WebAssembly (WASM): Revolutionizing Browser-Based Performance with Rust and C++
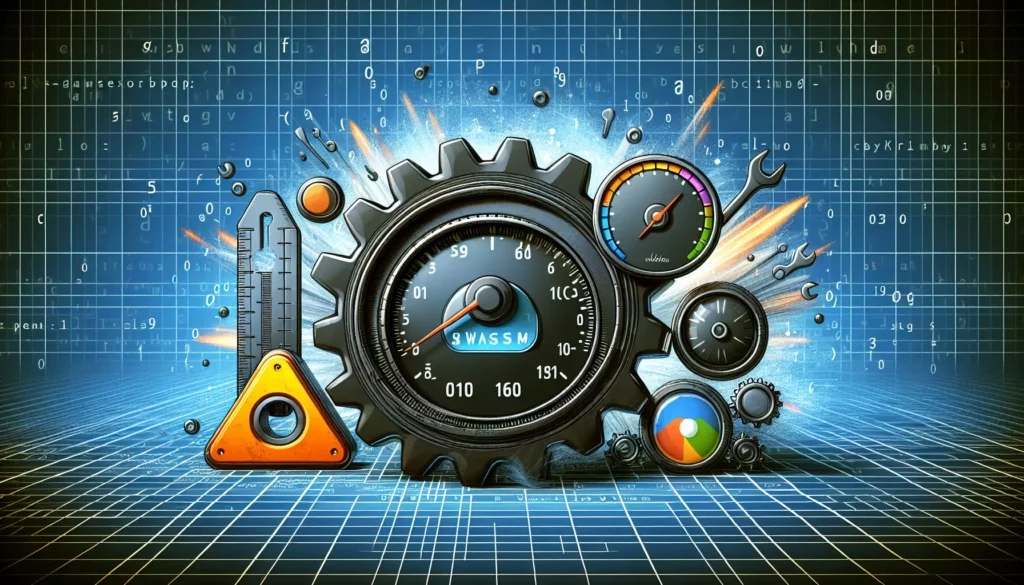
In the ever-evolving landscape of web development, performance has always been a critical factor. As web applications become increasingly complex, developers are constantly seeking ways to push the boundaries of what’s possible in the browser. Enter WebAssembly (WASM), a game-changing technology that’s revolutionizing the way we think about high-performance web applications. In this comprehensive guide, we’ll dive deep into the world of WebAssembly, exploring its potential, advantages, and how languages like Rust and C++ are leading the charge in this exciting new frontier.
What is WebAssembly?
WebAssembly, often abbreviated as WASM, is a binary instruction format designed for efficient execution in web browsers. It serves as a low-level language that can be used as a compilation target for high-level languages like C, C++, and Rust. The result is near-native performance for web applications, opening up new possibilities for complex computations and graphics-intensive tasks directly in the browser.
Key features of WebAssembly include:
- Efficiency: WASM code runs at near-native speed, significantly faster than JavaScript in many cases.
- Portability: WebAssembly is designed to run on all modern web browsers, ensuring wide compatibility.
- Safety: It operates within the same security sandbox as JavaScript, maintaining the web’s security model.
- Interoperability: WASM can work alongside JavaScript, allowing developers to use both technologies in the same application.
The Power of WebAssembly
WebAssembly’s potential to transform web development is immense. Here are some of the key advantages it brings to the table:
1. Enhanced Performance
One of the primary benefits of WebAssembly is its ability to execute code at near-native speeds. This makes it possible to run computationally intensive tasks in the browser that were previously impractical or impossible. From complex simulations to advanced graphics rendering, WASM opens up new horizons for web applications.
2. Language Flexibility
WebAssembly isn’t tied to a single programming language. It serves as a compilation target for various languages, with Rust and C++ being particularly popular choices. This flexibility allows developers to leverage their existing skills and choose the best language for their specific needs.
3. Improved User Experience
By enabling faster and more efficient code execution, WebAssembly contributes to smoother, more responsive web applications. This translates to a better user experience, particularly for complex or resource-intensive web apps.
