Learn Shell Scripting: Automate Tasks in Unix/Linux Environments with Bash and PowerShell
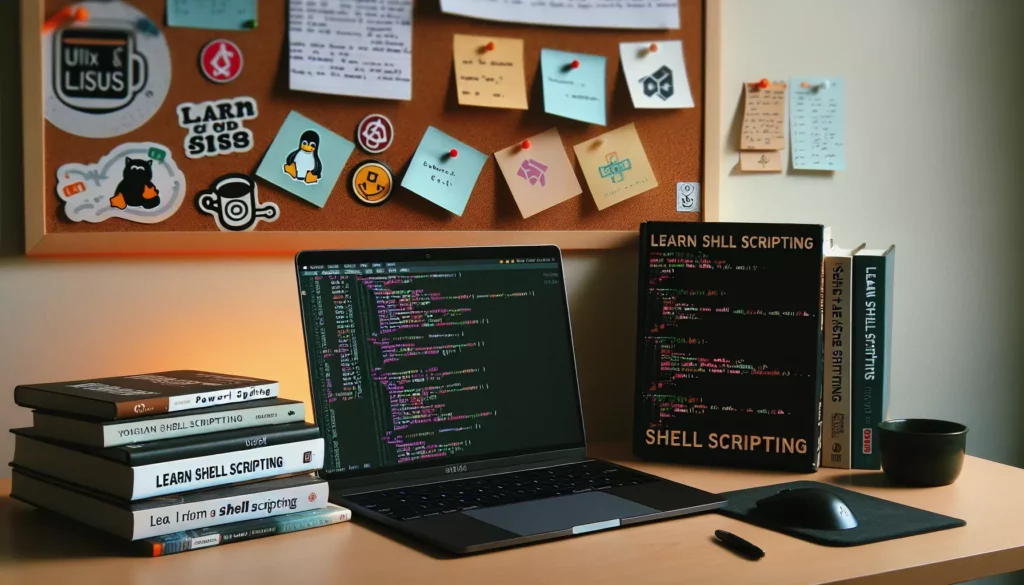
In today’s fast-paced world of technology, automation is key to increasing productivity and efficiency. For developers, system administrators, and IT professionals, shell scripting is an essential skill that allows you to automate repetitive tasks, manage systems, and streamline workflows. In this comprehensive guide, we’ll dive deep into shell scripting, focusing on two powerful scripting languages: Bash for Unix/Linux environments and PowerShell for Windows (and increasingly, cross-platform) automation.
Table of Contents
- Introduction to Shell Scripting
- Bash Scripting Basics
- Advanced Bash Scripting Techniques
- PowerShell Scripting Basics
- Advanced PowerShell Scripting Techniques
- Real-World Scripting Examples
- Best Practices and Tips
- Additional Resources and Learning Paths
- Conclusion
1. Introduction to Shell Scripting
Shell scripting is a method of automating command-line instructions to perform tasks efficiently. It involves writing a series of commands in a file that can be executed as a program. The two main types of shell scripting we’ll focus on are:
- Bash (Bourne Again Shell): The default shell for most Unix-like operating systems, including Linux distributions and macOS.
- PowerShell: Microsoft’s task automation framework, originally designed for Windows but now available on multiple platforms.
Learning shell scripting offers numerous benefits:
- Automate repetitive tasks
- Improve system administration efficiency
- Customize and extend command-line capabilities
- Enhance problem-solving skills
- Increase productivity in development and operations
2. Bash Scripting Basics
Bash (Bourne Again Shell) is a powerful scripting language that’s widely used in Unix-like environments. Let’s start with the basics:
Creating Your First Bash Script
- Open a text editor and create a new file with a .sh extension (e.g., myscript.sh).
- Add the shebang line at the top of the file to specify the interpreter:
#!/bin/bash
#!/bin/bash
echo "Hello, World!"
echo "This is my first Bash script."
chmod +x myscript.sh
./myscript.sh
Variables and Data Types
Bash uses variables to store data. Here’s how to work with variables:
#!/bin/bash
# Assigning values to variables
name="John Doe"
age=30
# Using variables
echo "My name is $name and I am $age years old."
