Multi-Platform Mobile Development: Mastering React Native and Flutter
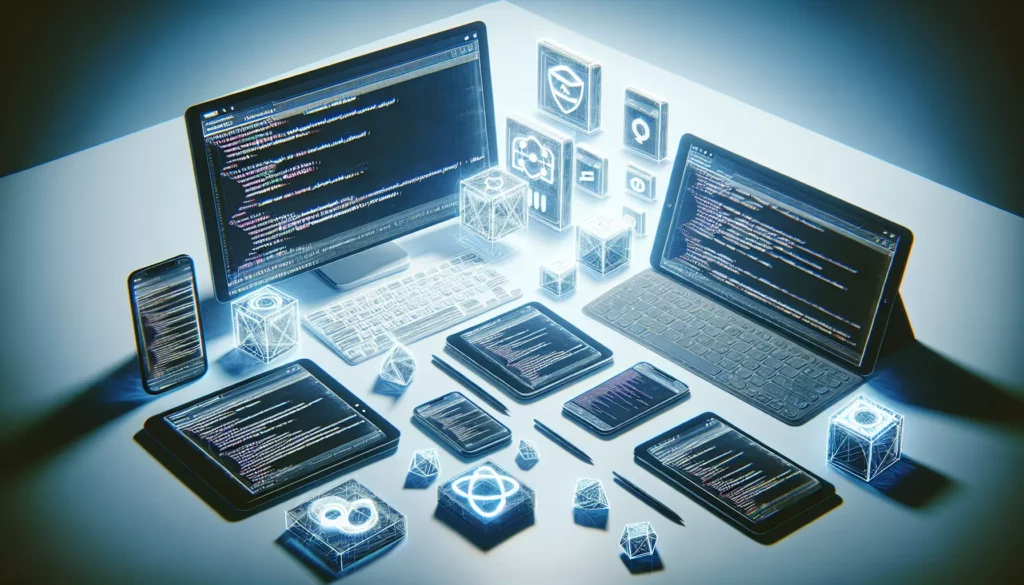
In today’s fast-paced digital world, mobile applications have become an integral part of our daily lives. As a developer, mastering multi-platform mobile development is crucial to stay competitive and efficient in the industry. This comprehensive guide will explore two powerful frameworks for cross-platform mobile development: React Native and Flutter. We’ll dive deep into their features, advantages, and how they can help you create stunning apps for both iOS and Android platforms simultaneously.
Understanding Multi-Platform Mobile Development
Before we delve into the specifics of React Native and Flutter, let’s first understand what multi-platform mobile development entails and why it’s becoming increasingly popular among developers and businesses alike.
What is Multi-Platform Mobile Development?
Multi-platform mobile development, also known as cross-platform development, is the practice of creating mobile applications that can run on multiple operating systems, primarily iOS and Android, using a single codebase. This approach allows developers to write code once and deploy it across different platforms, saving time, effort, and resources.
Benefits of Multi-Platform Development
- Cost-effective: Develop for multiple platforms with a single codebase
- Faster time-to-market: Streamlined development process
- Consistent user experience: Maintain uniformity across platforms
- Easier maintenance: Updates and bug fixes can be applied universally
- Larger audience reach: Target both iOS and Android users simultaneously
React Native: Building Native Apps with React
React Native, developed by Facebook, is an open-source framework that allows developers to build mobile applications using JavaScript and React. It’s designed to enable the development of truly native apps for both iOS and Android platforms.
Key Features of React Native
- Native Components: Uses real, native UI components
- Hot Reloading: Instant reflection of code changes
- Large Community: Extensive support and third-party libraries
- Performance: Near-native performance for most applications
- Code Reusability: Share code between web and mobile projects
Getting Started with React Native
To begin developing with React Native, you’ll need to set up your development environment. Here’s a quick guide to get you started:
- Install Node.js and npm (Node Package Manager)
- Install the React Native CLI globally:
npm install -g react-native-cli
- Create a new React Native project:
npx react-native init MyAwesomeApp
- Navigate to your project directory:
cd MyAwesomeApp
- Run your app on iOS or Android:
npx react-native run-ios
# or
npx react-native run-android
React Native Code Example
Here’s a simple example of a React Native component that displays a greeting:
import React from 'react';
import { Text, View } from 'react-native';
const Greeting = ({ name }) => {
return (
<View style={{ alignItems: 'center' }}>
<Text>Hello, {name}!</Text>
</View>
);
}
export default Greeting;
Flutter: Google’s UI Toolkit for Mobile, Web, and Desktop
Flutter, developed by Google, is an open-source UI software development kit used to create natively compiled applications for mobile, web, and desktop from a single codebase. It uses the Dart programming language and provides a rich set of pre-designed widgets.
