How to Write Test Cases During Technical Interviews: A Comprehensive Guide
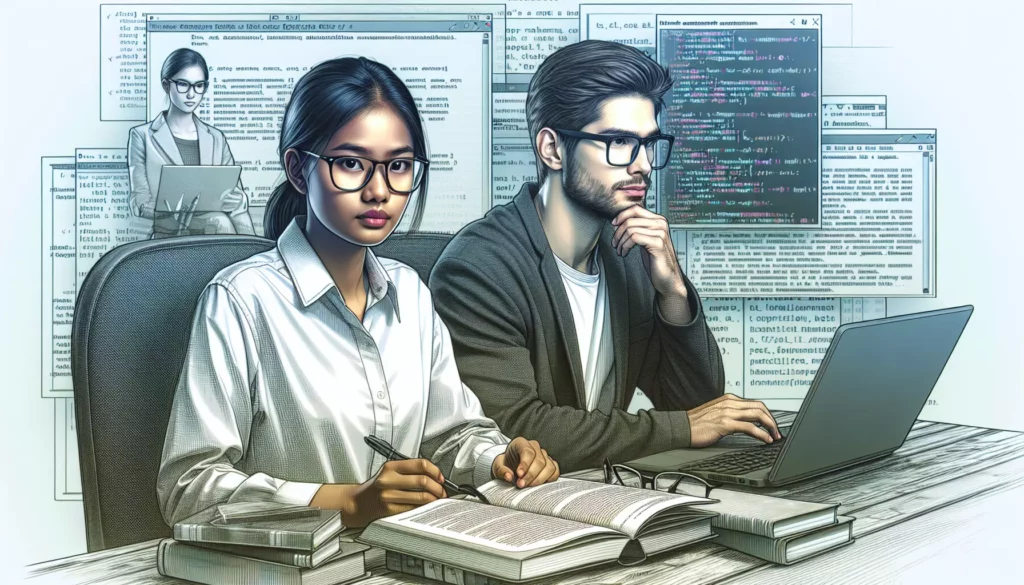
In the competitive world of software development, technical interviews are a crucial hurdle that every aspiring programmer must overcome. One of the most important skills that interviewers look for is the ability to write effective test cases. This skill demonstrates not only your coding proficiency but also your attention to detail, problem-solving abilities, and understanding of software quality assurance principles. In this comprehensive guide, we’ll explore the art of writing test cases during interviews, providing you with the tools and strategies you need to excel in this critical aspect of the interview process.
Why Test Cases Matter in Technical Interviews
Before we dive into the specifics of writing test cases, it’s essential to understand why they are so important in the context of technical interviews:
- Demonstrates thoroughness: Writing comprehensive test cases shows that you consider various scenarios and edge cases, not just the happy path.
- Reveals problem-solving skills: The process of creating test cases requires you to think critically about potential issues and how to address them.
- Indicates quality consciousness: By prioritizing testing, you show that you value producing high-quality, reliable code.
- Reflects real-world practices: In professional settings, writing test cases is a crucial part of the development process.
- Showcases communication skills: Clearly articulating test cases demonstrates your ability to convey technical concepts effectively.
The Anatomy of a Good Test Case
A well-structured test case typically includes the following components:
- Test case ID: A unique identifier for the test case.
- Test case description: A brief explanation of what the test is checking.
- Preconditions: Any necessary setup or initial state required for the test.
- Input data: The specific values or conditions being tested.
- Expected result: What should happen if the code is working correctly.
- Actual result: What actually happens when the test is run (to be filled in during execution).
- Pass/Fail status: Whether the test passed or failed based on the comparison of expected and actual results.
Strategies for Writing Effective Test Cases During Interviews
1. Understand the Problem Thoroughly
Before you start writing test cases, make sure you have a clear understanding of the problem at hand. Ask clarifying questions if needed, and don’t hesitate to discuss your assumptions with the interviewer.
2. Start with the Happy Path
Begin by writing test cases for the most common and straightforward scenarios. This establishes a baseline and demonstrates that you’re considering the primary functionality.
3. Consider Edge Cases
After covering the basic scenarios, think about potential edge cases. These might include:
- Boundary values (e.g., minimum and maximum allowed inputs)
- Empty or null inputs
- Very large or very small inputs
- Invalid inputs
- Unexpected data types
4. Test for Error Handling
Don’t forget to include test cases that verify how your code handles errors and exceptional situations. This shows that you’re thinking about robustness and user experience.
5. Use a Systematic Approach
Employ techniques like equivalence partitioning and boundary value analysis to systematically generate test cases. This demonstrates a methodical approach to testing.
6. Prioritize Test Cases
In an interview setting, time is limited. Prioritize your test cases based on their importance and the likelihood of uncovering issues. Start with the most critical tests and work your way down.
