Preparing for Algorithm-Intensive Interviews: Your Comprehensive Guide to Success
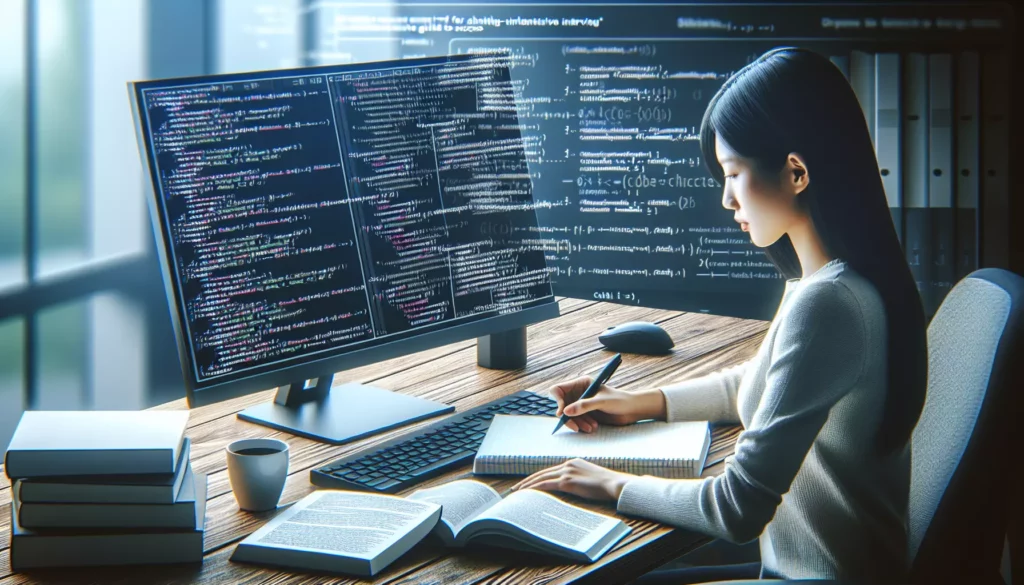
In today’s competitive tech landscape, securing a position at a top-tier company often involves navigating through rigorous algorithm-intensive interviews. These interviews, particularly common among FAANG (Facebook, Amazon, Apple, Netflix, Google) and other major tech companies, are designed to assess a candidate’s problem-solving skills, algorithmic thinking, and coding proficiency. For many aspiring software engineers, these interviews can be a significant hurdle. However, with the right preparation and mindset, you can significantly improve your chances of success. In this comprehensive guide, we’ll explore effective strategies and resources to help you prepare for algorithm-intensive interviews.
Understanding the Nature of Algorithm-Intensive Interviews
Before diving into preparation strategies, it’s crucial to understand what these interviews entail. Algorithm-intensive interviews typically involve:
- Solving complex algorithmic problems in a limited time frame
- Explaining your thought process and approach to the interviewer
- Analyzing the time and space complexity of your solutions
- Optimizing your initial solutions for better performance
- Coding your solution in a programming language of your choice
These interviews aim to assess not just your coding skills, but also your ability to think critically, communicate effectively, and perform under pressure.
Essential Components of Interview Preparation
1. Mastering Data Structures
A solid understanding of data structures is fundamental to solving algorithmic problems efficiently. Focus on mastering the following key data structures:
- Arrays and Strings
- Linked Lists
- Stacks and Queues
- Trees (Binary Trees, Binary Search Trees, Balanced Trees)
- Graphs
- Hash Tables
- Heaps
For each data structure, ensure you understand:
- Its basic properties and characteristics
- Common operations and their time complexities
- When and why to use it in problem-solving
- How to implement it from scratch
2. Algorithmic Techniques and Problem-Solving Patterns
Familiarize yourself with common algorithmic techniques and problem-solving patterns. These include:
- Brute Force
- Divide and Conquer
- Dynamic Programming
- Greedy Algorithms
- Backtracking
- Two Pointers
- Sliding Window
- Depth-First Search (DFS) and Breadth-First Search (BFS)
Understanding these techniques will help you recognize patterns in problems and apply the most suitable approach.
3. Time and Space Complexity Analysis
Being able to analyze and discuss the time and space complexity of your solutions is crucial. Make sure you:
- Understand Big O notation and its significance
- Can analyze the time and space complexity of different algorithms
- Are familiar with common time complexities (e.g., O(1), O(log n), O(n), O(n log n), O(n^2))
- Can identify opportunities for optimization in your solutions
4. Coding Practice
Consistent coding practice is essential for interview preparation. Aim to:
- Solve a diverse range of problems regularly
- Practice coding solutions without relying on an IDE
- Implement solutions in your preferred programming language
- Time yourself to simulate interview conditions
Effective Strategies for Interview Preparation
1. Develop a Structured Study Plan
Create a study plan that covers all essential topics. Allocate time for:
- Learning and reviewing data structures and algorithms
- Solving practice problems
- Reviewing and analyzing your solutions
- Mock interviews and timed coding sessions
Be consistent with your study schedule and adjust it as needed based on your progress.