Key Features of Flutter
- Hot Reload: Instant updates to the app as you code
- Custom Widgets: Highly customizable UI components
- Rich Animation Libraries: Create smooth, natural animations
- Performance: Compiled natively for each platform
- Single Codebase: Develop for iOS, Android, web, and desktop
Getting Started with Flutter
To begin developing with Flutter, follow these steps to set up your environment:
- Download and install the Flutter SDK
- Add Flutter to your PATH
- Install Android Studio or Visual Studio Code with Flutter and Dart plugins
- Run flutter doctor to check for any missing dependencies
- Create a new Flutter project:
flutter create my_flutter_app
- Navigate to your project directory:
cd my_flutter_app
- Run your app:
flutter run
Flutter Code Example
Here’s a simple example of a Flutter widget that displays a greeting:
import 'package:flutter/material.dart';
class Greeting extends StatelessWidget {
final String name;
const Greeting({Key? key, required this.name}) : super(key: key);
@override
Widget build(BuildContext context) {
return Center(
child: Text('Hello, $name!'),
);
}
}
React Native vs Flutter: A Comparison
While both React Native and Flutter are excellent choices for multi-platform mobile development, they have their own strengths and weaknesses. Let’s compare them across various aspects:
1. Programming Language
- React Native: Uses JavaScript, which is widely known and has a large developer community.
- Flutter: Uses Dart, a language developed by Google. It’s less common but designed specifically for UI development.
2. Performance
- React Native: Offers near-native performance through the use of native components.
- Flutter: Provides excellent performance due to its compiled nature and custom rendering engine.
3. User Interface
- React Native: Uses native UI components, resulting in a more platform-specific look and feel.
- Flutter: Uses its own widget library, allowing for more consistent UI across platforms but potentially less platform-specific design.
4. Learning Curve
- React Native: Easier for developers familiar with JavaScript and React.
- Flutter: May require learning Dart, but the framework itself is well-documented and relatively easy to pick up.
5. Community and Ecosystem
- React Native: Has a larger community and more third-party libraries available.
- Flutter: Growing rapidly, with strong support from Google and an increasing number of packages.
Best Practices for Multi-Platform Mobile Development
Regardless of which framework you choose, following these best practices will help you create high-quality, maintainable multi-platform mobile applications:
1. Plan Your Architecture
Before diving into coding, plan your application’s architecture carefully. Consider using design patterns like MVVM (Model-View-ViewModel) or Clean Architecture to separate concerns and make your code more maintainable.
2. Optimize Performance
Performance is crucial for mobile apps. Use tools like the React Native Performance Monitor or Flutter DevTools to identify and resolve performance bottlenecks.
3. Write Reusable Components
Create modular, reusable components to maximize code reuse across your application. This not only saves time but also ensures consistency in your UI.
4. Handle Platform-Specific Code Gracefully
While the goal is to share as much code as possible, there will be instances where you need platform-specific implementations. Use conditional statements or platform-specific files to handle these cases elegantly.
5. Test Thoroughly
Implement a comprehensive testing strategy, including unit tests, integration tests, and UI tests. Both React Native and Flutter provide tools for automated testing.
6. Stay Updated
Keep your framework and dependencies up to date to benefit from the latest features, performance improvements, and security patches.
Advanced Topics in Multi-Platform Development
As you become more proficient in multi-platform development, you may want to explore these advanced topics:
1. State Management
For React Native, consider using state management libraries like Redux or MobX. In Flutter, explore solutions like Provider, Riverpod, or BLoC pattern.
2. Native Module Integration
Learn how to integrate native modules when you need functionality not provided by the framework. This involves writing platform-specific code in Swift/Objective-C for iOS and Java/Kotlin for Android.
3. Continuous Integration and Deployment (CI/CD)
Set up CI/CD pipelines to automate your build, test, and deployment processes. Tools like Fastlane can be particularly useful for mobile app deployment.
4. App Store Optimization (ASO)
Learn strategies to improve your app’s visibility and ranking in the App Store and Google Play Store.
5. Analytics and Crash Reporting
Implement analytics and crash reporting tools to gather insights about your app’s usage and stability. Popular options include Firebase Analytics and Crashlytics.
Real-World Applications and Case Studies
To truly understand the power of multi-platform development, let’s look at some real-world applications built with React Native and Flutter:
React Native Success Stories
- Facebook: The social media giant uses React Native in many of its mobile apps, including the main Facebook app and Facebook Ads Manager.
- Instagram: Parts of the Instagram app are built with React Native, demonstrating its ability to scale.
- Walmart: The retail giant rebuilt its mobile app using React Native, improving performance and user experience.
Flutter Success Stories
- Google Ads: Google’s own advertising app is built with Flutter, showcasing its capabilities for complex, data-driven applications.
- Alibaba: The e-commerce platform uses Flutter in parts of its app to deliver a smooth, consistent experience across devices.
- Reflectly: This popular journaling app was rebuilt entirely in Flutter, resulting in improved performance and easier maintenance.
Future of Multi-Platform Mobile Development
The field of multi-platform mobile development is continuously evolving. Here are some trends to watch:
- Increased adoption of cross-platform frameworks by large companies
- Improvements in performance and native feature support
- Greater integration with emerging technologies like AR and VR
- Expansion beyond mobile to web and desktop platforms
Conclusion
Multi-platform mobile development with frameworks like React Native and Flutter offers an efficient and cost-effective way to build mobile applications for both iOS and Android. By mastering these technologies, you can significantly enhance your skills as a developer and create powerful, cross-platform applications.
Whether you choose React Native or Flutter depends on your specific project requirements, team expertise, and personal preferences. Both frameworks have their strengths and are capable of producing high-quality, performant applications.
As you continue your journey in multi-platform development, remember to stay curious, keep learning, and always strive to follow best practices. The world of mobile development is exciting and ever-changing, offering endless opportunities for innovation and growth.
Happy coding, and may your multi-platform development adventures be successful and rewarding!