Game Theory and Algorithms: Mastering Strategic Decision-Making in Computer Science
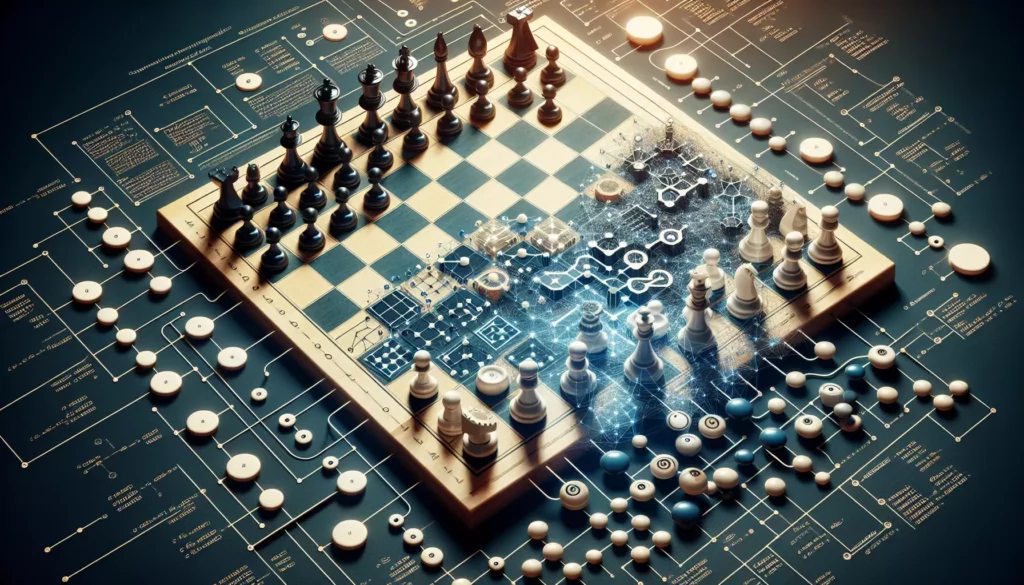
In the ever-evolving landscape of computer science and programming, understanding game theory and its application to algorithms is becoming increasingly crucial. This intersection of mathematics, economics, and computer science provides powerful tools for modeling complex interactions and decision-making processes. As we delve into this fascinating topic, we’ll explore how game theory principles can be applied to algorithmic design and problem-solving, ultimately enhancing your coding skills and preparing you for technical interviews at top tech companies.
What is Game Theory?
Game theory is a branch of mathematics that studies strategic decision-making in situations where multiple parties (or players) interact. It provides a framework for analyzing how rational individuals make choices when their decisions affect each other’s outcomes. Originally developed in economics, game theory has found applications in various fields, including computer science, biology, political science, and artificial intelligence.
At its core, game theory examines:
- Players: The decision-makers in a given scenario
- Strategies: The possible actions each player can take
- Payoffs: The outcomes or rewards for each combination of strategies
- Information: What each player knows about the game and other players
By modeling these elements, game theory helps predict behavior and identify optimal strategies in competitive or cooperative situations.
Key Concepts in Game Theory
Before we dive into the algorithmic applications of game theory, let’s review some fundamental concepts:
1. Nash Equilibrium
Named after mathematician John Nash, a Nash Equilibrium is a state in which no player can unilaterally improve their outcome by changing their strategy, assuming other players maintain their current strategies. This concept is crucial for understanding stable states in multi-agent systems.
2. Dominant Strategies
A dominant strategy is one that provides the best outcome for a player, regardless of what other players do. Identifying dominant strategies can simplify decision-making in complex scenarios.
3. Prisoner’s Dilemma
This classic game theory problem illustrates how individual rationality can lead to suboptimal outcomes for all players. It’s a powerful tool for understanding cooperation and competition in various contexts.
4. Zero-Sum Games
In zero-sum games, one player’s gain is exactly balanced by the other player’s loss. Many competitive situations in computer science, such as adversarial search algorithms, can be modeled as zero-sum games.
Game Theory in Algorithmic Design
Now that we’ve covered the basics of game theory, let’s explore how these concepts can be applied to algorithmic design and problem-solving in computer science.
1. Minimax Algorithm
The Minimax algorithm is a classic example of game theory applied to artificial intelligence and decision-making in two-player, zero-sum games. It’s commonly used in games like chess, tic-tac-toe, and Go.
