Computer Vision: Unlocking the Power of Visual Data with OpenCV
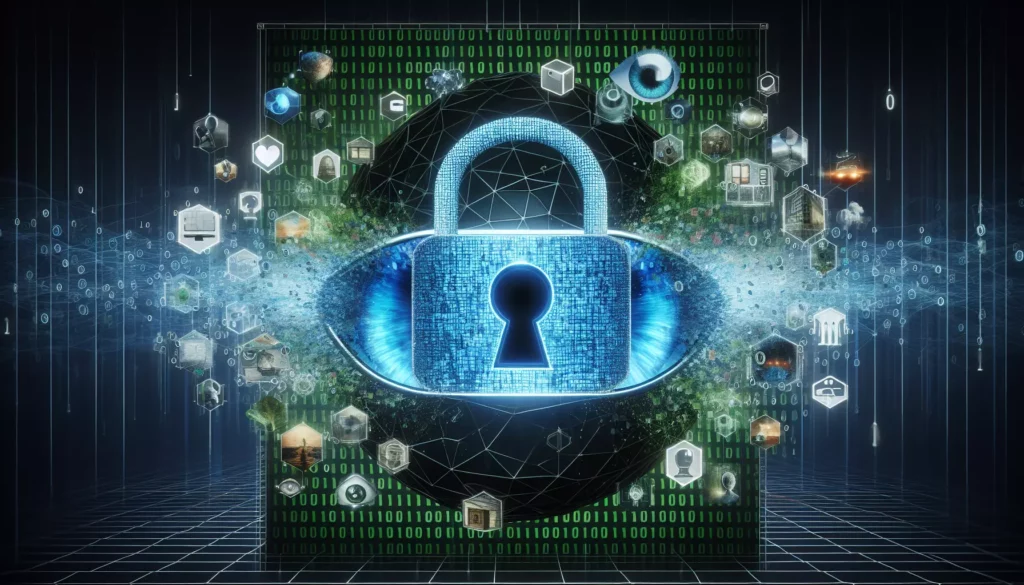
In the rapidly evolving world of technology, computer vision has emerged as a groundbreaking field that enables machines to interpret and understand visual information from the world around us. This exciting domain combines elements of artificial intelligence, machine learning, and image processing to create systems that can analyze, process, and make decisions based on visual data. One of the most powerful tools in a developer’s arsenal for working with computer vision is OpenCV (Open Source Computer Vision Library). In this comprehensive guide, we’ll explore the fascinating world of computer vision and dive deep into how you can leverage OpenCV to create intelligent applications that can see and understand the world.
Understanding Computer Vision
Before we delve into the specifics of OpenCV, it’s crucial to understand what computer vision is and why it’s such an important field in modern technology. Computer vision is a branch of artificial intelligence that focuses on training computers to interpret and understand visual information from the world, much like humans do. This involves tasks such as image recognition, object detection, facial recognition, and even complex scene understanding.
The applications of computer vision are vast and diverse, ranging from autonomous vehicles and robotics to medical imaging and augmented reality. As our world becomes increasingly digital and data-driven, the ability to process and analyze visual information automatically has become more critical than ever.
The Role of OpenCV in Computer Vision
OpenCV, short for Open Source Computer Vision Library, is a powerful and versatile library that provides a wide range of tools and algorithms for computer vision tasks. Originally developed by Intel in 1999, OpenCV has since become an open-source project supported by a vibrant community of developers and researchers worldwide.
Some key features of OpenCV include:
- Cross-platform support (Windows, Linux, macOS, Android, iOS)
- Interfaces for multiple programming languages (C++, Python, Java)
- Optimized algorithms for real-time applications
- A comprehensive set of both classic and state-of-the-art computer vision algorithms
- GPU acceleration support for improved performance
With its rich set of functionalities and excellent documentation, OpenCV has become the go-to library for developers and researchers working on computer vision projects.
Getting Started with OpenCV
To begin your journey with OpenCV, you’ll need to install the library and set up your development environment. While OpenCV supports multiple programming languages, we’ll focus on using Python in this guide, as it’s one of the most popular and beginner-friendly options.
Installing OpenCV
The easiest way to install OpenCV for Python is using pip, the Python package installer. Open your terminal or command prompt and run the following command:
pip install opencv-python
This command will install the main modules of OpenCV along with its dependencies. If you need additional modules (e.g., for contrib packages), you can install them separately:
pip install opencv-contrib-python
Verifying the Installation
To ensure that OpenCV has been installed correctly, open a Python interpreter and try importing the library:
import cv2
print(cv2.__version__)
If the installation was successful, this should print the version number of OpenCV installed on your system.
