Understanding Essential Algorithms Beyond the Basics: A Comprehensive Guide
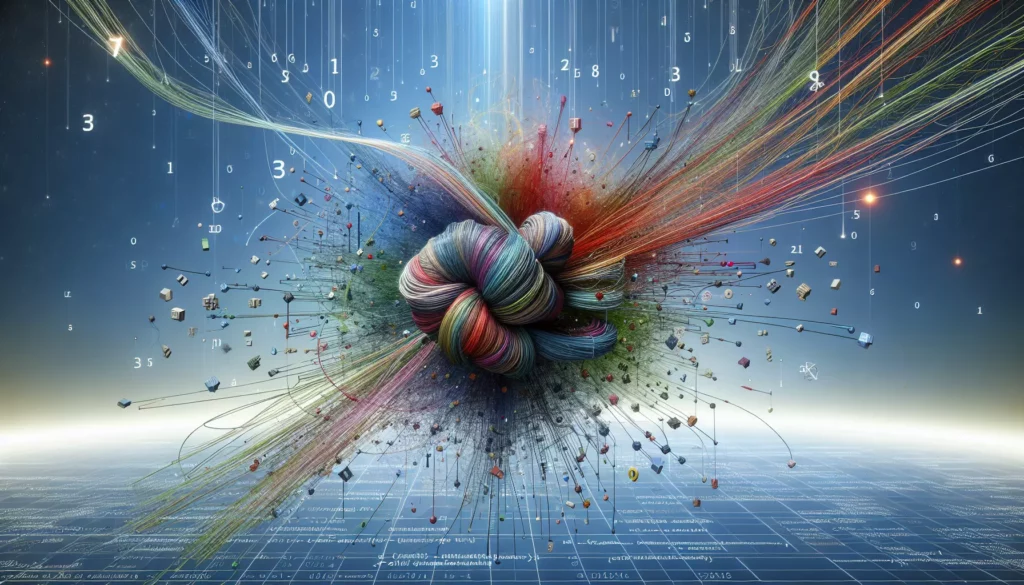
In the ever-evolving world of computer science and software development, understanding algorithms is crucial for any aspiring programmer or seasoned developer. While basic algorithms form the foundation of coding knowledge, diving deeper into more advanced algorithmic concepts can significantly enhance your problem-solving skills and make you a more efficient programmer. In this comprehensive guide, we’ll explore essential algorithms that go beyond the basics, helping you level up your coding game and prepare for technical interviews at top tech companies.
Why Advanced Algorithms Matter
Before we delve into specific algorithms, it’s important to understand why mastering advanced algorithms is vital in today’s competitive tech landscape:
- Improved Problem-Solving: Advanced algorithms provide powerful tools to tackle complex computational problems efficiently.
- Optimized Code: Understanding these algorithms allows you to write more optimized and performant code.
- Interview Preparation: Many technical interviews, especially at FAANG companies, focus heavily on algorithmic problem-solving.
- Career Advancement: Proficiency in advanced algorithms can set you apart in the job market and lead to better career opportunities.
1. Graph Algorithms
Graph algorithms are fundamental in solving a wide range of problems, from social network analysis to route planning. Let’s explore some essential graph algorithms:
1.1 Depth-First Search (DFS)
DFS is a graph traversal algorithm that explores as far as possible along each branch before backtracking. It’s useful for tasks like topological sorting and cycle detection.
def dfs(graph, start, visited=None):
if visited is None:
visited = set()
visited.add(start)
print(start)
for next in graph[start] - visited:
dfs(graph, next, visited)
return visited
1.2 Breadth-First Search (BFS)
BFS explores all the vertices of a graph in breadth-first order, visiting all the neighbors of a vertex before moving to the next level. It’s particularly useful for finding the shortest path in unweighted graphs.
from collections import deque
def bfs(graph, start):
visited = set()
queue = deque([start])
visited.add(start)
while queue:
vertex = queue.popleft()
print(vertex)
for neighbor in graph[vertex]:
if neighbor not in visited:
visited.add(neighbor)
queue.append(neighbor)
1.3 Dijkstra’s Algorithm
Dijkstra’s algorithm finds the shortest path between nodes in a graph, which may represent, for example, road networks.
