Understanding Graph Traversals: BFS and DFS
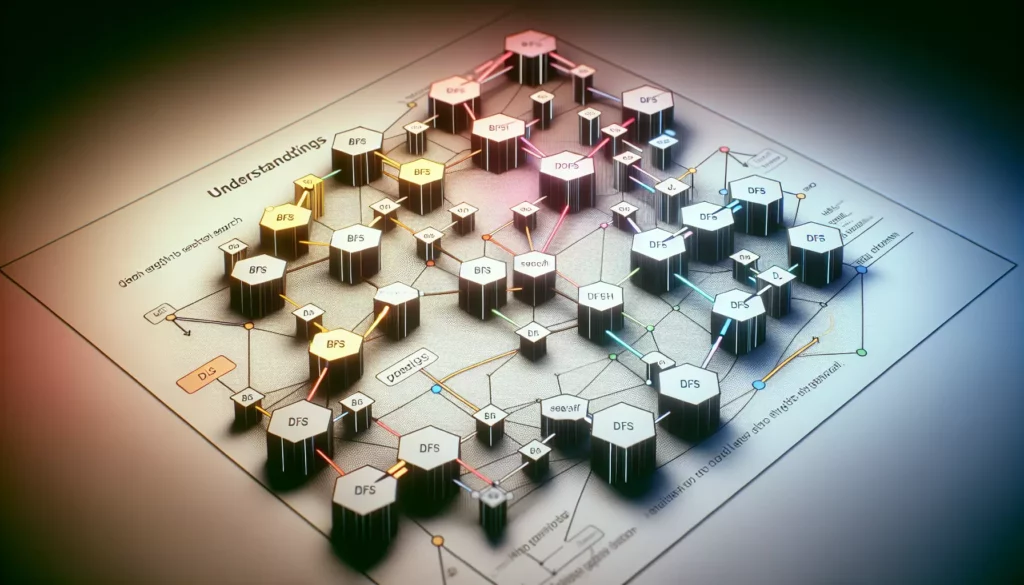
Graph traversal algorithms are fundamental techniques in computer science and play a crucial role in solving various problems related to networks, social connections, and data structures. Two of the most important graph traversal algorithms are Breadth-First Search (BFS) and Depth-First Search (DFS). In this comprehensive guide, we’ll dive deep into these algorithms, exploring their implementations, use cases, and the key differences between them.
What are Graph Traversals?
Before we delve into the specific algorithms, let’s first understand what graph traversals are. Graph traversal, also known as graph search, is the process of visiting (checking and/or updating) each vertex in a graph. The order in which the vertices are visited defines the type of traversal.
Graph traversals are used in various applications, including:
- Finding the shortest path between two nodes
- Web crawling
- Social network analysis
- Solving puzzles and mazes
- Detecting cycles in graphs
- Topological sorting
Breadth-First Search (BFS)
Breadth-First Search is an algorithm for traversing or searching tree or graph data structures. It starts at a chosen root vertex and explores all the neighboring nodes at the present depth before moving on to the nodes at the next depth level.
How BFS Works
- Start by choosing a starting vertex and add it to a queue.
- Remove the first vertex from the queue and mark it as visited.
- Add all unvisited neighbors of this vertex to the queue.
- Repeat steps 2 and 3 until the queue is empty.
BFS Implementation in Python
Here’s a simple implementation of BFS in Python using an adjacency list representation of a graph:
from collections import deque
def bfs(graph, start):
visited = set()
queue = deque([start])
visited.add(start)
while queue:
vertex = queue.popleft()
print(vertex, end=' ')
for neighbor in graph[vertex]:
if neighbor not in visited:
visited.add(neighbor)
queue.append(neighbor)
# Example usage
graph = {
'A': ['B', 'C'],
'B': ['A', 'D', 'E'],
'C': ['A', 'F'],
'D': ['B'],
'E': ['B', 'F'],
'F': ['C', 'E']
}
print("BFS traversal starting from vertex 'A':")
bfs(graph, 'A')
This implementation uses a queue (implemented using Python’s deque
) to keep track of the vertices to visit next. The visited
set ensures that we don’t visit the same vertex multiple times.
