How to Practice Coding Challenges Without Getting Overwhelmed: A Comprehensive Guide
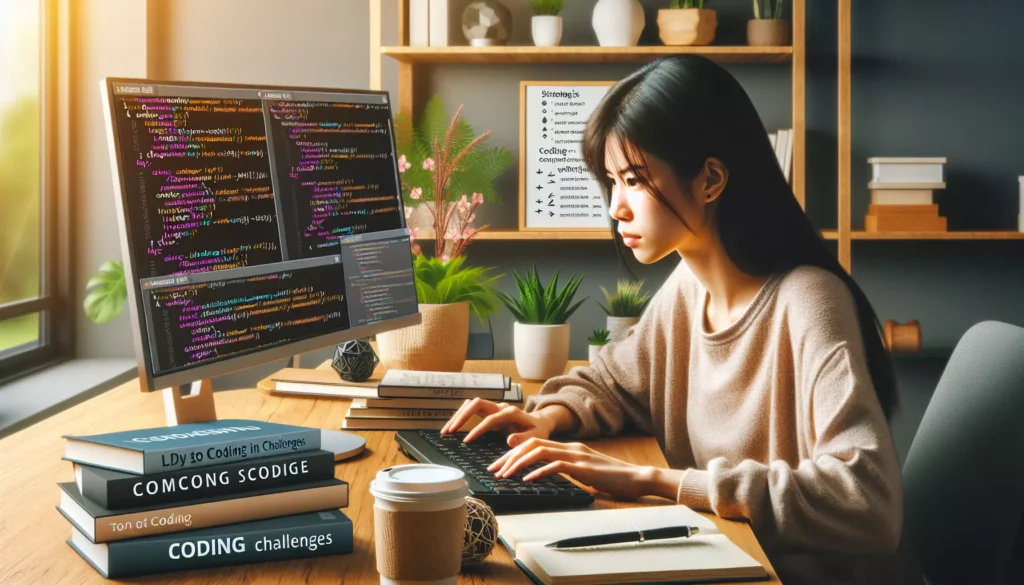
In the ever-evolving world of technology, coding skills have become increasingly valuable. Whether you’re a beginner looking to break into the tech industry or an experienced developer aiming to land a job at a major tech company, mastering coding challenges is crucial. However, the sheer volume of information and the complexity of algorithms can often leave learners feeling overwhelmed. This comprehensive guide will walk you through effective strategies to practice coding challenges without losing your motivation or burning out.
1. Understanding the Importance of Coding Challenges
Before diving into the strategies, it’s essential to understand why coding challenges are so important:
- They improve your problem-solving skills
- They help you think algorithmically
- They prepare you for technical interviews, especially at FAANG companies
- They enhance your coding efficiency and speed
- They expose you to a wide range of programming concepts and patterns
Recognizing these benefits can help you stay motivated during your learning journey.
2. Start with the Basics
One of the most common mistakes learners make is jumping into complex algorithms without a solid foundation. To avoid feeling overwhelmed, start with the basics:
2.1. Master the Fundamentals
Ensure you have a strong grasp of fundamental programming concepts such as:
- Variables and data types
- Control structures (if-else statements, loops)
- Functions and methods
- Arrays and lists
- Basic object-oriented programming concepts
2.2. Learn Basic Data Structures
Familiarize yourself with essential data structures:
- Arrays
- Linked Lists
- Stacks
- Queues
- Hash Tables
2.3. Understand Time and Space Complexity
Grasp the basics of Big O notation to analyze the efficiency of your algorithms.
3. Develop a Structured Learning Plan
Having a well-organized learning plan can significantly reduce feelings of being overwhelmed. Here’s how to create one:
3.1. Set Realistic Goals
Break down your learning into manageable chunks. For example:
- Week 1-2: Master array manipulation
- Week 3-4: Dive into string algorithms
- Week 5-6: Explore tree and graph algorithms
3.2. Allocate Dedicated Study Time
Consistency is key. Set aside specific times for coding practice, even if it’s just 30 minutes a day.
3.3. Use Spaced Repetition
Revisit concepts and problems at increasing intervals to reinforce your learning and improve long-term retention.
4. Leverage Online Resources and Platforms
Take advantage of the wealth of online resources available for coding practice:
4.1. Coding Platforms
Utilize platforms like AlgoCademy, LeetCode, HackerRank, or CodeSignal to access a wide range of coding challenges.
4.2. Interactive Tutorials
Engage with interactive coding tutorials that provide step-by-step guidance and immediate feedback.
4.3. Video Courses
Supplement your practice with video courses from platforms like Coursera, edX, or Udemy to gain a deeper understanding of algorithms and data structures.
5. Focus on Problem-Solving Techniques
Developing strong problem-solving skills is crucial for tackling coding challenges effectively:
5.1. Understand the Problem
Before writing any code, make sure you fully understand the problem statement. Break it down into smaller components and identify the inputs, outputs, and constraints.
