Mastering Coding Interviews in Go (Golang): Essential Tips and Strategies
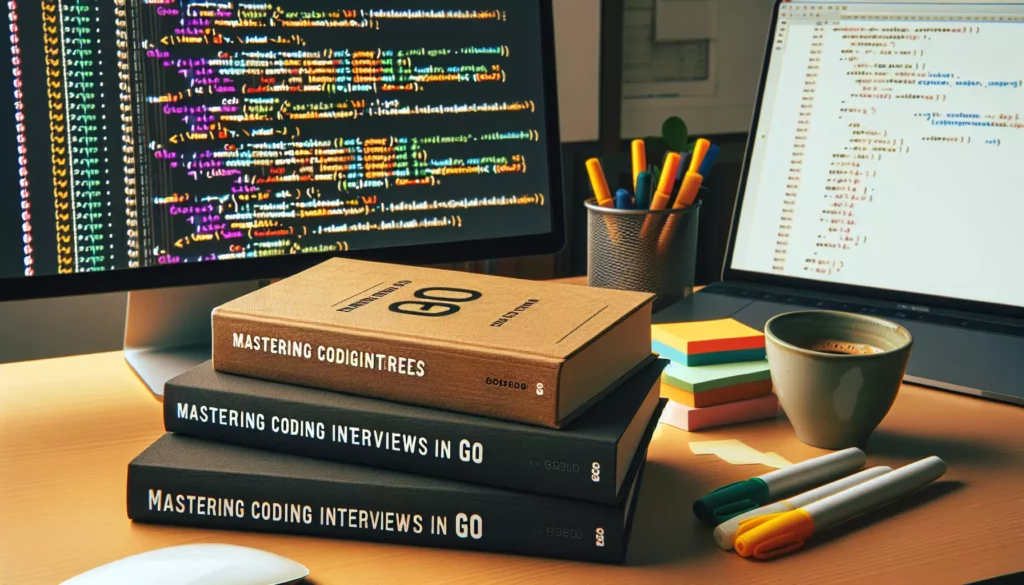
Are you preparing for a coding interview and considering using Go (Golang) as your language of choice? You’re in the right place! In this comprehensive guide, we’ll explore essential tips, strategies, and best practices to help you excel in coding interviews using Go. Whether you’re a seasoned Gopher or just starting with the language, these insights will boost your confidence and performance during technical interviews.
Table of Contents
- Why Choose Go for Coding Interviews?
- Preparing for Go Coding Interviews
- Mastering Common Data Structures in Go
- Essential Algorithms to Know in Go
- Leveraging Go’s Concurrency Features
- Go Best Practices for Clean and Efficient Code
- Common Pitfalls to Avoid in Go Interviews
- Conducting Mock Interviews in Go
- Additional Resources for Go Interview Preparation
- Conclusion
1. Why Choose Go for Coding Interviews?
Go, also known as Golang, has gained significant popularity in recent years, especially in the realm of backend development, cloud services, and distributed systems. Here are some compelling reasons to consider Go for your coding interviews:
- Simplicity and Readability: Go’s syntax is clean and straightforward, making it easier to write and understand code quickly – a crucial factor in time-constrained interviews.
- Standard Library: Go’s rich standard library provides many built-in functions and packages, reducing the need for external dependencies.
- Concurrency Support: With goroutines and channels, Go excels at handling concurrent operations, which can be a significant advantage in certain interview questions.
- Fast Compilation: Go compiles quickly, allowing for rapid testing and iteration during interviews.
- Growing Demand: Many tech companies, including Google (Go’s creator), are increasingly using Go in production, making it a valuable skill for job seekers.
2. Preparing for Go Coding Interviews
Before diving into specific Go tips, let’s outline a general preparation strategy:
- Master the Basics: Ensure you have a solid grasp of Go’s syntax, data types, and core concepts.
- Practice Regularly: Solve coding problems on platforms like LeetCode, HackerRank, or AlgoCademy using Go.
- Study Data Structures: Implement and understand common data structures in Go.
- Learn Algorithms: Focus on implementing and optimizing classic algorithms in Go.
- Understand Go’s Unique Features: Familiarize yourself with Go-specific concepts like goroutines, channels, and interfaces.
- Review Standard Library: Explore Go’s standard library to leverage built-in functions effectively.