Time and Space Complexity of BFS
The time complexity of BFS is O(V + E), where V is the number of vertices and E is the number of edges in the graph. This is because in the worst case, we might have to visit all vertices and edges.
The space complexity is O(V), as in the worst case, all vertices might be stored in the queue simultaneously.
Applications of BFS
- Shortest Path and Minimum Spanning Tree for unweighted graphs
- Peer to Peer Networks
- Crawlers in Search Engines
- Social Networking Websites
- GPS Navigation systems
- Broadcasting in Network
Depth-First Search (DFS)
Depth-First Search is an algorithm for traversing or searching tree or graph data structures. The algorithm starts at the root node and explores as far as possible along each branch before backtracking.
How DFS Works
- Start by choosing a starting vertex and push it to a stack.
- Pop a vertex from the stack and mark it as visited.
- Push all unvisited neighbors of this vertex to the stack.
- Repeat steps 2 and 3 until the stack is empty.
DFS Implementation in Python
Here’s a simple implementation of DFS in Python using recursion:
def dfs(graph, start, visited=None):
if visited is None:
visited = set()
visited.add(start)
print(start, end=' ')
for neighbor in graph[start]:
if neighbor not in visited:
dfs(graph, neighbor, visited)
# Example usage
graph = {
'A': ['B', 'C'],
'B': ['A', 'D', 'E'],
'C': ['A', 'F'],
'D': ['B'],
'E': ['B', 'F'],
'F': ['C', 'E']
}
print("DFS traversal starting from vertex 'A':")
dfs(graph, 'A')
This implementation uses recursion to traverse the graph. The visited
set keeps track of the vertices that have been visited to avoid cycles.
Time and Space Complexity of DFS
The time complexity of DFS is also O(V + E), where V is the number of vertices and E is the number of edges in the graph. This is because we visit each vertex once and each edge once.
The space complexity is O(V) in the worst case, due to the recursion stack.
Applications of DFS
- Detecting cycle in a graph
- Path Finding
- Topological Sorting
- Solving puzzles with only one solution, such as mazes
- Finding strongly connected components of a graph
- Generating words in order to plot the frequency-of-use graph
BFS vs DFS: Key Differences
While both BFS and DFS are graph traversal algorithms, they have some key differences:
Aspect | BFS | DFS |
---|---|---|
Traversal Order | Explores neighbors before going deeper | Goes as deep as possible before backtracking |
Data Structure | Queue | Stack (or recursion) |
Space Complexity | O(V) – can be high for wide graphs | O(h) where h is the height of the tree |
Completeness | Complete (finds all solutions) | Not complete (may not find all solutions) |
Optimal for | Finding shortest path | Memory-constrained systems |
When to Use BFS vs DFS
The choice between BFS and DFS depends on the problem you’re trying to solve:
Use BFS when:
- You need to find the shortest path between two vertices
- The graph is not too wide (to avoid high memory usage)
- You need to find all solutions, not just any solution
- The target is likely to be closer to the starting point
Use DFS when:
- You need to search the entire graph
- You’re working with a very wide graph and memory is a constraint
- You’re trying to solve puzzles with only one solution
- You need to perform topological sorting
Advanced Graph Traversal Techniques
While BFS and DFS are the fundamental graph traversal algorithms, there are several advanced techniques that build upon these basic algorithms:
1. Bidirectional Search
Bidirectional search is an algorithm that runs two simultaneous searches: one forward from the initial state and one backward from the goal state, hoping that the two searches meet in the middle. It can be significantly faster than standard BFS or DFS in many cases.
2. Iterative Deepening Depth-First Search (IDDFS)
IDDFS is a state space/graph search strategy in which a depth-limited version of depth-first search is run repeatedly with increasing depth limits until the goal is found. It combines the space-efficiency of DFS with the completeness of BFS.
3. A* Search Algorithm
A* is a best-first search algorithm that finds the least-cost path from a given initial node to one goal node. It uses a heuristic function to estimate the cost from any vertex to the goal, which helps guide the search more efficiently.
4. Dijkstra’s Algorithm
While not strictly a traversal algorithm, Dijkstra’s algorithm is crucial for finding the shortest paths between nodes in a graph, which can be seen as a form of intelligent traversal.
