Mastering Python for Coding Interviews: A Comprehensive Guide
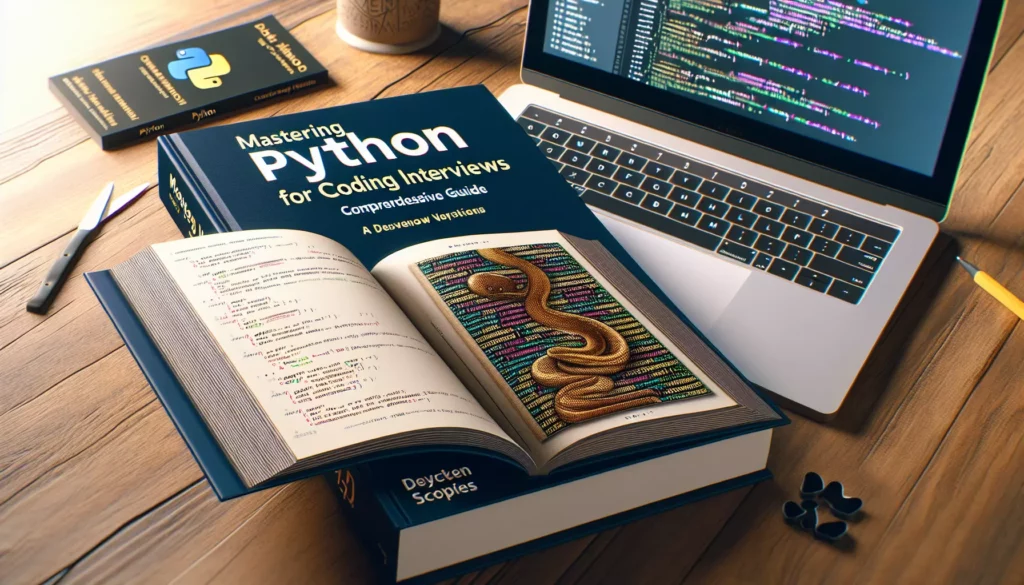
In today’s competitive tech landscape, securing a position at top companies like Google, Amazon, or Facebook often requires passing rigorous coding interviews. Python, with its simplicity and versatility, has become a popular language choice for these interviews. This comprehensive guide will walk you through the essential steps to prepare for coding interviews using Python, helping you build the skills and confidence needed to excel.
Table of Contents
- Why Python for Coding Interviews?
- Mastering Python Fundamentals
- Essential Data Structures in Python
- Key Algorithms to Master
- Developing Problem-Solving Skills
- Understanding Time and Space Complexity
- Practice Resources and Platforms
- Conducting Mock Interviews
- Common Pitfalls to Avoid
- Preparing for Interview Day
- Conclusion
1. Why Python for Coding Interviews?
Python has gained immense popularity in coding interviews for several reasons:
- Readability: Python’s clean and straightforward syntax makes it easy to write and understand code quickly.
- Versatility: It’s suitable for various domains, from web development to data science and machine learning.
- Rich Standard Library: Python comes with a comprehensive standard library, reducing the need for external dependencies.
- Rapid Prototyping: Python allows for quick implementation of ideas, which is crucial in time-constrained interview settings.
- Wide Acceptance: Many top tech companies use Python in their tech stacks and allow its use in interviews.
2. Mastering Python Fundamentals
Before diving into complex algorithms, ensure you have a solid grasp of Python basics:
Variables and Data Types
Understand how to work with integers, floats, strings, booleans, and None.
Control Structures
Master if-else statements, for and while loops, and list comprehensions.
Functions
Learn to define and use functions, including lambda functions and built-in functions.
Object-Oriented Programming
Familiarize yourself with classes, objects, inheritance, and polymorphism.
Exception Handling
Know how to use try-except blocks to handle errors gracefully.
Example: Basic Python Concepts
# Variables and data types
x = 5
y = 3.14
name = "Alice"
is_student = True
# Control structures
if x > 0:
print("Positive number")
else:
print("Non-positive number")
for i in range(5):
print(i)
# Functions
def greet(name):
return f"Hello, {name}!"
print(greet("Bob"))
# Lambda function
square = lambda x: x ** 2
print(square(4))
# List comprehension
squares = [x**2 for x in range(10)]
print(squares)
# Object-Oriented Programming
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
def introduce(self):
return f"My name is {self.name} and I'm {self.age} years old."
alice = Person("Alice", 30)
print(alice.introduce())
# Exception handling
try:
result = 10 / 0
except ZeroDivisionError:
print("Cannot divide by zero!")
3. Essential Data Structures in Python
Familiarity with Python’s built-in data structures is crucial for coding interviews. Focus on:
Lists
Dynamic arrays that can hold elements of different types.
Tuples
Immutable sequences, often used for fixed collections of elements.
Dictionaries
Key-value pairs for fast lookups and efficient data organization.
Sets
Unordered collections of unique elements, useful for quick membership tests.