5.2. Plan Your Approach
Outline your solution strategy before coding. This can include:
- Drawing diagrams or flowcharts
- Writing pseudocode
- Identifying potential edge cases
5.3. Implement the Solution
Once you have a clear plan, start coding your solution. Focus on writing clean, readable code.
5.4. Test and Debug
Test your solution with various inputs, including edge cases. Learn to use debugging tools effectively to identify and fix errors.
5.5. Optimize and Refactor
After getting a working solution, look for ways to optimize it for better time or space complexity. Refactor your code to improve readability and efficiency.
6. Practice Regularly and Consistently
Consistency is key to making progress without feeling overwhelmed:
6.1. Start Small
Begin with easier problems and gradually increase the difficulty level as you gain confidence.
6.2. Set a Daily or Weekly Goal
Aim to solve a certain number of problems each day or week. For example, start with 1-2 problems per day and increase as you become more comfortable.
6.3. Track Your Progress
Keep a log of the problems you’ve solved, noting any difficulties you encountered and how you overcame them.
7. Learn from Solutions and Discussions
Don’t just focus on solving problems; learn from others as well:
7.1. Study Official Solutions
After solving a problem, compare your solution with the official one or highly-rated community solutions.
7.2. Participate in Discussion Forums
Engage in community discussions to gain insights into different approaches and learn from others’ experiences.
7.3. Explain Your Solutions
Practice explaining your solutions to others, either in writing or verbally. This helps reinforce your understanding and improves your ability to communicate technical concepts.
8. Focus on Patterns and Categories
Grouping problems into categories can make the learning process more manageable:
8.1. Identify Common Patterns
Recognize recurring patterns in problem-solving, such as:
- Two-pointer technique
- Sliding window
- Divide and conquer
- Dynamic programming
8.2. Study One Category at a Time
Focus on mastering one category of problems before moving to the next. For example, spend a week on array problems, then move to string manipulation, and so on.
8.3. Create Cheat Sheets
Develop your own cheat sheets or quick reference guides for different problem types and their common solutions.
9. Utilize AI-Powered Assistance
Take advantage of AI-powered tools to enhance your learning experience:
9.1. AI Code Completion
Use AI-powered code completion tools to help you write code faster and learn best practices.
9.2. Personalized Learning Paths
Leverage AI algorithms that can suggest personalized learning paths based on your progress and areas for improvement.
9.3. Automated Code Review
Use AI-powered code review tools to get instant feedback on your code quality and suggestions for improvement.
10. Simulate Interview Conditions
As you progress, start preparing for technical interviews:
10.1. Time Your Problem-Solving
Practice solving problems within time constraints to simulate interview conditions.
10.2. Mock Interviews
Participate in mock interviews, either with peers or using online platforms that offer this service.
10.3. Verbalize Your Thought Process
Practice explaining your thought process out loud as you solve problems, as this is a crucial skill in technical interviews.
11. Take Care of Your Mental Health
Avoiding burnout is crucial for long-term success:
11.1. Take Regular Breaks
Use techniques like the Pomodoro method to ensure you’re taking regular breaks during your study sessions.
11.2. Celebrate Small Wins
Acknowledge and celebrate your progress, no matter how small. This helps maintain motivation and a positive attitude.
11.3. Practice Mindfulness
Incorporate mindfulness techniques or meditation to manage stress and maintain focus.
12. Join a Community
Being part of a community can provide support and motivation:
12.1. Online Forums
Participate in coding forums or communities like Stack Overflow or Reddit’s programming subreddits.
12.2. Study Groups
Form or join study groups to collaborate on problem-solving and share knowledge.
12.3. Attend Coding Events
Participate in hackathons, coding competitions, or local tech meetups to connect with other learners and professionals.
13. Apply Your Skills to Real Projects
Put your skills into practice with real-world applications:
13.1. Personal Projects
Develop personal projects that interest you and incorporate the algorithms and data structures you’ve learned.
13.2. Open Source Contributions
Contribute to open-source projects to gain experience working with large codebases and collaborating with other developers.
13.3. Coding Challenges
Participate in online coding challenges or competitions to test your skills in a more dynamic environment.
Conclusion
Mastering coding challenges is a journey that requires patience, persistence, and a structured approach. By following these strategies, you can make steady progress without feeling overwhelmed. Remember that everyone learns at their own pace, and it’s okay to take your time to truly understand each concept.
As you continue your learning journey, platforms like AlgoCademy can provide valuable resources, interactive tutorials, and AI-powered assistance to help you progress from beginner-level coding to preparing for technical interviews at major tech companies. With consistent practice and the right mindset, you’ll be well-equipped to tackle even the most challenging coding problems and advance your programming career.
Stay motivated, embrace the learning process, and remember that every problem you solve brings you one step closer to your goals. Happy coding!