Understanding Trie Data Structures for Coding Interviews
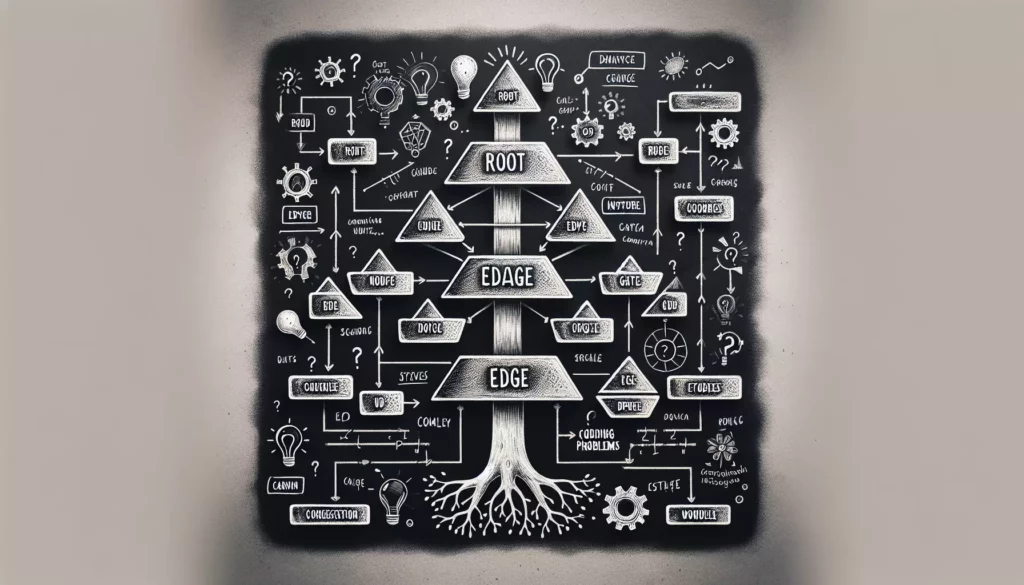
When preparing for coding interviews, especially those at major tech companies like FAANG (Facebook, Amazon, Apple, Netflix, and Google), it’s crucial to have a solid understanding of various data structures. One such structure that often appears in technical interviews is the trie, also known as a prefix tree. In this comprehensive guide, we’ll dive deep into trie data structures, exploring their implementation, use cases, and common interview questions.
What is a Trie?
A trie, derived from the word “retrieval,” is an efficient tree-like data structure used primarily for storing and searching strings. Unlike binary trees or hash tables, tries are particularly well-suited for tasks involving prefix matching and string manipulation.
The key features of a trie include:
- Each node in the trie represents a single character of a string
- The root node is typically empty
- Each path from the root to a node represents a prefix of one or more strings
- Leaf nodes or specially marked nodes indicate the end of a complete word
Basic Structure of a Trie Node
Before we dive into the implementation, let’s understand the basic structure of a trie node. In most cases, a trie node contains the following components:
- A boolean flag to indicate if the node represents the end of a word
- An array or map to store child nodes (usually 26 for lowercase English letters)
Here’s a simple implementation of a TrieNode class in Java:
class TrieNode {
boolean isEndOfWord;
TrieNode[] children;
public TrieNode() {
isEndOfWord = false;
children = new TrieNode[26]; // Assuming lowercase English letters
for (int i = 0; i < 26; i++) {
children[i] = null;
}
}
}
Implementing a Trie
Now that we have our TrieNode structure, let’s implement the basic operations of a trie: insertion, search, and prefix search.