4. Code Reuse and Legacy Integration
WebAssembly makes it possible to port existing C or C++ codebases to the web, allowing developers to reuse battle-tested libraries and algorithms without having to rewrite them in JavaScript.
WebAssembly and Rust: A Perfect Match
While WebAssembly supports multiple languages, Rust has emerged as a favorite among WASM developers. Let’s explore why Rust and WebAssembly make such a powerful combination:
1. Performance
Rust is designed for high performance, with zero-cost abstractions and fine-grained control over system resources. When compiled to WebAssembly, Rust code can achieve performance levels very close to native executables.
2. Safety
Rust’s strong type system and ownership model prevent common programming errors like null or dangling pointer dereferences, buffer overflows, and data races. This safety translates well to the web environment, where security is paramount.
3. No Garbage Collection
Unlike languages that rely on garbage collection, Rust’s memory management model aligns perfectly with WebAssembly’s lack of a built-in garbage collector. This results in predictable performance and lower memory overhead.
4. Rich Ecosystem
The Rust ecosystem has embraced WebAssembly, with excellent tooling and libraries available for WASM development. The wasm-pack
tool, for instance, simplifies the process of building and publishing Rust-generated WebAssembly modules.
Example: Rust to WebAssembly
Here’s a simple example of how you might write a Rust function and compile it to WebAssembly:
#[no_mangle]
pub extern "C" fn add(a: i32, b: i32) -> i32 {
a + b
}
This Rust function can be compiled to WebAssembly and then called from JavaScript like this:
WebAssembly.instantiateStreaming(fetch("add.wasm"))
.then(obj => {
const result = obj.instance.exports.add(5, 3);
console.log(result); // Outputs: 8
});
C++ and WebAssembly: Bringing Legacy Code to the Web
C++ is another language that pairs exceptionally well with WebAssembly. Its long history and vast ecosystem make it an attractive option for bringing existing codebases to the web. Here’s why C++ is a strong choice for WebAssembly development:
1. Performance
Like Rust, C++ is known for its high performance. When compiled to WebAssembly, C++ code can run at near-native speeds, making it suitable for computationally intensive tasks.
2. Existing Codebases
Many large-scale applications and libraries are written in C++. WebAssembly provides a path to bring these existing codebases to the web without a complete rewrite.
3. Low-Level Control
C++ offers fine-grained control over system resources, which aligns well with WebAssembly’s low-level nature. This allows developers to optimize their code for maximum efficiency.
4. Mature Tooling
The C++ ecosystem has developed robust tools for WebAssembly compilation, such as Emscripten, which simplifies the process of targeting WebAssembly from C++ code.
Example: C++ to WebAssembly
Here’s a simple C++ function that could be compiled to WebAssembly:
#include <emscripten/bind.h>
int multiply(int a, int b) {
return a * b;
}
EMSCRIPTEN_BINDINGS(my_module) {
emscripten::function("multiply", &multiply);
}
This C++ code can be compiled to WebAssembly using Emscripten and then used in JavaScript like this:
Module.onRuntimeInitialized = () => {
const result = Module.multiply(6, 7);
console.log(result); // Outputs: 42
};
Real-World Applications of WebAssembly
WebAssembly’s capabilities extend far beyond simple arithmetic operations. Let’s explore some real-world applications where WebAssembly is making a significant impact:
1. Game Development
WebAssembly has opened new possibilities for browser-based gaming. Game engines like Unity and Unreal Engine now support WebAssembly, allowing developers to bring complex 3D games to the web with performance comparable to native applications.
2. Video and Audio Processing
WASM’s performance benefits make it ideal for handling media processing tasks in the browser. Applications like video editors and audio workstations can now offer desktop-like performance on the web.
3. Scientific Simulations
Complex scientific simulations that were once the domain of desktop applications can now run efficiently in the browser thanks to WebAssembly. This has implications for fields like physics, chemistry, and climate science.
