Diving into Progressive Web Apps (PWAs): Building Web Applications with Native App Capabilities
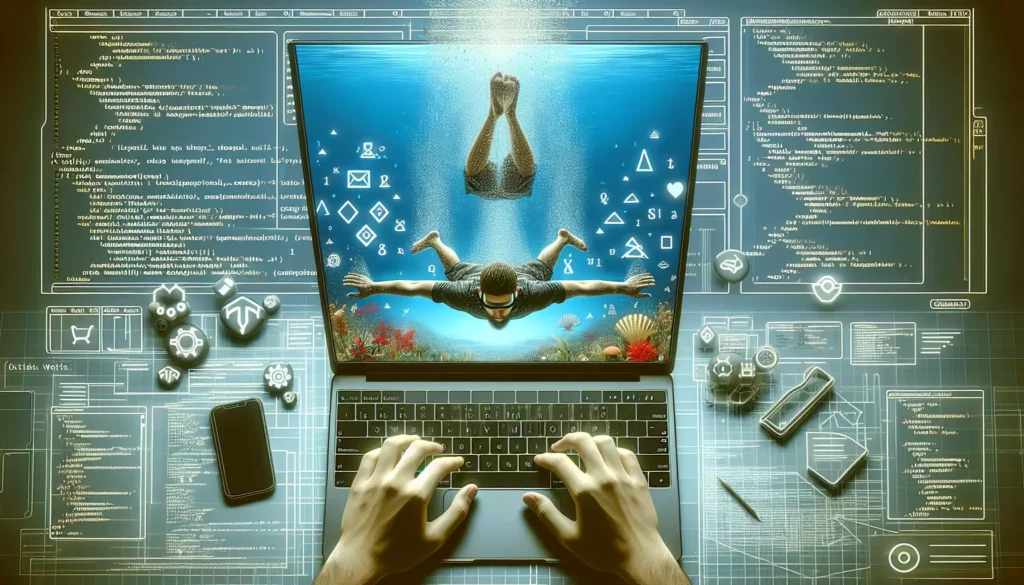
In today’s digital landscape, the line between web and native applications is becoming increasingly blurred. Progressive Web Apps (PWAs) are at the forefront of this convergence, offering a powerful solution that combines the best of both worlds. In this comprehensive guide, we’ll explore the world of PWAs, their benefits, and how to build them, with a focus on implementing features like offline access and push notifications.
Table of Contents
- What are Progressive Web Apps?
- Benefits of Progressive Web Apps
- Key Features of Progressive Web Apps
- Building Progressive Web Apps
- Implementing Offline Access
- Adding Push Notifications
- Testing and Debugging PWAs
- PWA Case Studies and Success Stories
- The Future of Progressive Web Apps
- Conclusion
1. What are Progressive Web Apps?
Progressive Web Apps (PWAs) are web applications that utilize modern web capabilities to deliver an app-like experience to users. They are designed to work on any platform that uses a standards-compliant browser, including both desktop and mobile devices. PWAs are progressive, meaning they work for every user, regardless of browser choice, using progressive enhancement principles.
The term “Progressive Web App” was coined by Google engineer Alex Russell and designer Frances Berriman in 2015. Since then, PWAs have gained significant traction among developers and businesses alike, offering a way to create fast, reliable, and engaging web experiences that can rival native applications.
2. Benefits of Progressive Web Apps
PWAs offer numerous advantages over traditional web applications and native apps:
- Cross-platform compatibility: PWAs work on any device with a modern web browser, reducing development costs and time.
- Improved performance: PWAs are typically faster and more responsive than traditional web apps.
- Offline functionality: Users can access content and features even without an internet connection.
- App-like experience: PWAs can be installed on the home screen and launched like native apps.
- Automatic updates: PWAs update automatically, ensuring users always have the latest version.
- Lower development and maintenance costs: A single PWA can replace multiple platform-specific apps.
- Improved discoverability: PWAs are indexable by search engines, making them easier to find.