import heapq
def dijkstra(graph, start):
distances = {node: float('infinity') for node in graph}
distances[start] = 0
pq = [(0, start)]
while pq:
current_distance, current_node = heapq.heappop(pq)
if current_distance > distances[current_node]:
continue
for neighbor, weight in graph[current_node].items():
distance = current_distance + weight
if distance < distances[neighbor]:
distances[neighbor] = distance
heapq.heappush(pq, (distance, neighbor))
return distances
2. Dynamic Programming
Dynamic programming is a method for solving complex problems by breaking them down into simpler subproblems. It’s particularly useful for optimization problems.
2.1 Fibonacci Sequence
While the Fibonacci sequence is a basic example, implementing it using dynamic programming showcases the power of this technique:
def fibonacci(n):
if n <= 1:
return n
fib = [0] * (n + 1)
fib[1] = 1
for i in range(2, n + 1):
fib[i] = fib[i-1] + fib[i-2]
return fib[n]
2.2 Longest Common Subsequence (LCS)
The LCS problem is a classic example of dynamic programming, often used in bioinformatics and text comparison:
def lcs(X, Y):
m, n = len(X), len(Y)
L = [[0] * (n + 1) for _ in range(m + 1)]
for i in range(1, m + 1):
for j in range(1, n + 1):
if X[i-1] == Y[j-1]:
L[i][j] = L[i-1][j-1] + 1
else:
L[i][j] = max(L[i-1][j], L[i][j-1])
return L[m][n]
3. Tree Algorithms
Trees are hierarchical data structures that are crucial in various applications. Understanding tree algorithms is essential for many programming tasks.
3.1 Binary Tree Traversal
There are three main ways to traverse a binary tree: in-order, pre-order, and post-order. Here’s an example of in-order traversal:
class TreeNode:
def __init__(self, val=0, left=None, right=None):
self.val = val
self.left = left
self.right = right
def inorder_traversal(root):
result = []
if root:
result += inorder_traversal(root.left)
result.append(root.val)
result += inorder_traversal(root.right)
return result
3.2 Lowest Common Ancestor (LCA)
Finding the LCA of two nodes in a binary tree is a common interview question and has practical applications in hierarchical structures:
def lowest_common_ancestor(root, p, q):
if not root or root == p or root == q:
return root
left = lowest_common_ancestor(root.left, p, q)
right = lowest_common_ancestor(root.right, p, q)
if left and right:
return root
return left if left else right
4. String Algorithms
String manipulation is a fundamental part of many programming tasks. Advanced string algorithms can significantly improve the efficiency of text processing operations.
4.1 Knuth-Morris-Pratt (KMP) Algorithm
The KMP algorithm is an efficient string-matching algorithm that uses the failure function to reduce the number of comparisons in the matching process:
def kmp_search(text, pattern):
m = len(pattern)
n = len(text)
# Compute failure function
failure = [0] * m
j = 0
for i in range(1, m):
while j > 0 and pattern[j] != pattern[i]:
j = failure[j-1]
if pattern[j] == pattern[i]:
j += 1
failure[i] = j
# Perform string matching
j = 0
for i in range(n):
while j > 0 and pattern[j] != text[i]:
j = failure[j-1]
if pattern[j] == text[i]:
j += 1
if j == m:
return i - m + 1 # Match found
return -1 # No match found
4.2 Rabin-Karp Algorithm
The Rabin-Karp algorithm is a string-searching algorithm that uses hashing to find patterns in strings:
def rabin_karp(text, pattern):
d = 256 # Number of characters in the input alphabet
q = 101 # A prime number
m = len(pattern)
n = len(text)
p = 0 # Hash value for pattern
t = 0 # Hash value for text
h = 1
# The value of h would be "pow(d, m-1) % q"
for i in range(m-1):
h = (h * d) % q
# Calculate the hash value of pattern and first window of text
for i in range(m):
p = (d * p + ord(pattern[i])) % q
t = (d * t + ord(text[i])) % q
# Slide the pattern over text one by one
for i in range(n - m + 1):
if p == t:
if text[i:i+m] == pattern:
return i
if i < n - m:
t = (d * (t - ord(text[i]) * h) + ord(text[i + m])) % q
if t < 0:
t += q
return -1
5. Sorting and Searching Algorithms
While basic sorting algorithms like Bubble Sort and Selection Sort are important to understand, more advanced sorting and searching algorithms offer better performance for large datasets.
