Approaching Optimization Problems Creatively: Mastering Algorithmic Thinking
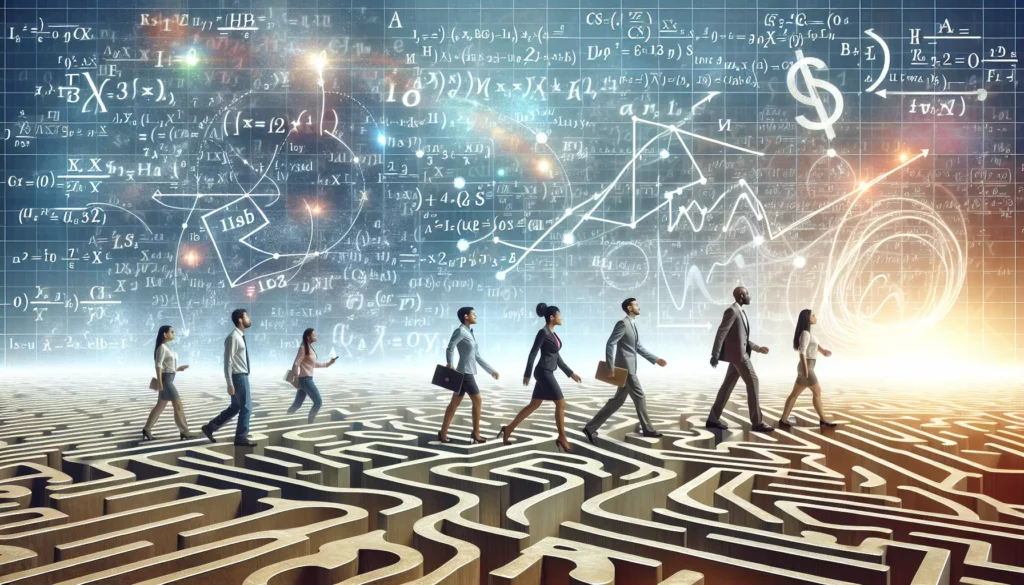
In the ever-evolving world of programming and software development, the ability to approach optimization problems creatively is a crucial skill that sets apart exceptional developers from the rest. As we delve into this topic, we’ll explore various strategies, techniques, and mindsets that can help you tackle complex optimization challenges with ingenuity and efficiency. Whether you’re a beginner looking to enhance your problem-solving skills or an experienced coder preparing for technical interviews at top tech companies, this comprehensive guide will equip you with the tools you need to excel in algorithmic thinking and optimization.
Understanding Optimization Problems
Before we dive into creative approaches, it’s essential to understand what optimization problems are and why they’re important in the world of programming.
What are Optimization Problems?
Optimization problems involve finding the best solution from all feasible solutions. In programming, this often translates to improving the efficiency of an algorithm or a piece of code in terms of time complexity, space complexity, or both. The goal is to minimize or maximize certain aspects of the program, such as:
- Execution time
- Memory usage
- Resource utilization
- Output quality
- Cost-effectiveness
Why are Optimization Problems Important?
In today’s competitive tech landscape, optimization is crucial for several reasons:
- Performance: Optimized code runs faster and more efficiently, providing a better user experience.
- Scalability: Well-optimized algorithms can handle larger datasets and more users without significant performance degradation.
- Cost-effectiveness: Efficient code requires fewer resources, leading to lower operational costs for businesses.
- Energy efficiency: Optimized programs consume less power, which is especially important for mobile and IoT devices.
- Competitive advantage: Companies that can deliver faster, more efficient solutions have an edge in the market.
Creative Approaches to Optimization
Now that we understand the importance of optimization, let’s explore some creative approaches to tackle these problems effectively.
1. Reframe the Problem
One of the most powerful techniques in creative problem-solving is to reframe the problem. This involves looking at the issue from a different perspective or changing the context in which you view it. Here are some ways to reframe optimization problems:
- Inverse thinking: Instead of asking how to make something faster, ask what’s making it slow.
- Analogies: Draw parallels with similar problems in other domains or real-life situations.
- Extreme cases: Consider what would happen if the input size was infinitely large or infinitesimally small.
- Different dimensions: Look at the problem in terms of time, space, or other relevant dimensions.
For example, when optimizing a sorting algorithm, instead of focusing solely on comparison-based methods, you might reframe the problem by asking, “What if we didn’t need to compare elements at all?” This line of thinking could lead you to explore non-comparison sorting algorithms like counting sort or radix sort for specific input types.
2. Break Down the Problem
Complex optimization problems can often be overwhelming. Breaking them down into smaller, more manageable sub-problems can reveal new insights and opportunities for optimization. This approach, known as “divide and conquer,” is not only a problem-solving strategy but also forms the basis of many efficient algorithms.
Steps to effectively break down a problem:
- Identify the main components or stages of the problem.
- Analyze each component independently.
- Look for patterns or commonalities among the components.
- Optimize each part separately.
- Combine the optimized parts and analyze the overall improvement.
For instance, when optimizing a complex data processing pipeline, you might break it down into data input, transformation, analysis, and output stages. By focusing on each stage individually, you can apply specific optimization techniques tailored to that particular operation.
