Understanding Sorting Algorithms: A Comprehensive Guide to Quicksort, Mergesort, and Beyond
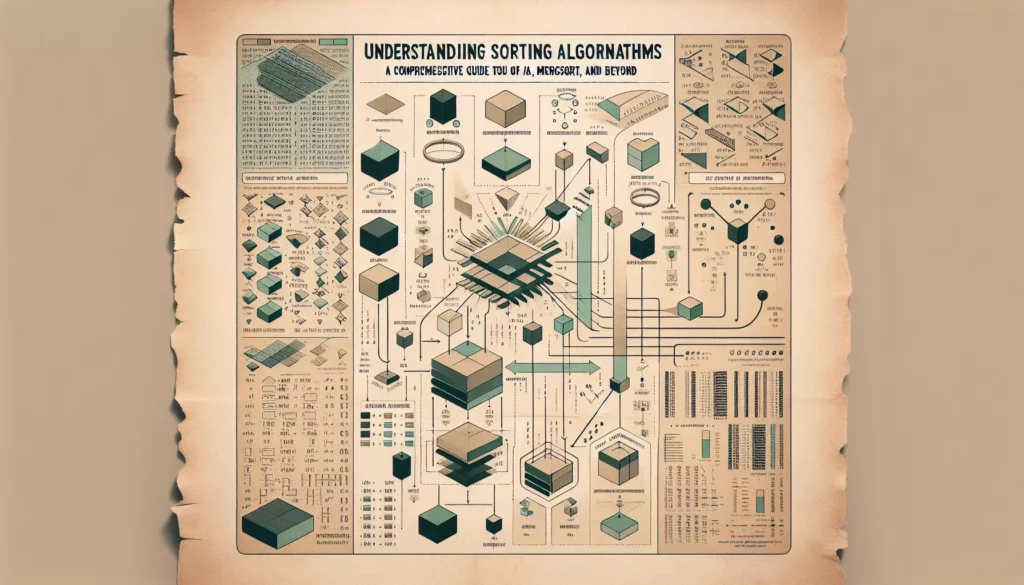
In the world of computer science and programming, sorting algorithms play a crucial role in organizing data efficiently. Whether you’re a beginner coder or preparing for technical interviews at major tech companies, understanding these fundamental algorithms is essential. In this comprehensive guide, we’ll dive deep into the world of sorting algorithms, with a particular focus on two popular and efficient methods: Quicksort and Mergesort.
Why Are Sorting Algorithms Important?
Before we delve into the specifics of different sorting algorithms, it’s crucial to understand why they are so important in computer science and programming:
- Efficiency: Sorting algorithms help organize data in a way that makes subsequent operations, such as searching, much faster and more efficient.
- Data Analysis: Sorted data is easier to analyze, compare, and process, making it invaluable in various applications, from databases to data science.
- Problem-Solving: Understanding sorting algorithms enhances your problem-solving skills and algorithmic thinking, which are crucial for coding interviews and real-world programming challenges.
- Foundation for Other Algorithms: Many advanced algorithms and data structures rely on sorted data as a prerequisite.
Common Sorting Algorithms
While we’ll focus primarily on Quicksort and Mergesort in this article, it’s worth mentioning some other common sorting algorithms:
- Bubble Sort
- Selection Sort
- Insertion Sort
- Heap Sort
- Radix Sort
Each of these algorithms has its own strengths, weaknesses, and use cases. However, Quicksort and Mergesort are particularly popular due to their efficiency and wide applicability.
Deep Dive: Quicksort
Quicksort is a highly efficient, divide-and-conquer sorting algorithm. It’s widely used due to its average-case time complexity of O(n log n) and its ability to sort in place, which saves memory.
How Quicksort Works
- Choose a pivot: Select an element from the array to serve as a pivot.
- Partitioning: Rearrange the array so that all elements smaller than the pivot come before it, and all elements larger come after it.
- Recursion: Recursively apply the above steps to the sub-array of elements with smaller values and the sub-array of elements with larger values.