- Practice Time Management: Work on solving problems within time constraints to simulate interview conditions.
3. Mastering Common Data Structures in Go
Understanding how to implement and use common data structures in Go is crucial for coding interviews. Let’s explore some key data structures and their Go implementations:
Arrays and Slices
Arrays in Go have a fixed size, while slices are dynamic. Slices are more commonly used in Go programs:
// Array
var arr [5]int
// Slice
slice := make([]int, 0, 5)
slice = append(slice, 1, 2, 3, 4, 5)
Maps
Maps in Go are similar to hash tables or dictionaries in other languages:
m := make(map[string]int)
m["key"] = 42
value, exists := m["key"]
if exists {
fmt.Println(value)
}
Linked Lists
While Go doesn’t have a built-in linked list, you can easily implement one:
type Node struct {
Value int
Next *Node
}
type LinkedList struct {
Head *Node
}
func (ll *LinkedList) Append(value int) {
newNode := &Node{Value: value}
if ll.Head == nil {
ll.Head = newNode
return
}
current := ll.Head
for current.Next != nil {
current = current.Next
}
current.Next = newNode
}
Stacks and Queues
Stacks and queues can be implemented using slices in Go:
// Stack
type Stack []int
func (s *Stack) Push(v int) {
*s = append(*s, v)
}
func (s *Stack) Pop() (int, bool) {
if len(*s) == 0 {
return 0, false
}
index := len(*s) - 1
element := (*s)[index]
*s = (*s)[:index]
return element, true
}
// Queue
type Queue []int
func (q *Queue) Enqueue(v int) {
*q = append(*q, v)
}
func (q *Queue) Dequeue() (int, bool) {
if len(*q) == 0 {
return 0, false
}
element := (*q)[0]
*q = (*q)[1:]
return element, true
}
Trees
Implementing a basic binary tree structure in Go:
type TreeNode struct {
Value int
Left *TreeNode
Right *TreeNode
}
func (t *TreeNode) Insert(value int) {
if t == nil {
t = &TreeNode{Value: value}
return
}
if value < t.Value {
if t.Left == nil {
t.Left = &TreeNode{Value: value}
} else {
t.Left.Insert(value)
}
} else {
if t.Right == nil {
t.Right = &TreeNode{Value: value}
} else {
t.Right.Insert(value)
}
}
}
4. Essential Algorithms to Know in Go
Mastering key algorithms and their Go implementations is crucial for coding interviews. Here are some essential algorithms you should be comfortable implementing in Go:
Sorting Algorithms
Go’s standard library provides a sort package, but understanding how to implement sorting algorithms is still important:
// Bubble Sort
func bubbleSort(arr []int) {
n := len(arr)
for i := 0; i < n-1; i++ {
for j := 0; j < n-i-1; j++ {
if arr[j] > arr[j+1] {
arr[j], arr[j+1] = arr[j+1], arr[j]
}
}
}
}
// Quick Sort
func quickSort(arr []int) []int {
if len(arr) <= 1 {
return arr
}
pivot := arr[len(arr)/2]
var left, right []int
for _, num := range arr {
if num < pivot {
left = append(left, num)
} else if num > pivot {
right = append(right, num)
}
}
return append(append(quickSort(left), pivot), quickSort(right)...)
