Understanding the Importance of Edge Cases in Coding Problems
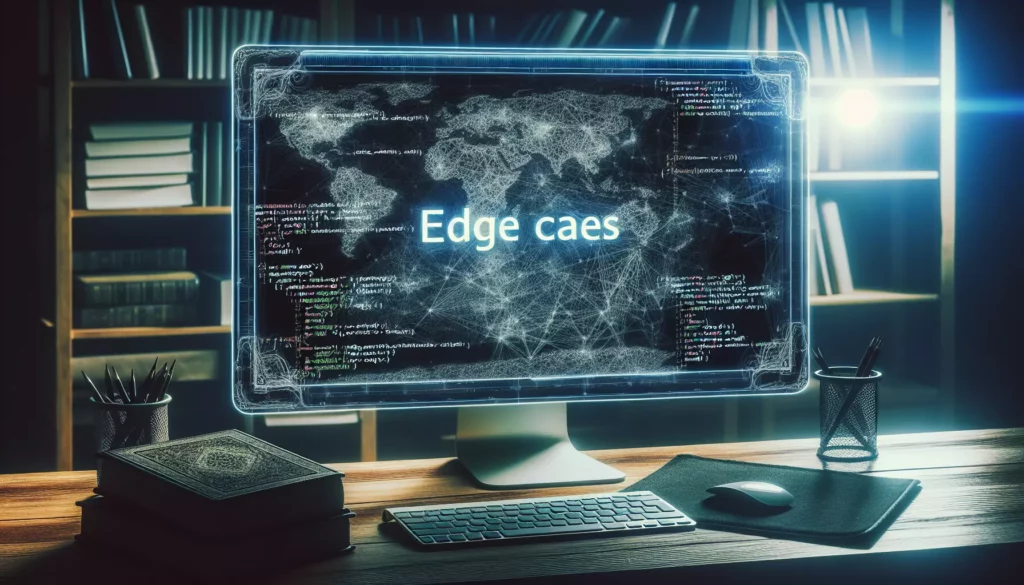
In the world of programming and software development, edge cases play a crucial role in ensuring the robustness and reliability of your code. As aspiring developers or seasoned professionals preparing for technical interviews at top tech companies, understanding and addressing edge cases is an essential skill that can set you apart from the competition. In this comprehensive guide, we’ll delve deep into the concept of edge cases, explore their significance in coding problems, and provide practical strategies for identifying and handling them effectively.
What Are Edge Cases?
Edge cases, also known as corner cases or boundary cases, are scenarios in which a program or algorithm is tested under extreme or unusual conditions. These situations often occur at the boundaries of input ranges or when dealing with exceptional or unexpected inputs. Edge cases are important because they can reveal hidden flaws or vulnerabilities in your code that might not be apparent during normal operation.
Consider the following examples of edge cases:
- Empty input (e.g., an empty string or array)
- Minimum or maximum possible values
- Negative numbers in a function expecting only positive inputs
- Null or undefined values
- Very large numbers that might cause overflow
- Inputs with special characters or formatting
Why Are Edge Cases Important?
Understanding and addressing edge cases is crucial for several reasons:
- Robustness: Handling edge cases makes your code more robust and less likely to fail under unexpected conditions.
- Reliability: By considering edge cases, you ensure that your program behaves correctly across a wide range of inputs.
- Bug Prevention: Identifying and addressing edge cases early in the development process can prevent bugs and errors that might otherwise go unnoticed until production.
- Security: Many security vulnerabilities arise from unhandled edge cases, making them a critical consideration for secure coding practices.
- Code Quality: Demonstrating attention to edge cases showcases your thoroughness and attention to detail as a programmer.