1. Insertion
To insert a word into the trie, we start from the root and traverse down, creating new nodes for characters that don’t exist and marking the last node as the end of the word.
class Trie {
private TrieNode root;
public Trie() {
root = new TrieNode();
}
public void insert(String word) {
TrieNode current = root;
for (char ch : word.toCharArray()) {
int index = ch - 'a';
if (current.children[index] == null) {
current.children[index] = new TrieNode();
}
current = current.children[index];
}
current.isEndOfWord = true;
}
}
2. Search
Searching for a word involves traversing the trie along the path defined by the characters of the word. If we reach the end of the word and the last node is marked as an end of word, the search is successful.
public boolean search(String word) {
TrieNode node = searchNode(word);
return node != null && node.isEndOfWord;
}
private TrieNode searchNode(String word) {
TrieNode current = root;
for (char ch : word.toCharArray()) {
int index = ch - 'a';
if (current.children[index] == null) {
return null;
}
current = current.children[index];
}
return current;
}
3. Prefix Search
Prefix search is similar to the regular search, but we don’t need to check if the last node is marked as an end of word.
public boolean startsWith(String prefix) {
return searchNode(prefix) != null;
}
Time and Space Complexity
Understanding the time and space complexity of trie operations is crucial for coding interviews. Let’s break it down:
Time Complexity:
- Insertion: O(m), where m is the length of the word being inserted
- Search: O(m), where m is the length of the word being searched
- Prefix Search: O(p), where p is the length of the prefix
Space Complexity:
The space complexity of a trie depends on the number of nodes. In the worst case, where no words share prefixes, the space complexity can be O(n*m), where n is the number of words and m is the average length of the words.
Advantages and Disadvantages of Tries
Like any data structure, tries have their pros and cons. Understanding these can help you decide when to use a trie in your solutions during coding interviews.
Advantages:
- Fast prefix searching: O(m) time complexity, where m is the length of the prefix
- Efficient for string-related problems, especially those involving prefixes
- Can be more space-efficient than hash tables when many keys share prefixes
- Supports ordered traversal of keys
Disadvantages:
- Can be memory-intensive, especially if there are few common prefixes
- Slower than hash tables for simple key lookups
- Implementation can be more complex compared to other data structures
Common Interview Questions Involving Tries
Now that we have a solid understanding of tries, let’s look at some common interview questions that can be efficiently solved using this data structure.
1. Implement an Autocomplete System
Problem: Design a system that can suggest word completions based on a given prefix.
Solution Approach: Use a trie to store all words. When given a prefix, traverse the trie to the node representing the end of the prefix, then perform a depth-first search from that node to find all complete words.
public List<String> autocomplete(String prefix) {
List<String> results = new ArrayList<>();
TrieNode node = searchNode(prefix);
if (node != null) {
dfsCollectWords(node, prefix, results);
}
return results;
}
private void dfsCollectWords(TrieNode node, String currentWord, List<String> results) {
if (node.isEndOfWord) {
results.add(currentWord);
}
for (int i = 0; i < 26; i++) {
if (node.children[i] != null) {
dfsCollectWords(node.children[i], currentWord + (char)('a' + i), results);
}
}
}
2. Word Search II
Problem: Given a 2D board of characters and a list of words, find all words on the board. The words can be constructed from letters of sequentially adjacent cells, where adjacent cells are horizontally or vertically neighboring. The same letter cell may not be used more than once in a word.
Solution Approach: Build a trie with the given words, then perform a depth-first search on the board, using the trie to prune unnecessary paths.
public List<String> findWords(char[][] board, String[] words) {
Trie trie = new Trie();
for (String word : words) {
trie.insert(word);
}
Set<String> result = new HashSet<>();
int m = board.length, n = board[0].length;
boolean[][] visited = new boolean[m][n];
for (int i = 0; i < m; i++) {
for (int j = 0; j < n; j++) {
dfs(board, i, j, trie.root, "", result, visited);
}
}
return new ArrayList<>(result);
}
private void dfs(char[][] board, int i, int j, TrieNode node, String word, Set<String> result, boolean[][] visited) {
if (i < 0 || j < 0 || i >= board.length || j >= board[0].length || visited[i][j]) {
return;
}
char ch = board[i][j];
int index = ch - 'a';
if (node.children[index] == null) {
return;
}
visited[i][j] = true;
node = node.children[index];
word += ch;
if (node.isEndOfWord) {
result.add(word);
}
dfs(board, i+1, j, node, word, result, visited);
dfs(board, i-1, j, node, word, result, visited);
dfs(board, i, j+1, node, word, result, visited);
dfs(board, i, j-1, node, word, result, visited);
visited[i][j] = false;
}
3. Longest Common Prefix
Problem: Write a function to find the longest common prefix string amongst an array of strings.
