Understanding Design Patterns and Their Interview Relevance: A Comprehensive Guide
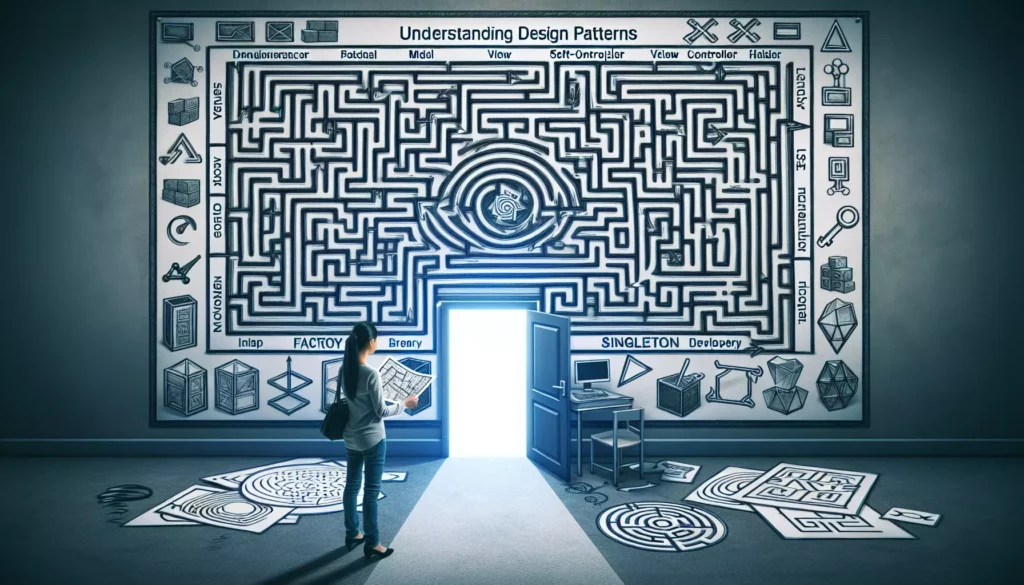
In the world of software development, design patterns are essential tools that every programmer should have in their toolkit. These patterns are not just theoretical concepts but practical solutions to common problems that developers face in their day-to-day work. Moreover, understanding design patterns can significantly boost your chances of success in technical interviews, especially when applying to major tech companies like FAANG (Facebook, Amazon, Apple, Netflix, and Google).
In this comprehensive guide, we’ll dive deep into the world of design patterns, exploring their importance, types, and how they can help you ace your next technical interview. Whether you’re a beginner looking to enhance your coding skills or an experienced developer preparing for a big interview, this article will provide valuable insights and practical knowledge.
Table of Contents
- What Are Design Patterns?
- Types of Design Patterns
- Benefits of Using Design Patterns
- Common Design Patterns and Their Use Cases
- Design Patterns in Technical Interviews
- Implementing Design Patterns in Code
- Best Practices for Using Design Patterns
- Anti-Patterns: What to Avoid
- Resources for Learning Design Patterns
- Conclusion
1. What Are Design Patterns?
Design patterns are reusable solutions to common problems that occur in software design. They are not specific implementations or finished designs that can be directly transformed into code. Instead, they are templates or blueprints that can be applied to solve recurring design problems in object-oriented programming.
The concept of design patterns was popularized by the “Gang of Four” (GoF) – Erich Gamma, Richard Helm, Ralph Johnson, and John Vlissides – in their seminal book “Design Patterns: Elements of Reusable Object-Oriented Software” published in 1994. This book cataloged 23 classic design patterns that are still widely used today.
Design patterns provide several benefits:
- They offer proven solutions to common problems
- They provide a common vocabulary for developers
- They enhance code reusability and maintainability
- They promote best practices in software design
2. Types of Design Patterns
Design patterns are typically categorized into three main types:
1. Creational Patterns
These patterns deal with object creation mechanisms, trying to create objects in a manner suitable to the situation. The basic form of object creation could result in design problems or add complexity to the design. Creational design patterns solve this problem by controlling the object creation process.
Examples of creational patterns include:
- Singleton
- Factory Method
- Abstract Factory
- Builder
- Prototype
2. Structural Patterns
Structural patterns are concerned with how classes and objects are composed to form larger structures. They help ensure that if one part of a system changes, the entire structure doesn’t need to change.
Examples of structural patterns include:
- Adapter
- Bridge
- Composite
- Decorator
- Facade
- Flyweight
- Proxy
3. Behavioral Patterns
Behavioral patterns are concerned with algorithms and the assignment of responsibilities between objects. They characterize complex control flow that’s difficult to follow at run-time. These patterns increase flexibility in carrying out communication between objects.
Examples of behavioral patterns include:
- Observer
- Strategy
- Command
- State
- Visitor
- Mediator
- Chain of Responsibility
3. Benefits of Using Design Patterns
Incorporating design patterns into your software development process offers numerous advantages:
1. Code Reusability
Design patterns provide tested, proven development paradigms. They can speed up the development process by providing tested, proven development paradigms.
