Mastering JavaScript: Strategies for Cracking Coding Interviews
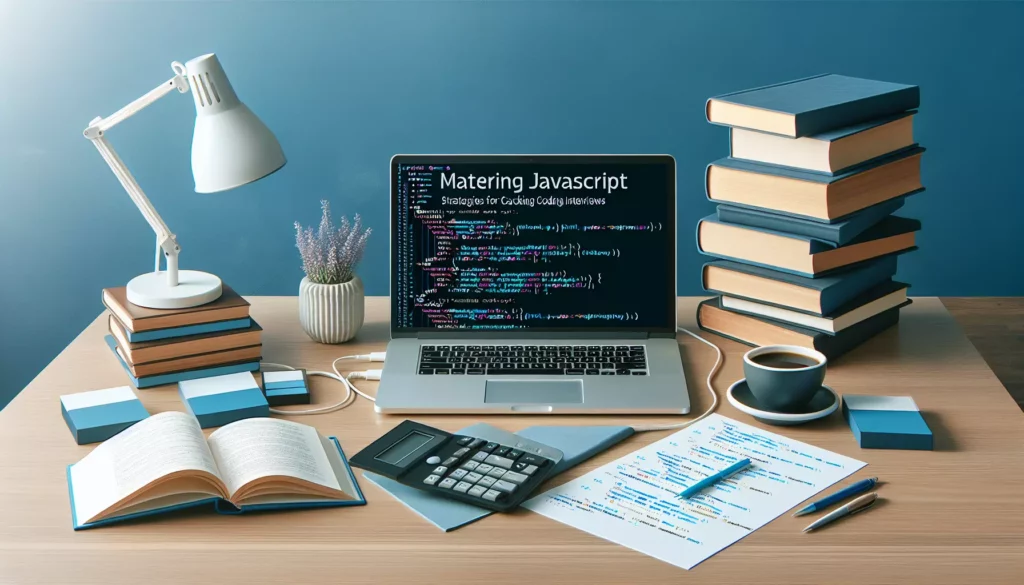
In today’s competitive tech landscape, mastering JavaScript and excelling in coding interviews are crucial skills for aspiring developers. Whether you’re aiming for a position at a FAANG company (Facebook, Amazon, Apple, Netflix, Google) or any other tech giant, being well-prepared can make all the difference. This comprehensive guide will walk you through effective strategies to crack coding interviews in JavaScript, helping you showcase your skills and land your dream job.
1. Understanding the Basics of JavaScript
Before diving into complex algorithms and data structures, it’s essential to have a solid grasp of JavaScript fundamentals. This includes:
- Variables and data types
- Functions and scope
- Objects and arrays
- Closures and callbacks
- Promises and async/await
- ES6+ features
Make sure you’re comfortable with these concepts, as they form the building blocks of more advanced JavaScript programming.
2. Mastering Data Structures in JavaScript
Data structures are fundamental to solving complex problems efficiently. Familiarize yourself with the following data structures and their implementations in JavaScript:
- Arrays
- Linked Lists
- Stacks and Queues
- Hash Tables
- Trees (Binary Trees, Binary Search Trees)
- Graphs
- Heaps
Let’s look at a simple implementation of a Stack in JavaScript:
class Stack {
constructor() {
this.items = [];
}
push(element) {
this.items.push(element);
}
pop() {
if (this.isEmpty()) {
return "Stack is empty";
}
return this.items.pop();
}
peek() {
return this.items[this.items.length - 1];
}
isEmpty() {
return this.items.length === 0;
}
size() {
return this.items.length;
}
clear() {
this.items = [];
}
print() {
console.log(this.items.toString());
}
}
// Usage
const stack = new Stack();
stack.push(10);
stack.push(20);
stack.push(30);
console.log(stack.pop()); // Output: 30
console.log(stack.peek()); // Output: 20
console.log(stack.size()); // Output: 2
3. Algorithmic Problem-Solving Techniques
Coding interviews often involve solving complex algorithmic problems. Familiarize yourself with common problem-solving techniques such as:
- Two-pointer technique
- Sliding window
- Divide and conquer
- Dynamic programming
- Greedy algorithms
- Backtracking
Here’s an example of the two-pointer technique to solve the “Container With Most Water” problem:
function maxArea(height) {
let left = 0;
let right = height.length - 1;
let maxArea = 0;
while (left < right) {
const width = right - left;
const containerHeight = Math.min(height[left], height[right]);
const area = width * containerHeight;
maxArea = Math.max(maxArea, area);
if (height[left] < height[right]) {
left++;
} else {
right--;
}
}
return maxArea;
}
// Usage
const heights = [1, 8, 6, 2, 5, 4, 8, 3, 7];
console.log(maxArea(heights)); // Output: 49
4. Time and Space Complexity Analysis
Understanding the time and space complexity of your solutions is crucial. Interviewers often ask about the efficiency of your code. Be prepared to analyze and discuss the Big O notation of your algorithms. Some key points to remember:
- O(1): Constant time
- O(log n): Logarithmic time
- O(n): Linear time
- O(n log n): Linearithmic time
- O(n^2): Quadratic time
- O(2^n): Exponential time
Always strive to optimize your solutions for better time and space complexity.
5. Practice, Practice, Practice
Consistent practice is key to mastering coding interviews. Utilize online platforms like LeetCode, HackerRank, or CodeSignal to solve a variety of problems. Aim to solve at least one problem daily, focusing on different difficulty levels and topics.
