance: SCM practices help maintain code quality by enabling code reviews, automated testing, and consistent build processes.
- Compliance: For regulated industries, SCM helps in meeting compliance requirements by providing documentation and traceability.
Version Control Systems: SVN and Git
At the heart of Software Configuration Management lie Version Control Systems (VCS). Two popular VCS tools widely used in the industry are Subversion (SVN) and Git. Let’s explore each of these in detail.
Subversion (SVN)
Subversion, often abbreviated as SVN, is a centralized version control system developed by Apache Software Foundation. It has been a popular choice for many years, especially in enterprise environments.
Key Features of SVN:
- Centralized Repository: All project files are stored in a single, central repository.
- Directory Versioning: SVN versions entire directory structures, not just individual files.
- Atomic Commits: Changes are committed as a single unit, ensuring data integrity.
- Branching and Tagging: Supports creating branches for parallel development and tags for marking specific points in history.
- Partial Checkouts: Allows checking out only a portion of the repository, saving time and disk space.
Basic SVN Commands:
svn checkout URL # Check out a working copy from the repository
svn update # Update your working copy with changes from the repository
svn add FILE # Add a new file to version control
svn commit -m "Message" # Commit changes to the repository
svn log # View the commit history
svn diff # Show the differences between your working copy and the repository
svn merge URL # Merge changes from another branch
Git
Git is a distributed version control system created by Linus Torvalds. It has gained immense popularity due to its speed, flexibility, and robust branching and merging capabilities.
Key Features of Git:
- Distributed System: Each developer has a full copy of the repository, including its history.
- Branching and Merging: Git excels at creating and merging branches, making it ideal for feature-based development workflows.
- Speed: Git operations are generally faster than SVN due to its distributed nature.
- Data Integrity: Git uses SHA-1 hashes to ensure the integrity of the codebase.
- Staging Area: Provides an intermediate stage between the working directory and the repository, allowing for more granular commits.
Basic Git Commands:
git init # Initialize a new Git repository
git clone URL # Clone a repository from a remote source
git add FILE # Add changes to the staging area
git commit -m "Message" # Commit changes to the local repository
git push # Push local commits to a remote repository
git pull # Fetch and merge changes from a remote repository
git branch # List, create, or delete branches
git merge BRANCH # Merge changes from another branch
git log # View commit history
git diff # Show differences between commits, branches, etc.
Choosing Between SVN and Git
When deciding between SVN and Git for your project, consider the following factors:
Use SVN if:
- You prefer a centralized workflow with a single source of truth.
- Your project involves large binary files or extensive documentation.
- You need fine-grained access control over different parts of the repository.
- Your team is more comfortable with a linear history and simpler branching model.
Use Git if:
- You want a distributed system that allows offline work and local commits.
- Your project requires frequent branching and merging.
- You need a system that’s fast and efficient for large codebases.
- You want to leverage the extensive ecosystem of Git-based tools and hosting platforms (e.g., GitHub, GitLab).
Best Practices for Software Configuration Management
Regardless of the version control system you choose, following these best practices will help you make the most of your SCM processes:
-
Commit Often, Commit Early
Make small, frequent commits rather than large, infrequent ones. This makes it easier to track changes, revert if necessary, and understand the evolution of your codebase.
-
Write Meaningful Commit Messages
Use clear, concise commit messages that describe what changes were made and why. This helps team members understand the purpose of each commit and makes it easier to navigate the project history.
-
Use Branches Effectively
Create branches for new features, bug fixes, or experiments. This allows parallel development without affecting the main codebase and facilitates code reviews before merging.
-
Implement a Branching Strategy
Adopt a branching strategy that suits your team’s workflow, such as GitFlow, GitHub Flow, or Trunk-Based Development. This ensures consistency and helps manage releases effectively.
-
Regularly Merge or Rebase
Keep your branches up-to-date with the main branch by regularly merging or rebasing. This reduces the likelihood of conflicts and makes integration easier.
-
Use Tags for Releases
Tag specific commits to mark release points. This makes it easy to identify and revert to specific versions of your software.
-
Automate Your Build and Deployment Processes
Implement Continuous Integration/Continuous Deployment (CI/CD) pipelines to automate building, testing, and deploying your software. This ensures consistency and reduces human error.
-
Implement Code Reviews
Use pull requests or merge requests to facilitate code reviews before merging changes into the main branch. This improves code quality and knowledge sharing within the team.
-
Document Your SCM Processes
Create and maintain documentation for your SCM workflows, branching strategies, and best practices. This helps onboard new team members and ensures consistency across the team.
-
Backup Your Repository
Regularly backup your repository to prevent data loss. For distributed systems like Git, ensure multiple team members have a full clone of the repository.