Solution Approach: Insert all words into a trie. The longest common prefix is the path from the root to the first node that has more than one child or is marked as the end of a word.
public String longestCommonPrefix(String[] strs) {
if (strs == null || strs.length == 0) {
return "";
}
Trie trie = new Trie();
for (String str : strs) {
trie.insert(str);
}
return trie.longestCommonPrefix();
}
// Add this method to the Trie class
public String longestCommonPrefix() {
StringBuilder prefix = new StringBuilder();
TrieNode current = root;
while (true) {
if (current.isEndOfWord) {
break;
}
int childCount = 0;
char nextChar = 'a';
for (int i = 0; i < 26; i++) {
if (current.children[i] != null) {
childCount++;
nextChar = (char)('a' + i);
}
}
if (childCount != 1) {
break;
}
prefix.append(nextChar);
current = current.children[nextChar - 'a'];
}
return prefix.toString();
}
Advanced Trie Concepts
As you progress in your understanding of tries, you may encounter some advanced concepts and variations. Here are a few to be aware of:
1. Compressed Trie (Radix Tree)
A compressed trie, also known as a radix tree, is an optimized version of a trie where nodes with only one child are merged with their parent. This can significantly reduce the space complexity of the trie, especially when dealing with long strings with few common prefixes.
2. Ternary Search Tree
A ternary search tree is a type of trie where each node has three children: left (for smaller values), right (for larger values), and equal (for the next character in the sequence). This structure can be more space-efficient than a standard trie for certain datasets.
3. Suffix Tree
A suffix tree is a compressed trie containing all suffixes of a given string. It’s particularly useful for problems involving substring searches and is often used in bioinformatics for DNA sequence analysis.
Practical Applications of Tries
Understanding the real-world applications of tries can help you appreciate their importance and potentially inspire you to use them in your own projects. Here are some common applications:
- Autocomplete and Predictive Text: As we saw in one of our example problems, tries are excellent for implementing autocomplete features in search engines, text editors, and smartphone keyboards.
- IP Routing Tables: Tries can efficiently store and lookup IP addresses, making them useful in network routers.
- Spell Checkers: Tries can quickly verify if a word exists in a dictionary and suggest corrections for misspelled words.
- Longest Prefix Matching: Used in various networking applications, including IP routing and packet classification.
- Dictionary Implementation: Tries can be used to implement dictionaries with efficient prefix-based operations.
- Full-text Search: In combination with other data structures, tries can be used to implement efficient full-text search engines.
Tips for Trie-based Interview Questions
When tackling trie-based problems in coding interviews, keep these tips in mind:
- Recognize Trie-Suitable Problems: Look for problems involving string prefixes, word searches, or efficient string storage and retrieval.
- Start with the Basic Structure: Begin by implementing the basic trie structure with insert and search operations.
- Consider Space-Time Tradeoffs: Be prepared to discuss the space-time tradeoffs of using a trie versus other data structures like hash tables.
- Practice Implementation: Implement tries from scratch multiple times to become comfortable with the structure.
- Combine with Other Algorithms: Many trie problems involve combining trie operations with depth-first search or breadth-first search.
- Optimize for the Problem: Adapt your trie implementation to the specific requirements of the problem. For example, you might not need a boolean flag for end-of-word in some cases.
Conclusion
Tries are powerful data structures that excel in string-related problems, especially those involving prefixes. By understanding their structure, implementation, and common applications, you’ll be well-prepared to tackle a wide range of coding interview questions.
Remember, the key to mastering tries, like any data structure, is practice. Implement tries from scratch, solve problems using tries, and analyze their performance in different scenarios. With time and effort, you’ll develop the intuition to recognize when a trie is the right tool for the job and how to implement it efficiently.
As you prepare for your coding interviews, particularly for major tech companies, keep tries in your algorithmic toolbox. They may not be as commonly used as arrays or hash tables, but when the right problem comes along, knowing how to implement and use a trie can be the key to an elegant and efficient solution.