5.1 Quick Sort
Quick Sort is an efficient, in-place sorting algorithm that uses divide-and-conquer strategy:
def quick_sort(arr):
if len(arr) <= 1:
return arr
pivot = arr[len(arr) // 2]
left = [x for x in arr if x < pivot]
middle = [x for x in arr if x == pivot]
right = [x for x in arr if x > pivot]
return quick_sort(left) + middle + quick_sort(right)
5.2 Merge Sort
Merge Sort is another divide-and-conquer algorithm that offers consistent O(n log n) performance:
def merge_sort(arr):
if len(arr) <= 1:
return arr
mid = len(arr) // 2
left = merge_sort(arr[:mid])
right = merge_sort(arr[mid:])
return merge(left, right)
def merge(left, right):
result = []
i, j = 0, 0
while i < len(left) and j < len(right):
if left[i] < right[j]:
result.append(left[i])
i += 1
else:
result.append(right[j])
j += 1
result.extend(left[i:])
result.extend(right[j:])
return result
5.3 Binary Search
Binary Search is an efficient algorithm for searching a sorted array:
def binary_search(arr, target):
left, right = 0, len(arr) - 1
while left <= right:
mid = (left + right) // 2
if arr[mid] == target:
return mid
elif arr[mid] < target:
left = mid + 1
else:
right = mid - 1
return -1
6. Advanced Data Structures
Understanding advanced data structures is crucial for solving complex problems efficiently. Let’s look at a couple of important ones:
6.1 Trie
A Trie, or prefix tree, is an efficient data structure for storing and searching strings:
class TrieNode:
def __init__(self):
self.children = {}
self.is_end = False
class Trie:
def __init__(self):
self.root = TrieNode()
def insert(self, word):
node = self.root
for char in word:
if char not in node.children:
node.children[char] = TrieNode()
node = node.children[char]
node.is_end = True
def search(self, word):
node = self.root
for char in word:
if char not in node.children:
return False
node = node.children[char]
return node.is_end
def starts_with(self, prefix):
node = self.root
for char in prefix:
if char not in node.children:
return False
node = node.children[char]
return True
6.2 Disjoint Set (Union-Find)
The Disjoint Set data structure is useful for problems involving set operations and connectivity:
class DisjointSet:
def __init__(self, vertices):
self.parent = {v: v for v in vertices}
self.rank = {v: 0 for v in vertices}
def find(self, item):
if self.parent[item] != item:
self.parent[item] = self.find(self.parent[item])
return self.parent[item]
def union(self, x, y):
xroot = self.find(x)
yroot = self.find(y)
if self.rank[xroot] < self.rank[yroot]:
self.parent[xroot] = yroot
elif self.rank[xroot] > self.rank[yroot]:
self.parent[yroot] = xroot
else:
self.parent[yroot] = xroot
self.rank[xroot] += 1
7. Bit Manipulation
Bit manipulation techniques can lead to highly optimized solutions for certain problems. Here are a couple of common bit manipulation operations:
7.1 Checking if a number is a power of 2
def is_power_of_two(n):
return n > 0 and (n & (n - 1)) == 0
7.2 Finding the single number in an array where every other number appears twice
def find_single_number(nums):
result = 0
for num in nums:
result ^= num
return result
Conclusion
Mastering these advanced algorithms and data structures is crucial for any programmer looking to excel in their career, especially when preparing for technical interviews at top tech companies. While this guide provides a solid foundation, remember that the key to truly understanding these concepts is through consistent practice and application.
As you continue your journey in algorithm mastery, consider using platforms like AlgoCademy that offer interactive coding tutorials and AI-powered assistance. These resources can help you apply these algorithms to real-world problems and prepare effectively for technical interviews.
Remember, the world of algorithms is vast and ever-evolving. Stay curious, keep practicing, and never stop learning. With dedication and the right resources, you’ll be well on your way to becoming a skilled algorithmic thinker and problem solver.