Quicksort Implementation in Python
Here’s a basic implementation of Quicksort in Python:
def quicksort(arr):
if len(arr) <= 1:
return arr
else:
pivot = arr[len(arr) // 2]
left = [x for x in arr if x < pivot]
middle = [x for x in arr if x == pivot]
right = [x for x in arr if x > pivot]
return quicksort(left) + middle + quicksort(right)
# Example usage
arr = [3, 6, 8, 10, 1, 2, 1]
sorted_arr = quicksort(arr)
print(sorted_arr) # Output: [1, 1, 2, 3, 6, 8, 10]
Advantages of Quicksort
- Efficient for large datasets
- In-place sorting (doesn’t require extra memory)
- Works well with virtual memory
Disadvantages of Quicksort
- Worst-case time complexity of O(n^2) when poorly implemented
- Not stable (doesn’t preserve the relative order of equal elements)
Deep Dive: Mergesort
Mergesort is another efficient, divide-and-conquer algorithm. It consistently performs with a time complexity of O(n log n), making it reliable across various input scenarios.
How Mergesort Works
- Divide: Recursively divide the unsorted list into n sublists, each containing one element (a list of one element is considered sorted).
- Conquer: Repeatedly merge sublists to produce new sorted sublists until there is only one sublist remaining. This will be the sorted list.
Mergesort Implementation in Python
Here’s a basic implementation of Mergesort in Python:
def merge_sort(arr):
if len(arr) <= 1:
return arr
mid = len(arr) // 2
left = merge_sort(arr[:mid])
right = merge_sort(arr[mid:])
return merge(left, right)
def merge(left, right):
result = []
i, j = 0, 0
while i < len(left) and j < len(right):
if left[i] <= right[j]:
result.append(left[i])
i += 1
else:
result.append(right[j])
j += 1
result.extend(left[i:])
result.extend(right[j:])
return result
# Example usage
arr = [38, 27, 43, 3, 9, 82, 10]
sorted_arr = merge_sort(arr)
print(sorted_arr) # Output: [3, 9, 10, 27, 38, 43, 82]
Advantages of Mergesort
- Stable sorting algorithm
- Guaranteed O(n log n) time complexity in all cases
- Well-suited for external sorting (when data doesn’t fit in memory)
Disadvantages of Mergesort
- Requires additional O(n) space complexity
- Slower for smaller tasks compared to other algorithms like insertion sort
Comparing Quicksort and Mergesort
While both Quicksort and Mergesort are efficient sorting algorithms, they have some key differences:
Aspect | Quicksort | Mergesort |
---|---|---|
Average Time Complexity | O(n log n) | O(n log n) |
Worst-case Time Complexity | O(n^2) | O(n log n) |
Space Complexity | O(log n) | O(n) |
Stability | Not stable | Stable |
In-place Sorting | Yes | No |
Other Important Sorting Algorithms
While Quicksort and Mergesort are among the most popular sorting algorithms, it’s important to be familiar with other sorting methods as well. Let’s briefly explore some of these:
1. Bubble Sort
Bubble Sort is a simple sorting algorithm that repeatedly steps through the list, compares adjacent elements and swaps them if they are in the wrong order. While it’s not efficient for large lists, it’s easy to understand and implement.
def bubble_sort(arr):
n = len(arr)
for i in range(n):
for j in range(0, n-i-1):
if arr[j] > arr[j+1]:
arr[j], arr[j+1] = arr[j+1], arr[j]
return arr
# Example usage
arr = [64, 34, 25, 12, 22, 11, 90]
sorted_arr = bubble_sort(arr)
print(sorted_arr) # Output: [11, 12, 22, 25, 34, 64, 90]
2. Insertion Sort
Insertion Sort builds the final sorted array one item at a time. It’s much less efficient on large lists than more advanced algorithms such as Quicksort, Heapsort, or Merge Sort. However, it performs well for small datasets and is often used as part of more sophisticated algorithms.
def insertion_sort(arr):
for i in range(1, len(arr)):
key = arr[i]
j = i-1
while j >= 0 and key < arr[j]:
arr[j+1] = arr[j]
j -= 1
arr[j+1] = key
return arr
# Example usage
arr = [12, 11, 13, 5, 6]
sorted_arr = insertion_sort(arr)
print(sorted_arr) # Output: [5, 6, 11, 12, 13]
3. Heap Sort
Heap Sort is a comparison-based sorting algorithm that uses a binary heap data structure. It’s similar to Selection Sort where we first find the minimum element and place it at the beginning. We repeat this process for the remaining elements.
