How to Deal with Ambiguous Questions in Interviews: A Comprehensive Guide
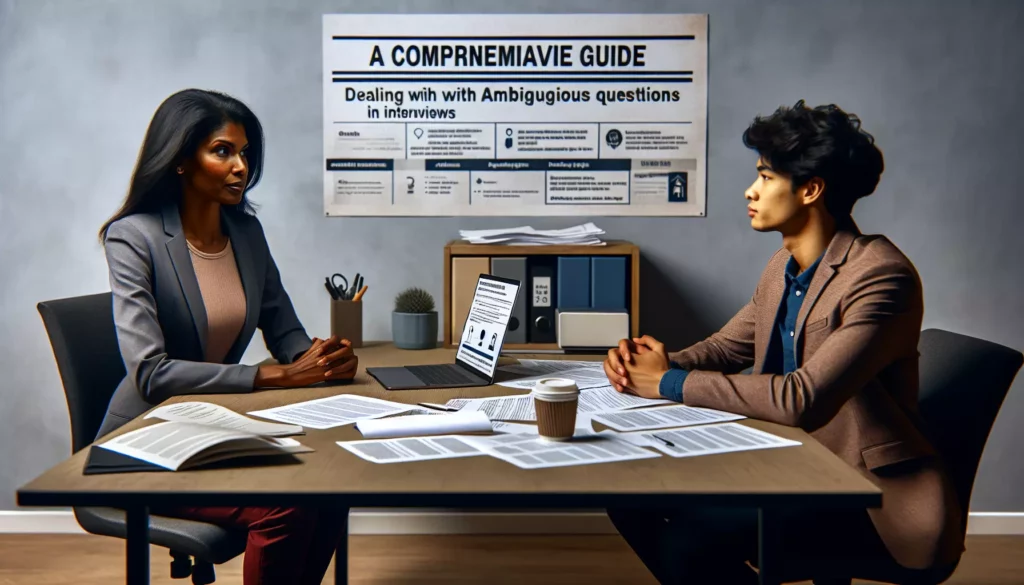
In the world of technical interviews, particularly those conducted by major tech companies like FAANG (Facebook, Amazon, Apple, Netflix, Google), candidates often encounter ambiguous questions. These questions are designed to test not just your coding skills, but also your problem-solving abilities, communication skills, and how you approach uncertainty. In this comprehensive guide, we’ll explore strategies to effectively deal with ambiguous questions in interviews, helping you navigate this challenging aspect of the hiring process.
Understanding Ambiguous Questions
Before we dive into strategies, it’s crucial to understand what ambiguous questions are and why interviewers use them. Ambiguous questions are intentionally vague or open-ended, often lacking specific details or clear requirements. They’re used to:
- Assess your problem-solving approach
- Evaluate your communication skills
- Test your ability to work with incomplete information
- Gauge your creativity and critical thinking
- Observe how you handle uncertainty and pressure
Examples of ambiguous questions might include:
- “How would you design a system to manage traffic lights in a city?”
- “Estimate the number of piano tuners in Chicago.”
- “Design a parking lot system.”
These questions are intentionally broad and lack specific requirements, allowing the interviewer to see how you approach problem-solving in a real-world scenario where information is often incomplete.
Strategies for Handling Ambiguous Questions
1. Don’t Panic – Take a Breath
When faced with an ambiguous question, it’s natural to feel a bit overwhelmed. The key is to remain calm. Take a deep breath and remember that the interviewer isn’t expecting an immediate, perfect answer. They’re more interested in your thought process and how you approach the problem.
2. Clarify the Question
One of the most crucial steps in dealing with ambiguous questions is seeking clarification. Don’t be afraid to ask questions to better understand the problem. This shows that you’re thoughtful and thorough in your approach. Some questions you might ask include:
- “Can you provide more context about the problem?”
- “What are the main goals or objectives we’re trying to achieve?”
- “Are there any specific constraints or requirements I should be aware of?”
- “Who are the primary users or stakeholders for this system/solution?”
Remember, the interviewer may not provide all the answers, but the process of asking these questions demonstrates your analytical skills and attention to detail.