- Push notifications: PWAs can send push notifications to engage users, even when the app is not active.
3. Key Features of Progressive Web Apps
To qualify as a PWA, an application must possess certain key features:
- Progressive: Works for every user, regardless of browser choice.
- Responsive: Fits any form factor: desktop, mobile, tablet, or whatever is next.
- Connectivity independent: Works offline or with poor network conditions.
- App-like: Uses the app-shell model to provide app-style navigations and interactions.
- Fresh: Always up-to-date thanks to the service worker update process.
- Safe: Served via HTTPS to prevent snooping and ensure content hasn’t been tampered with.
- Discoverable: Is identifiable as an “application” thanks to W3C manifests and service worker registration scope.
- Re-engageable: Makes re-engagement easy through features like push notifications.
- Installable: Allows users to “keep” apps they find most useful on their home screen without the hassle of an app store.
- Linkable: Easily shared via URL and does not require complex installation.
4. Building Progressive Web Apps
Building a PWA involves several key components and technologies:
4.1. Web App Manifest
The Web App Manifest is a JSON file that provides information about the web application. It includes details such as the app’s name, icons, theme colors, and display preferences. Here’s an example of a basic manifest file:
{
"name": "My PWA",
"short_name": "PWA",
"start_url": "/",
"display": "standalone",
"background_color": "#ffffff",
"theme_color": "#000000",
"icons": [
{
"src": "icon-192x192.png",
"sizes": "192x192",
"type": "image/png"
},
{
"src": "icon-512x512.png",
"sizes": "512x512",
"type": "image/png"
}
]
}
4.2. Service Workers
Service Workers are scripts that run in the background, separate from the web page. They enable features like offline functionality, background sync, and push notifications. Here’s a basic example of registering a service worker:
if ('serviceWorker' in navigator) {
window.addEventListener('load', function() {
navigator.serviceWorker.register('/sw.js').then(function(registration) {
console.log('ServiceWorker registration successful with scope: ', registration.scope);
}, function(err) {
console.log('ServiceWorker registration failed: ', err);
});
});
}
4.3. HTTPS
PWAs must be served over HTTPS to ensure security. This protects user data and prevents man-in-the-middle attacks. Many features of PWAs, such as service workers, require HTTPS to function.
4.4. Responsive Design
PWAs should be designed to work on any device or screen size. This typically involves using responsive design techniques, such as fluid grids, flexible images, and CSS media queries.
5. Implementing Offline Access
One of the key features of PWAs is their ability to work offline or in low-network conditions. This is achieved through the use of service workers and the Cache API.
5.1. Caching Resources
To cache resources for offline use, you can use the Cache API within your service worker. Here’s an example of caching files during the service worker installation:
const CACHE_NAME = 'my-pwa-cache-v1';
const urlsToCache = [
'/',
'/styles/main.css',
'/script/main.js'
];
self.addEventListener('install', function(event) {
event.waitUntil(
caches.open(CACHE_NAME)
.then(function(cache) {
console.log('Opened cache');
return cache.addAll(urlsToCache);
})
);
});
5.2. Serving Cached Content
Once resources are cached, you can serve them when the user is offline. Here’s an example of intercepting fetch requests and serving cached content:
self.addEventListener('fetch', function(event) {
event.respondWith(
caches.match(event.request)
.then(function(response) {
if (response) {
return response;
}
return fetch(event.request);
}
)
);
});
5.3. Background Sync
For actions that require network connectivity, such as submitting a form, you can use background sync to defer the action until the user is back online:
if ('serviceWorker' in navigator && 'SyncManager' in window) {
navigator.serviceWorker.ready.then(function(reg) {
return reg.sync.register('myFirstSync');
});
}
// In the service worker
self.addEventListener('sync', function(event) {
if (event.tag == 'myFirstSync') {
event.waitUntil(doSomeAsyncThing());
}
});
6. Adding Push Notifications
Push notifications are a powerful way to re-engage users and provide timely updates. Implementing push notifications in a PWA involves several steps:
6.1. Requesting Permission
First, you need to request permission from the user to send notifications:
