Edge Computing: Revolutionizing Data Processing at the Network’s Edge
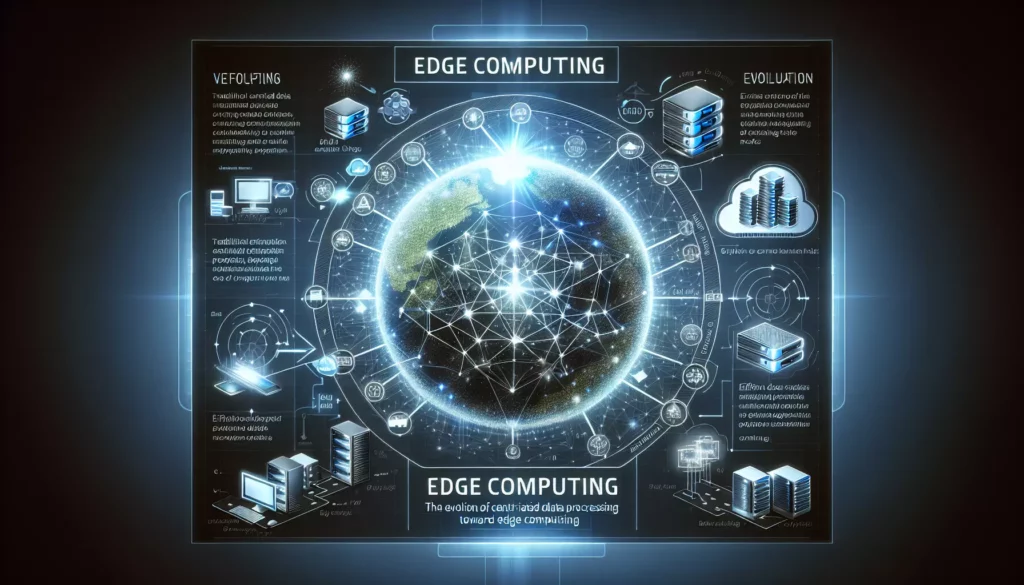
In today’s interconnected world, where data is generated at an unprecedented rate, the need for efficient data processing and reduced latency has never been more critical. Enter edge computing, a paradigm shift in how we handle and process data. This innovative approach brings computation and data storage closer to the source of data generation, offering numerous benefits in terms of speed, efficiency, and bandwidth utilization. In this comprehensive guide, we’ll explore the ins and outs of edge computing, its applications, and how it’s reshaping the landscape of modern computing.
What is Edge Computing?
Edge computing is a distributed computing paradigm that brings data processing and storage closer to the location where it is needed. Instead of relying solely on centralized data centers or cloud computing systems, edge computing pushes computing applications, data, and services away from centralized nodes to the logical extremes of a network. This approach enables faster processing times and reduces the amount of long-distance communication between client and server.
Key Characteristics of Edge Computing:
- Proximity: Computing resources are located closer to the data source
- Low latency: Reduced delay in data processing and transmission
- Bandwidth efficiency: Less data needs to be sent to centralized locations
- Real-time processing: Enables faster decision-making and responses
- Improved privacy and security: Sensitive data can be processed locally
The Evolution of Computing: From Centralized to Distributed
To understand the significance of edge computing, it’s essential to look at the evolution of computing paradigms:
- Centralized Computing: Early computing systems relied on mainframes, where all processing was done in a central location.
- Client-Server Model: This introduced distributed computing, with processing split between central servers and client devices.
- Cloud Computing: Enabled on-demand access to shared computing resources over the internet.
- Edge Computing: Brings computation closer to data sources, complementing cloud computing.
Edge computing doesn’t replace cloud computing but rather complements it. While cloud computing offers vast computational resources and storage, edge computing provides localized processing for time-sensitive data and applications.
How Edge Computing Works
Edge computing operates by distributing processing, storage, and networking resources closer to where data is generated. This can be at various points in the network topology:
- Device Edge: Computing occurs directly on IoT devices or sensors.
- Local Edge: Processing happens on local servers or gateways near the data source.
- Regional Edge: Computation takes place in small data centers distributed across geographical regions.
The edge computing process typically involves the following steps:
- Data is generated by devices or sensors.