Basic Image Operations with OpenCV
Now that we have OpenCV installed, let’s explore some basic operations you can perform on images using the library.
Reading and Displaying Images
One of the most fundamental operations in computer vision is reading and displaying images. Here’s how you can do this with OpenCV:
import cv2
import matplotlib.pyplot as plt
# Read an image
image = cv2.imread('path_to_your_image.jpg')
# Convert BGR to RGB (OpenCV uses BGR by default)
image_rgb = cv2.cvtColor(image, cv2.COLOR_BGR2RGB)
# Display the image
plt.imshow(image_rgb)
plt.axis('off')
plt.show()
In this example, we use cv2.imread()
to read an image file, convert it from BGR to RGB color space (as OpenCV uses BGR by default), and then display it using matplotlib.
Image Resizing
Resizing images is a common operation in computer vision, often used for preprocessing or to fit images to a specific size requirement. Here’s how you can resize an image with OpenCV:
import cv2
# Read an image
image = cv2.imread('path_to_your_image.jpg')
# Resize the image
resized_image = cv2.resize(image, (300, 200)) # New size: 300x200 pixels
# Display the resized image
cv2.imshow('Resized Image', resized_image)
cv2.waitKey(0)
cv2.destroyAllWindows()
In this example, we use cv2.resize()
to change the dimensions of the image to 300×200 pixels. You can adjust these values based on your requirements.
Image Filtering
Filtering is a fundamental operation in image processing, used for tasks such as noise reduction, edge detection, and image enhancement. Let’s look at how to apply a Gaussian blur filter to an image:
import cv2
import numpy as np
# Read an image
image = cv2.imread('path_to_your_image.jpg')
# Apply Gaussian blur
blurred_image = cv2.GaussianBlur(image, (5, 5), 0)
# Display the original and blurred images
cv2.imshow('Original Image', image)
cv2.imshow('Blurred Image', blurred_image)
cv2.waitKey(0)
cv2.destroyAllWindows()
Here, we use cv2.GaussianBlur()
to apply a Gaussian blur to the image. The (5, 5) parameter specifies the kernel size, which determines the amount of blurring.
Advanced Computer Vision Techniques with OpenCV
While basic image operations are important, the real power of computer vision lies in more advanced techniques. Let’s explore some of these capabilities using OpenCV.
Object Detection
Object detection is a crucial task in computer vision, with applications ranging from autonomous vehicles to security systems. OpenCV provides several methods for object detection, including the popular Haar Cascade classifier. Here’s an example of how to use a pre-trained Haar Cascade classifier for face detection:
import cv2
# Load the pre-trained face detector
face_cascade = cv2.CascadeClassifier(cv2.data.haarcascades + 'haarcascade_frontalface_default.xml')
# Read an image
image = cv2.imread('path_to_your_image.jpg')
gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
# Detect faces
faces = face_cascade.detectMultiScale(gray, scaleFactor=1.1, minNeighbors=5, minSize=(30, 30))
# Draw rectangles around the faces
for (x, y, w, h) in faces:
cv2.rectangle(image, (x, y), (x+w, y+h), (255, 0, 0), 2)
# Display the result
cv2.imshow('Face Detection', image)
cv2.waitKey(0)
cv2.destroyAllWindows()
This script loads a pre-trained Haar Cascade classifier for face detection, applies it to a grayscale version of the input image, and then draws rectangles around the detected faces.
Feature Detection and Matching
Feature detection and matching are essential techniques in computer vision, used in applications like image stitching, object recognition, and augmented reality. OpenCV provides several algorithms for this purpose, including SIFT (Scale-Invariant Feature Transform) and ORB (Oriented FAST and Rotated BRIEF). Here’s an example using ORB:
import cv2
import numpy as np
# Read two images
img1 = cv2.imread('image1.jpg', cv2.IMREAD_GRAYSCALE)
img2 = cv2.imread('image2.jpg', cv2.IMREAD_GRAYSCALE)
# Initialize ORB detector
orb = cv2.ORB_create()
# Find keypoints and descriptors
kp1, des1 = orb.detectAndCompute(img1, None)
kp2, des2 = orb.detectAndCompute(img2, None)
# Create BFMatcher object
bf = cv2.BFMatcher(cv2.NORM_HAMMING, crossCheck=True)
# Match descriptors
matches = bf.match(des1, des2)
# Sort them in the order of their distance
matches = sorted(matches, key=lambda x: x.distance)
# Draw first 10 matches
img3 = cv2.drawMatches(img1, kp1, img2, kp2, matches[:10], None, flags=cv2.DrawMatchesFlags_NOT_DRAW_SINGLE_POINTS)
# Display the result
cv2.imshow('ORB Feature Matching', img3)
cv2.waitKey(0)
cv2.destroyAllWindows()
This script demonstrates how to use the ORB algorithm to detect and match features between two images. It’s particularly useful for tasks like image registration or finding similar objects across different images.
