How to Learn from Failed Interviews: Turning Setbacks into Stepping Stones
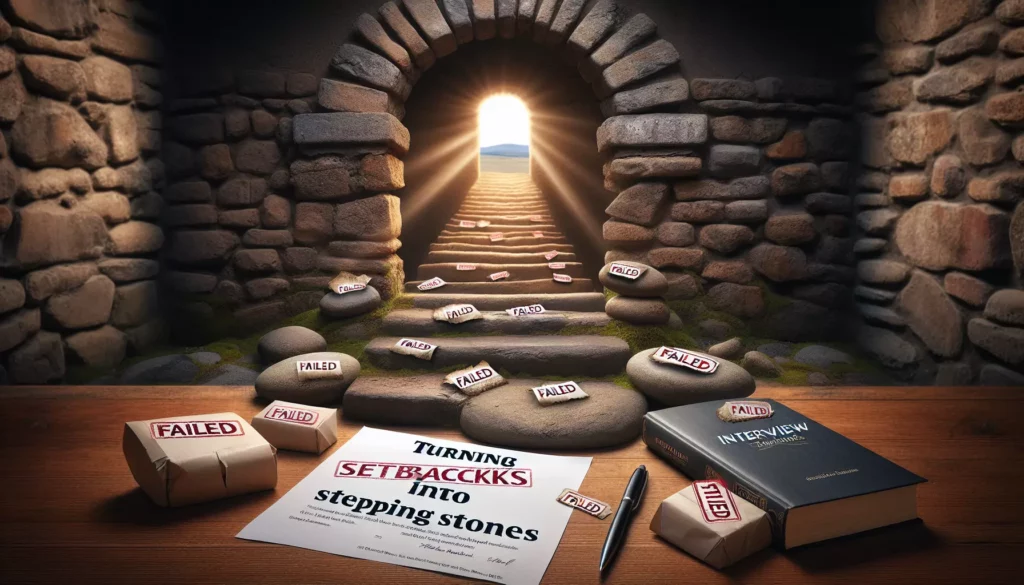
In the competitive world of tech, job interviews can be challenging, especially when aiming for positions at major companies like FAANG (Facebook, Amazon, Apple, Netflix, Google). While it’s natural to feel disappointed after a failed interview, it’s crucial to view these experiences as valuable learning opportunities. This article will guide you through the process of extracting meaningful lessons from unsuccessful interviews, helping you transform setbacks into stepping stones for future success.
1. The Importance of a Growth Mindset
Before diving into specific strategies, it’s essential to adopt a growth mindset. This concept, popularized by psychologist Carol Dweck, emphasizes the belief that abilities and intelligence can be developed through dedication and hard work. When approaching failed interviews with a growth mindset, you’re more likely to:
- View challenges as opportunities for learning
- Persist in the face of setbacks
- Learn from criticism and feedback
- Find inspiration in others’ success
By cultivating this mindset, you’ll be better equipped to extract valuable insights from your interview experiences, regardless of the outcome.
2. Immediate Post-Interview Reflection
The moments immediately following an interview are crucial for capturing fresh insights. Even if you feel the interview didn’t go well, take time to reflect and document your experience:
2.1 Write a Detailed Account
As soon as possible after the interview, write down everything you can remember:
- Questions asked
- Your responses
- Coding problems presented
- Your approach to solving them
- Feedback received (if any)
- Your overall impressions and feelings
This exercise serves two purposes: it helps you process the experience emotionally and provides a valuable record for future reference.
2.2 Identify Areas of Strength and Weakness
Review your account and objectively assess your performance:
- Which questions did you handle confidently?
- Where did you struggle or feel unprepared?
- Were there any technical concepts you found challenging?
- How was your communication throughout the interview?
This self-assessment will help you pinpoint areas for improvement and recognize your strengths.
3. Analyzing Technical Challenges
Technical interviews, especially for FAANG companies, often involve complex coding problems and algorithmic challenges. Here’s how to learn from these experiences:
3.1 Recreate the Problem
Try to recreate the coding problems you encountered during the interview. If you can’t remember the exact details, focus on the core concepts or similar problems. Use platforms like AlgoCademy to find and practice similar challenges.
3.2 Explore Multiple Solutions
Once you’ve recreated the problem, explore different approaches to solving it:
- Implement your original solution (if you had one)
- Research and implement alternative solutions
- Analyze the time and space complexity of each approach
- Consider edge cases and how to handle them
This process will deepen your understanding of the problem and expand your problem-solving toolkit.