3. State Your Assumptions
After seeking clarification, you’ll likely still need to make some assumptions to proceed. Clearly state these assumptions before diving into your solution. This shows that you’re aware of the gaps in information and are making reasonable judgments to move forward. For example:
“Based on the information provided, I’m assuming that:
- The system needs to handle X number of users
- We’re primarily concerned with performance and scalability
- The solution should be compatible with existing infrastructure”
By stating your assumptions, you’re also giving the interviewer an opportunity to correct any misunderstandings or provide additional information.
4. Break Down the Problem
Once you have a clearer understanding of the question and have stated your assumptions, start breaking down the problem into smaller, manageable components. This approach, often referred to as “divide and conquer,” helps in several ways:
- It makes the problem less overwhelming
- It allows you to focus on one aspect at a time
- It helps in identifying potential challenges or bottlenecks
- It provides a structured approach to your solution
For example, if asked to design a parking lot system, you might break it down into components like:
- Entry and exit points
- Payment system
- Space allocation and tracking
- User interface (for both drivers and parking lot operators)
- Reporting and analytics
5. Think Aloud
As you work through the problem, articulate your thoughts out loud. This gives the interviewer insight into your problem-solving process and allows them to provide guidance if needed. Thinking aloud also helps you organize your thoughts and catch potential issues in your reasoning.
For instance, you might say something like:
“I’m considering using a hash table for quick lookups of available parking spaces. This would give us O(1) time complexity for finding an empty spot. However, I’m wondering if we need to consider the physical layout of the parking lot, which might require a different data structure…”
6. Start with a High-Level Approach
Begin by outlining a high-level approach to the problem. This demonstrates your ability to see the big picture before diving into details. You might use techniques like:
- Flowcharts
- System diagrams
- Pseudocode
For example, if designing a traffic light management system, you might start with a high-level diagram showing the main components:
<!-- ASCII art representation of a system diagram -->
+----------------+ +-------------------+
| Traffic Lights |<-->| Central Controller |
+----------------+ +-------------------+
^ ^
| |
v v
+----------------+ +-------------------+
| Sensors/Cameras| | User Interface |
+----------------+ +-------------------+
7. Consider Multiple Solutions
Don’t settle for the first solution that comes to mind. Consider multiple approaches and discuss their pros and cons. This shows your ability to think critically and evaluate different options. For each solution, consider factors like:
- Time and space complexity
- Scalability
- Maintainability
- Cost-effectiveness
- Potential challenges or limitations
Discussing multiple solutions also demonstrates your breadth of knowledge and flexibility in problem-solving.
8. Use Analogies and Real-World Examples
Drawing parallels to real-world scenarios or existing systems can help clarify your thoughts and make your solution more relatable. For instance, if designing a system to manage traffic lights, you might compare it to how air traffic control systems work, highlighting similarities and differences.
9. Be Prepared to Adapt
As you discuss your solution, the interviewer might introduce new information or constraints. Be prepared to adapt your approach based on this new input. This flexibility shows your ability to handle changing requirements, a valuable skill in real-world software development.
10. Discuss Trade-offs
No solution is perfect, and it’s important to acknowledge the trade-offs in your approach. Discuss the pros and cons of your solution, and explain why you’ve chosen certain trade-offs over others. This demonstrates your ability to make informed decisions and your understanding of the complexities involved in software design.
Practical Example: Designing a Parking Lot System
Let’s walk through a practical example of how to approach an ambiguous question in an interview. The question is: “Design a parking lot system.”
Step 1: Clarify the Question
You might ask:
- “Is this for a specific type of parking lot (e.g., airport, shopping mall, residential)?”
- “What are the main features we need to consider (e.g., payment, space allocation, reporting)?”
- “Are there any specific constraints or requirements (e.g., size, technology limitations)?”
Step 2: State Assumptions
Based on the interviewer’s responses, you might state:
“I’ll assume we’re designing for a multi-level parking lot in a shopping mall. The system should handle entry/exit, payment, and space allocation. We’ll need to support multiple payment methods and provide real-time availability information.”