- Local edge devices or gateways collect and process the data.
- Relevant data is analyzed and acted upon locally.
- Only necessary data is sent to the cloud for further processing or long-term storage.
Benefits of Edge Computing
Edge computing offers numerous advantages over traditional centralized computing models:
1. Reduced Latency
By processing data closer to its source, edge computing significantly reduces the time it takes for data to travel to a centralized server and back. This is crucial for applications that require real-time processing, such as autonomous vehicles or industrial automation.
2. Bandwidth Optimization
Edge computing reduces the amount of data that needs to be transmitted to central servers or the cloud. By processing and filtering data locally, only relevant information is sent over the network, leading to more efficient use of bandwidth.
3. Improved Reliability
Edge computing can continue to function even when internet connectivity is limited or unavailable. This makes it ideal for applications in remote locations or industries where continuous operation is critical.
4. Enhanced Security and Privacy
By processing sensitive data locally, edge computing reduces the risk of data breaches during transmission. It also helps organizations comply with data privacy regulations by keeping certain data within specific geographical boundaries.
5. Scalability
Edge computing allows for more flexible and scalable deployments. Organizations can add edge devices as needed without having to significantly upgrade their central infrastructure.
6. Cost Efficiency
While initial setup costs may be higher, edge computing can lead to long-term cost savings by reducing bandwidth usage and cloud computing expenses.
Applications of Edge Computing
Edge computing is finding applications across various industries and use cases:
1. Internet of Things (IoT)
Edge computing is crucial for IoT devices, enabling them to process data locally and make quick decisions. This is particularly important in smart home systems, industrial IoT, and connected vehicles.
2. Autonomous Vehicles
Self-driving cars generate massive amounts of data that need to be processed in real-time. Edge computing allows for quick decision-making based on sensor data, crucial for navigation and safety.
3. Healthcare
In healthcare, edge computing enables real-time monitoring of patients, quick analysis of medical imaging, and secure processing of sensitive patient data.
4. Retail
Edge computing powers smart retail applications like inventory management, personalized shopping experiences, and real-time analytics for in-store customer behavior.
5. Manufacturing
In industrial settings, edge computing facilitates predictive maintenance, real-time quality control, and automation of manufacturing processes.
6. Gaming and AR/VR
Edge computing reduces latency in online gaming and enables more immersive augmented and virtual reality experiences by processing data closer to the user.
Challenges and Considerations in Edge Computing
While edge computing offers numerous benefits, it also presents several challenges:
1. Security
Distributing computing resources increases the attack surface. Implementing robust security measures across all edge devices is crucial.
2. Management and Orchestration
Managing a distributed network of edge devices can be complex. Effective orchestration tools are necessary for deployment, updates, and monitoring.
3. Standardization
The lack of standardization in edge computing can lead to interoperability issues. Industry-wide standards are needed to ensure seamless integration of edge devices and systems.
4. Resource Constraints
Edge devices often have limited processing power and storage compared to cloud data centers. Efficient resource allocation and management are essential.
5. Connectivity
While edge computing can operate with limited connectivity, ensuring reliable communication between edge devices and central systems remains a challenge in some environments.
Implementing Edge Computing: Best Practices
To successfully implement edge computing in your organization, consider the following best practices:
1. Define Clear Use Cases
Identify specific applications and scenarios where edge computing can provide tangible benefits to your organization.
2. Choose the Right Edge Architecture
Determine whether you need device edge, local edge, or regional edge computing based on your requirements and constraints.
3. Prioritize Security
Implement robust security measures, including encryption, access controls, and regular security audits for all edge devices and systems.
4. Ensure Scalability
Design your edge computing infrastructure to be scalable, allowing for easy addition of new devices and capabilities as your needs grow.
5. Implement Effective Data Management
Develop clear policies for data processing, storage, and transmission between edge devices and central systems.
6. Leverage Containerization
Use containerization technologies like Docker to ensure consistency and ease of deployment across diverse edge environments.
7. Monitor and Optimize Performance
Implement robust monitoring tools to track the performance of your edge computing infrastructure and optimize as needed.