3.3 Study Optimal Solutions
Research the most efficient solutions to the problems you encountered. Many coding platforms provide detailed explanations and optimal solutions. Study these carefully, understanding the reasoning behind each step. Here’s an example of how you might analyze an optimal solution for a common interview problem:
// Problem: Two Sum
// Given an array of integers nums and an integer target, return indices of the two numbers such that they add up to target.
function twoSum(nums, target) {
const map = new Map();
for (let i = 0; i < nums.length; i++) {
const complement = target - nums[i];
if (map.has(complement)) {
return [map.get(complement), i];
}
map.set(nums[i], i);
}
return [];
}
In this solution, we use a hash map to achieve O(n) time complexity, which is more efficient than the naive O(n^2) approach of using nested loops. Understanding such optimizations can significantly improve your problem-solving skills.
4. Improving Soft Skills
Technical prowess alone isn’t enough to succeed in interviews. Soft skills play a crucial role in how you present yourself and interact with interviewers. Here’s how to enhance these skills based on your interview experience:
4.1 Communication
Reflect on how effectively you communicated your thoughts and solutions during the interview:
- Did you clearly explain your problem-solving approach?
- Were you able to ask clarifying questions when needed?
- How well did you handle moments of uncertainty or confusion?
To improve, practice explaining complex concepts to others, participate in coding discussions, and consider joining a coding study group.
4.2 Handling Pressure
Technical interviews can be stressful. Analyze how you performed under pressure:
- Did anxiety affect your problem-solving abilities?
- Were you able to maintain composure when faced with difficult questions?
- How did you manage your time during coding challenges?
To build resilience, practice coding under timed conditions and simulate interview scenarios with friends or mentors.
4.3 Cultural Fit
FAANG companies often place significant emphasis on cultural fit. Reflect on how you presented yourself in this context:
- Did you effectively communicate your passion for technology and learning?
- Were you able to provide examples of teamwork and collaboration?
- How well did you align your responses with the company’s values and mission?
Research the culture of your target companies and practice framing your experiences in a way that highlights your fit.
5. Seeking and Utilizing Feedback
While not all companies provide detailed feedback after interviews, when available, it can be invaluable for your growth.
5.1 Requesting Feedback
If you haven’t received feedback, consider reaching out to your interviewer or the hiring manager. Be polite and express your genuine interest in improving:
Dear [Interviewer's Name],
I hope this email finds you well. I wanted to thank you again for the opportunity to interview for the [Position] role at [Company]. While I understand I wasn't selected to move forward, I'm committed to improving my skills for future opportunities.
If possible, I would greatly appreciate any feedback you could provide on my performance during the interview. Specific insights on areas where I could improve would be incredibly valuable for my professional development.
Thank you for your time and consideration.
Best regards,
[Your Name]
5.2 Analyzing Feedback
When you receive feedback, approach it with an open mind:
- Look for specific areas of improvement mentioned
- Compare the feedback with your self-assessment
- Identify any gaps between your perception and the interviewer’s assessment
5.3 Creating an Action Plan
Based on the feedback and your analysis, create a concrete action plan:
- List the key areas for improvement
- Set specific, measurable goals for each area
- Identify resources and strategies to address each goal
- Set a timeline for working on these improvements
For example, if the feedback suggests improving your knowledge of system design, your action plan might look like this:
Goal: Improve system design knowledge
Timeline: 3 months
Action items:
1. Read "Designing Data-Intensive Applications" by Martin Kleppmann
2. Complete AlgoCademy's system design course
3. Solve one system design problem per week on LeetCode
4. Participate in two system design discussions on tech forums monthly
5. Create a blog post explaining a complex system design concept
6. Expanding Your Knowledge Base
Failed interviews often reveal gaps in your knowledge or skills. Use these insights to guide your learning:
6.1 Identifying Knowledge Gaps
Review the questions or topics where you struggled during the interview. Common areas that candidates often need to improve include:
- Data structures and algorithms
- System design and scalability
- Specific programming languages or frameworks
- Computer science fundamentals (OS, networking, databases)
- Company-specific technologies or products
6.2 Structured Learning
Once you’ve identified areas for improvement, create a structured learning plan:
- Choose reputable learning resources (books, online courses, documentation)
- Set aside dedicated study time
- Break down complex topics into manageable chunks
- Use active learning techniques (practice problems, projects, teaching others)
- Regularly review and reinforce your learning
Platforms like AlgoCademy can be particularly helpful for structured learning, offering interactive tutorials and AI-powered assistance tailored to your skill level.