Image Segmentation
Image segmentation is the process of partitioning an image into multiple segments or objects. This technique is crucial for understanding the content of images at a deeper level. OpenCV offers various methods for image segmentation, including thresholding and watershed algorithm. Here’s an example of simple thresholding:
import cv2
import numpy as np
# Read an image in grayscale
image = cv2.imread('path_to_your_image.jpg', cv2.IMREAD_GRAYSCALE)
# Apply binary thresholding
_, thresh = cv2.threshold(image, 127, 255, cv2.THRESH_BINARY)
# Display the original and thresholded images
cv2.imshow('Original Image', image)
cv2.imshow('Thresholded Image', thresh)
cv2.waitKey(0)
cv2.destroyAllWindows()
This example demonstrates how to use simple binary thresholding to segment an image into foreground and background regions based on pixel intensity.
Real-world Applications of Computer Vision
The techniques we’ve explored so far are just the tip of the iceberg when it comes to computer vision. In real-world scenarios, these methods are combined and extended to create powerful applications across various industries. Let’s look at some examples:
Autonomous Vehicles
Computer vision plays a crucial role in the development of self-driving cars. These vehicles use a combination of cameras, LiDAR, and other sensors to perceive their environment. Computer vision algorithms process this data to detect and classify objects (like other vehicles, pedestrians, and road signs), understand traffic patterns, and make decisions about navigation and collision avoidance.
Medical Imaging
In the healthcare industry, computer vision is revolutionizing medical imaging. Machine learning models trained on large datasets of medical images can assist radiologists in detecting and diagnosing diseases. For example, convolutional neural networks (CNNs) have shown promising results in detecting cancerous tumors in mammograms and identifying retinal diseases from eye scans.
Facial Recognition
Facial recognition technology, powered by computer vision algorithms, has found applications in security systems, smartphone unlocking mechanisms, and even payment systems. These systems use techniques like feature extraction and deep learning to identify and verify individuals based on their facial features.
Augmented Reality (AR)
AR applications rely heavily on computer vision to understand the real world and seamlessly integrate virtual elements. Techniques like feature detection and tracking are used to identify surfaces and anchor points in the real world, allowing virtual objects to be placed convincingly in the user’s environment.
Challenges and Future Directions in Computer Vision
While computer vision has made significant strides in recent years, there are still many challenges to overcome and exciting directions for future research:
Robustness and Generalization
Many computer vision models perform well under controlled conditions but struggle in real-world scenarios with varying lighting, occlusions, and diverse environments. Improving the robustness and generalization capabilities of these models is an active area of research.
Ethical Considerations
As computer vision technologies become more prevalent in our daily lives, ethical concerns around privacy, bias, and misuse of these technologies are coming to the forefront. Addressing these concerns through responsible development and deployment of computer vision systems is crucial.
3D Vision
While much of computer vision focuses on 2D images, there’s growing interest in 3D vision, which aims to understand the three-dimensional structure of the world from visual data. This has applications in robotics, autonomous navigation, and virtual reality.
Integration with Other AI Technologies
The future of computer vision likely involves tighter integration with other AI technologies like natural language processing and reinforcement learning. This could lead to more sophisticated systems that can understand and interact with the world in more human-like ways.
Conclusion
Computer vision, powered by libraries like OpenCV, is transforming the way machines perceive and interact with the visual world. From basic image processing to advanced object detection and recognition, the field offers a wealth of possibilities for developers and researchers alike. As we’ve seen, the applications of computer vision span across numerous industries, from healthcare to autonomous vehicles, and its potential is far from fully realized.
As you continue your journey in computer vision, remember that the key to mastery lies in practice and experimentation. Start with the basics we’ve covered here, then gradually move on to more complex techniques and real-world applications. The field is constantly evolving, so staying updated with the latest research and tools is crucial.
Whether you’re a beginner just starting out or an experienced developer looking to expand your skills, computer vision offers an exciting and rewarding path. With libraries like OpenCV at your disposal, you have the tools to bring your visual ideas to life and contribute to the cutting edge of technology. So dive in, experiment, and let your imagination guide you in exploring the fascinating world of computer vision!