Implementing Graph Traversals in Different Programming Languages
While we’ve seen Python implementations, it’s valuable to understand how these algorithms can be implemented in other popular programming languages. Here are examples in Java and C++:
BFS in Java
import java.util.*;
public class Graph {
private int V;
private LinkedList<Integer> adj[];
Graph(int v) {
V = v;
adj = new LinkedList[v];
for (int i=0; i<v; ++i)
adj[i] = new LinkedList();
}
void addEdge(int v, int w) {
adj[v].add(w);
}
void BFS(int s) {
boolean visited[] = new boolean[V];
LinkedList<Integer> queue = new LinkedList<Integer>();
visited[s] = true;
queue.add(s);
while (queue.size() != 0) {
s = queue.poll();
System.out.print(s + " ");
Iterator<Integer> i = adj[s].listIterator();
while (i.hasNext()) {
int n = i.next();
if (!visited[n]) {
visited[n] = true;
queue.add(n);
}
}
}
}
public static void main(String args[]) {
Graph g = new Graph(4);
g.addEdge(0, 1);
g.addEdge(0, 2);
g.addEdge(1, 2);
g.addEdge(2, 0);
g.addEdge(2, 3);
g.addEdge(3, 3);
System.out.println("BFS traversal starting from vertex 2:");
g.BFS(2);
}
}
DFS in C++
#include <iostream>
#include <list>
#include <vector>
using namespace std;
class Graph {
int V;
list<int> *adj;
void DFSUtil(int v, vector<bool> &visited);
public:
Graph(int V);
void addEdge(int v, int w);
void DFS(int v);
};
Graph::Graph(int V) {
this->V = V;
adj = new list<int>[V];
}
void Graph::addEdge(int v, int w) {
adj[v].push_back(w);
}
void Graph::DFSUtil(int v, vector<bool> &visited) {
visited[v] = true;
cout << v << " ";
list<int>::iterator i;
for (i = adj[v].begin(); i != adj[v].end(); ++i)
if (!visited[*i])
DFSUtil(*i, visited);
}
void Graph::DFS(int v) {
vector<bool> visited(V, false);
DFSUtil(v, visited);
}
int main() {
Graph g(4);
g.addEdge(0, 1);
g.addEdge(0, 2);
g.addEdge(1, 2);
g.addEdge(2, 0);
g.addEdge(2, 3);
g.addEdge(3, 3);
cout << "DFS traversal starting from vertex 2:" << endl;
g.DFS(2);
return 0;
}
Graph Traversals in Real-World Applications
Graph traversal algorithms have numerous real-world applications across various domains. Here are some examples:
1. Social Network Analysis
Both BFS and DFS are used in social network analysis. For instance, BFS can be used to find the shortest path between two users in a social network, which is useful for features like “degrees of separation” or “friend suggestions”.
2. Web Crawling
Search engines use graph traversal algorithms to crawl and index web pages. BFS is often preferred as it explores web pages level by level, which is usually more efficient for discovering new content.
3. GPS and Navigation Systems
Navigation systems use graph traversal algorithms to find the shortest or fastest route between two points. While more advanced algorithms like A* are often used, they are built upon the principles of BFS and DFS.
4. Computer Networks
Routing algorithms in computer networks often use graph traversal techniques to find efficient paths for data packets.
5. Artificial Intelligence
In game playing and problem-solving AI, graph traversal algorithms are used to explore possible moves or states. For example, a chess-playing AI might use a variant of DFS to explore possible future board states.
Conclusion
Understanding graph traversals, particularly BFS and DFS, is crucial for any programmer or computer scientist. These algorithms form the basis of many more complex algorithms and have wide-ranging applications in various fields of computer science and beyond.
As you continue your journey in programming and algorithm design, you’ll find that mastering these fundamental graph traversal techniques will give you a solid foundation for tackling more advanced problems. Whether you’re preparing for technical interviews, working on a complex software project, or just exploring the fascinating world of algorithms, BFS and DFS will be invaluable tools in your programming toolkit.
Remember, the key to mastering these algorithms is practice. Try implementing them in different programming languages, solve graph-related problems on coding platforms, and think about how you can apply these algorithms to real-world problems. Happy coding!