Strings
Immutable sequences of characters with various built-in methods.
Example: Working with Data Structures
# Lists
fruits = ["apple", "banana", "cherry"]
fruits.append("date")
print(fruits[1]) # Output: banana
# Tuples
coordinates = (10, 20)
x, y = coordinates
print(f"X: {x}, Y: {y}")
# Dictionaries
person = {"name": "John", "age": 30, "city": "New York"}
print(person["name"])
person["job"] = "Engineer"
# Sets
unique_numbers = {1, 2, 3, 4, 5, 5, 4, 3}
print(unique_numbers) # Output: {1, 2, 3, 4, 5}
# Strings
message = "Hello, World!"
print(message.lower())
print(message.split(", "))
4. Key Algorithms to Master
Understanding and implementing common algorithms is essential for coding interviews. Focus on:
Sorting Algorithms
- Quick Sort
- Merge Sort
- Bubble Sort
- Insertion Sort
Searching Algorithms
- Binary Search
- Depth-First Search (DFS)
- Breadth-First Search (BFS)
Graph Algorithms
- Dijkstra’s Algorithm
- Floyd-Warshall Algorithm
- Kruskal’s Algorithm
Dynamic Programming
- Fibonacci Sequence
- Longest Common Subsequence
- Knapsack Problem
Example: Implementing Binary Search
def binary_search(arr, target):
left, right = 0, len(arr) - 1
while left <= right:
mid = (left + right) // 2
if arr[mid] == target:
return mid
elif arr[mid] < target:
left = mid + 1
else:
right = mid - 1
return -1 # Target not found
# Example usage
sorted_array = [1, 3, 5, 7, 9, 11, 13, 15]
target = 7
result = binary_search(sorted_array, target)
print(f"Target {target} found at index: {result}")
5. Developing Problem-Solving Skills
Coding interviews are not just about knowing algorithms; they test your problem-solving abilities. Here are some strategies to improve:
Understand the Problem
Take time to fully grasp the problem statement. Ask clarifying questions if needed.
Break It Down
Divide complex problems into smaller, manageable sub-problems.
Plan Your Approach
Outline your solution before coding. Consider different approaches and their trade-offs.
Start with a Brute Force Solution
Begin with a simple, working solution, then optimize if time allows.
Test Your Solution
Consider edge cases and test your code with various inputs.
Example: Problem-Solving Approach
Let’s solve the “Two Sum” problem: Given an array of integers and a target sum, return indices of two numbers that add up to the target.
def two_sum(nums, target):
# Step 1: Understand the problem
# We need to find two numbers in the array that sum up to the target
# Step 2: Consider approaches
# Brute force: Check all pairs (O(n^2))
# Optimized: Use a hash table for O(n) time complexity
# Step 3: Implement the optimized solution
num_dict = {}
for i, num in enumerate(nums):
complement = target - num
if complement in num_dict:
return [num_dict[complement], i]
num_dict[num] = i
# Step 4: Handle the case where no solution is found
return []
# Step 5: Test the solution
nums = [2, 7, 11, 15]
target = 9
result = two_sum(nums, target)
print(f"Indices of two numbers that add up to {target}: {result}")
6. Understanding Time and Space Complexity
Analyzing the efficiency of your algorithms is crucial in coding interviews. You should be able to:
Analyze Time Complexity
Understand Big O notation and how to calculate the time complexity of your algorithms.
Evaluate Space Complexity
Consider the memory usage of your solutions, especially for large inputs.
Common Time Complexities
- O(1): Constant time
- O(log n): Logarithmic time (e.g., binary search)
- O(n): Linear time
- O(n log n): Linearithmic time (e.g., efficient sorting algorithms)
- O(n^2): Quadratic time
- O(2^n): Exponential time
Example: Analyzing Complexity
def find_max(arr):
if not arr:
return None
max_val = arr[0]
for num in arr:
if num > max_val:
max_val = num
return max_val
# Time Complexity: O(n) - we iterate through the array once
# Space Complexity: O(1) - we use a constant amount of extra space
def bubble_sort(arr):
n = len(arr)
for i in range(n):
for j in range(0, n - i - 1):
if arr[j] > arr[j + 1]:
arr[j], arr[j + 1] = arr[j + 1], arr[j]
return arr
# Time Complexity: O(n^2) - nested loops
# Space Complexity: O(1) - in-place sorting
7. Practice Resources and Platforms
Regular practice is key to mastering coding interviews. Here are some valuable resources:
Online Platforms
- LeetCode: Offers a wide range of coding problems with varying difficulty levels.
- HackerRank: Provides coding challenges and competitions.
- CodeSignal: Offers coding assessments and interview practice.
Books
- “Cracking the Coding Interview” by Gayle Laakmann McDowell
- “Elements of Programming Interviews in Python” by Adnan Aziz, Tsung-Hsien Lee, and Amit Prakash
Courses
- AlgoCademy: Interactive coding tutorials and interview preparation resources.