Step 3: Break Down the Problem
You could break it down into these components:
- Entry and Exit System
- Space Allocation and Tracking
- Payment System
- User Interface
- Reporting and Analytics
Step 4: High-Level Design
Start with a high-level system diagram:
<!-- ASCII art representation of a parking lot system -->
+------------+ +-----------------+ +----------------+
| Entry/Exit |<--->| Central System |<--->| Space Tracking |
+------------+ +-----------------+ +----------------+
^
|
v
+------------+ +-----------------+ +----------------+
| Payment |<--->| User Interface |<--->| Reporting |
+------------+ +-----------------+ +----------------+
Step 5: Detailed Design
Now, you can dive into the details of each component. For example, for the Space Allocation and Tracking system:
class ParkingLot:
def __init__(self, levels, spots_per_level):
self.levels = [Level(i, spots_per_level) for i in range(levels)]
def park_vehicle(self, vehicle):
for level in self.levels:
spot = level.find_available_spot(vehicle.type)
if spot:
spot.occupy(vehicle)
return True
return False
def remove_vehicle(self, vehicle):
for level in self.levels:
if level.remove_vehicle(vehicle):
return True
return False
class Level:
def __init__(self, level_number, spots):
self.level_number = level_number
self.spots = [ParkingSpot(i) for i in range(spots)]
def find_available_spot(self, vehicle_type):
for spot in self.spots:
if spot.is_available() and spot.can_fit(vehicle_type):
return spot
return None
def remove_vehicle(self, vehicle):
for spot in self.spots:
if spot.vehicle == vehicle:
spot.remove_vehicle()
return True
return False
class ParkingSpot:
def __init__(self, spot_number):
self.spot_number = spot_number
self.vehicle = None
def is_available(self):
return self.vehicle is None
def can_fit(self, vehicle_type):
# Logic to check if the spot can accommodate the vehicle type
pass
def occupy(self, vehicle):
self.vehicle = vehicle
def remove_vehicle(self):
self.vehicle = None
Step 6: Discuss Trade-offs
As you explain your design, discuss trade-offs. For example:
“This design allows for efficient space allocation with O(n) time complexity where n is the number of spots. However, it might not be optimal for very large parking lots. For larger scales, we might consider using a more sophisticated data structure like a binary search tree to reduce search time to O(log n).”
Common Pitfalls to Avoid
When dealing with ambiguous questions in interviews, be aware of these common pitfalls:
1. Rushing to Code
While it’s important to demonstrate your coding skills, rushing to write code without fully understanding the problem can lead to inefficient or incorrect solutions. Take the time to clarify the question and plan your approach before coding.
2. Failing to Communicate
Remember, the interviewer can’t read your mind. Even if you’re on the right track, failing to communicate your thoughts clearly can work against you. Practice explaining your thought process out loud.
3. Ignoring Constraints
Pay attention to any constraints mentioned by the interviewer, even if they seem minor. These constraints often play a crucial role in shaping the optimal solution.
4. Not Considering Edge Cases
When designing your solution, think about potential edge cases or unusual scenarios. Discussing these shows your attention to detail and thoroughness.
5. Sticking to One Approach
If you’re struggling with one approach, don’t be afraid to step back and consider alternatives. Flexibility and the ability to pivot when necessary are valuable skills.
Preparing for Ambiguous Questions
While you can’t predict exactly what ambiguous questions you’ll face in an interview, you can prepare yourself to handle them effectively:
1. Practice, Practice, Practice
Regularly work on open-ended coding problems and system design questions. Platforms like AlgoCademy offer a wealth of resources for this kind of practice.
2. Study System Design
Many ambiguous questions in tech interviews relate to system design. Study common system design patterns and architectures to build a solid foundation.
3. Improve Your Communication Skills
Practice explaining your thought process out loud. You could do this by teaching concepts to others or participating in mock interviews.
4. Broaden Your Knowledge
Stay updated with current trends and technologies in software development. This broader knowledge can help you approach problems from different angles.
5. Develop a Problem-Solving Framework
Create a personal framework for approaching ambiguous problems. This might include steps like clarifying the question, stating assumptions, breaking down the problem, etc.
Conclusion
Dealing with ambiguous questions in interviews can be challenging, but it’s also an opportunity to showcase your problem-solving skills, creativity, and ability to work with incomplete information. By following the strategies outlined in this guide and practicing regularly, you can improve your ability to handle these types of questions effectively.
Remember, the key is not always to find the perfect solution, but to demonstrate your approach to problem-solving, your ability to communicate clearly, and your capacity to think critically under pressure. These skills are invaluable not just in interviews, but in real-world software development scenarios as well.
As you continue your journey in coding education and skills development, platforms like AlgoCademy can provide valuable resources and practice opportunities to help you prepare for technical interviews, including those tricky ambiguous questions. Keep learning, keep practicing, and approach each challenge as an opportunity to grow and improve your skills.