3. Visualize the Problem
Visual thinking can be a powerful tool in approaching optimization problems creatively. By representing the problem or algorithm visually, you can often spot patterns, bottlenecks, or optimization opportunities that might not be apparent in code or pseudocode.
Ways to visualize optimization problems:
- Flowcharts: Represent the algorithm’s logic and decision points.
- Data structure diagrams: Visualize how data is stored and accessed.
- Time complexity graphs: Plot the algorithm’s performance against input size.
- Memory usage diagrams: Illustrate how memory is allocated and deallocated.
- State transition diagrams: Show how the program moves between different states.
For example, when optimizing a graph algorithm, drawing out the graph and tracing the algorithm’s path can help you identify redundant operations or opportunities for parallelization.
4. Apply Cross-Disciplinary Knowledge
Some of the most innovative solutions come from applying knowledge from seemingly unrelated fields. By drawing inspiration from various disciplines, you can bring fresh perspectives to optimization problems.
Areas that can inspire optimization techniques:
- Biology: Genetic algorithms and neural networks inspired by biological processes.
- Physics: Simulated annealing inspired by thermodynamics.
- Economics: Game theory for optimizing decision-making algorithms.
- Mathematics: Linear programming and other mathematical optimization techniques.
- Psychology: Understanding cognitive biases to optimize user interfaces and experiences.
For instance, the PageRank algorithm, which revolutionized web search, was inspired by academic citation analysis from the field of bibliometrics.
5. Challenge Assumptions
Many optimization problems come with implicit assumptions that limit our thinking. By actively challenging these assumptions, you can open up new avenues for optimization.
Common assumptions to challenge:
- The current algorithm is the best approach.
- All data needs to be processed sequentially.
- The entire dataset must be held in memory.
- Computations must be exact (sometimes approximations are sufficient).
- The problem can’t be solved in a distributed manner.
For example, when optimizing a search algorithm, you might challenge the assumption that all searches start from scratch. This could lead to implementing caching mechanisms or precomputing certain results to speed up subsequent searches.
Practical Techniques for Creative Optimization
Now that we’ve explored some high-level approaches, let’s dive into specific techniques you can use to optimize your code creatively.
1. Memoization and Caching
Memoization is a technique where you store the results of expensive function calls and return the cached result when the same inputs occur again. This can dramatically improve performance for functions with repetitive calculations.
Here’s a simple example of memoization in Python:
def memoize(func):
cache = {}
def memoized(*args):
if args in cache:
return cache[args]
result = func(*args)
cache[args] = result
return result
return memoized
@memoize
def fibonacci(n):
if n < 2:
return n
return fibonacci(n-1) + fibonacci(n-2)
print(fibonacci(100)) # This will be much faster than without memoization
2. Lazy Evaluation
Lazy evaluation is a strategy where you delay the evaluation of an expression until its value is needed. This can save computational resources and improve performance, especially when dealing with large datasets or infinite sequences.
Python’s generators are a great example of lazy evaluation:
def infinite_sequence():
num = 0
while True:
yield num
num += 1
gen = infinite_sequence()
print(next(gen)) # 0
print(next(gen)) # 1
# The sequence is generated on-demand, not all at once
3. Parallel Processing
With the prevalence of multi-core processors, parallel processing has become an essential optimization technique. By dividing work across multiple cores or machines, you can significantly reduce execution time for certain types of problems.
Here’s a simple example using Python’s multiprocessing module:
from multiprocessing import Pool
def f(x):
return x*x
if __name__ == '__main__':
with Pool(5) as p:
print(p.map(f, [1, 2, 3, 4, 5]))
4. Space-Time Tradeoffs
Sometimes, you can trade memory for speed or vice versa. This involves carefully analyzing your algorithm to see if you can use more memory to reduce computation time, or if you can sacrifice some speed to reduce memory usage.
For example, using a hash table to store intermediate results can often speed up algorithms at the cost of increased memory usage:
def two_sum(nums, target):
seen = {}
for i, num in enumerate(nums):
complement = target - num
if complement in seen:
return [seen[complement], i]
seen[num] = i
return [] # No solution found
5. Approximate Algorithms
For some problems, finding an exact solution might be too computationally expensive. In such cases, approximate algorithms that provide a “good enough” solution can be a creative way to optimize.
For instance, when dealing with large graphs, algorithms like the PageRank can be approximated:
import numpy as np
def approximate_pagerank(graph, damping_factor=0.85, epsilon=1e-8, max_iterations=100):
n = len(graph)
r = np.ones(n) / n
for _ in range(max_iterations):
r_new = (1 - damping_factor) / n + damping_factor * graph.T.dot(r)
if np.sum(np.abs(r_new - r)) < epsilon:
return r_new
r = r_new
return r
Case Studies: Creative Optimization in Action
To better understand how these creative approaches and techniques can be applied in real-world scenarios, let’s examine a few case studies of optimization problems and their innovative solutions.
Case Study 1: Google’s MapReduce
Problem: Processing and analyzing massive datasets efficiently.