# Arithmetic operations
result=$((age + 5))
echo "In 5 years, I will be $result years old."
Control Structures
Bash supports various control structures for decision-making and looping:
If-Else Statements
#!/bin/bash
age=18
if [ $age -ge 18 ]; then
echo "You are an adult."
else
echo "You are a minor."
fi
Loops
#!/bin/bash
# For loop
for i in {1..5}; do
echo "Iteration $i"
done
# While loop
count=0
while [ $count -lt 5 ]; do
echo "Count: $count"
count=$((count + 1))
done
3. Advanced Bash Scripting Techniques
As you become more comfortable with Bash scripting, you can explore advanced techniques to create more powerful and flexible scripts.
Functions
Functions allow you to organize your code into reusable blocks:
#!/bin/bash
# Function definition
greet() {
echo "Hello, $1!"
}
# Function call
greet "Alice"
greet "Bob"
Input and Output
Bash provides various ways to handle input and output:
#!/bin/bash
# Reading user input
echo "What's your name?"
read name
# Writing to a file
echo "Hello, $name!" > greeting.txt
# Reading from a file
content=$(cat greeting.txt)
echo "File content: $content"
Error Handling
Proper error handling is crucial for robust scripts:
#!/bin/bash
# Exit on error
set -e
# Custom error handling
error_handler() {
echo "An error occurred on line $1"
exit 1
}
trap 'error_handler $LINENO' ERR
# Simulate an error
ls /nonexistent_directory
4. PowerShell Scripting Basics
PowerShell is a powerful scripting language and shell environment developed by Microsoft. It’s designed for system administration and automation tasks. Let’s explore the basics:
Creating Your First PowerShell Script
- Open a text editor and create a new file with a .ps1 extension (e.g., myscript.ps1).
- Write your script commands:
Write-Host "Hello, World!"
Write-Host "This is my first PowerShell script."
.\myscript.ps1
Variables and Data Types
PowerShell uses strongly-typed variables:
$name = "John Doe"
$age = 30
Write-Host "My name is $name and I am $age years old."
$result = $age + 5
Write-Host "In 5 years, I will be $result years old."
Control Structures
If-Else Statements
$age = 18
if ($age -ge 18) {
Write-Host "You are an adult."
} else {
Write-Host "You are a minor."
}
Loops
# ForEach loop
1..5 | ForEach-Object {
Write-Host "Iteration $_"
}
# While loop
$count = 0
while ($count -lt 5) {
Write-Host "Count: $count"
$count++
}
5. Advanced PowerShell Scripting Techniques
PowerShell offers advanced features for more complex scripting scenarios:
Functions
function Greet {
param($name)
Write-Host "Hello, $name!"
}
Greet -name "Alice"
Greet -name "Bob"
Working with Objects
PowerShell is object-oriented, allowing you to work with complex data structures:
$person = New-Object PSObject -Property @{
Name = "John Doe"
Age = 30
City = "New York"
}
$person | Format-Table -AutoSize
Error Handling
try {
# Simulate an error
Get-ChildItem -Path C:\NonexistentFolder -ErrorAction Stop
} catch {
Write-Host "An error occurred: $_"
} finally {
Write-Host "This block always executes."