4. Cryptography
WebAssembly’s speed makes it suitable for implementing cryptographic algorithms in the browser. This can enhance the security of web applications without sacrificing performance.
5. Image Processing
Tasks like real-time image filtering, face detection, and computer vision can benefit greatly from WebAssembly’s performance, enabling sophisticated image processing directly in the browser.
Getting Started with WebAssembly
If you’re interested in exploring WebAssembly development, here are some steps to get started:
1. Choose Your Language
Decide whether you want to start with Rust, C++, or another supported language. Your choice may depend on your existing skills or the specific requirements of your project.
2. Set Up Your Development Environment
For Rust:
- Install Rust:
curl --proto '=https' --tlsv1.2 -sSf https://sh.rustup.rs | sh
- Install wasm-pack:
cargo install wasm-pack
For C++:
- Install Emscripten: Follow the instructions at https://emscripten.org/docs/getting_started/downloads.html
3. Create a Simple Project
Start with a small project to get familiar with the workflow. For example, you could create a simple math library or a basic image processing function.
4. Compile to WebAssembly
Use the appropriate tools to compile your code to WebAssembly. For Rust, you’d use wasm-pack
. For C++, you’d use Emscripten.
5. Integrate with JavaScript
Learn how to load and use your WebAssembly module from JavaScript. This typically involves using the WebAssembly JavaScript API.
6. Explore Advanced Topics
As you become more comfortable with WebAssembly, explore more advanced topics like:
- Optimizing WebAssembly performance
- Debugging WebAssembly code
- Integrating WebAssembly with existing web frameworks
- Using WebAssembly in Node.js environments
Challenges and Considerations
While WebAssembly offers exciting possibilities, it’s important to be aware of some challenges and considerations:
1. Learning Curve
If you’re primarily a JavaScript developer, there may be a significant learning curve involved in picking up languages like Rust or C++ and understanding the nuances of WebAssembly.
2. Browser Support
While WebAssembly is supported in all modern browsers, you may need to provide fallbacks for users on older browsers.
3. Debugging
Debugging WebAssembly can be more challenging than debugging JavaScript. While tools are improving, it’s not yet as seamless as traditional web development debugging.
4. File Size
WebAssembly modules can be larger than equivalent JavaScript code, which could impact load times. It’s important to balance performance gains against increased download sizes.
5. Complexity
Introducing WebAssembly into your project adds another layer of complexity. It’s important to weigh the benefits against the added complexity in your development process.
The Future of WebAssembly
WebAssembly is an evolving technology, and its future looks bright. Here are some developments to watch for:
1. Garbage Collection
There are proposals to add garbage collection support to WebAssembly, which could make it easier to use languages that rely on garbage collection.
2. Threading
Improved support for multi-threading in WebAssembly could lead to even better performance for certain types of applications.
3. SIMD Support
Single Instruction, Multiple Data (SIMD) support in WebAssembly could significantly boost performance for tasks like audio and video processing.
4. WebAssembly System Interface (WASI)
WASI aims to provide a standardized system interface for WebAssembly, potentially allowing WebAssembly to be used outside of web browsers in a portable and secure way.
Conclusion
WebAssembly represents a significant leap forward in web technology, offering the potential for high-performance applications that run directly in the browser. By leveraging languages like Rust and C++, developers can create web applications that rival desktop software in terms of speed and capability.
As we’ve explored in this article, WebAssembly opens up new possibilities for game development, scientific computing, media processing, and much more. While it comes with its own set of challenges, the benefits of WebAssembly make it a technology worth investing in.
Whether you’re a seasoned C++ developer looking to bring your applications to the web, a Rust enthusiast excited about the language’s synergy with WebAssembly, or a web developer eager to push the boundaries of what’s possible in the browser, WebAssembly offers exciting opportunities to expand your skills and create cutting-edge web applications.
As WebAssembly continues to evolve and mature, we can expect to see even more innovative uses of this technology in the future. The web platform is becoming more powerful than ever, and WebAssembly is playing a crucial role in this transformation. So dive in, experiment, and be part of the WebAssembly revolution!