Here’s a strategy for effective practice:
- Start with easy problems to build confidence
- Gradually move to medium and hard problems
- Time yourself to simulate interview conditions
- Review and learn from others’ solutions
- Revisit problems after a few weeks to reinforce your learning
6. Mock Interviews and Pair Programming
Participate in mock interviews and pair programming sessions to gain real-world experience. This helps you:
- Improve your communication skills
- Learn to explain your thought process clearly
- Handle pressure and time constraints
- Receive feedback on your performance
Platforms like Pramp or interviewing.io offer free mock interviews with peers or experienced interviewers.
7. Mastering JavaScript-Specific Concepts
While general problem-solving skills are important, mastering JavaScript-specific concepts can give you an edge in interviews. Focus on:
7.1 Closures and Scope
Understanding closures is crucial in JavaScript. Here’s an example:
function createCounter() {
let count = 0;
return function() {
count++;
return count;
};
}
const counter = createCounter();
console.log(counter()); // Output: 1
console.log(counter()); // Output: 2
console.log(counter()); // Output: 3
7.2 Prototypal Inheritance
JavaScript uses prototypal inheritance, which is different from class-based inheritance in languages like Java or C++. Understanding this concept is essential:
function Animal(name) {
this.name = name;
}
Animal.prototype.speak = function() {
console.log(this.name + ' makes a sound.');
};
function Dog(name) {
Animal.call(this, name);
}
Dog.prototype = Object.create(Animal.prototype);
Dog.prototype.constructor = Dog;
Dog.prototype.bark = function() {
console.log(this.name + ' barks.');
};
const dog = new Dog('Rex');
dog.speak(); // Output: Rex makes a sound.
dog.bark(); // Output: Rex barks.
7.3 Asynchronous Programming
Mastering asynchronous programming in JavaScript is crucial. Understand Promises, async/await, and callback patterns:
function fetchData(url) {
return new Promise((resolve, reject) => {
setTimeout(() => {
if (Math.random() > 0.5) {
resolve("Data fetched successfully");
} else {
reject("Error fetching data");
}
}, 1000);
});
}
async function getData() {
try {
const result = await fetchData('https://api.example.com/data');
console.log(result);
} catch (error) {
console.error(error);
}
}
getData();
8. System Design and Architecture
For more senior positions, you may be asked about system design and architecture. While this is more common in general software engineering interviews, having a basic understanding can be beneficial. Key areas to focus on include:
- Scalability and performance optimization
- Database design (SQL vs NoSQL)
- Caching strategies
- Microservices architecture
- RESTful API design
9. Behavioral Questions and Soft Skills
Don’t neglect the importance of behavioral questions and soft skills. Interviewers often assess your ability to work in a team, handle conflicts, and communicate effectively. Prepare stories and examples that demonstrate your:
- Problem-solving abilities
- Leadership skills
- Adaptability and learning agility
- Collaboration and teamwork
- Project management experience
Use the STAR (Situation, Task, Action, Result) method to structure your responses effectively.
10. Stay Updated with JavaScript Ecosystem
The JavaScript ecosystem is constantly evolving. Stay updated with the latest trends, frameworks, and best practices. This includes:
- Modern JavaScript features (ES6+)
- Popular frameworks like React, Vue, or Angular
- Node.js and server-side JavaScript
- Testing frameworks (Jest, Mocha)
- Build tools and module bundlers (Webpack, Rollup)
11. Optimize Your Problem-Solving Approach
Develop a structured approach to problem-solving during interviews. Follow these steps:
- Understand the problem thoroughly
- Ask clarifying questions
- Discuss potential approaches and trade-offs
- Choose an approach and outline the solution
- Implement the solution step by step
- Test your solution with various inputs
- Analyze time and space complexity
- Discuss potential optimizations
Here’s an example of how to approach the “Two Sum” problem:
function twoSum(nums, target) {
const numMap = new Map();
for (let i = 0; i < nums.length; i++) {
const complement = target - nums[i];
if (numMap.has(complement)) {
return [numMap.get(complement), i];
}
numMap.set(nums[i], i);
}
return []; // No solution found
}
// Test the function
const nums = [2, 7, 11, 15];
const target = 9;
console.log(twoSum(nums, target)); // Output: [0, 1]
12. Learn from Your Mistakes
After each interview or practice session, reflect on your performance. Identify areas for improvement and work on them. Keep a journal of common mistakes and how to avoid them in the future.
13. Develop a Growth Mindset
Approach coding interviews with a growth mindset. View challenges as opportunities to learn and improve. Embrace feedback and use it constructively to enhance your skills.
14. Leverage Online Resources and Communities
Take advantage of online resources and communities to enhance your learning:
- Join coding forums and discussion groups
- Participate in coding challenges and competitions
- Watch coding interview preparation videos on platforms like YouTube
- Read technical blogs and articles
- Contribute to open-source projects
15. Prepare for Virtual Interviews
With the increasing prevalence of remote work, many coding interviews are now conducted virtually. Prepare for this format by:
- Setting up a quiet, well-lit space for video interviews
- Testing your audio and video equipment beforehand
- Familiarizing yourself with online coding platforms (e.g., CoderPad, HackerRank)
- Practicing coding while explaining your thought process verbally
Conclusion
Cracking coding interviews in JavaScript requires a combination of technical skills, problem-solving abilities, and effective communication. By following these strategies and consistently practicing, you’ll be well-prepared to tackle even the most challenging coding interviews.
Remember, the key to success is not just about memorizing solutions but understanding the underlying concepts and developing a problem-solving mindset. Stay curious, keep learning, and approach each interview as an opportunity to showcase your skills and passion for programming.
With dedication and the right approach, you’ll be well on your way to acing your JavaScript coding interviews and landing your dream job in the tech industry. Good luck!