The algorithm works by recursively evaluating all possible moves and their outcomes, assuming that both players play optimally. Here’s a simplified implementation of the Minimax algorithm in Python:
def minimax(board, depth, is_maximizing):
if game_over(board) or depth == 0:
return evaluate(board)
if is_maximizing:
best_score = float('-inf')
for move in get_possible_moves(board):
score = minimax(make_move(board, move), depth - 1, False)
best_score = max(score, best_score)
return best_score
else:
best_score = float('inf')
for move in get_possible_moves(board):
score = minimax(make_move(board, move), depth - 1, True)
best_score = min(score, best_score)
return best_score
This algorithm demonstrates how game theory principles can be translated into code to create intelligent decision-making systems.
2. Alpha-Beta Pruning
Alpha-Beta pruning is an optimization technique for the Minimax algorithm. It significantly reduces the number of nodes evaluated in the search tree by eliminating branches that cannot possibly influence the final decision.
Here’s an example of how Alpha-Beta pruning can be implemented:
def alpha_beta(board, depth, alpha, beta, is_maximizing):
if game_over(board) or depth == 0:
return evaluate(board)
if is_maximizing:
best_score = float('-inf')
for move in get_possible_moves(board):
score = alpha_beta(make_move(board, move), depth - 1, alpha, beta, False)
best_score = max(score, best_score)
alpha = max(alpha, best_score)
if beta <= alpha:
break
return best_score
else:
best_score = float('inf')
for move in get_possible_moves(board):
score = alpha_beta(make_move(board, move), depth - 1, alpha, beta, True)
best_score = min(score, best_score)
beta = min(beta, best_score)
if beta <= alpha:
break
return best_score
This optimization showcases how understanding game theory can lead to more efficient algorithms in practice.
3. Nash Equilibrium in Load Balancing
The concept of Nash Equilibrium can be applied to distributed systems and load balancing algorithms. In a network of servers, each server can be considered a player trying to optimize its resource allocation. The Nash Equilibrium represents a state where no server can improve its performance by unilaterally changing its strategy.
Here’s a simplified example of how Nash Equilibrium concepts can be applied to load balancing:
class Server:
def __init__(self, capacity):
self.capacity = capacity
self.load = 0
def add_load(self, amount):
self.load += amount
def remove_load(self, amount):
self.load -= amount
def get_utilization(self):
return self.load / self.capacity
def balance_load(servers, total_load):
while True:
max_util_server = max(servers, key=lambda s: s.get_utilization())
min_util_server = min(servers, key=lambda s: s.get_utilization())
if max_util_server.get_utilization() - min_util_server.get_utilization() < 0.1:
break
load_to_transfer = (max_util_server.load - min_util_server.load) / 2
max_util_server.remove_load(load_to_transfer)
min_util_server.add_load(load_to_transfer)
return servers
This simplified algorithm demonstrates how servers can reach a Nash Equilibrium-like state where no server can improve its utilization by transferring load to another server.
Game Theory in Algorithmic Problem-Solving
Beyond specific algorithms, game theory provides valuable insights and strategies for approaching algorithmic problem-solving in general. Here are some ways game theory can enhance your coding skills and interview preparation:
1. Strategic Thinking
Game theory encourages you to think about problems from multiple perspectives, considering how different “players” (which could be parts of your algorithm or system) interact and influence each other. This strategic thinking is invaluable when designing complex systems or optimizing algorithms for efficiency.
2. Trade-off Analysis
Many algorithmic problems involve trade-offs between different objectives, such as time complexity vs. space complexity. Game theory provides frameworks for analyzing these trade-offs and making informed decisions about which strategies to pursue.
3. Adversarial Thinking
In security-related problems or when designing robust systems, it’s crucial to think about potential adversaries or worst-case scenarios. Game theory’s focus on strategic interactions helps develop this adversarial thinking, leading to more secure and resilient algorithms.
4. Equilibrium Analysis
Understanding concepts like Nash Equilibrium can help in designing stable distributed systems, where multiple agents or components need to work together without constant centralized coordination.
Practical Applications in Tech Interviews
As you prepare for technical interviews at top tech companies, understanding game theory and its applications can give you a significant advantage. Here are some areas where game theory concepts might come into play:
1. Optimization Problems
Many interview questions involve optimizing algorithms for efficiency. Game theory concepts can help you approach these problems by considering the strategic interactions between different parts of your algorithm.
2. System Design
In system design interviews, you may need to design distributed systems or load balancing algorithms. Knowledge of game theory can help you create more robust and efficient designs.
3. AI and Machine Learning
For roles involving AI or machine learning, understanding game theory is crucial. Many AI algorithms, especially in reinforcement learning and multi-agent systems, rely heavily on game theory principles.