Notification.requestPermission().then(function(result) {
if (result === 'granted') {
console.log('Notification permission granted!');
}
});
6.2. Subscribing to Push Notifications
Once permission is granted, you can subscribe the user to push notifications:
navigator.serviceWorker.ready.then(function(reg) {
reg.pushManager.subscribe({
userVisibleOnly: true,
applicationServerKey: 'BLKe_PuZ4wBkTAaYZhc-HlKWEBDzgf8rI0pB4rPaFjAWCDP6I6AnGO4TXCQG2xhyk2nKiVAL_GXAcB8sV44rELk'
}).then(function(sub) {
console.log('Endpoint URL: ', sub.endpoint);
}).catch(function(e) {
if (Notification.permission === 'denied') {
console.warn('Permission for notifications was denied');
} else {
console.error('Unable to subscribe to push', e);
}
});
});
6.3. Handling Push Events
In your service worker, you can handle incoming push events and display notifications:
self.addEventListener('push', function(event) {
if (event.data) {
console.log('Push event!! ', event.data.text());
showNotification(event.data.text());
} else {
console.log('Push event but no data');
}
});
function showNotification(message) {
self.registration.showNotification('PWA Notification', {
body: message,
});
}
7. Testing and Debugging PWAs
Testing and debugging PWAs requires a different approach compared to traditional web applications. Here are some tools and techniques you can use:
7.1. Chrome DevTools
Chrome DevTools provides a suite of PWA-specific tools:
- Application panel: Inspect your web app manifest, service workers, and caches.
- Lighthouse: Audit your PWA for performance, accessibility, and PWA best practices.
- Network panel: Simulate offline conditions to test your app’s offline functionality.
7.2. Workbox
Workbox is a set of libraries and Node modules that make it easy to cache assets and take full advantage of features used to build Progressive Web Apps.
7.3. PWA Builder
PWA Builder is an online tool that helps you build and test your PWA. It can generate necessary files like the web app manifest and provide suggestions for improvement.
8. PWA Case Studies and Success Stories
Many companies have successfully implemented PWAs and seen significant improvements in user engagement and conversions:
- Twitter Lite: Reduced data usage by 70% and increased tweets and page views.
- Pinterest: Increased user-generated ad revenue by 44% and core engagements by 60%.
- Alibaba: Increased conversions for new users across browsers by 104%.
- Uber: Made their app accessible to anyone on any network or device.
9. The Future of Progressive Web Apps
The future of PWAs looks bright, with continued support and development from major tech companies:
- Improved integration with operating systems: PWAs are becoming more deeply integrated with operating systems, blurring the line between web and native apps.
- Enhanced capabilities: New web APIs are continually being developed, giving PWAs access to more device features.
- Better discoverability: App stores are beginning to list PWAs alongside native apps, improving their visibility.
- Increased adoption: As more businesses recognize the benefits of PWAs, we’re likely to see increased adoption across various industries.
10. Conclusion
Progressive Web Apps represent a significant leap forward in web application development, offering the best of both web and native app worlds. By implementing features like offline access and push notifications, developers can create fast, reliable, and engaging web experiences that rival native applications.
As we’ve explored in this guide, building a PWA involves leveraging modern web technologies such as service workers, the Cache API, and the Web App Manifest. While there’s a learning curve involved, the benefits in terms of user engagement, cross-platform compatibility, and reduced development costs make PWAs an attractive option for many businesses.
As web technologies continue to evolve, we can expect PWAs to become even more powerful and widespread. Whether you’re a developer looking to enhance your skill set or a business owner seeking to improve your web presence, understanding and implementing PWAs is a valuable investment in the future of web development.
Remember, the journey to creating a successful PWA is an iterative process. Start small, focus on providing value to your users, and continuously improve your app based on feedback and performance metrics. With persistence and creativity, you can harness the power of PWAs to create web applications that truly stand out in today’s digital landscape.