2. Utilize Online Coding Platforms
Take advantage of online coding platforms that offer a wide range of algorithmic problems. Popular options include:
- LeetCode
- HackerRank
- CodeSignal
- AlgoExpert
These platforms often categorize problems by difficulty and topic, allowing you to focus on areas that need improvement.
3. Implement the “Explain Your Approach” Technique
Practice explaining your thought process out loud as you solve problems. This helps in:
- Improving your communication skills
- Clarifying your thinking
- Preparing for the interactive nature of technical interviews
4. Analyze and Learn from Sample Solutions
After solving a problem, review different solutions, especially those that are more efficient or elegant than yours. This helps you:
- Learn new approaches and techniques
- Understand different ways to optimize solutions
- Expand your problem-solving toolkit
5. Participate in Coding Contests
Engaging in coding contests can:
- Expose you to a variety of challenging problems
- Help you practice working under time pressure
- Allow you to benchmark your skills against others
6. Join Study Groups or Find a Study Partner
Collaborating with others can enhance your learning experience by:
- Providing opportunities for knowledge sharing
- Offering different perspectives on problem-solving
- Creating a support system for motivation and accountability
Tackling Common Interview Problems
While the specific problems you encounter in interviews may vary, there are certain types of problems that frequently appear. Let’s look at a few examples and discuss approaches to solving them.
1. Two Sum Problem
Problem: Given an array of integers and a target sum, return indices of two numbers such that they add up to the target.
Approach:
def two_sum(nums, target):
num_dict = {}
for i, num in enumerate(nums):
complement = target - num
if complement in num_dict:
return [num_dict[complement], i]
num_dict[num] = i
return []
This solution uses a hash table to achieve O(n) time complexity.
2. Reverse a Linked List
Problem: Reverse a singly linked list.
Approach:
def reverse_linked_list(head):
prev = None
current = head
while current:
next_node = current.next
current.next = prev
prev = current
current = next_node
return prev
This iterative solution reverses the links in place, achieving O(n) time complexity and O(1) space complexity.
3. Binary Tree Level Order Traversal
Problem: Given a binary tree, return the level order traversal of its nodes’ values.
Approach:
from collections import deque
def level_order_traversal(root):
if not root:
return []
result = []
queue = deque([root])
while queue:
level_size = len(queue)
current_level = []
for _ in range(level_size):
node = queue.popleft()
current_level.append(node.val)
if node.left:
queue.append(node.left)
if node.right:
queue.append(node.right)
result.append(current_level)
return result
This solution uses a breadth-first search approach with a queue to traverse the tree level by level.
Advanced Topics to Consider
As you progress in your preparation, consider delving into more advanced topics that often appear in interviews at top tech companies:
1. System Design
While not strictly algorithmic, system design questions are common in interviews, especially for more senior positions. Key areas to focus on include:
- Scalability principles
- Database design and optimization
- Caching strategies
- Load balancing
- Microservices architecture
2. Concurrency and Multithreading
Understanding concurrent programming is increasingly important. Focus on:
- Thread synchronization mechanisms
- Deadlock prevention
- Parallel algorithms
- Asynchronous programming patterns
3. Advanced Data Structures
While less common, knowledge of advanced data structures can set you apart:
- Trie
- Segment Tree
- Fenwick Tree (Binary Indexed Tree)
- Disjoint Set Union (Union-Find)
Preparing for the Interview Day
As your interview day approaches, consider these final preparation steps:
1. Review Your Past Solutions
Go through the problems you’ve solved, focusing on:
- The approach you used
- Time and space complexity analysis
- Any optimizations or alternative solutions
2. Practice Mock Interviews
Engage in mock interviews with friends, mentors, or through online platforms. This helps you:
- Get comfortable with the interview format
- Practice explaining your thought process
- Receive feedback on your performance
3. Prepare Questions for the Interviewer
Remember that interviews are also an opportunity for you to learn about the company. Prepare thoughtful questions about:
- The team and its projects
- The company’s technology stack
- Growth and learning opportunities
4. Take Care of Logistics
Ensure you’re prepared for the practical aspects of the interview:
- Test your equipment for virtual interviews
- Prepare a quiet, well-lit space
- Have a pen and paper ready for notes or diagrams
- Get a good night’s sleep before the interview
Conclusion
Preparing for algorithm-intensive interviews requires dedication, consistent practice, and a structured approach. By focusing on mastering data structures, algorithmic techniques, and problem-solving skills, you can significantly improve your chances of success. Remember that the journey of preparation is also an opportunity for personal growth and skill development.
As you prepare, leverage resources like AlgoCademy that offer interactive coding tutorials, AI-powered assistance, and step-by-step guidance. These tools can provide valuable support in your learning journey, helping you progress from beginner-level coding to tackling complex algorithmic problems confidently.
Stay persistent, embrace the learning process, and approach each problem as an opportunity to grow. With thorough preparation and the right mindset, you’ll be well-equipped to tackle even the most challenging algorithm-intensive interviews. Good luck!