Common Types of Edge Cases
To better understand edge cases, let’s explore some common types you might encounter in coding problems:
1. Empty or Null Inputs
Empty or null inputs are classic edge cases that can cause unexpected behavior if not handled properly. For example, consider a function that calculates the average of an array of numbers:
function calculateAverage(numbers) {
let sum = 0;
for (let num of numbers) {
sum += num;
}
return sum / numbers.length;
}
This function works fine for non-empty arrays, but what happens if we pass an empty array? It will return NaN (Not a Number) because we’re dividing by zero. To handle this edge case, we could modify the function as follows:
function calculateAverage(numbers) {
if (numbers.length === 0) {
return 0; // or throw an error, depending on the requirements
}
let sum = 0;
for (let num of numbers) {
sum += num;
}
return sum / numbers.length;
}
2. Boundary Values
Boundary values are inputs at the extreme ends of the expected range. For instance, if a function expects an integer between 1 and 100, the boundary values would be 1, 100, 0, and 101. Testing these values can reveal off-by-one errors or incorrect handling of out-of-range inputs.
Consider a function that determines whether a year is a leap year:
function isLeapYear(year) {
return (year % 4 === 0 && year % 100 !== 0) || (year % 400 === 0);
}
While this function works for most inputs, it doesn’t handle negative years or years before the Gregorian calendar was introduced (1582). To improve it, we could add checks for these edge cases:
function isLeapYear(year) {
if (year < 1582) {
throw new Error("Year must be 1582 or later");
}
return (year % 4 === 0 && year % 100 !== 0) || (year % 400 === 0);
}
3. Large Inputs
Large inputs can reveal performance issues or cause unexpected behavior due to integer overflow or memory limitations. For example, consider a function that calculates the factorial of a number:
function factorial(n) {
if (n === 0 || n === 1) {
return 1;
}
return n * factorial(n - 1);
}
This function works well for small inputs, but for large numbers, it can cause a stack overflow due to excessive recursion. Additionally, it doesn’t handle negative numbers or non-integer inputs. We can improve it as follows:
function factorial(n) {
if (!Number.isInteger(n) || n < 0) {
throw new Error("Input must be a non-negative integer");
}
if (n === 0 || n === 1) {
return 1;
}
let result = 1;
for (let i = 2; i <= n; i++) {
result *= i;
if (!Number.isFinite(result)) {
throw new Error("Result too large to represent");
}
}
return result;
}
4. Special Characters and Formatting
When dealing with string inputs, special characters or unexpected formatting can lead to errors if not handled properly. Consider a function that reverses a string:
function reverseString(str) {
return str.split('').reverse().join('');
}
This function works for most inputs, but it doesn’t handle Unicode surrogate pairs correctly. For instance, emoji characters might be split incorrectly. A more robust version would be:
function reverseString(str) {
return [...str].reverse().join('');
}
Strategies for Identifying Edge Cases
Developing a systematic approach to identifying edge cases is crucial for writing robust code and excelling in technical interviews. Here are some strategies to help you spot potential edge cases:
1. Analyze Input Ranges
Consider the full range of possible inputs for your function or algorithm. Ask yourself:
- What are the minimum and maximum values allowed?
- Are there any restrictions on the input type or format?
- How should the function handle inputs outside the expected range?
2. Consider Empty or Null Inputs
Always think about how your code should behave when given empty or null inputs. This includes:
- Empty strings or arrays
- Null or undefined values
- Objects with missing properties
3. Think About Data Types
If your function expects a certain data type, consider what happens when it receives inputs of different types. For example:
- Passing a string to a function expecting a number
- Providing a float when an integer is expected
- Handling objects or arrays when primitive types are expected
4. Visualize Extreme Scenarios
Try to imagine the most extreme or unusual scenarios in which your code might be used. This could include:
- Very large or very small inputs
- Inputs with unusual patterns or structures
- Scenarios where multiple edge cases occur simultaneously
5. Use Test-Driven Development (TDD)
Adopting a test-driven development approach can help you identify edge cases early in the development process. By writing tests before implementing the code, you’re forced to consider various scenarios, including edge cases.