Advanced SCM Concepts and Tools
As you become more proficient with basic SCM practices, consider exploring these advanced concepts and tools to further enhance your software development workflow:
1. Git Hooks
Git hooks are scripts that run automatically before or after specific Git commands. They can be used to enforce coding standards, run tests, or trigger notifications. For example:
#!/bin/sh
# pre-commit hook to run linter
npm run lint
# If linting fails, prevent the commit
if [ $? -ne 0 ]; then
echo "Linting failed. Please fix the errors before committing."
exit 1
fi
2. Git Large File Storage (LFS)
Git LFS is an extension that improves handling of large files in Git repositories. It replaces large files with text pointers in Git, while storing the file contents on a remote server.
git lfs install
git lfs track "*.psd"
git add .gitattributes
3. Semantic Versioning
Adopt semantic versioning (SemVer) for your releases. This versioning scheme uses a three-part version number (MAJOR.MINOR.PATCH) to convey meaning about the underlying changes.
4. Merge Strategies
Understand different merge strategies in Git, such as fast-forward, recursive, and octopus merges. Choose the appropriate strategy based on your branching model and project needs.
5. Rebase vs. Merge
Learn when to use rebase instead of merge. Rebasing can create a cleaner, more linear project history, but it rewrites commit history and should be used cautiously on shared branches.
git checkout feature-branch
git rebase main
6. Submodules and Subtrees
For projects that depend on external codebases, explore Git submodules or subtrees. These allow you to include other repositories within your main repository.
git submodule add https://github.com/example/repo.git externals/repo
7. GitOps
Explore GitOps principles, where the entire system state is version controlled and automated deployments are triggered by changes to the repository.
8. Monorepo vs. Multirepo
Understand the trade-offs between using a monorepo (single repository for multiple projects) and multirepo (separate repositories for each project) approach.
Integrating SCM with Development Workflows
To fully leverage the power of Software Configuration Management, it’s crucial to integrate SCM practices into your overall development workflow. Here are some strategies to achieve this:
1. Implement Trunk-Based Development
Trunk-Based Development is a source-control branching model where developers collaborate on code in a single branch called “trunk,” resisting any pressure to create other long-lived development branches. It’s designed to support continuous integration and continuous delivery.
2. Utilize Feature Flags
Feature flags (or feature toggles) allow you to modify system behavior without changing code. They complement SCM by allowing you to deploy features that are not yet ready for all users.
if (featureFlags.isEnabled("new-feature")) {
// New feature code
} else {
// Old feature code
}
3. Implement Continuous Integration (CI)
Set up CI pipelines that automatically build and test your code whenever changes are pushed to the repository. This helps catch integration issues early and ensures that the main branch is always in a releasable state.
4. Adopt Continuous Deployment (CD)
Extend your CI pipeline to automatically deploy changes to production or staging environments when tests pass. This reduces the time between writing code and getting it into the hands of users.
5. Use Infrastructure as Code (IaC)
Version control your infrastructure configurations using tools like Terraform or AWS CloudFormation. This allows you to track changes to your infrastructure alongside your application code.
resource "aws_instance" "example" {
ami = "ami-0c55b159cbfafe1f0"
instance_type = "t2.micro"
tags = {
Name = "example-instance"
}
}
6. Implement Database Schema Version Control
Use tools like Flyway or Liquibase to version control your database schemas. This ensures that database changes are tracked and can be rolled back if necessary.
7. Adopt Code Review Practices
Implement a code review process using pull requests or merge requests. This improves code quality, facilitates knowledge sharing, and helps catch issues before they make it into the main branch.
8. Use Issue Tracking Integration
Integrate your version control system with your issue tracking system (e.g., Jira, GitHub Issues). This allows you to link commits and branches to specific issues or user stories.
Conclusion
Software Configuration Management is a critical aspect of modern software development. By mastering tools like SVN and Git and implementing best practices, you can significantly improve your team’s productivity, code quality, and ability to manage complex projects. As you continue to grow as a developer, remember that effective SCM is not just about using the right tools, but also about fostering a culture of collaboration, continuous improvement, and attention to detail.
Whether you’re preparing for technical interviews at top tech companies or working on personal projects, a solid understanding of SCM principles and practices will serve you well. It demonstrates your ability to work effectively in team environments, manage complex codebases, and contribute to large-scale software projects – all valuable skills in today’s competitive tech industry.
As you apply these concepts in your coding journey, don’t hesitate to experiment with different workflows and tools. The field of software development is constantly evolving, and staying adaptable and curious will help you stay at the forefront of best practices in Software Configuration Management.