4. Security and Cryptography
In security-related questions, game theory can help you think about potential vulnerabilities and attack strategies, leading to more secure designs.
Implementing Game Theory Concepts in Code
To further illustrate how game theory concepts can be implemented in code, let’s look at a few more examples:
1. Implementing the Prisoner’s Dilemma
The Prisoner’s Dilemma is a classic game theory problem. Here’s a simple implementation in Python:
def prisoners_dilemma(strategy1, strategy2, rounds):
scores = {"cooperate": {"cooperate": (3, 3), "defect": (0, 5)},
"defect": {"cooperate": (5, 0), "defect": (1, 1)}}
score1, score2 = 0, 0
for _ in range(rounds):
move1 = strategy1(score1, score2)
move2 = strategy2(score2, score1)
round_score1, round_score2 = scores[move1][move2]
score1 += round_score1
score2 += round_score2
return score1, score2
def always_cooperate(my_score, opponent_score):
return "cooperate"
def always_defect(my_score, opponent_score):
return "defect"
def tit_for_tat(my_score, opponent_score):
global last_move
if "last_move" not in globals():
last_move = "cooperate"
result = last_move
last_move = "cooperate" if opponent_score > my_score else "defect"
return result
# Example usage
score1, score2 = prisoners_dilemma(always_cooperate, always_defect, 100)
print(f"Always Cooperate vs Always Defect: {score1} vs {score2}")
score1, score2 = prisoners_dilemma(tit_for_tat, always_defect, 100)
print(f"Tit for Tat vs Always Defect: {score1} vs {score2}")
This implementation allows you to experiment with different strategies and see how they perform over multiple rounds of the Prisoner’s Dilemma.
2. Implementing a Simple Auction Algorithm
Auctions are another area where game theory principles are often applied. Here’s a simple implementation of a second-price sealed-bid auction:
import random
class Bidder:
def __init__(self, name, value):
self.name = name
self.value = value
def bid(self):
# In a second-price auction, bidding true value is a dominant strategy
return self.value
def second_price_auction(bidders):
bids = [(bidder, bidder.bid()) for bidder in bidders]
winner, highest_bid = max(bids, key=lambda x: x[1])
second_highest_bid = max(bid for bidder, bid in bids if bidder != winner)
return winner, second_highest_bid
# Example usage
bidders = [
Bidder("Alice", random.uniform(0, 100)),
Bidder("Bob", random.uniform(0, 100)),
Bidder("Charlie", random.uniform(0, 100)),
Bidder("David", random.uniform(0, 100))
]
winner, price = second_price_auction(bidders)
print(f"Winner: {winner.name}")
print(f"Price: {price:.2f}")
print(f"Winner's value: {winner.value:.2f}")
This implementation demonstrates how game theory concepts can be applied to create fair and efficient auction mechanisms.
Advanced Topics in Game Theory and Algorithms
As you progress in your understanding of game theory and its applications to algorithms, there are several advanced topics you might want to explore:
1. Evolutionary Game Theory
Evolutionary game theory extends classical game theory by considering how strategies evolve over time in a population. This has applications in genetic algorithms and modeling complex adaptive systems.
2. Mechanism Design
Mechanism design is the art of designing games or economic mechanisms to achieve specific outcomes. It’s particularly relevant in designing auction systems, voting mechanisms, and resource allocation algorithms.
3. Algorithmic Game Theory
This field combines game theory with algorithm design and complexity theory. It’s concerned with designing and analyzing algorithms for strategic environments where multiple self-interested agents interact.
4. Multi-Agent Reinforcement Learning
This area combines game theory with machine learning, focusing on how multiple agents can learn optimal strategies through interaction and feedback.
Conclusion
Game theory provides a powerful framework for understanding and designing algorithms, especially in scenarios involving strategic decision-making and multi-agent interactions. By incorporating game theory principles into your algorithmic thinking, you can develop more sophisticated problem-solving skills and create more robust and efficient solutions.
As you continue your journey in coding education and prepare for technical interviews, keep these game theory concepts in mind. They can provide valuable insights and approaches to a wide range of algorithmic problems, from optimization and system design to AI and security.
Remember, the key to mastering these concepts is practice. Try implementing game theory algorithms, experiment with different strategies, and analyze how they perform in various scenarios. As you gain experience, you’ll find that the strategic thinking cultivated by game theory becomes an invaluable tool in your programming toolkit.
Whether you’re aiming for a position at a top tech company or simply want to enhance your problem-solving skills, understanding the intersection of game theory and algorithms will undoubtedly give you a competitive edge in the world of computer science and software engineering.