}
Search Algorithms
Implement both linear and binary search algorithms:
// Linear Search
func linearSearch(arr []int, target int) int {
for i, v := range arr {
if v == target {
return i
}
}
return -1
}
// Binary Search
func binarySearch(arr []int, target int) int {
left, right := 0, len(arr)-1
for left <= right {
mid := (left + right) / 2
if arr[mid] == target {
return mid
} else if arr[mid] < target {
left = mid + 1
} else {
right = mid - 1
}
}
return -1
}
Graph Algorithms
Implement basic graph traversal algorithms like DFS and BFS:
type Graph struct {
adjacencyList map[int][]int
}
func (g *Graph) AddEdge(v, w int) {
g.adjacencyList[v] = append(g.adjacencyList[v], w)
g.adjacencyList[w] = append(g.adjacencyList[w], v)
}
// Depth-First Search
func (g *Graph) DFS(start int, visited map[int]bool) {
if visited == nil {
visited = make(map[int]bool)
}
visited[start] = true
fmt.Printf("%d ", start)
for _, neighbor := range g.adjacencyList[start] {
if !visited[neighbor] {
g.DFS(neighbor, visited)
}
}
}
// Breadth-First Search
func (g *Graph) BFS(start int) {
visited := make(map[int]bool)
queue := []int{start}
visited[start] = true
for len(queue) > 0 {
vertex := queue[0]
queue = queue[1:]
fmt.Printf("%d ", vertex)
for _, neighbor := range g.adjacencyList[vertex] {
if !visited[neighbor] {
visited[neighbor] = true
queue = append(queue, neighbor)
}
}
}
}
Dynamic Programming
Understand and implement dynamic programming solutions. Here’s an example of the Fibonacci sequence using dynamic programming:
func fibonacci(n int) int {
if n <= 1 {
return n
}
dp := make([]int, n+1)
dp[0], dp[1] = 0, 1
for i := 2; i <= n; i++ {
dp[i] = dp[i-1] + dp[i-2]
}
return dp[n]
}
5. Leveraging Go’s Concurrency Features
Go’s concurrency model, based on goroutines and channels, is one of its standout features. Understanding and leveraging these concepts can give you an edge in coding interviews:
Goroutines
Goroutines are lightweight threads managed by the Go runtime. They allow for concurrent execution:
func printNumbers(n int) {
for i := 1; i <= n; i++ {
fmt.Printf("%d ", i)
}
}
func main() {
go printNumbers(5)
go printNumbers(5)
time.Sleep(time.Second)
}
Channels
Channels are used for communication between goroutines:
func sum(s []int, c chan int) {
sum := 0
for _, v := range s {
sum += v
}
c <- sum // Send sum to channel
}
func main() {
s := []int{7, 2, 8, -9, 4, 0}
c := make(chan int)
go sum(s[:len(s)/2], c)
go sum(s[len(s)/2:], c)
x, y := <-c, <-c // Receive from channel
fmt.Println(x, y, x+y)
}
Select Statement
The select statement allows you to wait on multiple channel operations:
func fibonacci(c, quit chan int) {
x, y := 0, 1
for {
select {
case c <- x:
x, y = y, x+y
case <-quit:
fmt.Println("quit")
return
}
}
}
func main() {
c := make(chan int)
quit := make(chan int)
go func() {
for i := 0; i < 10; i++ {
fmt.Println(<-c)
}
quit <- 0
}()
fibonacci(c, quit)
}
6. Go Best Practices for Clean and Efficient Code
Writing clean, efficient, and idiomatic Go code is crucial for impressing interviewers. Here are some best practices to keep in mind:
Use Meaningful Variable Names
Choose descriptive and concise names for variables, functions, and types:
// Good
userAge := 30
func calculateTotalPrice(items []Item) float64 {
// ...
}
// Avoid
a := 30
func calc(i []Item) float64 {
// ...
}
Utilize Go’s Built-in Functions and Packages
Leverage Go’s standard library whenever possible:
import (
"sort"
"strings"
)
// Using sort package
sort.Ints(numbers)
// Using strings package
trimmedString := strings.TrimSpace(input)
Handle Errors Properly
Go encourages explicit error handling. Always check and handle errors:
file, err := os.Open("file.txt")
if err != nil {
log.Fatal("Error opening file:", err)
}
defer file.Close()
Use Defer for Cleanup
The defer keyword is useful for cleanup operations:
func processFile(filename string) error {
f, err := os.Open(filename)
if err != nil {
return err
}
defer f.Close()
// Process the file...
