Understanding Real-Time Programming: From Embedded Systems to Gaming
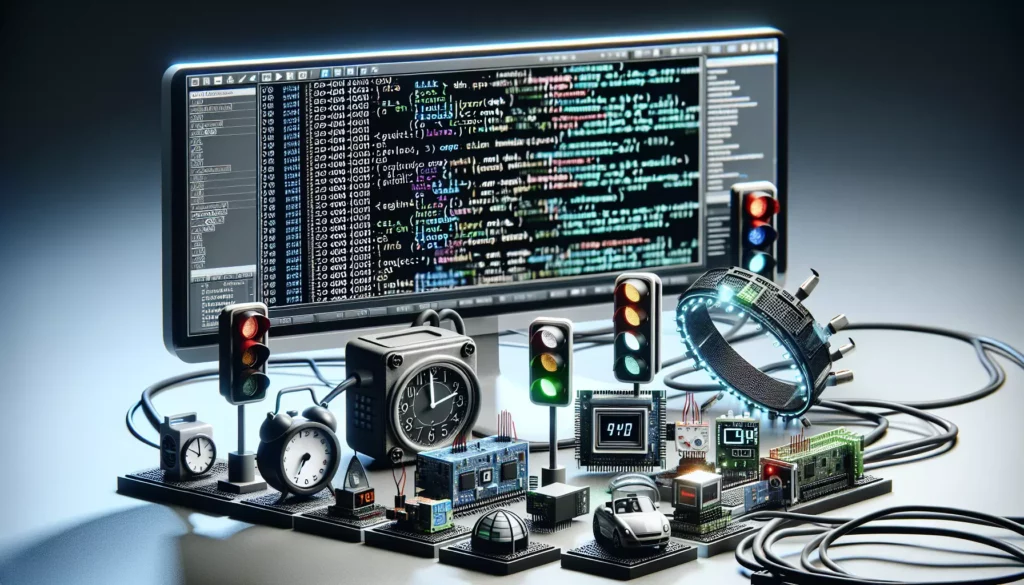
In the fast-paced world of technology, where every millisecond counts, real-time programming has become an indispensable skill for developers. Whether you’re working on embedded systems that control critical infrastructure or creating immersive gaming experiences, understanding real-time programming is crucial. In this comprehensive guide, we’ll dive deep into the world of real-time systems, exploring their applications, challenges, and the skills you need to master them.
What is Real-Time Programming?
Real-time programming refers to the development of software systems that must respond to events or process data within strict time constraints. These systems are designed to guarantee a response within a specified time frame, often measured in milliseconds or even microseconds. The key characteristic of real-time systems is their ability to meet deadlines consistently, ensuring that critical operations are performed on time, every time.
Real-time systems are typically categorized into two main types:
- Hard Real-Time Systems: These systems have absolutely no tolerance for missed deadlines. A failure to meet a deadline can result in catastrophic consequences. Examples include aircraft control systems, medical devices, and industrial robotics.
- Soft Real-Time Systems: While these systems also have time constraints, occasional missed deadlines are tolerable and don’t lead to system failure. However, the overall quality of the system degrades if deadlines are frequently missed. Examples include online gaming, multimedia streaming, and some telecommunications applications.
Applications of Real-Time Programming
Real-time programming finds applications in various domains, each with its unique set of challenges and requirements. Let’s explore some of the most prominent areas where real-time systems are essential:
1. Embedded Systems
Embedded systems are specialized computer systems designed to perform specific functions within larger mechanical or electrical systems. These systems often have real-time constraints and are found in a wide range of devices, from simple household appliances to complex industrial machinery.
Examples of embedded systems with real-time requirements include:
- Automotive control systems (e.g., anti-lock braking systems, engine control units)
- Medical devices (e.g., pacemakers, insulin pumps)
- Avionics systems in aircraft
- Industrial automation and robotics
- Smart home devices and IoT sensors
In embedded systems, real-time programming ensures that critical operations are performed within strict time constraints, often in resource-constrained environments with limited processing power and memory.
2. Gaming
The gaming industry heavily relies on real-time programming to create immersive and responsive experiences for players. Game developers must ensure that the game world updates smoothly, player inputs are processed instantly, and complex physics simulations run in real-time.
Key aspects of real-time programming in gaming include:
- Frame rate management to maintain smooth visuals
- Input processing and response
- Physics simulations for realistic object interactions
- AI behavior and decision-making
- Networking for multiplayer games
While gaming often falls under the soft real-time category, maintaining consistent performance is crucial for player satisfaction and competitive fairness in multiplayer scenarios.
3. Telecommunications
The telecommunications industry relies heavily on real-time systems to ensure reliable and efficient communication. From mobile networks to internet infrastructure, real-time programming plays a vital role in managing data traffic, routing calls, and maintaining quality of service.