2. Common Vocabulary
Design patterns provide a standard terminology and are specific to particular scenarios. For example, a singleton pattern signifies the use of a single object. This common vocabulary helps developers communicate more efficiently.
3. Best Practices
Design patterns have been evolved over a long period, and they provide the best solutions to certain problems faced during software development. Learning these patterns helps inexperienced developers to learn software design in an easy and faster way.
4. Scalability and Maintainability
By using design patterns, you can make your code more flexible, reusable, and maintainable. The patterns allow you to keep your code loosely coupled, which makes it easier to scale and maintain.
5. Improved Software Architecture
Design patterns provide general solutions, documented in a format that doesn’t require specific implementation details. They can speed up the development process by providing tested, proven development paradigms.
4. Common Design Patterns and Their Use Cases
Let’s explore some of the most commonly used design patterns and their typical use cases:
1. Singleton Pattern
The Singleton pattern ensures a class has only one instance and provides a global point of access to it. This is useful when exactly one object is needed to coordinate actions across the system.
Use cases:
- Managing a connection to a database
- File manager
- Print spooler
2. Factory Method Pattern
The Factory Method pattern defines an interface for creating an object, but lets subclasses decide which class to instantiate. It allows a class to defer instantiation to subclasses.
Use cases:
- When a class can’t anticipate the type of objects it needs to create
- When a class wants its subclasses to specify the objects it creates
- When classes delegate responsibility to one of several helper subclasses, and you want to localize the knowledge of which helper subclass is the delegate
3. Observer Pattern
The Observer pattern defines a one-to-many dependency between objects so that when one object changes state, all its dependents are notified and updated automatically.
Use cases:
- Implementing event handling systems
- Implementing subscription functionality
- MVC (Model-View-Controller) architectural pattern
4. Strategy Pattern
The Strategy pattern defines a family of algorithms, encapsulates each one, and makes them interchangeable. It lets the algorithm vary independently from clients that use it.
Use cases:
- When you want to define a class that will have one behavior that is similar to other behaviors in a list
- When you need to use one of several behaviors dynamically
- When you have a conditional statement that chooses between several algorithms or behaviors
5. Decorator Pattern
The Decorator pattern attaches additional responsibilities to an object dynamically. It provides a flexible alternative to subclassing for extending functionality.
Use cases:
- Adding functionality to individual objects without affecting other objects of the same class
- When extension by subclassing is impractical
- When you want to add responsibilities to objects dynamically and transparently, without affecting other objects
5. Design Patterns in Technical Interviews
Understanding design patterns is crucial for technical interviews, especially when applying to major tech companies. Here’s why:
1. Demonstrates Problem-Solving Skills
Knowledge of design patterns shows that you can recognize and apply proven solutions to common problems. This demonstrates your problem-solving skills and ability to think abstractly.
2. Shows Code Quality Awareness
Using appropriate design patterns indicates that you care about code quality, maintainability, and scalability – all crucial aspects that interviewers look for.
3. Communicates Effectively
Design patterns provide a common vocabulary that allows you to communicate complex ideas quickly and effectively during an interview.
4. Indicates Experience
Familiarity with design patterns often indicates a certain level of experience and exposure to real-world programming challenges.
5. Helps in System Design Questions
Many system design interview questions can be addressed more effectively with the application of appropriate design patterns.
Common Interview Questions Related to Design Patterns
Here are some typical questions you might encounter in a technical interview:
- Can you explain the Singleton pattern and when you would use it?
- What’s the difference between the Factory Method and Abstract Factory patterns?
- How does the Observer pattern work, and what are its advantages and disadvantages?
- Can you give an example of when you’ve used the Strategy pattern in your work?
- How does the Decorator pattern differ from simple inheritance?
- What’s the purpose of the Adapter pattern, and when would you use it?
- Can you explain the concept of loose coupling and how design patterns help achieve it?
- How would you implement a thread-safe Singleton in Java?
- What’s the difference between the Composite and Decorator patterns?
- How does the Command pattern help in implementing undo functionality?
6. Implementing Design Patterns in Code
Let’s look at how to implement some common design patterns in Java:
1. Singleton Pattern
public class Singleton {
private static Singleton instance;
private Singleton() {}
public static synchronized Singleton getInstance() {
if (instance == null) {
instance = new Singleton();
}
return instance;
}
}
2. Factory Method Pattern
interface Animal {
void makeSound();
}
class Dog implements Animal {
public void makeSound() {
System.out.println("Woof");
}
}
class Cat implements Animal {
public void makeSound() {
System.out.println("Meow");
}
}
class AnimalFactory {
public Animal getAnimal(String animalType) {
if (animalType == null) {
return null;
}
if (animalType.equalsIgnoreCase("DOG")) {
return new Dog();
} else if (animalType.equalsIgnoreCase("CAT")) {
return new Cat();
}
return null;
}
}
3. Observer Pattern
import java.util.ArrayList;
import java.util.List;
interface Observer {
void update(String message);
}
class Subject {
private List<Observer> observers = new ArrayList<>();
private String message;
public void attach(Observer observer) {
observers.add(observer);
}
public void notifyAllObservers() {
for (Observer observer : observers) {
observer.update(message);
}
}
public void setMessage(String message) {
this.message = message;
notifyAllObservers();
}
}
class ConcreteObserver implements Observer {
private String name;
public ConcreteObserver(String name) {
this.name = name;
}
@Override
public void update(String message) {
System.out.println(name + " received message: " + message);
}
}
7. Best Practices for Using Design Patterns
While design patterns are powerful tools, it’s important to use them judiciously. Here are some best practices to keep in mind:
1. Don’t Force It
Use design patterns only when they are appropriate for your problem. Don’t try to force a design pattern into a situation where it doesn’t fit.