return nil
}
Utilize Interfaces for Flexibility
Go’s interfaces allow for flexible and testable code:
type Stringer interface {
String() string
}
func printString(s Stringer) {
fmt.Println(s.String())
}
Write Testable Code
Design your functions and methods to be easily testable:
func Add(a, b int) int {
return a + b
}
func TestAdd(t *testing.T) {
result := Add(2, 3)
if result != 5 {
t.Errorf("Add(2, 3) = %d; want 5", result)
}
}
7. Common Pitfalls to Avoid in Go Interviews
Being aware of common mistakes can help you avoid them during your Go coding interviews:
Ignoring Error Handling
Always handle errors returned by functions:
// Incorrect
result, _ := someFunction()
// Correct
result, err := someFunction()
if err != nil {
// Handle the error
}
Misusing Goroutines
Be cautious when using goroutines, especially in interview settings:
// Incorrect (may exit before goroutine finishes)
func main() {
go printNumbers()
}
// Correct
func main() {
go printNumbers()
time.Sleep(time.Second) // Or use WaitGroup for proper synchronization
}
Forgetting to Close Channels
Always close channels when you’re done with them:
ch := make(chan int)
go func() {
// Send data
close(ch) // Don't forget to close
}()
for n := range ch {
fmt.Println(n)
}
Inefficient Slice Operations
Be mindful of slice capacity when appending:
// Inefficient
s := make([]int, 0)
for i := 0; i < 10000; i++ {
s = append(s, i)
}
// More efficient
s := make([]int, 0, 10000)
for i := 0; i < 10000; i++ {
s = append(s, i)
}
Ignoring Receiver Types
Choose between value and pointer receivers wisely:
type Counter struct {
count int
}
// Value receiver (doesn't modify the original)
func (c Counter) Increment() {
c.count++
}
// Pointer receiver (modifies the original)
func (c *Counter) Increment() {
c.count++
}
8. Conducting Mock Interviews in Go
Practicing with mock interviews is an excellent way to prepare for real coding interviews in Go. Here are some tips for effective mock interviews:
Set Up a Realistic Environment
- Use an online code editor or IDE that supports Go.
- Set a timer to simulate time constraints.
- Practice explaining your thought process out loud.
Sample Mock Interview Question
Here’s an example of a Go coding interview question you might encounter:
/*
Problem: Implement a function that finds the longest substring without repeating characters in a given string.
Example:
Input: "abcabcbb"
Output: 3 (The answer is "abc", with the length of 3)
Input: "bbbbb"
Output: 1 (The answer is "b", with the length of 1)
*/
func lengthOfLongestSubstring(s string) int {
// Your implementation here
}
// Test cases
func main() {
fmt.Println(lengthOfLongestSubstring("abcabcbb")) // Should output 3
fmt.Println(lengthOfLongestSubstring("bbbbb")) // Should output 1
fmt.Println(lengthOfLongestSubstring("pwwkew")) // Should output 3
}
Solution Approach
Here’s one way to solve this problem using Go:
func lengthOfLongestSubstring(s string) int {
charIndex := make(map[rune]int)
maxLength := 0
start := 0
for i, char := range s {
if lastIndex, found := charIndex[char]; found && lastIndex >= start {
start = lastIndex + 1
}
charIndex[char] = i
currentLength := i - start + 1
if currentLength > maxLength {
maxLength = currentLength
}
}
return maxLength
}
Explanation
- We use a map to store the last index of each character.
- We maintain a sliding window with a start index.
- As we iterate through the string, we update the start index if we find a repeating character.
- We keep track of the maximum length of the non-repeating substring.
9. Additional Resources for Go Interview Preparation
To further enhance your Go interview skills, consider these resources:
- Books:
- “The Go Programming Language” by Alan A. A. Donovan and Brian W. Kernighan
- “Go in Action” by William Kennedy, Brian Ketelsen, and Erik St. Martin
- Online Platforms:
- LeetCode – Offers a Go environment for solving coding problems
- HackerRank – Provides Go-specific challenges and competitions
- AlgoCademy – Offers interactive coding tutorials and AI-powered assistance
- Go-specific Resources:
- The official Go documentation (golang.org)
- Go by Example (gobyexample.com)
- Effective Go (golang.org/doc/effective_go.html)
- Practice Projects:
- Build a RESTful API using Go
- Implement a basic web server
- Create a concurrent web scraper
10. Conclusion
Mastering Go for coding interviews requires a combination of language proficiency, problem-solving skills, and practice. By following the tips and strategies outlined in this guide, you’ll be well-prepared to tackle Go coding interviews with confidence. Remember to:
- Regularly practice coding problems in Go
- Master essential data structures and algorithms
- Leverage Go’s unique features, especially concurrency
- Write clean, efficient, and idiomatic Go code
- Conduct mock interviews to simulate real interview conditions
With dedication and consistent practice, you’ll be well on your way to acing your Go coding interviews and landing your dream job. Good luck, and happy coding!