Real-time applications in telecommunications include:
- Voice over IP (VoIP) systems
- Network routing and switching
- Traffic management and load balancing
- Quality of Service (QoS) monitoring and management
- Real-time data compression and encryption
4. Financial Systems
In the world of finance, where milliseconds can mean millions of dollars, real-time systems are essential for various operations. High-frequency trading, fraud detection, and risk management all rely on real-time processing to make split-second decisions based on vast amounts of data.
Real-time applications in finance include:
- Algorithmic trading systems
- Real-time market data processing
- Fraud detection and prevention
- Risk assessment and management
- Payment processing systems
5. Multimedia and Streaming
The rise of digital media and streaming services has created a demand for real-time systems capable of processing and delivering high-quality audio and video content with minimal latency. From live streaming platforms to video conferencing applications, real-time programming ensures smooth and synchronized multimedia experiences.
Real-time aspects of multimedia and streaming include:
- Audio/video encoding and decoding
- Streaming protocol implementation
- Buffer management for smooth playback
- Synchronization of audio and video streams
- Adaptive bitrate streaming for varying network conditions
Challenges in Real-Time Programming
Developing real-time systems comes with its own set of challenges that programmers must overcome to ensure reliable and efficient operation. Some of the key challenges include:
1. Timing Constraints
The most fundamental challenge in real-time programming is meeting strict timing constraints. Developers must ensure that tasks are completed within their specified deadlines, which often requires careful scheduling and optimization of code execution.
2. Determinism
Real-time systems must behave predictably, producing the same output for the same input within the same time frame. Achieving determinism can be challenging, especially when dealing with complex systems or unpredictable external events.
3. Resource Management
Many real-time systems operate in resource-constrained environments, requiring efficient management of CPU time, memory, and other system resources. Balancing performance with resource utilization is a constant challenge for real-time programmers.
4. Concurrency and Synchronization
Real-time systems often involve multiple tasks running concurrently, which can lead to race conditions, deadlocks, and other synchronization issues. Proper handling of concurrent processes is crucial for maintaining system stability and meeting timing requirements.
5. Fault Tolerance
In critical real-time systems, fault tolerance is essential to ensure continuous operation even in the face of hardware or software failures. Implementing robust error detection and recovery mechanisms adds complexity to real-time system design.
6. Testing and Verification
Thoroughly testing real-time systems can be challenging due to the need to simulate various timing scenarios and edge cases. Verifying that a system meets its timing requirements under all possible conditions is a complex and time-consuming process.
Key Concepts and Techniques in Real-Time Programming
To overcome the challenges of real-time programming and develop efficient and reliable systems, developers must be familiar with several key concepts and techniques:
1. Task Scheduling
Task scheduling is at the heart of real-time systems, determining how and when different tasks are executed to meet timing constraints. Common scheduling algorithms include:
- Rate Monotonic Scheduling (RMS)
- Earliest Deadline First (EDF)
- Deadline Monotonic Scheduling (DMS)
Understanding these algorithms and their trade-offs is crucial for designing effective real-time systems.
2. Interrupt Handling
Interrupts are essential for real-time systems to respond quickly to external events. Proper interrupt handling ensures that high-priority tasks are executed promptly without compromising system stability.
3. Memory Management
Efficient memory management is critical in real-time systems, especially in embedded environments with limited resources. Techniques such as static memory allocation, memory pools, and careful management of dynamic memory can help avoid unpredictable behavior and memory fragmentation.
4. Real-Time Operating Systems (RTOS)
Real-Time Operating Systems are specialized operating systems designed to support the development and execution of real-time applications. RTOSes provide features such as priority-based scheduling, deterministic behavior, and low-latency interrupt handling. Popular RTOSes include:
- FreeRTOS
- VxWorks
- QNX
- RTLinux
5. Time Management and Synchronization
Accurate time management and synchronization are crucial in real-time systems. Techniques such as timers, watchdogs, and clock synchronization protocols help ensure that tasks are executed at the right time and that distributed systems remain coordinated.
6. Performance Optimization
Optimizing code for performance is essential in real-time systems. This may involve techniques such as:
- Inline assembly for time-critical operations
- Loop unrolling and function inlining
- Efficient data structures and algorithms
- Cache optimization
7. Formal Methods and Verification
For critical real-time systems, formal methods and verification techniques can be used to mathematically prove that the system meets its timing and safety requirements. Tools and techniques in this area include:
- Model checking
- Theorem proving
- Static analysis
- Worst-case execution time (WCET) analysis
Programming Languages for Real-Time Systems
While real-time systems can be developed in various programming languages, some languages are particularly well-suited for real-time programming due to their features and performance characteristics. Here are some popular choices:
1. C
C remains one of the most widely used languages for real-time programming, especially in embedded systems. Its low-level control, efficient performance, and predictable behavior make it an excellent choice for time-critical applications.