Creative Solution: Google engineers Jeffrey Dean and Sanjay Ghemawat developed MapReduce, a programming model inspired by functional programming concepts. MapReduce breaks down large data processing tasks into smaller, distributable units that can be processed in parallel across many machines.
Key Innovations:
- Reframing the problem of large-scale data processing as a series of map and reduce operations.
- Applying functional programming concepts to distributed computing.
- Automating the parallelization, distribution, and fault-tolerance aspects of large-scale computations.
Impact: MapReduce revolutionized big data processing and laid the groundwork for modern big data frameworks like Hadoop and Spark.
Case Study 2: Bloom Filters
Problem: Quickly determining whether an element is a member of a set, with minimal memory usage.
Creative Solution: Burton Howard Bloom invented the Bloom filter, a space-efficient probabilistic data structure that uses multiple hash functions to test whether an element is a member of a set.
Key Innovations:
- Trading absolute certainty for probabilistic results to save space.
- Using multiple hash functions to reduce false positives.
- Allowing for a controllable rate of false positives in exchange for extreme space efficiency.
Impact: Bloom filters are widely used in databases, caching systems, and network routing tables to optimize memory usage and lookup times.
Case Study 3: Fast Inverse Square Root
Problem: Calculating the inverse square root quickly for 3D graphics rendering.
Creative Solution: Developers at id Software created an algorithm that uses a clever bit manipulation trick to approximate the inverse square root much faster than traditional methods.
Key Innovations:
- Reframing the mathematical problem as a bit manipulation problem.
- Exploiting the IEEE 754 floating-point representation.
- Trading some accuracy for significant speed improvements.
Impact: This algorithm significantly improved the performance of 3D graphics rendering in games and other applications.
Developing Your Creative Optimization Skills
Becoming proficient at creative optimization is a journey that requires practice, patience, and continuous learning. Here are some strategies to help you develop and hone your skills:
1. Study Classic Algorithms and Data Structures
Understanding fundamental algorithms and data structures provides a strong foundation for creative optimization. Familiarize yourself with:
- Sorting algorithms (quicksort, mergesort, heapsort)
- Search algorithms (binary search, depth-first search, breadth-first search)
- Data structures (arrays, linked lists, trees, graphs, hash tables)
- Dynamic programming techniques
- Greedy algorithms
Resources like “Introduction to Algorithms” by Cormen, Leiserson, Rivest, and Stein (often called CLRS) can provide a comprehensive understanding of these topics.
2. Practice Problem-Solving Regularly
Engage in regular problem-solving exercises to sharpen your skills. Platforms like LeetCode, HackerRank, and Project Euler offer a wide range of algorithmic problems to solve. When practicing:
- Start with easier problems and gradually increase difficulty.
- Try to solve problems in multiple ways.
- Analyze and understand solutions proposed by others.
- Focus on optimizing your solutions after getting them to work correctly.
3. Analyze Existing Systems and Codebases
Studying how existing systems and codebases handle optimization can provide valuable insights. Some ways to do this:
- Contribute to open-source projects and review their optimization strategies.
- Read technical blogs and case studies from major tech companies.
- Analyze the source code of popular libraries and frameworks.
4. Experiment with Different Programming Paradigms
Different programming paradigms can offer unique perspectives on problem-solving and optimization. Explore:
- Functional programming (e.g., Haskell, Scala)
- Logic programming (e.g., Prolog)
- Concurrent programming
- Data-oriented design
5. Learn from Other Disciplines
As mentioned earlier, cross-disciplinary knowledge can spark creative solutions. Consider studying:
- Discrete mathematics and graph theory
- Operations research
- Machine learning and artificial intelligence
- Systems design and architecture
6. Use Profiling and Benchmarking Tools
Profiling tools can help you identify bottlenecks and optimization opportunities in your code. Familiarize yourself with:
- Language-specific profilers (e.g., cProfile for Python, JProfiler for Java)
- Memory profiling tools
- Benchmarking frameworks
7. Participate in Coding Competitions
Coding competitions can push you to think creatively under time pressure. Platforms like Codeforces, TopCoder, and Google Code Jam offer regular competitions.
8. Collaborate and Discuss with Others
Engaging with other developers can expose you to new ideas and approaches. Consider:
- Joining coding meetups or study groups
- Participating in online forums and communities
- Pair programming with colleagues or friends
Conclusion
Approaching optimization problems creatively is a skill that can significantly enhance your capabilities as a programmer and problem solver. By reframing problems, breaking them down, visualizing solutions, applying cross-disciplinary knowledge, and challenging assumptions, you can uncover innovative ways to improve the efficiency and performance of your code.
Remember that creative optimization is not just about applying a set of techniques; it’s about developing a mindset that constantly seeks improvement and questions the status quo. As you continue to practice and refine your skills, you’ll find that creative optimization becomes an integral part of your problem-solving toolkit, enabling you to tackle even the most complex challenges with confidence and ingenuity.
Whether you’re preparing for technical interviews at top tech companies or working on large-scale software projects, the ability to approach optimization problems creatively will set you apart and drive innovation in your work. Embrace the challenge, stay curious, and never stop exploring new ways to optimize and improve your code.