Edge Computing and Software Development
For software developers, edge computing introduces new paradigms and challenges in application development. Here are some key considerations:
1. Distributed Application Architecture
Developers need to design applications that can run efficiently in a distributed environment, with components that can operate both at the edge and in the cloud.
2. Resource-Aware Programming
Given the limited resources of many edge devices, developers must optimize their code for efficiency and minimal resource usage.
3. Offline-First Design
Applications should be designed to function effectively even when internet connectivity is limited or unavailable.
4. Edge-Specific Frameworks and Tools
Familiarize yourself with edge-specific development frameworks and tools, such as AWS Greengrass, Azure IoT Edge, or open-source options like EdgeX Foundry.
5. Data Synchronization
Implement effective data synchronization mechanisms between edge devices and central systems to ensure data consistency and integrity.
6. Security-First Approach
Incorporate security best practices into your development process, including secure communication protocols, data encryption, and access controls.
Code Example: Simple Edge Computing Simulation
To illustrate the concept of edge computing, let’s look at a simple Python script that simulates data processing at the edge:
import random
import time
class EdgeDevice:
def __init__(self, device_id):
self.device_id = device_id
self.data_buffer = []
def generate_data(self):
return random.randint(0, 100)
def process_data(self):
if len(self.data_buffer) > 0:
avg = sum(self.data_buffer) / len(self.data_buffer)
self.data_buffer = []
return avg
return None
def send_to_cloud(self, data):
print(f"Device {self.device_id} sending data to cloud: {data}")
def simulate_edge_computing():
edge_device = EdgeDevice(1)
for _ in range(20):
# Generate and buffer data
data = edge_device.generate_data()
edge_device.data_buffer.append(data)
print(f"Device {edge_device.device_id} generated data: {data}")
# Process data at the edge every 5 iterations
if (_ + 1) % 5 == 0:
processed_data = edge_device.process_data()
if processed_data:
edge_device.send_to_cloud(processed_data)
time.sleep(1)
if __name__ == "__main__":
simulate_edge_computing()
This script simulates an edge device that generates random data, processes it locally by calculating the average, and only sends the processed data to the cloud periodically. This demonstrates the basic principle of edge computing: local data processing and reduced data transmission to central systems.
The Future of Edge Computing
As technology continues to evolve, edge computing is poised to play an increasingly important role in our digital infrastructure. Several trends are shaping the future of edge computing:
1. 5G Integration
The rollout of 5G networks will provide faster, more reliable connectivity, further enhancing the capabilities of edge computing systems.
2. AI and Machine Learning at the Edge
Advances in hardware and algorithms are enabling more sophisticated AI and machine learning models to run directly on edge devices, opening up new possibilities for intelligent, real-time decision-making.
3. Edge-Cloud Continuum
The line between edge and cloud computing will continue to blur, with seamless integration allowing for flexible distribution of computing tasks across the entire network topology.
4. Industry-Specific Edge Solutions
We can expect to see more tailored edge computing solutions for specific industries, addressing unique challenges in healthcare, manufacturing, smart cities, and more.
5. Edge-Native Applications
Just as cloud-native applications emerged with the rise of cloud computing, we’ll likely see the development of edge-native applications designed specifically to leverage the unique characteristics of edge environments.
Conclusion
Edge computing represents a significant shift in how we approach data processing and network architecture. By bringing computation closer to the source of data, it offers solutions to many of the challenges posed by the explosive growth of IoT devices and the increasing demand for real-time processing. While it comes with its own set of challenges, the benefits of reduced latency, improved bandwidth utilization, and enhanced privacy make edge computing a crucial technology for the future of computing.
For developers and organizations alike, understanding and embracing edge computing will be essential in creating the next generation of efficient, responsive, and intelligent applications. As we move forward, the synergy between edge computing, cloud computing, and emerging technologies like 5G and AI will undoubtedly lead to innovative solutions that we can scarcely imagine today.
In the realm of coding education and skill development, platforms like AlgoCademy play a crucial role in preparing developers for this evolving landscape. By focusing on algorithmic thinking, problem-solving, and practical coding skills, learners can develop the foundational knowledge needed to work effectively with distributed systems like edge computing. As the demand for edge computing expertise grows, having a solid grounding in these fundamental skills will be invaluable for developers looking to excel in this exciting and rapidly evolving field.