- Coursera’s “Algorithms Specialization” by Stanford University
GitHub Repositories
- TheAlgorithms/Python: Collection of algorithms implemented in Python
- donnemartin/interactive-coding-challenges: Interactive Python coding interview challenges
8. Conducting Mock Interviews
Simulating the interview environment is crucial for preparation. Here’s how to conduct effective mock interviews:
Find a Practice Partner
Team up with a friend, classmate, or use platforms like Pramp for peer mock interviews.
Simulate Real Conditions
Use a whiteboard or a simple text editor without auto-completion to mimic interview conditions.
Time Your Sessions
Set a timer to practice working under time constraints.
Practice Thinking Aloud
Verbalize your thought process as you solve problems.
Give and Receive Feedback
After each session, discuss areas of improvement and strengths.
Example: Mock Interview Question
Here’s a sample question you might encounter in a mock interview:
# Problem: Implement a function to check if a binary tree is balanced.
# A balanced tree is defined to be a tree such that the heights of the
# two subtrees of any node never differ by more than one.
class TreeNode:
def __init__(self, val=0, left=None, right=None):
self.val = val
self.left = left
self.right = right
def is_balanced(root):
def check_height(node):
if not node:
return 0
left_height = check_height(node.left)
if left_height == -1:
return -1
right_height = check_height(node.right)
if right_height == -1:
return -1
if abs(left_height - right_height) > 1:
return -1
return max(left_height, right_height) + 1
return check_height(root) != -1
# Test the function
root = TreeNode(1)
root.left = TreeNode(2)
root.right = TreeNode(3)
root.left.left = TreeNode(4)
root.left.right = TreeNode(5)
print(is_balanced(root)) # Should return True
9. Common Pitfalls to Avoid
Being aware of common mistakes can help you perform better in coding interviews:
Not Clarifying the Problem
Always ask questions to fully understand the problem before starting to code.
Jumping to Code Too Quickly
Take time to plan your approach before writing code.
Ignoring Edge Cases
Consider and handle edge cases in your solution.
Poor Time Management
Practice solving problems within time constraints to improve your pacing.
Neglecting Code Quality
Write clean, readable code even under pressure.
Not Testing Your Code
Always test your solution with various inputs, including edge cases.
Example: Handling Edge Cases
def divide_numbers(a, b):
# Poor implementation
return a / b
# Better implementation with edge case handling
def divide_numbers_safe(a, b):
if b == 0:
raise ValueError("Cannot divide by zero")
return a / b
# Test cases
print(divide_numbers_safe(10, 2)) # Output: 5.0
try:
print(divide_numbers_safe(10, 0))
except ValueError as e:
print(f"Error: {e}") # Output: Error: Cannot divide by zero
10. Preparing for Interview Day
As the interview day approaches, follow these tips to ensure you’re at your best:
Review Key Concepts
Go over important algorithms, data structures, and problem-solving techniques.
Prepare Your Environment
Ensure your computer, internet connection, and any required software are working properly.
Get a Good Night’s Sleep
Being well-rested is crucial for clear thinking during the interview.
Arrive Early or Log In Early
For in-person interviews, arrive at least 15 minutes early. For virtual interviews, log in 5-10 minutes before the scheduled time.
Bring Necessary Items
For in-person interviews, bring a notepad, pen, and any required documents.
Stay Calm and Confident
Take deep breaths and remember that you’ve prepared well.
Example: Pre-Interview Checklist
Interview Preparation Checklist:
[ ] Review common algorithms and data structures
[ ] Practice explaining your thought process out loud
[ ] Prepare questions to ask the interviewer
[ ] Test your computer and internet connection (for virtual interviews)
[ ] Have a glass of water nearby
[ ] Review the job description and company information
[ ] Prepare a brief introduction about yourself
[ ] Get a good night's sleep
[ ] Set multiple alarms to wake up on time
[ ] Dress appropriately (even for virtual interviews)
[ ] Have a backup plan for technical issues (e.g., phone number to call)
11. Conclusion
Preparing for coding interviews in Python requires dedication, practice, and a structured approach. By mastering Python fundamentals, key data structures and algorithms, and honing your problem-solving skills, you’ll be well-equipped to tackle even the most challenging interview questions.
Remember that the journey to becoming proficient in coding interviews is a marathon, not a sprint. Consistent practice, learning from your mistakes, and continuously challenging yourself will lead to improvement over time.
As you prepare, leverage resources like AlgoCademy, which offers interactive coding tutorials and personalized learning paths to help you progress from beginner-level coding to mastering technical interviews. With AI-powered assistance and step-by-step guidance, you can efficiently build the skills needed to excel in interviews at top tech companies.
Stay motivated, embrace the learning process, and approach each practice problem as an opportunity to grow. With persistence and the right preparation, you’ll be well on your way to acing your Python coding interviews and landing your dream job in the tech industry.