Handling Edge Cases Effectively
Once you’ve identified potential edge cases, it’s important to handle them effectively in your code. Here are some best practices for dealing with edge cases:
1. Input Validation
Implement thorough input validation at the beginning of your functions to catch and handle unexpected inputs early. This can include:
- Checking for null or undefined values
- Verifying that inputs are within the expected range
- Ensuring that inputs are of the correct type
Example:
function calculateSquareRoot(num) {
if (typeof num !== 'number' || isNaN(num)) {
throw new Error('Input must be a valid number');
}
if (num < 0) {
throw new Error('Cannot calculate square root of a negative number');
}
return Math.sqrt(num);
}
2. Defensive Programming
Adopt a defensive programming mindset by anticipating and guarding against potential errors. This includes:
- Using try-catch blocks to handle exceptions
- Providing default values or fallback behavior for unexpected scenarios
- Implementing checks throughout your code to ensure data integrity
Example:
function getFirstElement(arr) {
try {
if (!Array.isArray(arr)) {
throw new Error('Input must be an array');
}
return arr.length > 0 ? arr[0] : null;
} catch (error) {
console.error('Error:', error.message);
return null;
}
}
3. Clear Documentation
Document your functions and methods clearly, specifying:
- Expected input types and ranges
- Behavior for edge cases
- Any exceptions that might be thrown
Example:
/**
* Calculates the average of an array of numbers.
* @param {number[]} numbers - An array of numbers.
* @returns {number} The average of the input numbers.
* @throws {Error} If the input is not an array or if the array is empty.
*/
function calculateAverage(numbers) {
if (!Array.isArray(numbers)) {
throw new Error('Input must be an array');
}
if (numbers.length === 0) {
throw new Error('Array must not be empty');
}
const sum = numbers.reduce((acc, num) => acc + num, 0);
return sum / numbers.length;
}
4. Graceful Degradation
Design your code to gracefully handle unexpected situations by providing reasonable default behavior or partial functionality when possible. This approach can improve the user experience and prevent complete system failures.
Example:
function fetchUserData(userId) {
return fetch(`/api/users/${userId}`)
.then(response => response.json())
.catch(error => {
console.error('Error fetching user data:', error);
return { id: userId, name: 'Unknown User', error: true };
});
}
Edge Cases in Technical Interviews
Understanding and addressing edge cases is particularly important in technical interviews, especially when applying to top tech companies. Interviewers often use edge cases to assess a candidate’s problem-solving skills, attention to detail, and ability to write robust code. Here are some tips for handling edge cases during interviews:
1. Clarify Requirements
Before diving into a solution, ask clarifying questions about the expected behavior for edge cases. This demonstrates your thoughtfulness and helps you avoid making incorrect assumptions.
2. Think Aloud
As you consider possible edge cases, verbalize your thought process. This gives the interviewer insight into your problem-solving approach and allows them to provide guidance if needed.
3. Start with a Naive Solution
Begin with a simple solution that works for the main cases, then iterate and improve it to handle edge cases. This approach shows your ability to write working code quickly and then refine it.
4. Test Your Code
After implementing your solution, walk through it with various test cases, including edge cases. This demonstrates your testing mindset and ability to catch potential issues.
5. Discuss Trade-offs
If time allows, discuss the trade-offs between different approaches to handling edge cases, such as performance implications or code complexity.
Conclusion
Understanding and effectively handling edge cases is a crucial skill for any programmer, whether you’re a beginner learning the ropes or an experienced developer preparing for technical interviews at top tech companies. By developing a systematic approach to identifying and addressing edge cases, you can write more robust, reliable, and secure code.
Remember that dealing with edge cases is not just about preventing errors; it’s about creating software that gracefully handles unexpected situations and provides a better user experience. As you continue to practice and refine your coding skills, make edge case analysis an integral part of your problem-solving process.
By mastering the art of handling edge cases, you’ll not only improve your coding abilities but also demonstrate the kind of thorough, thoughtful approach that top tech companies value in their engineers. So, the next time you’re faced with a coding problem, take a moment to consider those edge cases – your code (and your future interviewers) will thank you for it!