7. Be Concise but Clear
While it’s important to be thorough, remember that you’re working within time constraints. Be concise in your descriptions while ensuring they’re clear and unambiguous.
8. Explain Your Thought Process
As you write test cases, explain your reasoning to the interviewer. This gives them insight into your thought process and problem-solving approach.
Example: Writing Test Cases for a String Reversal Function
Let’s walk through an example of writing test cases for a function that reverses a string. Here’s the function signature:
function reverseString(input: string): string
Now, let’s write some test cases:
-
Test case ID: TC001
Description: Test reversing a simple string
Input: “hello”
Expected Output: “olleh” -
Test case ID: TC002
Description: Test reversing an empty string
Input: “”
Expected Output: “” -
Test case ID: TC003
Description: Test reversing a string with spaces
Input: “hello world”
Expected Output: “dlrow olleh” -
Test case ID: TC004
Description: Test reversing a palindrome
Input: “racecar”
Expected Output: “racecar” -
Test case ID: TC005
Description: Test reversing a string with special characters
Input: “Hello, World!”
Expected Output: “!dlroW ,olleH” -
Test case ID: TC006
Description: Test reversing a very long string
Input: “a” repeated 1,000,000 times
Expected Output: “a” repeated 1,000,000 times -
Test case ID: TC007
Description: Test handling of null input
Input: null
Expected Output: Error or exception (depending on language/implementation)
Common Pitfalls to Avoid
When writing test cases during interviews, be aware of these common mistakes:
- Overlooking edge cases: Don’t focus solely on the happy path. Consider unusual or extreme inputs.
- Ignoring performance: While functionality is crucial, also consider test cases that might reveal performance issues with large inputs.
- Being too verbose: While detail is important, avoid writing overly long or complicated test cases that take too much time to explain.
- Not considering the user perspective: Remember to include test cases that reflect real-world usage scenarios.
- Forgetting to test for security: If relevant to the problem, include test cases that check for potential security vulnerabilities.
Advanced Techniques for Test Case Writing
As you become more proficient in writing test cases, consider incorporating these advanced techniques:
1. Parameterized Testing
Instead of writing multiple similar test cases, use parameterized testing to run the same test with different inputs. This can be particularly useful for boundary value analysis.
@ParameterizedTest
@ValueSource(strings = {"hello", "world", "OpenAI", ""})
void testReverseString(String input) {
String reversed = reverseString(input);
assertEquals(new StringBuilder(input).reverse().toString(), reversed);
}
2. Property-Based Testing
Property-based testing involves defining properties that your function should always satisfy, rather than specific input-output pairs. For example, for our string reversal function:
@Property
void reverseTwiceIsIdentity(@ForAll String input) {
String reversed = reverseString(input);
String doubleReversed = reverseString(reversed);
assertEquals(input, doubleReversed);
}
3. Mutation Testing
While you might not have time to implement mutation testing during an interview, discussing it can demonstrate advanced knowledge. Mutation testing involves intentionally introducing bugs (mutations) into the code and ensuring that your tests catch these mutations.
4. Fuzzing
Fuzzing involves generating random, often malformed, inputs to test the robustness of your function. While you can’t perform extensive fuzzing during an interview, you can discuss how you might approach it:
void testFuzzing() {
Random rand = new Random();
for (int i = 0; i
Adapting to Different Interview Formats
The approach to writing test cases may vary depending on the interview format:
Whiteboard Interviews
In whiteboard interviews, you’ll need to write test cases by hand. Focus on clear, concise descriptions and cover the most important cases. Use a tabular format for clarity if possible.
Coding Interviews
During live coding interviews, you can often write and run actual unit tests. Take advantage of this to demonstrate your practical testing skills:
@Test
void testReverseString() {
assertEquals("olleh", reverseString("hello"));
assertEquals("", reverseString(""));
assertEquals("a", reverseString("a"));
assertEquals("!dlroW ,olleH", reverseString("Hello, World!"));
}
Take-Home Assignments
For take-home assignments, you have more time to be thorough. Include a comprehensive test suite that demonstrates your attention to detail and understanding of testing principles.
Conclusion
Writing effective test cases is a crucial skill for any software developer, and it’s particularly important during technical interviews. By following the strategies outlined in this guide, you can demonstrate your thoroughness, problem-solving abilities, and commitment to code quality.
Remember, the key to success lies in understanding the problem, considering various scenarios, and clearly communicating your thought process. Practice writing test cases for different types of problems, and you’ll be well-prepared to tackle this aspect of technical interviews with confidence.
As you continue to develop your skills, consider exploring more advanced testing techniques and staying up-to-date with best practices in the field. With dedication and practice, you’ll not only excel in interviews but also become a more effective and valuable software developer in your career.