def heapify(arr, n, i):
largest = i
l = 2 * i + 1
r = 2 * i + 2
if l < n and arr[i] < arr[l]:
largest = l
if r < n and arr[largest] < arr[r]:
largest = r
if largest != i:
arr[i], arr[largest] = arr[largest], arr[i]
heapify(arr, n, largest)
def heap_sort(arr):
n = len(arr)
for i in range(n // 2 - 1, -1, -1):
heapify(arr, n, i)
for i in range(n-1, 0, -1):
arr[i], arr[0] = arr[0], arr[i]
heapify(arr, i, 0)
return arr
# Example usage
arr = [12, 11, 13, 5, 6, 7]
sorted_arr = heap_sort(arr)
print(sorted_arr) # Output: [5, 6, 7, 11, 12, 13]
Choosing the Right Sorting Algorithm
Selecting the appropriate sorting algorithm depends on various factors:
- Size of the dataset: For small datasets, simpler algorithms like Insertion Sort might be faster. For larger datasets, more complex algorithms like Quicksort or Mergesort are typically more efficient.
- Memory constraints: If memory is a concern, in-place sorting algorithms like Quicksort might be preferable.
- Stability requirements: If maintaining the relative order of equal elements is important, stable sorting algorithms like Mergesort should be used.
- Data distribution: Some algorithms perform better or worse depending on how the data is initially distributed.
- Time complexity guarantees: If consistent performance is crucial, algorithms with guaranteed O(n log n) performance like Mergesort might be preferred over algorithms with variable performance like Quicksort.
Sorting Algorithms in Practice
Understanding sorting algorithms is not just an academic exercise. These algorithms are widely used in real-world applications:
- Database Systems: Efficient sorting is crucial for database operations, especially for indexing and query optimization.
- File Systems: Operating systems use sorting algorithms to organize and retrieve files efficiently.
- Search Engines: Sorting is fundamental in ranking and presenting search results.
- Data Analysis and Visualization: Sorting helps in organizing data for analysis and creating meaningful visualizations.
- Compression Algorithms: Some compression techniques rely on sorting data before applying compression.
Advanced Topics in Sorting
As you progress in your understanding of sorting algorithms, you might want to explore some advanced topics:
1. Parallel Sorting Algorithms
With the rise of multi-core processors and distributed systems, parallel sorting algorithms have become increasingly important. These algorithms distribute the sorting task across multiple processors or machines to achieve faster sorting times for very large datasets.
2. External Sorting
External sorting algorithms are used when the data being sorted does not fit into the main memory of a computing device and instead must reside in slower external memory, typically a hard drive. Mergesort is often used as a basis for external sorting algorithms.
3. Hybrid Sorting Algorithms
Hybrid algorithms combine two or more sorting techniques to leverage their respective strengths. For example, Introsort starts with Quicksort and switches to Heapsort if the recursion depth exceeds a certain level, thus avoiding Quicksort’s worst-case scenario.
Preparing for Coding Interviews
If you’re preparing for technical interviews, especially at major tech companies, understanding sorting algorithms is crucial. Here are some tips:
- Know the basics: Be able to implement common sorting algorithms from scratch.
- Understand time and space complexity: Know the best, average, and worst-case scenarios for different algorithms.
- Practice variations: Be prepared to modify sorting algorithms for specific scenarios, like sorting in reverse order or sorting objects based on multiple criteria.
- Consider edge cases: Think about how your sorting algorithm would handle empty arrays, arrays with one element, or arrays with all identical elements.
- Optimize: Be ready to discuss how you might optimize a sorting algorithm for a specific use case.
Conclusion
Sorting algorithms are a fundamental part of computer science and are essential for efficient data manipulation and analysis. While Quicksort and Mergesort are two of the most popular and efficient algorithms, understanding a range of sorting techniques will make you a more versatile and effective programmer.
Remember, the key to mastering these algorithms is practice. Implement them from scratch, experiment with different input sizes and distributions, and try to optimize them for specific scenarios. As you become more comfortable with these concepts, you’ll find that your problem-solving skills and algorithmic thinking improve dramatically, setting you up for success in both coding interviews and real-world programming challenges.
Keep exploring, keep coding, and remember that every great programmer started where you are now. Happy sorting!