Example of a simple real-time task in C using FreeRTOS:
#include <FreeRTOS.h>
#include <task.h>
void vTaskFunction(void *pvParameters) {
for (;;) {
// Perform real-time task
vTaskDelay(pdMS_TO_TICKS(100)); // Delay for 100ms
}
}
int main(void) {
xTaskCreate(vTaskFunction, "RTTask", configMINIMAL_STACK_SIZE, NULL, tskIDLE_PRIORITY + 1, NULL);
vTaskStartScheduler();
return 0;
}
2. C++
C++ combines the efficiency of C with object-oriented programming features, making it suitable for larger and more complex real-time systems. Many game engines and high-performance applications are written in C++.
3. Ada
Ada was designed specifically for real-time and embedded systems, with built-in support for concurrency, strong typing, and other features that promote reliability and maintainability. It’s commonly used in aerospace and defense applications.
4. Rust
Rust is gaining popularity for systems programming, including real-time applications. Its focus on memory safety and zero-cost abstractions makes it an attractive option for developing reliable and efficient real-time systems.
5. Java (Real-Time Specification for Java – RTSJ)
While standard Java is not suitable for hard real-time systems due to its garbage collection and JIT compilation, the Real-Time Specification for Java (RTSJ) provides extensions that enable the development of real-time applications in Java.
Tools and Frameworks for Real-Time Development
Developing real-time systems often requires specialized tools and frameworks. Here are some popular options:
1. Real-Time Operating Systems (RTOS)
- FreeRTOS: A popular open-source RTOS for microcontrollers and small microprocessors
- VxWorks: A commercial RTOS widely used in aerospace and defense
- QNX: A Unix-like RTOS used in automotive and industrial applications
2. Integrated Development Environments (IDEs)
- Eclipse CDT: An open-source IDE with support for C/C++ development and various RTOS integrations
- IAR Embedded Workbench: A commercial IDE for embedded systems development
- Visual Studio with Real-Time Extensions: Microsoft’s IDE with support for real-time application development
3. Debugging and Profiling Tools
- Tracealyzer: A visualization tool for analyzing the runtime behavior of RTOS-based systems
- SEGGER J-Link: A debug probe and software for embedded systems development
- Valgrind: An open-source tool for memory debugging, memory leak detection, and profiling
4. Simulation and Modeling Tools
- MATLAB and Simulink: Widely used for modeling and simulating real-time systems
- LabVIEW: A graphical programming environment for real-time data acquisition and control systems
- Ptolemy II: An open-source modeling and simulation framework for real-time and embedded systems
Best Practices for Real-Time Programming
To develop reliable and efficient real-time systems, consider the following best practices:
- Understand the system requirements: Clearly define the timing constraints, resource limitations, and functional requirements of your real-time system.
- Use appropriate design patterns: Implement design patterns that promote modularity, reusability, and maintainability in real-time systems.
- Minimize system jitter: Reduce variations in task execution times to ensure consistent system behavior.
- Avoid dynamic memory allocation: Use static memory allocation or memory pools to prevent unpredictable behavior and fragmentation.
- Implement proper error handling: Design robust error detection and recovery mechanisms to maintain system stability.
- Optimize critical paths: Identify and optimize the most time-critical sections of your code for maximum performance.
- Use appropriate synchronization mechanisms: Choose the right synchronization primitives (e.g., mutexes, semaphores) to avoid deadlocks and priority inversion.
- Conduct thorough testing: Perform extensive testing under various conditions to verify that your system meets its timing and functional requirements.
- Document thoroughly: Maintain clear and comprehensive documentation of your real-time system’s architecture, design decisions, and implementation details.
- Stay updated: Keep abreast of the latest developments in real-time programming techniques, tools, and best practices.
Conclusion
Real-time programming is a challenging yet essential field in today’s technology-driven world. From embedded systems controlling critical infrastructure to gaming engines delivering immersive experiences, real-time systems play a crucial role in numerous applications. By understanding the principles, challenges, and best practices of real-time programming, developers can create robust, efficient, and reliable systems that meet the demanding requirements of time-critical applications.
As you embark on your journey into real-time programming, remember that practice and hands-on experience are key to mastering these concepts. Start with small projects, gradually increasing complexity as you become more comfortable with real-time constraints and techniques. Whether you’re interested in embedded systems, game development, or any other field that requires real-time processing, the skills you develop in this area will be invaluable in your career as a programmer.
Keep exploring, experimenting, and pushing the boundaries of what’s possible in real-time systems. The field is constantly evolving, with new challenges and opportunities emerging as technology advances. By staying curious and committed to learning, you’ll be well-equipped to tackle the exciting world of real-time programming and contribute to the next generation of innovative, time-critical applications.