2. Understand the Problem First
Before applying a design pattern, make sure you fully understand the problem you’re trying to solve. The pattern should be a natural fit for the problem, not a forced solution.
3. Keep It Simple
Start with the simplest solution that could possibly work. Only use a design pattern if it truly simplifies your code or solves a specific problem you’re facing.
4. Consider the Trade-offs
Every design pattern comes with its own set of trade-offs. Make sure you understand these trade-offs and that they are acceptable for your specific use case.
5. Combine Patterns
Often, the best solutions come from combining multiple design patterns. Don’t be afraid to use patterns in conjunction with each other when appropriate.
6. Document Your Use of Patterns
When you use a design pattern, make sure to document it in your code comments. This helps other developers (including your future self) understand your design decisions.
7. Stay Updated
Design patterns evolve over time, and new patterns emerge. Stay updated with the latest developments in software design patterns.
8. Anti-Patterns: What to Avoid
Just as important as knowing which patterns to use is understanding which patterns to avoid. Anti-patterns are common responses to recurring problems that are usually ineffective and risk being highly counterproductive.
Common Anti-Patterns:
- God Object: A single class that tries to do too much, often violating the Single Responsibility Principle.
- Spaghetti Code: Code that’s hard to read, understand, or maintain due to its complex or tangled control structure.
- Golden Hammer: Trying to solve every problem with a familiar tool or technique, even when it’s not the best fit.
- Premature Optimization: Optimizing code before it’s necessary, often leading to more complex and harder to maintain code.
- Copy-Paste Programming: Copying and pasting code instead of creating reusable components or applying appropriate design patterns.
- Reinventing the Wheel: Solving a problem from scratch when a well-known solution already exists.
- Boat Anchor: Keeping parts of a system that are no longer useful or necessary.
Avoiding these anti-patterns is just as crucial as implementing good design patterns. They can help you write cleaner, more maintainable code and avoid common pitfalls in software development.
9. Resources for Learning Design Patterns
To deepen your understanding of design patterns, here are some valuable resources:
Books:
- “Design Patterns: Elements of Reusable Object-Oriented Software” by Erich Gamma, Richard Helm, Ralph Johnson, and John Vlissides (the “Gang of Four” book)
- “Head First Design Patterns” by Eric Freeman, Elisabeth Robson, Bert Bates, and Kathy Sierra
- “Patterns of Enterprise Application Architecture” by Martin Fowler
Online Courses:
- Coursera: “Design Patterns” by University of Alberta
- Udemy: “Design Patterns in Java” by Dmitri Nesteruk
- Pluralsight: “Design Patterns Library” by various authors
Websites:
- Refactoring.Guru: Excellent explanations and examples of design patterns
- SourceMaking: Comprehensive guide to design patterns, anti-patterns, and refactoring
- DoFactory: .NET-focused design pattern tutorials
GitHub Repositories:
- Java Design Patterns: Implementation of design patterns in Java
- Design Patterns for Humans: An ultra-simplified explanation of design patterns
10. Conclusion
Design patterns are an essential part of a software developer’s toolkit. They provide proven solutions to common problems, enhance code reusability and maintainability, and demonstrate a deep understanding of software design principles. In the context of technical interviews, especially for positions at major tech companies, knowledge of design patterns can set you apart as a candidate who not only codes but also designs robust, scalable solutions.
However, it’s crucial to remember that design patterns are not a silver bullet. They should be applied judiciously, with a clear understanding of the problem at hand and the trade-offs involved. Overuse or misuse of design patterns can lead to unnecessarily complex code that’s hard to understand and maintain.
As you continue your journey in software development, make it a point to regularly study and practice implementing different design patterns. Try to identify situations in your day-to-day coding where a particular pattern might be beneficial. Over time, this will become second nature, and you’ll find yourself naturally reaching for the right pattern when faced with a design challenge.
Remember, the goal is not to use as many design patterns as possible, but to write clean, maintainable, and efficient code. Design patterns are tools to help you achieve this goal. With practice and experience, you’ll develop the wisdom to know when to apply a pattern and when a simpler solution might suffice.
Happy coding, and may your designs always be patterns of excellence!