6.3 Practical Application
Theory alone isn’t enough. Apply your newfound knowledge through:
- Personal projects
- Open-source contributions
- Coding challenges and competitions
- Writing technical blog posts or creating tutorials
These activities not only reinforce your learning but also provide tangible evidence of your skills for future interviews.
7. Mock Interviews and Peer Review
Practicing with mock interviews is an excellent way to apply the lessons learned from failed interviews and prepare for future opportunities.
7.1 Conducting Mock Interviews
Arrange mock interviews with peers, mentors, or through online platforms:
- Simulate real interview conditions (time constraints, technical questions, behavioral assessments)
- Use a mix of familiar and unfamiliar problems
- Practice explaining your thought process out loud
- Experiment with different problem-solving approaches
7.2 Peer Code Reviews
Engage in peer code reviews to gain different perspectives on your coding style and problem-solving approaches:
- Share your solutions to interview-style problems with peers
- Review and provide feedback on others’ code
- Discuss alternative solutions and optimization techniques
- Practice explaining and defending your design decisions
7.3 Recording and Analyzing Performance
Consider recording your mock interviews (with permission) to analyze your performance:
- Review your communication style and body language
- Identify filler words or nervous habits to work on
- Assess the clarity and structure of your explanations
- Evaluate your time management during problem-solving
8. Building Resilience and Maintaining Motivation
Learning from failed interviews is as much about emotional resilience as it is about technical improvement. Here are strategies to stay motivated and build resilience:
8.1 Reframe Failure as Learning
Adopt a perspective that views each interview, successful or not, as a learning experience:
- Celebrate the courage it took to attempt the interview
- Acknowledge the specific skills or knowledge you gained from the process
- Recognize that even experienced developers face rejection
8.2 Set Incremental Goals
Break down your larger career goals into smaller, achievable milestones:
- Master one new algorithm or data structure each week
- Complete a certain number of coding challenges monthly
- Contribute to an open-source project quarterly
Celebrating these smaller victories helps maintain motivation and provides tangible signs of progress.
8.3 Cultivate a Support Network
Surround yourself with supportive peers and mentors:
- Join coding communities or study groups
- Attend tech meetups or conferences
- Share your journey with friends and family who can offer encouragement
8.4 Practice Self-Care
Remember that your worth isn’t defined by interview outcomes. Prioritize your well-being:
- Maintain a healthy work-life balance
- Engage in activities outside of coding that bring you joy
- Practice mindfulness or meditation to manage stress
- Celebrate your efforts and progress, not just outcomes
9. Preparing for Future Interviews
As you learn from past experiences, focus on strategic preparation for future interviews:
9.1 Targeted Company Research
For each company you’re interviewing with:
- Study their products, technologies, and recent developments
- Understand their company culture and values
- Research common interview questions specific to that company
- Prepare thoughtful questions to ask your interviewers
9.2 Customized Preparation
Tailor your preparation based on the lessons learned from previous interviews:
- Focus on strengthening areas where you previously struggled
- Practice explaining complex concepts in simple terms
- Prepare anecdotes that showcase your problem-solving skills and teamwork
- Develop a consistent method for approaching coding problems
9.3 Continuous Learning
Stay updated with the latest developments in your field:
- Follow tech blogs and news sources
- Experiment with new technologies or frameworks
- Participate in coding challenges or hackathons
- Contribute to open-source projects
10. Conclusion: Embracing the Journey
Learning from failed interviews is a crucial skill in the ever-evolving tech industry. By adopting a growth mindset, systematically analyzing your experiences, and continuously improving your skills, you transform each setback into a stepping stone towards your goals.
Remember that even the most successful developers have faced rejection and learned from it. Your journey is unique, and each interview, regardless of the outcome, brings you closer to your aspirations. Stay curious, remain persistent, and trust in your ability to grow and improve.
As you apply these strategies and continue your learning journey, platforms like AlgoCademy can provide valuable resources and support. With interactive tutorials, AI-assisted learning, and a wealth of practice problems, you’ll be well-equipped to tackle future interviews with confidence and skill.
Embrace each interview as an opportunity to learn, grow, and showcase your evolving skills. Your dedication to improvement will not only prepare you for success in future interviews but also contribute to your long-term growth as a skilled and adaptable developer.