}
6. Real-World Scripting Examples
Let’s explore some practical examples of shell scripting that demonstrate how these skills can be applied in real-world scenarios:
Bash: Automated Backup Script
#!/bin/bash
# Automated backup script
# Set variables
source_dir="/path/to/source"
backup_dir="/path/to/backup"
date=$(date +"%Y%m%d_%H%M%S")
backup_file="backup_$date.tar.gz"
# Create backup
tar -czf "$backup_dir/$backup_file" "$source_dir"
# Check if backup was successful
if [ $? -eq 0 ]; then
echo "Backup created successfully: $backup_file"
else
echo "Backup failed"
exit 1
fi
# Remove backups older than 30 days
find "$backup_dir" -name "backup_*.tar.gz" -mtime +30 -delete
echo "Backup process completed"
PowerShell: System Health Check Script
# System Health Check Script
function Check-DiskSpace {
$disks = Get-WmiObject Win32_LogicalDisk -Filter "DriveType=3"
foreach ($disk in $disks) {
$freeSpacePercent = [math]::Round(($disk.FreeSpace / $disk.Size) * 100, 2)
Write-Host "Drive $($disk.DeviceID) - Free Space: $freeSpacePercent%"
}
}
function Check-CPUUsage {
$cpu = Get-WmiObject Win32_Processor | Measure-Object -Property LoadPercentage -Average
Write-Host "CPU Usage: $($cpu.Average)%"
}
function Check-MemoryUsage {
$memory = Get-WmiObject Win32_OperatingSystem
$usedMemory = $memory.TotalVisibleMemorySize - $memory.FreePhysicalMemory
$usedMemoryPercent = [math]::Round(($usedMemory / $memory.TotalVisibleMemorySize) * 100, 2)
Write-Host "Memory Usage: $usedMemoryPercent%"
}
Write-Host "=== System Health Check ==="
Check-DiskSpace
Check-CPUUsage
Check-MemoryUsage
7. Best Practices and Tips
To write effective and maintainable shell scripts, consider the following best practices:
- Use meaningful variable names: Choose descriptive names that clearly indicate the purpose of each variable.
- Comment your code: Add comments to explain complex logic or the purpose of specific sections.
- Modularize your code: Use functions to break down complex tasks into smaller, reusable components.
- Handle errors gracefully: Implement proper error handling to make your scripts more robust.
- Use version control: Store your scripts in a version control system like Git to track changes and collaborate with others.
- Test your scripts: Thoroughly test your scripts in a safe environment before using them in production.
- Follow style guides: Adhere to established style guides for consistent and readable code.
- Use shellcheck (for Bash): Utilize tools like shellcheck to identify and fix common scripting errors.
- Secure your scripts: Be mindful of security implications, especially when handling sensitive data or executing commands with elevated privileges.
- Keep scripts updated: Regularly review and update your scripts to ensure they remain compatible with system changes and security updates.
8. Additional Resources and Learning Paths
To further enhance your shell scripting skills, consider exploring these resources:
Bash Resources
PowerShell Resources
Online Courses and Tutorials
- Codecademy: Learn the Command Line
- Udemy: Linux Shell Scripting: A Project-Based Approach to Learning
- Pluralsight: PowerShell Skill Path
Books
- “Learning the bash Shell” by Cameron Newham
- “Mastering PowerShell Scripting” by Chris Dent
- “Shell Scripting: Expert Recipes for Linux, Bash, and More” by Steve Parker
9. Conclusion
Shell scripting is an invaluable skill for anyone working in software development, system administration, or IT operations. By mastering Bash for Unix/Linux environments and PowerShell for Windows (and increasingly cross-platform) automation, you’ll be able to streamline your workflows, automate repetitive tasks, and solve complex problems more efficiently.
This guide has provided you with a solid foundation in both Bash and PowerShell scripting, covering everything from basic syntax to advanced techniques and real-world examples. As you continue to practice and apply these skills, you’ll discover new ways to leverage shell scripting in your daily work.
Remember that the key to becoming proficient in shell scripting is consistent practice and continuous learning. Start with small scripts to automate simple tasks, and gradually work your way up to more complex projects. Don’t hesitate to explore the additional resources provided and engage with the scripting communities to further enhance your skills.
As you progress in your scripting journey, you’ll find that these skills not only make you more productive but also open up new career opportunities in areas such as DevOps, cloud computing, and system administration. So keep scripting, keep learning, and enjoy the power of automation at your fingertips!